KPIMTextEdit::TextHTMLBuilder
#include <grantlee/texthtmlbuilder.h>
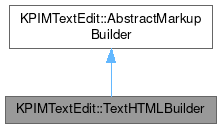
Public Member Functions | |
void | addNewline () override |
void | addSingleBreakLine () override |
void | appendLiteralText (const QString &text) override |
void | appendRawText (const QString &text) override |
void | beginAnchor (const QString &href={}, const QString &name={}) override |
void | beginBackground (const QBrush &brush) override |
void | beginEmph () override |
void | beginFontFamily (const QString &family) override |
void | beginFontPointSize (int size) override |
void | beginForeground (const QBrush &brush) override |
void | beginHeader (int level) override |
void | beginList (QTextListFormat::Style type) override |
void | beginListItem () override |
void | beginParagraph (Qt::Alignment al=Qt::AlignLeft, qreal topMargin=0.0, qreal bottomMargin=0.0, qreal leftMargin=0.0, qreal rightMargin=0.0, bool leftToRightText=false) override |
void | beginStrikeout () override |
void | beginStrong () override |
void | beginSubscript () override |
void | beginSuperscript () override |
void | beginTable (qreal cellpadding, qreal cellspacing, const QString &width) override |
void | beginTableCell (const QString &width, int colspan, int rowspan) override |
void | beginTableHeaderCell (const QString &width, int colspan, int rowspan) override |
void | beginTableRow () override |
void | beginUnderline () override |
void | endAnchor () override |
void | endBackground () override |
void | endEmph () override |
void | endFontFamily () override |
void | endFontPointSize () override |
void | endForeground () override |
void | endHeader (int level) override |
void | endList () override |
void | endListItem () override |
void | endParagraph () override |
void | endStrikeout () override |
void | endStrong () override |
void | endSubscript () override |
void | endSuperscript () override |
void | endTable () override |
void | endTableCell () override |
void | endTableHeaderCell () override |
void | endTableRow () override |
void | endUnderline () override |
QString | getResult () override |
void | insertHorizontalRule (int width=-1) override |
void | insertImage (const QString &src, qreal width, qreal height) override |
![]() | |
virtual | ~AbstractMarkupBuilder ()=default |
Detailed Description
The TextHTMLBuilder creates a clean html markup output.
This class creates html output which is as minimal as possible and restricted to the rich text features supported in Qt. (https://doc.qt.io/qt-5/richtext-html-subset.html)
The output contains only the body content, not the head element or other metadata.
eg:
instead of the content produced by Qt:
Such tags should be created separately. For example:
Font formatting information on elements is represented by individual span elements.
eg:
instead of
It my be possible to change this if necessary.
Definition at line 85 of file texthtmlbuilder.h.
Constructor & Destructor Documentation
◆ TextHTMLBuilder()
TextHTMLBuilder::TextHTMLBuilder | ( | ) |
Definition at line 35 of file texthtmlbuilder.cpp.
◆ ~TextHTMLBuilder()
|
override |
Definition at line 41 of file texthtmlbuilder.cpp.
Member Function Documentation
◆ addNewline()
|
overridevirtual |
Add a newline to the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 262 of file texthtmlbuilder.cpp.
◆ addSingleBreakLine()
|
overridevirtual |
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 473 of file texthtmlbuilder.cpp.
◆ appendLiteralText()
|
overridevirtual |
Reimplemented from AbstractMarkupBuilder.
This implementation escapes the text before appending so that
A sample <b>bold</b> word.
becomes
A sample <b>bold</b> word.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 432 of file texthtmlbuilder.cpp.
◆ appendRawText()
|
overridevirtual |
Append text
without escaping.
This is useful if extending MarkupDirector
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 459 of file texthtmlbuilder.cpp.
◆ beginAnchor()
|
overridevirtual |
Begin a url anchor element in the markup.
- Parameters
-
href The href of the anchor. name The name of the anchor.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 118 of file texthtmlbuilder.cpp.
◆ beginBackground()
|
overridevirtual |
Begin a decorarated background element in the markup (A text background color) using brush
.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 106 of file texthtmlbuilder.cpp.
◆ beginEmph()
|
overridevirtual |
Begin an emphasised element in the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 58 of file texthtmlbuilder.cpp.
◆ beginFontFamily()
|
overridevirtual |
Begin a new font family element in the markup.
- Parameters
-
family The name of the font family to begin.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 140 of file texthtmlbuilder.cpp.
◆ beginFontPointSize()
|
overridevirtual |
Begin a new font point size.
- Parameters
-
size The new size to begin.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 152 of file texthtmlbuilder.cpp.
◆ beginForeground()
|
overridevirtual |
Begin a decorarated foreground element in the markup (A text color) using brush
.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 94 of file texthtmlbuilder.cpp.
◆ beginHeader()
|
overridevirtual |
Begin a new header element.
- Parameters
-
level The new level to begin.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 202 of file texthtmlbuilder.cpp.
◆ beginList()
|
overridevirtual |
Begin a new list element in the markup.
A list element contains list items, and may contain other lists.
- Parameters
-
style The style of list to create.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 290 of file texthtmlbuilder.cpp.
◆ beginListItem()
|
overridevirtual |
Begin a new list item in the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 344 of file texthtmlbuilder.cpp.
◆ beginParagraph()
|
overridevirtual |
Begin a new paragraph.
- Parameters
-
al The new paragraph alignment topMargin The new paragraph topMargin bottomMargin The new paragraph bottomMargin leftMargin The new paragraph leftMargin rightMargin The new paragraph rightMargin
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 164 of file texthtmlbuilder.cpp.
◆ beginStrikeout()
|
overridevirtual |
Begin a struck out element in the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 82 of file texthtmlbuilder.cpp.
◆ beginStrong()
|
overridevirtual |
Begin a bold element in the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 46 of file texthtmlbuilder.cpp.
◆ beginSubscript()
|
overridevirtual |
Begin a subscript element.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 368 of file texthtmlbuilder.cpp.
◆ beginSuperscript()
|
overridevirtual |
Begin a superscript element.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 356 of file texthtmlbuilder.cpp.
◆ beginTable()
|
overridevirtual |
Begin a table element.
- Parameters
-
cellpadding The padding attribute for the table. cellspacing The spacing attribute for the table. width The width of the table. May be either an integer, or a percentage value.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 380 of file texthtmlbuilder.cpp.
◆ beginTableCell()
|
overridevirtual |
Begin a new table cell.
- Parameters
-
width The width of the cell. colSpan The column span of the cell. rowSpan The row span of the cell.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 402 of file texthtmlbuilder.cpp.
◆ beginTableHeaderCell()
|
overridevirtual |
Begin a new table header cell.
- Parameters
-
width The width of the cell. colSpan The column span of the cell. rowSpan The row span of the cell.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 396 of file texthtmlbuilder.cpp.
◆ beginTableRow()
|
overridevirtual |
Begin a new table row.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 390 of file texthtmlbuilder.cpp.
◆ beginUnderline()
|
overridevirtual |
Begin an underlined element in the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 70 of file texthtmlbuilder.cpp.
◆ endAnchor()
|
overridevirtual |
Close the anchor element.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 134 of file texthtmlbuilder.cpp.
◆ endBackground()
|
overridevirtual |
Close the decorarated background element in the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 112 of file texthtmlbuilder.cpp.
◆ endEmph()
|
overridevirtual |
Close the emphasised element in the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 64 of file texthtmlbuilder.cpp.
◆ endFontFamily()
|
overridevirtual |
End font family element.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 146 of file texthtmlbuilder.cpp.
◆ endFontPointSize()
|
overridevirtual |
End font point size element.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 158 of file texthtmlbuilder.cpp.
◆ endForeground()
|
overridevirtual |
Close the decorarated foreground element in the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 100 of file texthtmlbuilder.cpp.
◆ endHeader()
|
overridevirtual |
End a header element.
- Parameters
-
level The new level to end.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 229 of file texthtmlbuilder.cpp.
◆ endList()
|
overridevirtual |
Close the list.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 323 of file texthtmlbuilder.cpp.
◆ endListItem()
|
overridevirtual |
End the list item.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 350 of file texthtmlbuilder.cpp.
◆ endParagraph()
|
overridevirtual |
Close the paragraph in the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 256 of file texthtmlbuilder.cpp.
◆ endStrikeout()
|
overridevirtual |
Close the struck out element in the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 88 of file texthtmlbuilder.cpp.
◆ endStrong()
|
overridevirtual |
Close the bold element in the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 52 of file texthtmlbuilder.cpp.
◆ endSubscript()
|
overridevirtual |
End subscript element.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 374 of file texthtmlbuilder.cpp.
◆ endSuperscript()
|
overridevirtual |
End superscript element.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 362 of file texthtmlbuilder.cpp.
◆ endTable()
|
overridevirtual |
End a table element.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 408 of file texthtmlbuilder.cpp.
◆ endTableCell()
|
overridevirtual |
End a table cell.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 426 of file texthtmlbuilder.cpp.
◆ endTableHeaderCell()
|
overridevirtual |
End a table header cell.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 420 of file texthtmlbuilder.cpp.
◆ endTableRow()
|
overridevirtual |
End a table row.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 414 of file texthtmlbuilder.cpp.
◆ endUnderline()
|
overridevirtual |
Close the underlined element in the markup.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 76 of file texthtmlbuilder.cpp.
◆ getResult()
|
nodiscardoverridevirtual |
Return the fully marked up result of the building process.
This may contain metadata etc, such as a head element in html.
- Returns
- The fully marked up text.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 465 of file texthtmlbuilder.cpp.
◆ insertHorizontalRule()
|
overridevirtual |
Insert a horizontal rule into the markup.
- Parameters
-
width The width of the rule. Default is full width.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 268 of file texthtmlbuilder.cpp.
◆ insertImage()
|
overridevirtual |
Insert a new image element into the markup.
- Parameters
-
url The url of the image width The width of the image height The height of the image.
Implements KPIMTextEdit::AbstractMarkupBuilder.
Definition at line 277 of file texthtmlbuilder.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 12:00:02 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.