KPIMTextEdit::RichTextComposerControler
#include <richtextcomposercontroler.h>
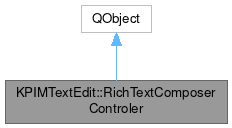
Public Member Functions | |
RichTextComposerControler (RichTextComposer *richtextComposer, QObject *parent=nullptr) | |
void | addQuotes (const QString &defaultQuote) |
bool | canDedentList () const |
bool | canIndentList () const |
RichTextComposerImages * | composerImages () const |
QString | currentLinkText () const |
QString | currentLinkUrl () const |
void | disablePainter () |
void | ensureCursorVisible () |
void | insertLink (const QString &url) |
bool | isFormattingUsed () const |
NestedListHelper * | nestedListHelper () const |
bool | painterActive () const |
RichTextComposer * | richTextComposer () const |
void | selectLinkText () const |
void | setCursorPositionFromStart (unsigned int pos) |
void | setFontForWholeText (const QFont &font) |
void | textModeChanged (KPIMTextEdit::RichTextComposer::Mode mode) |
QString | toCleanHtml () const |
QString | toCleanPlainText (const QString &plainText=QString()) const |
QString | toWrappedPlainText () const |
QString | toWrappedPlainText (QTextDocument *doc) const |
void | updateLink (const QString &linkUrl, const QString &linkText) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
bool | event (QEvent *ev) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
The RichTextComposerControler class.
Definition at line 22 of file richtextcomposercontroler.h.
Constructor & Destructor Documentation
◆ RichTextComposerControler()
|
explicit |
Definition at line 141 of file richtextcomposercontroler.cpp.
Member Function Documentation
◆ addCheckbox
|
slot |
Definition at line 159 of file richtextcomposercontroler.cpp.
◆ addQuotes()
void RichTextComposerControler::addQuotes | ( | const QString & | defaultQuote | ) |
Definition at line 796 of file richtextcomposercontroler.cpp.
◆ alignCenter
|
slot |
Definition at line 242 of file richtextcomposercontroler.cpp.
◆ alignJustify
|
slot |
Definition at line 256 of file richtextcomposercontroler.cpp.
◆ alignLeft
|
slot |
Definition at line 235 of file richtextcomposercontroler.cpp.
◆ alignRight
|
slot |
Definition at line 249 of file richtextcomposercontroler.cpp.
◆ canDedentList()
|
nodiscard |
Definition at line 589 of file richtextcomposercontroler.cpp.
◆ canIndentList()
|
nodiscard |
Definition at line 584 of file richtextcomposercontroler.cpp.
◆ composerImages()
RichTextComposerImages * RichTextComposerControler::composerImages | ( | ) | const |
Definition at line 201 of file richtextcomposercontroler.cpp.
◆ currentLinkText()
|
nodiscard |
Definition at line 460 of file richtextcomposercontroler.cpp.
◆ currentLinkUrl()
|
nodiscard |
Definition at line 455 of file richtextcomposercontroler.cpp.
◆ disablePainter()
void RichTextComposerControler::disablePainter | ( | ) |
Definition at line 189 of file richtextcomposercontroler.cpp.
◆ ensureCursorVisible()
void RichTextComposerControler::ensureCursorVisible | ( | ) |
Definition at line 657 of file richtextcomposercontroler.cpp.
◆ ensureCursorVisibleDelayed
|
slot |
Definition at line 211 of file richtextcomposercontroler.cpp.
◆ event()
|
overrideprotectedvirtual |
Reimplemented from QObject.
Definition at line 897 of file richtextcomposercontroler.cpp.
◆ indentListLess
|
slot |
Definition at line 600 of file richtextcomposercontroler.cpp.
◆ indentListMore
|
slot |
Definition at line 594 of file richtextcomposercontroler.cpp.
◆ insertHorizontalRule
|
slot |
Definition at line 221 of file richtextcomposercontroler.cpp.
◆ insertLink()
void RichTextComposerControler::insertLink | ( | const QString & | url | ) |
Definition at line 612 of file richtextcomposercontroler.cpp.
◆ isFormattingUsed()
|
nodiscard |
Definition at line 682 of file richtextcomposercontroler.cpp.
◆ makeLeftToRight
|
slot |
Definition at line 274 of file richtextcomposercontroler.cpp.
◆ makeRightToLeft
|
slot |
Definition at line 263 of file richtextcomposercontroler.cpp.
◆ manageLink
|
slot |
Definition at line 474 of file richtextcomposercontroler.cpp.
◆ nestedListHelper()
|
nodiscard |
Definition at line 206 of file richtextcomposercontroler.cpp.
◆ painterActive()
|
nodiscard |
Definition at line 154 of file richtextcomposercontroler.cpp.
◆ richTextComposer()
|
nodiscard |
Definition at line 216 of file richtextcomposercontroler.cpp.
◆ selectLinkText()
void RichTextComposerControler::selectLinkText | ( | ) | const |
Definition at line 467 of file richtextcomposercontroler.cpp.
◆ setChangeTextBackgroundColor
|
slot |
Definition at line 440 of file richtextcomposercontroler.cpp.
◆ setChangeTextForegroundColor
|
slot |
Definition at line 426 of file richtextcomposercontroler.cpp.
◆ setCursorPositionFromStart()
void RichTextComposerControler::setCursorPositionFromStart | ( | unsigned int | pos | ) |
Definition at line 646 of file richtextcomposercontroler.cpp.
◆ setFont
|
slot |
Definition at line 357 of file richtextcomposercontroler.cpp.
◆ setFontFamily
|
slot |
Definition at line 339 of file richtextcomposercontroler.cpp.
◆ setFontForWholeText()
void RichTextComposerControler::setFontForWholeText | ( | const QFont & | font | ) |
Definition at line 179 of file richtextcomposercontroler.cpp.
◆ setFontSize
|
slot |
Definition at line 348 of file richtextcomposercontroler.cpp.
◆ setHeadingLevel
|
slot |
Definition at line 384 of file richtextcomposercontroler.cpp.
◆ setListStyle
|
slot |
Definition at line 605 of file richtextcomposercontroler.cpp.
◆ setTextBackgroundColor
|
slot |
Definition at line 330 of file richtextcomposercontroler.cpp.
◆ setTextBold
|
slot |
Definition at line 285 of file richtextcomposercontroler.cpp.
◆ setTextForegroundColor
|
slot |
Definition at line 321 of file richtextcomposercontroler.cpp.
◆ setTextItalic
|
slot |
Definition at line 294 of file richtextcomposercontroler.cpp.
◆ setTextStrikeOut
|
slot |
Definition at line 312 of file richtextcomposercontroler.cpp.
◆ setTextSubScript
|
slot |
Definition at line 375 of file richtextcomposercontroler.cpp.
◆ setTextSuperScript
|
slot |
Definition at line 366 of file richtextcomposercontroler.cpp.
◆ setTextUnderline
|
slot |
Definition at line 303 of file richtextcomposercontroler.cpp.
◆ slotAddEmoticon
|
slot |
Definition at line 691 of file richtextcomposercontroler.cpp.
◆ slotAddImage
|
slot |
Definition at line 716 of file richtextcomposercontroler.cpp.
◆ slotAddQuotes
|
slot |
Definition at line 791 of file richtextcomposercontroler.cpp.
◆ slotFormatPainter
|
slot |
Definition at line 832 of file richtextcomposercontroler.cpp.
◆ slotFormatReset
|
slot |
Definition at line 736 of file richtextcomposercontroler.cpp.
◆ slotInsertHtml
|
slot |
Definition at line 697 of file richtextcomposercontroler.cpp.
◆ slotPasteAsQuotation
|
slot |
Definition at line 743 of file richtextcomposercontroler.cpp.
◆ slotPasteWithoutFormatting
|
slot |
Definition at line 755 of file richtextcomposercontroler.cpp.
◆ slotRemoveQuotes
|
slot |
Definition at line 767 of file richtextcomposercontroler.cpp.
◆ textModeChanged()
void RichTextComposerControler::textModeChanged | ( | KPIMTextEdit::RichTextComposer::Mode | mode | ) |
Definition at line 845 of file richtextcomposercontroler.cpp.
◆ toCleanHtml()
|
nodiscard |
Definition at line 545 of file richtextcomposercontroler.cpp.
◆ toCleanPlainText()
|
nodiscard |
Definition at line 852 of file richtextcomposercontroler.cpp.
◆ toWrappedPlainText() [1/2]
|
nodiscard |
Definition at line 859 of file richtextcomposercontroler.cpp.
◆ toWrappedPlainText() [2/2]
|
nodiscard |
Definition at line 865 of file richtextcomposercontroler.cpp.
◆ updateLink()
Definition at line 488 of file richtextcomposercontroler.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 12:00:02 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.