KSmtp::Session
#include <session.h>
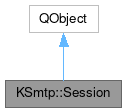
Public Types | |
enum | EncryptionMode { Unencrypted , TLS , STARTTLS } |
enum | State { Disconnected = 0 , Ready , Handshake , NotAuthenticated , Authenticated , Quitting } |
Signals | |
void | connectionError (const QString &error) |
void | stateChanged (KSmtp::Session::State state) |
Public Member Functions | |
Session (const QString &hostName, quint16 port, QObject *parent=nullptr) | |
bool | allowsDsn () const |
bool | allowsTls () const |
QStringList | availableAuthModes () const |
QString | customHostname () const |
EncryptionMode | encryptionMode () const |
QString | hostName () const |
void | open () |
quint16 | port () const |
void | quit () |
void | setCustomHostname (const QString &hostname) |
void | setEncryptionMode (EncryptionMode mode) |
void | setSocketTimeout (int ms) |
void | setUiProxy (const SessionUiProxy::Ptr &uiProxy) |
void | setUseNetworkProxy (bool useProxy) |
int | sizeLimit () const |
int | socketTimeout () const |
State | state () const |
SessionUiProxy::Ptr | uiProxy () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Member Enumeration Documentation
◆ EncryptionMode
◆ State
Enumerator | |
---|---|
Disconnected | The Session is not connected to the server. |
Ready | (internal) |
Handshake | (internal) |
NotAuthenticated | The Session is ready for login.
|
Authenticated | The Session is ready to send email.
|
Quitting | (internal) |
Constructor & Destructor Documentation
◆ Session()
Creates a new SMTP session to the specified host and port.
After creating the session, call setUseNetworkProxy() if necessary and then either open() to open the connection.
- See also
- open()
Definition at line 86 of file session.cpp.
Member Function Documentation
◆ allowsDsn()
|
nodiscard |
Returns true if the SMTP server has indicated that it allows Delivery Status Notification (DSN) support, false otherwise.
Definition at line 151 of file session.cpp.
◆ allowsTls()
|
nodiscard |
Returns true if the SMTP server has indicated that it allows TLS connections, false otherwise.
The session must be at least in the NotAuthenticated state. Before that, allowsTls() always returns false.
- See also
- KSmtp::LoginJob::setUseTls()
Definition at line 146 of file session.cpp.
◆ availableAuthModes()
|
nodiscard |
- Todo
- : return parsed auth modes, instead of strings.
Definition at line 156 of file session.cpp.
◆ customHostname()
|
nodiscard |
Definition at line 191 of file session.cpp.
◆ encryptionMode()
|
nodiscard |
Returns the requested encryption mode for this session.
Definition at line 136 of file session.cpp.
◆ hostName()
|
nodiscard |
Returns the host name that has been provided in the Session's constructor.
- See also
- port()
Definition at line 121 of file session.cpp.
◆ open()
void Session::open | ( | ) |
Opens the connection to the server.
You should connect to stateChanged() before calling this method, and wait until the session's state is NotAuthenticated (Session is ready for a LoginJob) or Disconnected (connecting to the server failed)
Make sure to call setEncryptionMode()
before.
- See also
- setEncryptionMode
Definition at line 196 of file session.cpp.
◆ port()
|
nodiscard |
Returns the port number that has been provided in the Session's constructor.
- See also
- hostName()
Definition at line 126 of file session.cpp.
◆ quit()
void Session::quit | ( | ) |
Requests the server to quit the connection.
This sends a "QUIT" command to the server and will not close the connection until it receives a response. That means you should not delete this object right after calling close, instead wait for stateChanged() to change to Disconnected.
See RFC 821, Chapter 4.1.1, "QUIT".
Definition at line 204 of file session.cpp.
◆ setCustomHostname()
void Session::setCustomHostname | ( | const QString & | hostname | ) |
Custom hostname to send in EHLO/HELO command.
Definition at line 186 of file session.cpp.
◆ setEncryptionMode()
void Session::setEncryptionMode | ( | Session::EncryptionMode | mode | ) |
Sets the encryption mode for this session.
Has to be called before open()
.
Definition at line 141 of file session.cpp.
◆ setSocketTimeout()
void Session::setSocketTimeout | ( | int | ms | ) |
Definition at line 166 of file session.cpp.
◆ setUiProxy()
void Session::setUiProxy | ( | const SessionUiProxy::Ptr & | uiProxy | ) |
Definition at line 111 of file session.cpp.
◆ setUseNetworkProxy()
void Session::setUseNetworkProxy | ( | bool | useProxy | ) |
Sets whether the SMTP network connection should use the system proxy settings.
The default is to not use the proxy.
Definition at line 106 of file session.cpp.
◆ sizeLimit()
|
nodiscard |
Returns the maximum message size in bytes that the server accepts.
You can use SendJob::size() to get the size of the message that you are trying to send
- See also
- KSmtp::SendJob::size()
Definition at line 161 of file session.cpp.
◆ socketTimeout()
|
nodiscard |
Definition at line 181 of file session.cpp.
◆ state()
|
nodiscard |
Definition at line 131 of file session.cpp.
◆ uiProxy()
|
nodiscard |
Definition at line 116 of file session.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:57:27 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.