MailCommon::FilterLog
#include <filterlog.h>
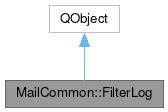
Public Types | |
enum | ContentType { Meta = 1 , PatternDescription = 2 , RuleResult = 4 , PatternResult = 8 , AppliedAction = 16 } |
Signals | |
void | logEntryAdded (const QString &entry) |
void | logShrinked () |
void | logStateChanged () |
Public Member Functions | |
~FilterLog () override | |
void | add (const QString &entry, ContentType type) |
void | addSeparator () |
void | clear () |
void | dump () |
bool | isContentTypeEnabled (ContentType type) const |
bool | isLogging () const |
QStringList | logEntries () const |
long | maxLogSize () const |
bool | saveToFile (const QString &fileName) const |
void | setContentTypeEnabled (ContentType type, bool enabled) |
void | setLogging (bool active) |
void | setMaxLogSize (long size=-1) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static FilterLog * | instance () |
static QString | recode (const QString &plain) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The filter log helps to collect log information about the filter process in KMail. It's implemented as singleton, so it's easy to direct pieces of information to a unique instance. It's possible to activate / deactivate logging. All collected log information can get thrown away, the next added log entry is the first one until another clearing. A signal is emitted whenever a new logentry is added, when the log was cleared or any log state was changed.
Definition at line 32 of file filterlog.h.
Member Enumeration Documentation
◆ ContentType
Describes the type of content that will be logged.
Enumerator | |
---|---|
Meta | Log all meta data. |
PatternDescription | Log all pattern description. |
RuleResult | Log all rule matching results. |
PatternResult | Log all pattern matching results. |
AppliedAction | Log all applied actions. |
Definition at line 50 of file filterlog.h.
Constructor & Destructor Documentation
◆ ~FilterLog()
|
overridedefault |
Destroys the filter log.
Member Function Documentation
◆ add()
Adds the given log entry
under the given content type
to the log.
Definition at line 128 of file filterlog.cpp.
◆ addSeparator()
Adds a separator line to the log.
Definition at line 145 of file filterlog.cpp.
◆ clear()
Clears the log.
Definition at line 150 of file filterlog.cpp.
◆ dump()
◆ instance()
|
static |
Returns the single global instance of the filter log.
Definition at line 71 of file filterlog.cpp.
◆ isContentTypeEnabled()
|
nodiscard |
Returns whether the given content type
is enabled for logging.
Definition at line 123 of file filterlog.cpp.
◆ isLogging()
|
nodiscard |
Returns whether the filter log is currently active.
Definition at line 80 of file filterlog.cpp.
◆ logEntries()
|
nodiscard |
Returns the list of log entries.
Definition at line 156 of file filterlog.cpp.
◆ logEntryAdded
|
signal |
This signal is emitted whenever a new entry
has been added to the log.
◆ logShrinked
|
signal |
This signal is emitted whenever the log has shrunk.
◆ logStateChanged
|
signal |
This signal is emitted whenever the activity of the filter log has been changed.
◆ maxLogSize()
|
nodiscard |
Returns the maximum size of the log in bytes.
Definition at line 107 of file filterlog.cpp.
◆ recode()
|
staticnodiscard |
Returns an escaped version of the log which can be used in a HTML document.
Definition at line 188 of file filterlog.cpp.
◆ saveToFile()
Saves the log to the file with the given fileName
.
- Returns
true
on success orfalse
on failure.
Definition at line 170 of file filterlog.cpp.
◆ setContentTypeEnabled()
Sets whether a given content type
will be enabled
for logging.
Definition at line 112 of file filterlog.cpp.
◆ setLogging()
Sets whether the filter log is currently active
.
Definition at line 85 of file filterlog.cpp.
◆ setMaxLogSize()
Sets the maximum size
of the log in bytes.
Definition at line 91 of file filterlog.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 4 2025 12:00:27 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.