KChartEnums
#include <KChartEnums.h>
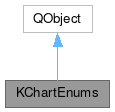
Public Types | |
enum | GranularitySequence { GranularitySequence_10_20 , GranularitySequence_10_50 , GranularitySequence_25_50 , GranularitySequence_125_25 , GranularitySequenceIrregular } |
enum | MeasureCalculationMode { MeasureCalculationModeAbsolute , MeasureCalculationModeRelative , MeasureCalculationModeAuto , MeasureCalculationModeAutoArea , MeasureCalculationModeAutoOrientation } |
enum | MeasureOrientation { MeasureOrientationAuto , MeasureOrientationHorizontal , MeasureOrientationVertical , MeasureOrientationMinimum , MeasureOrientationMaximum } |
enum | PositionValue { PositionUnknown = 0 , PositionCenter = 1 , PositionNorthWest = 2 , PositionNorth = 3 , PositionNorthEast = 4 , PositionEast = 5 , PositionSouthEast = 6 , PositionSouth = 7 , PositionSouthWest = 8 , PositionWest = 9 , PositionFloating =10 } |
enum | TextLayoutPolicy { LayoutJustOverwrite , LayoutPolicyRotate , LayoutPolicyShiftVertically , LayoutPolicyShiftHorizontally , LayoutPolicyShrinkFontSize } |
Static Public Member Functions | |
static QString | granularitySequenceToString (GranularitySequence sequence) |
static QString | layoutPolicyToString (TextLayoutPolicy type) |
static QString | measureCalculationModeToString (MeasureCalculationMode mode) |
static QString | measureOrientationToString (MeasureOrientation mode) |
static GranularitySequence | stringToGranularitySequence (const QString &string) |
static TextLayoutPolicy | stringToLayoutPolicy (const QString &string) |
static MeasureCalculationMode | stringToMeasureCalculationMode (const QString &string) |
static MeasureOrientation | stringToMeasureOrientation (const QString &string) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Project global class providing some enums needed both by KChartParams and by KChartCustomBox.
Definition at line 26 of file KChartEnums.h.
Member Enumeration Documentation
◆ GranularitySequence
GranularitySequence specifies the values, that may be applied, to determine a step width within a given data range.
- Note
- Granularity with can be set for Linear axis calculation mode only, there is no way to specify a step width for Logarithmic axes.
Value occurring in the GranularitySequence names only are showing their respective relation ship. For real data they will most times not be used directly, but be multiplied by positive (or negative, resp.) powers of ten.
A granularity sequence is a sequence of values from the following set: 1, 1.25, 2, 2.5, 5.
The reason for using one of the following three pre-defined granularity sequences (instead of just using the best matching step width) is to follow a simple rule: If scaling becomes finer (== smaller step width) no value, that has been on a grid line before, shall loose its line and be NOT on a grid line anymore!
This means: Smaller step width may not remove any grid lines, but it may add additional lines in between.
GranularitySequence_10_20
Step widths can be 1, or 2, but they never can be 2.5 nor 5, nor 1.25.GranularitySequence_10_50
Step widths can be 1, or 5, but they never can be 2, nor 2.5, nor 1.25.GranularitySequence_25_50
Step widths can be 2.5, or 5, but they never can be 1, nor 2, nor 1.25.GranularitySequence_125_25
Step widths can be 1.25 or 2.5 but they never can be 1, nor 2, nor 5.GranularitySequenceIrregular
Step widths can be all of these values: 1, or 1.25, or 2, or 2.5, or 5.
- Note
- When ever possible, try to avoid using GranularitySequenceIrregular! Allowing all possible step values, using this granularity sequence involves a serious risk: Your users might be irritated due to 'jumping' grid lines, when step size is changed from 2.5 to 2 (or vice versa, resp.). In case you still want to use GranularitySequenceIrregular just make sure to NOT draw any sub-grid lines, because in most cases you will get not-matching step widths for the sub-grid. In short: GranularitySequenceIrregular can safely be used if your data range is not changing at all AND (b) you will not allow the coordinate plane to be zoomed AND (c) you are not displaying any sub-grid lines.
Since you probably like having the value 1 as an allowed step width, the granularity sequence decision boils down to a boolean question:
- To get ten divided by five you use GranularitySequence_10_20, while
- for having it divided by two GranularitySequence_10_50 is your choice.
Definition at line 78 of file KChartEnums.h.
◆ MeasureCalculationMode
Measure calculation mode: the way how the absolute value of a KChart::Measure is determined during KChart's internal geometry calculation time.
KChart::Measure values either are relative (calculated in relation to a given AbstractArea), or they are absolute (used as fixed values).
Values stored in relative measure always are interpreted as per-mille of a reference area's height (or width, resp.) depending on the orientation set for the KChart::Measure.
MeasureCalculationModeAbsolute
Value set by setValue() is absolute, to be used unchanged.MeasureCalculationModeRelative
Value is relative, the reference area is specified by setReferenceArea(), and orientation specified by setOrientation().MeasureCalculationModeAuto
Value is relative, KChart will automatically determine which reference area to use, and it will determine the orientation too.MeasureCalculationModeAutoArea
Value is relative, Orientation is specified by setOrientation(), and KChart will automatically determine which reference area to use.MeasureCalculationModeAutoOrientation
Value is relative, Area is specified by setReferenceArea(), and KChart will automatically determine which orientation to use.
- See also
- KChart::Measure::setCalculationMode
Definition at line 211 of file KChartEnums.h.
◆ MeasureOrientation
Measure orientation mode: the way how the absolute value of a KChart::Measure is determined during KChart's internal geometry calculation time.
KChart::Measure values either are relative (calculated in relation to a given AbstractArea), or they are absolute (used as fixed values).
Values stored in relative measure take into account the width (and/or the height, resp.) of a so-called reference area, that is either specified by KChart::Measure::setReferenceArea, or determined by KChart automatically, respectively.
MeasureOrientationAuto
Value is calculated, based upon the width (or on the height, resp.) of the reference area: KChart will automatically determie an appropriate way.MeasureOrientationHorizontal
Value is calculated, based upon the width of the reference area.MeasureOrientationVertical
Value is calculated, based upon the height of the reference area.MeasureOrientationMinimum
Value is calculated, based upon the width (or on the height, resp.) of the reference area - which ever is smaller.MeasureOrientationMaximum
Value is calculated, based upon the width (or on the height, resp.) of the reference area - which ever is smaller.
- See also
- KChart::Measure::setOrientationMode
Definition at line 282 of file KChartEnums.h.
◆ PositionValue
Numerical values of the static KChart::Position instances, for using a Position::value() with a switch () statement.
- See also
- Position
Definition at line 180 of file KChartEnums.h.
◆ TextLayoutPolicy
Text layout policy: what to do if text that is to be drawn would cover neighboring text or neighboring areas.
LayoutJustOverwrite
Just ignore the layout collision and write the text nevertheless.LayoutPolicyRotate
Try counter-clockwise rotation to make the text fit into the space.LayoutPolicyShiftVertically
Shift the text baseline upwards (or downwards, resp.) and draw a connector line between the text and its anchor.LayoutPolicyShiftHorizontally
Shift the text baseline to the left (or to the right, resp.) and draw a connector line between the text and its anchor.LayoutPolicyShrinkFontSize
Reduce the text font size.
- See also
- KChartParams::setPrintDataValues
Definition at line 147 of file KChartEnums.h.
Member Function Documentation
◆ granularitySequenceToString()
|
inlinestatic |
Converts the specified granularity sequence enum to a string representation.
- Parameters
-
sequence the granularity sequence enum to convert
- Returns
- the string representation of the granularity sequence
Definition at line 95 of file KChartEnums.h.
◆ layoutPolicyToString()
|
static |
Converts the specified text layout policy enum to a string representation.
- Parameters
-
type the text layout policy to convert
- Returns
- the string representation of the text layout policy enum
◆ measureCalculationModeToString()
|
inlinestatic |
Converts the specified measure calculation mode enum to a string representation.
- Parameters
-
mode the measure calculation mode to convert
- Returns
- the string representation of the Measure calculation mode enum
Definition at line 227 of file KChartEnums.h.
◆ measureOrientationToString()
|
inlinestatic |
Converts the specified measure orientation enum to a string representation.
- Parameters
-
mode the measure orientation to convert
- Returns
- the string representation of the measure orientation enum
Definition at line 298 of file KChartEnums.h.
◆ stringToGranularitySequence()
|
inlinestatic |
Converts the specified string to a granularity sequence enum value.
- Parameters
-
string the string to convert
- Returns
- the granularity sequence enum value
Definition at line 119 of file KChartEnums.h.
◆ stringToLayoutPolicy()
|
static |
Converts the specified string to a text layout policy enum value.
- Parameters
-
string the string to convert
- Returns
- the text layout policy enum value
◆ stringToMeasureCalculationMode()
|
inlinestatic |
Converts the specified string to a measure calculation mode enum value.
- Parameters
-
string the string to convert
- Returns
- the measure calculation mode enum value
Definition at line 251 of file KChartEnums.h.
◆ stringToMeasureOrientation()
|
inlinestatic |
Converts the specified string to a measure orientation enum value.
- Parameters
-
string the string to convert
- Returns
- the measure orientation enum value
Definition at line 322 of file KChartEnums.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:49:52 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.