kviewshell
ArrayRep Class Reference
#include <Arrays.h>
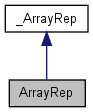
Public Member Functions | |
ArrayRep (const ArrayRep &rep) | |
ArrayRep (int elsize, void(*xdestroy)(void *, int, int), void(*xinit1)(void *, int, int), void(*xinit2)(void *, int, int, const void *, int, int), void(*xcopy)(void *, int, int, const void *, int, int), void(*xinsert)(void *, int, int, const void *, int), int lobound, int hibound) | |
ArrayRep (int elsize, void(*xdestroy)(void *, int, int), void(*xinit1)(void *, int, int), void(*xinit2)(void *, int, int, const void *, int, int), void(*xcopy)(void *, int, int, const void *, int, int), void(*xinsert)(void *, int, int, const void *, int), int hibound) | |
ArrayRep (int elsize, void(*xdestroy)(void *, int, int), void(*xinit1)(void *, int, int), void(*xinit2)(void *, int, int, const void *, int, int), void(*xcopy)(void *, int, int, const void *, int, int), void(*xinsert)(void *, int, int, const void *, int)) | |
void | del (int n, unsigned int howmany=1) |
void | ins (int n, const void *what, unsigned int howmany) |
ArrayRep & | operator= (const ArrayRep &rep) |
void | resize (int lobound, int hibound) |
void | shift (int disp) |
virtual | ~ArrayRep () |
Arrays.h | |
Files #"Arrays.h"# and #"Arrays.cpp"# implement three array template classes. Class {TArray} implements an array of objects of trivial types such as char#, int#, float#, etc. It is faster than general implementation for any type done in {DArray} because it does not cope with element's constructors, destructors and copy operators. Although implemented as a template, which makes it possible to incorrectly use {TArray} with non-trivial classes, it should not be done. A lot of things is shared by these three arrays. That is why there are more base classes: {itemize} {ArrayBase} defines functions independent of the elements type {ArrayBaseT} template class defining functions shared by {DArray} and {TArray} {itemize} The main difference between {GArray} (now obsolete) and these ones is the copy-on-demand strategy, which allows you to copy array objects without copying the real data. It's the same thing, which has been implemented in {GString} long ago: as long as you don't try to modify the underlying data, it may be shared between several copies of array objects. As soon as you attempt to make any changes, a private copy is created automatically and transparently for you - the procedure, that we call "copy-on-demand". Also, please note that now there is no separate class, which does fast sorting. Both {TArray} (dynamic array for trivial types) and {DArray} (dynamic array for arbitrary types) can sort their elements. { Historical comments} --- Leon chose to implement his own arrays because the STL classes were not universally available and the compilers were rarely able to deal with such a template galore. Later it became clear that there is no really good reason why arrays should be derived from containers. It was also suggested to create separate arrays implementation for simple classes and do the copy-on-demand strategy, which would allow to assign array objects without immediate copying of their elements. At this point {DArray} and {TArray} should only be used when it is critical to have the copy-on-demand feature. The {GArray} implementation is a lot more efficient. Template array classes.
| |
void | empty () |
int | hbound () const |
int | lbound () const |
int | size () const |
void | touch (int n) |
Public Attributes | |
void * | data |
int | elsize |
int | hibound |
int | lobound |
int | maxhi |
int | minlo |
Detailed Description
Definition at line 185 of file Arrays.h.
Constructor & Destructor Documentation
ArrayRep::ArrayRep | ( | int | elsize, | |
void(*)(void *, int, int) | xdestroy, | |||
void(*)(void *, int, int) | xinit1, | |||
void(*)(void *, int, int, const void *, int, int) | xinit2, | |||
void(*)(void *, int, int, const void *, int, int) | xcopy, | |||
void(*)(void *, int, int, const void *, int) | xinsert | |||
) |
Definition at line 75 of file Arrays.cpp.
ArrayRep::ArrayRep | ( | int | elsize, | |
void(*)(void *, int, int) | xdestroy, | |||
void(*)(void *, int, int) | xinit1, | |||
void(*)(void *, int, int, const void *, int, int) | xinit2, | |||
void(*)(void *, int, int, const void *, int, int) | xcopy, | |||
void(*)(void *, int, int, const void *, int) | xinsert, | |||
int | hibound | |||
) |
Definition at line 87 of file Arrays.cpp.
ArrayRep::ArrayRep | ( | int | elsize, | |
void(*)(void *, int, int) | xdestroy, | |||
void(*)(void *, int, int) | xinit1, | |||
void(*)(void *, int, int, const void *, int, int) | xinit2, | |||
void(*)(void *, int, int, const void *, int, int) | xcopy, | |||
void(*)(void *, int, int, const void *, int) | xinsert, | |||
int | lobound, | |||
int | hibound | |||
) |
Definition at line 100 of file Arrays.cpp.
ArrayRep::ArrayRep | ( | const ArrayRep & | rep | ) |
Definition at line 113 of file Arrays.cpp.
ArrayRep::~ArrayRep | ( | ) | [virtual] |
Definition at line 122 of file Arrays.cpp.
Member Function Documentation
void ArrayRep::del | ( | int | n, | |
unsigned int | howmany = 1 | |||
) |
Definition at line 214 of file Arrays.cpp.
void ArrayRep::ins | ( | int | n, | |
const void * | what, | |||
unsigned int | howmany | |||
) |
Definition at line 227 of file Arrays.cpp.
Definition at line 130 of file Arrays.cpp.
void ArrayRep::resize | ( | int | lobound, | |
int | hibound | |||
) |
Definition at line 141 of file Arrays.cpp.
void ArrayRep::shift | ( | int | disp | ) |
Definition at line 205 of file Arrays.cpp.
Member Data Documentation
void* ArrayRep::data |
int ArrayRep::elsize |
int ArrayRep::maxhi |
int ArrayRep::minlo |
The documentation for this class was generated from the following files: