kviewshell
EmptyRenderer Class Reference
#include <emptyRenderer.h>
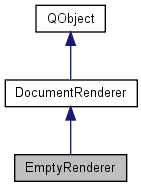
Public Member Functions | |
void | drawPage (double res, RenderedDocumentPage *page) |
EmptyRenderer (QWidget *parent) | |
virtual bool | setFile (const QString &, const KURL &) |
Detailed Description
Definition at line 29 of file emptyRenderer.h.
Constructor & Destructor Documentation
EmptyRenderer::EmptyRenderer | ( | QWidget * | parent | ) |
Definition at line 24 of file emptyRenderer.cpp.
Member Function Documentation
void EmptyRenderer::drawPage | ( | double | resolution, | |
RenderedDocumentPage * | page | |||
) | [inline, virtual] |
rendering of documents
This purely virtual method is the most important method in the DocumentRenderer class. It must be re-implemented by authors who want to write plugins for the kviewshell program. The purpose of this method is to render a graphical representation into a documentPage structure. More specifically, the implementation needs to
- call the documentPage::clear() on *page
and the do all of the following, in no particular order
- obtain the pointer to the QPaintDevice from the documentPage using the documentPage::getPaintDevice() method and draw a graphical representation of the page number page->getPageNumber() into the QPaintDevice, using the given resolution. If the member accessibilityBackground is true, the accessibilityBackgroundColor should be used for a background color, if possible. Otherwise, white should be used, if possible. If you need to compute the size of the page in pixel, do this as follows: Don't use page->height() or page->width() to calculate the sizes ---KViewShell uses transformations e.g. to rotate the page, and sets the argument 'resolution' accordingly; these changes are not reflected in page->height() and page->width(). Similar problems occur if KViewShell required a shrunken version of the page, e.g. to print multiple pages on one sheet of paper.
SimplePageSize psize = pageSizes[page->getPageNumber() - 1]; if (psize.isValid()) { int width_in_pixel = resolution * psize.width().getLength_in_inch(); int height_in_pixel = resolution * psize.height().getLength_in_inch(); <...> }
- if your document contains hyperlinks, fill the documentPage::hyperLinkList with HyperLinks, using pixel coordinates for the coordinates in the Hyperlink::box member of the Hyperlink. The Hyperlink::baseline member of the Hyperlink can be ignored. The linkText member of the Hyperlink should either be an absolute URL ("http://www.kde.org"), or be of the form "#anch", where the string "anch" is contained in the anchorList.
- if your plugin supports full-text information, fill documentPage::textLinkList with HyperLinks, using pixel coordinates for the coordinates in the Hyperlink::box and Hyperlink::baseline members of the Hyperlink. The entries in the documentPage::textLinkList should have a natural ordering, "first text first" (left-to-right, up-to-down for western languages, right-to-left for hebrew, etc.). This is important so that text selection with the mouse works properly, and only continuous blocks of text can be selected.
- Note:
- This method is often called in a paintEvent, so that care must be taken to return as soon as possible. No user interaction should be done during the execution.
If your plugin supports full-text information, you probably want to re-implement the method supportsTextSearch() below.
- Warning:
- As mentioned above, it may be tempting to compute the image size in pixel, using page->height() and page->width(). DON'T DO THAT. KViewShell uses transformations e.g. to rotate the page, and sets the argument 'resolution' accordingly; these changes are not reflected in page->height() and page->width(). Similar problems occur if KViewShell required a shrunken version of the page, e.g. to print multiple pages on one sheet of paper.
The signal documentIsChanged() must not be emitted while the method runs.
Future versions of kviewshell will use threading to keep the GUI responsive while pages are rendered. As a result, IT IS ABSOLUTELY NECESSARY that your implementation is THREAD-SAFE, if not, this can result in infrequent and very hard-to-find crashes of your programm. Use the member mutex to make your implemetation thread-safe.
- Parameters:
-
resolution this argument contains the resolution of the display device. In principle. In fact, kviewshell implements zooming by using values that are not exactly the resolution of the display, but multiplied with the zoom factor. Bottom line: the DocumentRenderer should act as if this field indeed contains resolution of the display device. page pointer to a documentPage structure that this method rendered into.
Implements DocumentRenderer.
Definition at line 38 of file emptyRenderer.h.
virtual bool EmptyRenderer::setFile | ( | const QString & | fname, | |
const KURL & | base | |||
) | [inline, virtual] |
loading of files
This is a purely virtual method that must be re-implemented. It is called when a file should be loaded. The implementation must then do the following
- initialize internal data structures so the document pointed to by 'fname' can be rendered quickly. It is not necessary actually load the file; if the implementation choses to load only parts of a large file and leave the rest on the disc, this is perfectly fine.
- return 'true' on success and 'false' on failure. Before 'false' is returned, the method clear() should be called
When the method returns 'true', it is expected that
- the member _isModified is set to 'false'
- the member 'numPages' is either set to 0 if the document is empty, or else to the number of page in the document
- the vector pageSizes *must* either be empty (e.g. if your file format does not specify a page size), or must be of size totalPages(), and contain the sizes of all pages in the document.
- the anchorList is filled with data; it is perfectly fine to leave the anchorList empty, if your file format does not support anchors, or if your document doesn't contain any.
- the list 'bookmarks' is filled with data; it is perfectly fine to leave this list empty, if your file format does not support bookmarks or if your document doesn't contain any.
- the method drawPage() works
- Note:
- It is perfectly possible that setFile() is called several times in a row, with the same or with different filenames.
- Warning:
- The signal documentIsChanged() must not be emitted while the method runs.
Future versions of kviewshell will use threading to keep the GUI responsive while pages are rendered. As a result, IT IS ABSOLUTELY NECESSARY that your implementation is THREAD-SAFE, if not, this can result in infrequent and very hard-to-find crashes of your programm. Use the member mutex to make your implemetation thread-safe.
- Parameters:
-
fname name of the file to open. It is not guaranteed that the file exists, that it is a file, or that it is readable. base original URL of the file that was opened.
- Returns:
- 'true' on success and 'false' on failure. Even after this method returns 'false' the class must act reasonably, i.e. by clear()ing the document
Implements DocumentRenderer.
Definition at line 36 of file emptyRenderer.h.
The documentation for this class was generated from the following files: