kviewshell
GArrayTemplate< TYPE > Class Template Reference
Common base class for all dynamic arrays. More...
#include <GContainer.h>
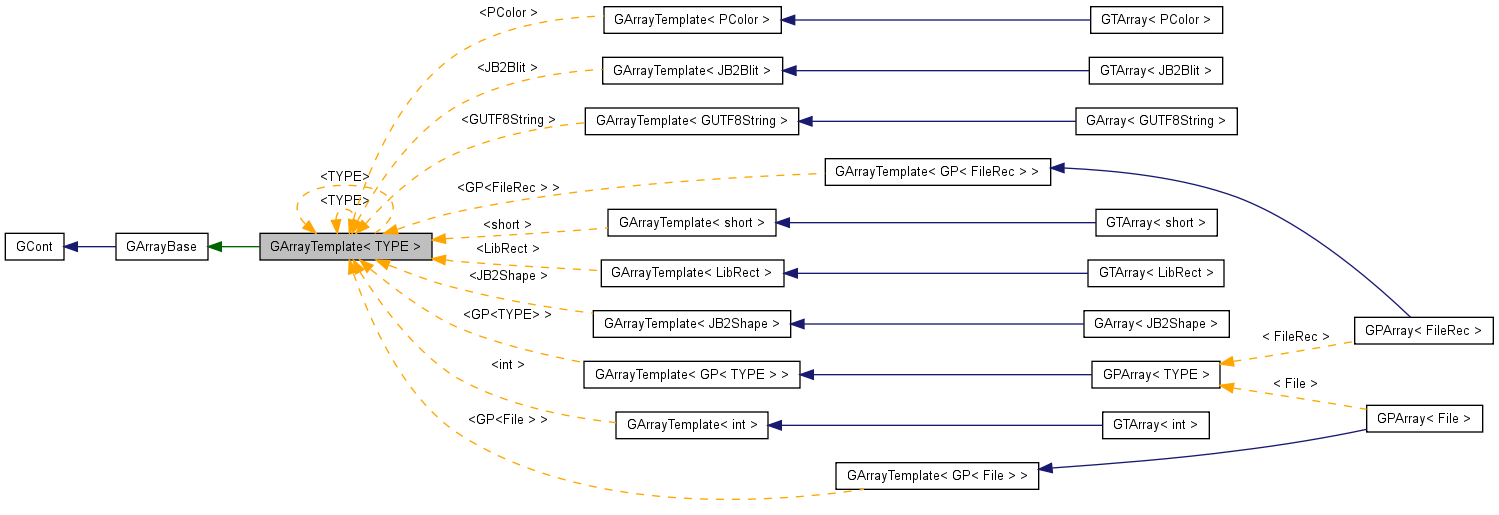
Public Member Functions | |
void | del (int n, int howmany=1) |
void | empty () |
GArrayTemplate (const Traits &traits, int lobound, int hibound) | |
GArrayTemplate (const Traits &traits) | |
int | hbound () const |
void | ins (int n, const TYPE &val, int howmany=1) |
void | ins (int n, int howmany=1) |
int | lbound () const |
operator const TYPE * () | |
operator const TYPE * () const | |
operator TYPE * () | |
const TYPE & | operator[] (int n) const |
TYPE & | operator[] (int const n) |
void | resize (int lobound, int hibound) |
void | resize (int hibound) |
void | shift (int disp) |
int | size () const |
void | sort (int lo, int hi) |
void | sort () |
void | steal (GArrayTemplate &ga) |
void | touch (int n) |
Detailed Description
template<class TYPE>
class GArrayTemplate< TYPE >
Common base class for all dynamic arrays.
Class {GArrayTemplate} implements all methods for manipulating arrays of type TYPE#. You should not however create instances of this class. You should instead use class {GArray}, {GTArray} or {GPArray}.
Definition at line 350 of file GContainer.h.
Constructor & Destructor Documentation
GArrayTemplate< TYPE >::GArrayTemplate | ( | const Traits & | traits | ) | [inline] |
Definition at line 354 of file GContainer.h.
GArrayTemplate< TYPE >::GArrayTemplate | ( | const Traits & | traits, | |
int | lobound, | |||
int | hibound | |||
) | [inline] |
Definition at line 355 of file GContainer.h.
Member Function Documentation
void GArrayTemplate< TYPE >::del | ( | int | n, | |
int | howmany = 1 | |||
) | [inline] |
Deletes array elements.
The array elements corresponding to subscripts n#...n+howmany-1# are destroyed. All array elements previously located at subscripts greater or equal to n+howmany# are moved to subscripts starting with n#. The new subscript upper bound is reduced in order to account for this shift.
Reimplemented from GArrayBase.
Definition at line 442 of file GContainer.h.
void GArrayTemplate< TYPE >::empty | ( | ) | [inline] |
Erases the array contents.
All elements in the array are destroyed. The valid subscript range is set to the empty range.
Reimplemented from GArrayBase.
Definition at line 402 of file GContainer.h.
int GArrayTemplate< TYPE >::hbound | ( | ) | const [inline] |
void GArrayTemplate< TYPE >::ins | ( | int | n, | |
const TYPE & | val, | |||
int | howmany = 1 | |||
) | [inline] |
Insert new elements into an array.
The new elements are constructed by copying element val# using the copy constructor for type TYPE#. See {ins(int n, unsigned int howmany=1)}.
Definition at line 456 of file GContainer.h.
void GArrayTemplate< TYPE >::ins | ( | int | n, | |
int | howmany = 1 | |||
) | [inline] |
Insert new elements into an array.
This function inserts howmany# elements at position n# into the array. These elements are constructed using the default constructor for type TYPE#. All array elements previously located at subscripts n# and higher are moved to subscripts n+howmany# and higher. The upper bound of the valid subscript range is increased in order to account for this shift.
Definition at line 451 of file GContainer.h.
int GArrayTemplate< TYPE >::lbound | ( | ) | const [inline] |
GArrayTemplate< TYPE >::operator const TYPE * | ( | ) | [inline] |
Definition at line 397 of file GContainer.h.
GArrayTemplate< TYPE >::operator const TYPE * | ( | ) | const [inline] |
Returns a pointer for reading (but not modifying) the array elements.
This pointer can be used to access the array elements with the same subscripts and the usual bracket syntax. This pointer remains valid as long as the valid subscript range is unchanged. If you change the subscript range, you must stop using the pointers returned by prior invocation of this conversion operator.
Definition at line 395 of file GContainer.h.
GArrayTemplate< TYPE >::operator TYPE * | ( | ) | [inline] |
Returns a pointer for reading or writing the array elements.
This pointer can be used to access the array elements with the same subscripts and the usual bracket syntax. This pointer remains valid as long as the valid subscript range is unchanged. If you change the subscript range, you must stop using the pointers returned by prior invocation of this conversion operator.
Definition at line 387 of file GContainer.h.
const TYPE& GArrayTemplate< TYPE >::operator[] | ( | int | n | ) | const [inline] |
Returns a constant reference to the array element for subscript n#.
This reference can only be used for reading (as "#a[n]#") an array element. This operation will not extend the valid subscript range: an exception {GException} is thrown if argument n# is not in the valid subscript range. This variant of operator[]# is necessary when dealing with a const GArray<TYPE>#.
TYPE& GArrayTemplate< TYPE >::operator[] | ( | int const | n | ) | [inline] |
Returns a reference to the array element for subscript n#.
This reference can be used for both reading (as "#a[n]#") and writing (as "#a[n]=v#") an array element. This operation will not extend the valid subscript range: an exception {GException} is thrown if argument n# is not in the valid subscript range.
void GArrayTemplate< TYPE >::resize | ( | int | lobound, | |
int | hibound | |||
) | [inline] |
Resets the valid subscript range to lobound#---hibound#.
This function may destroy some array elements and may construct new array elements with the null constructor. Setting lobound# to #0# and hibound# to #-1# resets the valid subscript range to the empty range.
Reimplemented from GArrayBase.
Definition at line 430 of file GContainer.h.
void GArrayTemplate< TYPE >::resize | ( | int | hibound | ) | [inline] |
Resets the valid subscript range to #0#---hibound#.
This function may destroy some array elements and may construct new array elements with the null constructor. Setting hibound# to #-1# resets the valid subscript range to the empty range.
Definition at line 424 of file GContainer.h.
void GArrayTemplate< TYPE >::shift | ( | int | disp | ) | [inline] |
Shifts the valid subscript range.
Argument disp# is added to both bounds of the valid subscript range. Array elements previously located at subscript x# will now be located at subscript x+disp#.
Reimplemented from GArrayBase.
Definition at line 435 of file GContainer.h.
int GArrayTemplate< TYPE >::size | ( | void | ) | const [inline] |
void GArrayTemplate< TYPE >::sort | ( | int | lo, | |
int | hi | |||
) |
Sort array elements in subscript range lo# to hi#.
Sort all array elements whose subscripts are in range lo# to hi# in ascending order according to the less-or-equal comparison operator for type TYPE#. The other elements of the array are left untouched. An exception is thrown if arguments lo# and hi# are not in the valid subscript range.
void GArrayTemplate< TYPE >::sort | ( | ) | [inline] |
Sort array elements.
Sort all array elements in ascending order according to the less-or-equal comparison operator for type TYPE#.
Definition at line 466 of file GContainer.h.
void GArrayTemplate< TYPE >::steal | ( | GArrayTemplate< TYPE > & | ga | ) | [inline] |
Steals contents from array ga#.
After this call, array ga# is empty, and this array contains everything previously contained in ga#.
Definition at line 460 of file GContainer.h.
void GArrayTemplate< TYPE >::touch | ( | int | n | ) | [inline] |
Extends the subscript range so that it contains n#.
This function does nothing if n# is already int the valid subscript range. If the valid range was empty, both the lower bound and the upper bound are set to n#. Otherwise the valid subscript range is extended to encompass n#. This function is very handy when called before setting an array element: {verbatim} int lineno=1; GArray<GString> a; while (! end_of_file()) { a.touch(lineno); a[lineno++] = read_a_line(); } {verbatim}
Reimplemented from GArrayBase.
Definition at line 418 of file GContainer.h.
The documentation for this class was generated from the following file: