kviewshell
GListTemplate< TYPE, TI > Class Template Reference
Common base class for all doubly linked lists. More...
#include <GContainer.h>
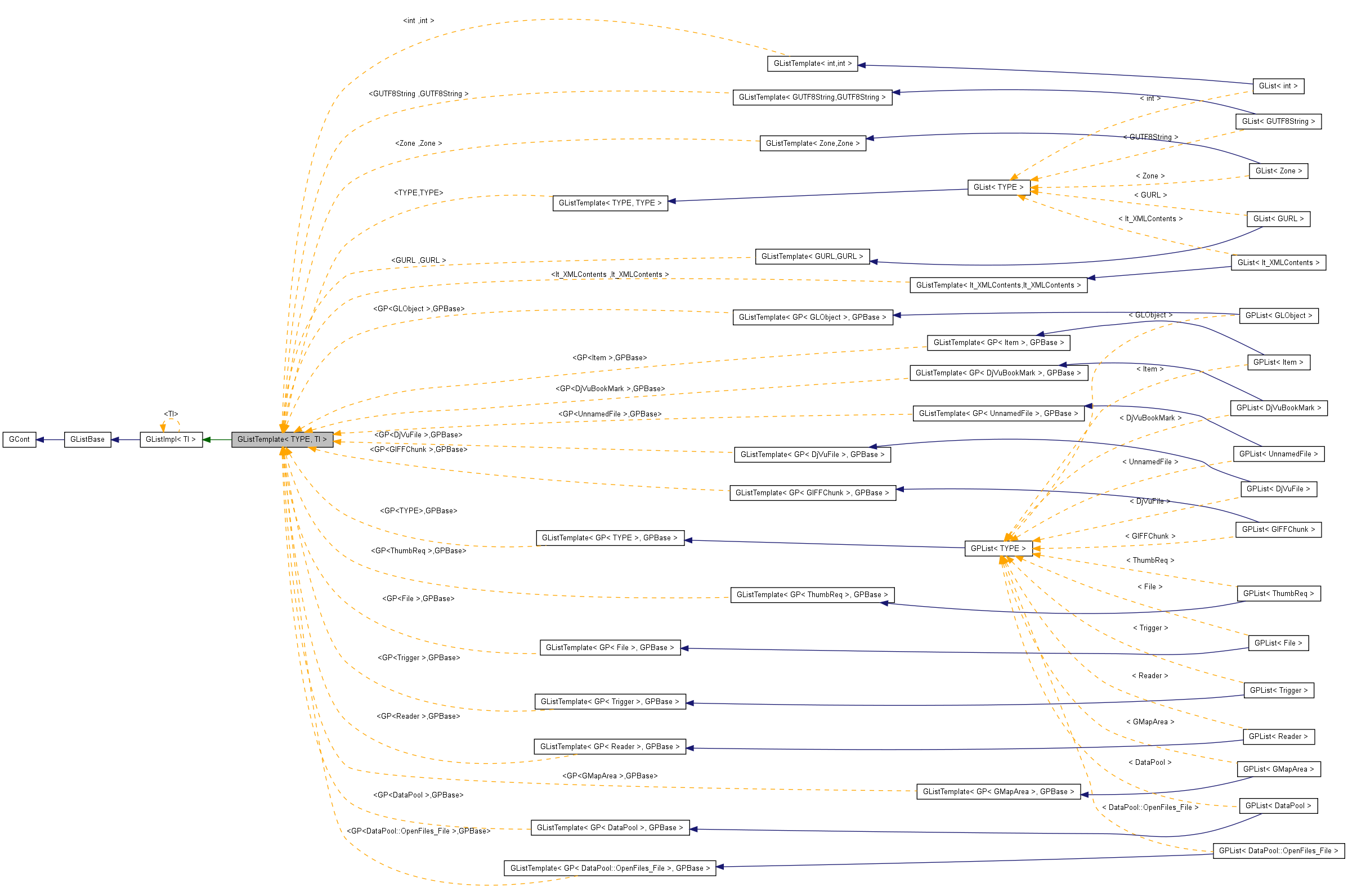
Public Member Functions | |
void | append (const TYPE &elt) |
GPosition | contains (const TYPE &elt) const |
void | del (GPosition &pos) |
void | empty () |
void | first (GPosition &pos) const |
GPosition | firstpos () const |
void | insert_after (GPosition pos, const TYPE &elt) |
void | insert_before (GPosition pos, GListTemplate< TYPE, TI > &fromlist, GPosition &frompos) |
void | insert_before (GPosition pos, const TYPE &elt) |
int | isempty () const |
void | last (GPosition &pos) const |
GPosition | lastpos () const |
TYPE * | next (GPosition &pos) |
const TYPE * | next (GPosition &pos) const |
int | nth (unsigned int n, GPosition &pos) const |
GPosition | nth (unsigned int n) const |
operator GPosition () const | |
int | operator== (const GListTemplate< TYPE, TI > &l2) const |
const TYPE & | operator[] (GPosition pos) const |
TYPE & | operator[] (GPosition pos) |
void | prepend (const TYPE &elt) |
TYPE * | prev (GPosition &pos) |
const TYPE * | prev (GPosition &pos) const |
int | search (const TYPE &elt, GPosition &pos) const |
int | size () const |
Detailed Description
template<class TYPE, class TI>
class GListTemplate< TYPE, TI >
Common base class for all doubly linked lists.
Class {GListTemplate} implements all methods for manipulating lists of of objects of type TYPE#. You should not however create instances of this class. You should instead use class {GList} or {GPList}.
Definition at line 814 of file GContainer.h.
Member Function Documentation
void GListTemplate< TYPE, TI >::append | ( | const TYPE & | elt | ) | [inline] |
Inserts an element after the last element of the list.
The new element is initialized with a copy of argument elt#.
Definition at line 889 of file GContainer.h.
GPosition GListTemplate< TYPE, TI >::contains | ( | const TYPE & | elt | ) | const [inline] |
Tests whether the list contains a given element.
If the list contains elt#, the position of the the first list element equal to elt# as checked by TYPE::operator==(const TYPE&)# is returned. Otherwise an invalid position is returned.
Definition at line 869 of file GContainer.h.
void GListTemplate< TYPE, TI >::del | ( | GPosition & | pos | ) | [inline] |
Destroys the list element at position pos#.
This function does nothing unless position pos# is a valid position.
Reimplemented from GListBase.
Definition at line 914 of file GContainer.h.
void GListTemplate< TYPE, TI >::empty | ( | ) | [inline] |
Erases the list contents.
All list elements are destroyed and unlinked. The list is left with zero elements.
Reimplemented from GListBase.
Definition at line 885 of file GContainer.h.
void GListTemplate< TYPE, TI >::first | ( | GPosition & | pos | ) | const [inline] |
Definition at line 918 of file GContainer.h.
GPosition GListTemplate< TYPE, TI >::firstpos | ( | ) | const [inline] |
Returns the first position in the list.
See {GPosition}.
Reimplemented from GListBase.
Definition at line 822 of file GContainer.h.
void GListTemplate< TYPE, TI >::insert_after | ( | GPosition | pos, | |
const TYPE & | elt | |||
) | [inline] |
Inserts a new element after the list element at position pos#.
When position pos# is null the element is inserted at the beginning of the list. The new element is initialized with a copy of elt#.
Definition at line 898 of file GContainer.h.
void GListTemplate< TYPE, TI >::insert_before | ( | GPosition | pos, | |
GListTemplate< TYPE, TI > & | fromlist, | |||
GPosition & | frompos | |||
) | [inline] |
Inserts an element of another list into this list.
This function removes the element at position frompos# in list frompos#, inserts it in the current list before the element at position pos#, and advances frompos# to the next element in list fromlist#. When position pos# is null the element is inserted at the end of the list.
Definition at line 910 of file GContainer.h.
void GListTemplate< TYPE, TI >::insert_before | ( | GPosition | pos, | |
const TYPE & | elt | |||
) | [inline] |
Inserts a new element before the list element at position pos#.
When position pos# is null the element is inserted at the end of the list. The new element is initialized with a copy of elt#.
Definition at line 903 of file GContainer.h.
int GListTemplate< TYPE, TI >::isempty | ( | ) | const [inline] |
Tests whether a list is empty.
Returns a non zero value if the list contains no elements.
Reimplemented from GListBase.
Definition at line 848 of file GContainer.h.
void GListTemplate< TYPE, TI >::last | ( | GPosition & | pos | ) | const [inline] |
Definition at line 919 of file GContainer.h.
GPosition GListTemplate< TYPE, TI >::lastpos | ( | ) | const [inline] |
Returns the last position in the list.
See {GPosition}.
Reimplemented from GListBase.
Definition at line 825 of file GContainer.h.
TYPE* GListTemplate< TYPE, TI >::next | ( | GPosition & | pos | ) | [inline] |
Definition at line 924 of file GContainer.h.
const TYPE* GListTemplate< TYPE, TI >::next | ( | GPosition & | pos | ) | const [inline] |
Definition at line 920 of file GContainer.h.
int GListTemplate< TYPE, TI >::nth | ( | unsigned int | n, | |
GPosition & | pos | |||
) | const [inline] |
Definition at line 863 of file GContainer.h.
GPosition GListTemplate< TYPE, TI >::nth | ( | unsigned int | n | ) | const [inline] |
Returns the position pos# of the n#-th list element.
An invalid position is returned if the list contains less than n# elements. The operation works by sequentially scanning the list until reaching the n#-th element.
Reimplemented from GListBase.
Definition at line 860 of file GContainer.h.
GListTemplate< TYPE, TI >::operator GPosition | ( | ) | const [inline] |
int GListTemplate< TYPE, TI >::operator== | ( | const GListTemplate< TYPE, TI > & | l2 | ) | const [inline] |
Compares two lists.
Returns a non zero value if and only if both lists contain the same elements (as tested by TYPE::operator==(const TYPE&)# in the same order.
Definition at line 853 of file GContainer.h.
const TYPE& GListTemplate< TYPE, TI >::operator[] | ( | GPosition | pos | ) | const [inline] |
Returns a constant reference to the list element at position pos#.
This reference only be used for reading a list element. An exception {GException} is thrown if pos# is not a valid position. This variant of operator[]# is necessary when dealing with a const GList<TYPE>#. See {GPosition} for efficient operations on positions.
Definition at line 843 of file GContainer.h.
TYPE& GListTemplate< TYPE, TI >::operator[] | ( | GPosition | pos | ) | [inline] |
Returns a reference to the list element at position pos#.
This reference can be used for both reading (as "#a[n]#") and modifying (as "#a[n]=v#") a list element. Using an invalid position will cause a segmentation violation. See {GPosition} for efficient operations on positions.
Definition at line 835 of file GContainer.h.
void GListTemplate< TYPE, TI >::prepend | ( | const TYPE & | elt | ) | [inline] |
Inserts an element before the first element of the list.
The new element is initialized with a copy of argument elt#.
Definition at line 893 of file GContainer.h.
TYPE* GListTemplate< TYPE, TI >::prev | ( | GPosition & | pos | ) | [inline] |
Definition at line 926 of file GContainer.h.
const TYPE* GListTemplate< TYPE, TI >::prev | ( | GPosition & | pos | ) | const [inline] |
Definition at line 922 of file GContainer.h.
int GListTemplate< TYPE, TI >::search | ( | const TYPE & | elt, | |
GPosition & | pos | |||
) | const [inline] |
Searches the list for a given element.
If position pos# is a valid position for this list, the search starts at the specified position. If position pos# is not a valid position, the search starts at the beginning of the list. The list elements are sequentially compared with elt# using TYPE::operator==(const TYPE&)#. As soon as a list element is equal to elt#, function search# sets argument pos# with the position of this list element and returns 1. If however the search reaches the end of the list, function search# returns 0 and leaves pos# unchanged.
Definition at line 880 of file GContainer.h.
int GListTemplate< TYPE, TI >::size | ( | void | ) | const [inline] |
The documentation for this class was generated from the following file: