dcop
ICElibint.h File Reference
#include "config.h"
#include <KDE-ICE/ICEproto.h>
#include <KDE-ICE/ICEconn.h>
#include <KDE-ICE/ICEmsg.h>
#include <stdlib.h>
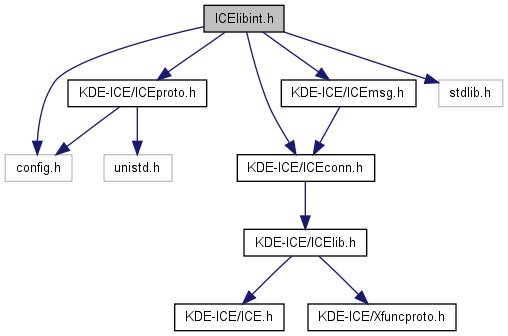
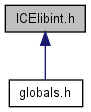
Go to the source code of this file.
Classes | |
struct | _IceConnectionError |
struct | _IceConnectionReply |
struct | _IceListenObj |
struct | _IceProtocolError |
struct | _IceProtocolReply |
union | _IceReply |
struct | _IceVersion |
struct | _IceWatchedConnection |
struct | _IceWatchProc |
Defines | |
#define | _IceAddOpcodeMapping _kde_IceAddOpcodeMapping |
#define | _IceAddReplyWait _kde_IceAddReplyWait |
#define | _IceCheckReplyReady _kde_IceCheckReplyReady |
#define | _IceConnectionClosed _kde_IceConnectionClosed |
#define | _IceConnectionOpened _kde_IceConnectionOpened |
#define | _IceErrorAuthenticationFailed _kde_IceErrorAuthenticationFailed |
#define | _IceErrorAuthenticationRejected _kde_IceErrorAuthenticationRejected |
#define | _IceErrorBadMajor _kde_IceErrorBadMajor |
#define | _IceErrorMajorOpcodeDuplicate _kde_IceErrorMajorOpcodeDuplicate |
#define | _IceErrorNoAuthentication _kde_IceErrorNoAuthentication |
#define | _IceErrorNoVersion _kde_IceErrorNoVersion |
#define | _IceErrorProtocolDuplicate _kde_IceErrorProtocolDuplicate |
#define | _IceErrorSetupFailed _kde_IceErrorSetupFailed |
#define | _IceErrorUnknownProtocol _kde_IceErrorUnknownProtocol |
#define | _IceFreeConnection _kde_IceFreeConnection |
#define | _IceGetPaAuthData _kde_IceGetPaAuthData |
#define | _IceGetPaValidAuthIndices _kde_IceGetPaValidAuthIndices |
#define | _IceGetPeerName _kde_IceGetPeerName |
#define | _IceGetPoAuthData _kde_IceGetPoAuthData |
#define | _IceGetPoValidAuthIndices _kde_IceGetPoValidAuthIndices |
#define | _IceSearchReplyWaits _kde_IceSearchReplyWaits |
#define | _IceSetReplyReady _kde_IceSetReplyReady |
#define | _SIZEOF(x) sz_##x |
#define | EXTRACT_CARD16(_pBuf, _swap, _val) |
#define | EXTRACT_CARD32(_pBuf, _swap, _val) |
#define | EXTRACT_CARD8(_pBuf, _val) |
#define | EXTRACT_LISTOF_STRING(_pBuf, _swap, _count, _strings) |
#define | EXTRACT_STRING(_pBuf, _swap, _string) |
#define | ICE_CONNECTION_ERROR 2 |
#define | ICE_CONNECTION_REPLY 1 |
#define | ICE_INBUFSIZE 1024 |
#define | ICE_MAX_AUTH_DATA_ENTRIES 100 |
#define | ICE_OUTBUFSIZE 1024 |
#define | ICE_PROTOCOL_ERROR 4 |
#define | ICE_PROTOCOL_REPLY 3 |
#define | IceLockConn(_iceConn) |
#define | IceReleaseString "1.0" |
#define | IceUnlockConn(_iceConn) |
#define | IceVendorString "MIT" |
#define | lswapl(_val) |
#define | lswaps(_val) ((((_val) & 0xff) << 8) | (((_val) >> 8) & 0xff)) |
#define | MAX_ICE_AUTH_NAMES 32 |
#define | NULL 0 |
#define | PAD32(_bytes) ((4 - ((unsigned int) (_bytes) % 4)) % 4) |
#define | PAD64(_bytes) ((8 - ((unsigned int) (_bytes) % 8)) % 8) |
#define | PADDED_BYTES32(_bytes) (_bytes + PAD32 (_bytes)) |
#define | PADDED_BYTES64(_bytes) (_bytes + PAD64 (_bytes)) |
#define | SIZEOF(x) _SIZEOF(x) |
#define | SKIP_LISTOF_STRING(_pBuf, _swap, _count, _end, _bail) |
#define | SKIP_STRING(_pBuf, _swap, _end, _bail) |
#define | STORE_CARD16(_pBuf, _val) |
#define | STORE_CARD32(_pBuf, _val) |
#define | STORE_CARD8(_pBuf, _val) |
#define | STORE_STRING(_pBuf, _string) |
#define | STRING_BYTES(_string) (2 + strlen (_string) + PAD32 (2 + strlen (_string))) |
#define | WORD32COUNT(_bytes) (((unsigned int) ((_bytes) + 3)) >> 2) |
#define | WORD64COUNT(_bytes) (((unsigned int) ((_bytes) + 7)) >> 3) |
Typedefs | |
typedef void(* | _IceProcessCoreMsgProc )() |
Functions | |
void | _IceAddOpcodeMapping () |
void | _IceAddReplyWait () |
Bool | _IceCheckReplyReady () |
void | _IceConnectionClosed () |
void | _IceConnectionOpened () |
void | _IceErrorAuthenticationFailed () |
void | _IceErrorAuthenticationRejected () |
void | _IceErrorBadMajor () |
void | _IceErrorMajorOpcodeDuplicate () |
void | _IceErrorNoAuthentication () |
void | _IceErrorNoVersion () |
void | _IceErrorProtocolDuplicate () |
void | _IceErrorSetupFailed () |
void | _IceErrorUnknownProtocol () |
void | _IceFreeConnection () |
void | _IceGetPaAuthData () |
void | _IceGetPaValidAuthIndices () |
char * | _IceGetPeerName () |
void | _IceGetPoAuthData () |
void | _IceGetPoValidAuthIndices () |
IceReplyWaitInfo * | _IceSearchReplyWaits () |
void | _IceSetReplyReady () |
Variables | |
int | _IceAuthCount |
const char * | _IceAuthNames [] |
int | _IceConnectionCount |
IceConn | _IceConnectionObjs [] |
char * | _IceConnectionStrings [] |
IcePaAuthProc | _IcePaAuthProcs [] |
IcePoAuthProc | _IcePoAuthProcs [] |
int | _IceVersionCount |
Define Documentation
#define _IceAddOpcodeMapping _kde_IceAddOpcodeMapping |
Definition at line 498 of file ICElibint.h.
#define _IceAddReplyWait _kde_IceAddReplyWait |
Definition at line 524 of file ICElibint.h.
#define _IceCheckReplyReady _kde_IceCheckReplyReady |
Definition at line 551 of file ICElibint.h.
#define _IceConnectionClosed _kde_IceConnectionClosed |
Definition at line 568 of file ICElibint.h.
#define _IceConnectionOpened _kde_IceConnectionOpened |
Definition at line 560 of file ICElibint.h.
#define _IceErrorAuthenticationFailed _kde_IceErrorAuthenticationFailed |
Definition at line 461 of file ICElibint.h.
#define _IceErrorAuthenticationRejected _kde_IceErrorAuthenticationRejected |
Definition at line 451 of file ICElibint.h.
#define _IceErrorBadMajor _kde_IceErrorBadMajor |
Definition at line 411 of file ICElibint.h.
#define _IceErrorMajorOpcodeDuplicate _kde_IceErrorMajorOpcodeDuplicate |
Definition at line 480 of file ICElibint.h.
#define _IceErrorNoAuthentication _kde_IceErrorNoAuthentication |
Definition at line 423 of file ICElibint.h.
#define _IceErrorNoVersion _kde_IceErrorNoVersion |
Definition at line 432 of file ICElibint.h.
#define _IceErrorProtocolDuplicate _kde_IceErrorProtocolDuplicate |
Definition at line 471 of file ICElibint.h.
#define _IceErrorSetupFailed _kde_IceErrorSetupFailed |
Definition at line 441 of file ICElibint.h.
#define _IceErrorUnknownProtocol _kde_IceErrorUnknownProtocol |
Definition at line 489 of file ICElibint.h.
#define _IceFreeConnection _kde_IceFreeConnection |
Definition at line 516 of file ICElibint.h.
#define _IceGetPaAuthData _kde_IceGetPaAuthData |
Definition at line 588 of file ICElibint.h.
#define _IceGetPaValidAuthIndices _kde_IceGetPaValidAuthIndices |
Definition at line 613 of file ICElibint.h.
#define _IceGetPeerName _kde_IceGetPeerName |
Definition at line 508 of file ICElibint.h.
#define _IceGetPoAuthData _kde_IceGetPoAuthData |
Definition at line 576 of file ICElibint.h.
#define _IceGetPoValidAuthIndices _kde_IceGetPoValidAuthIndices |
Definition at line 600 of file ICElibint.h.
#define _IceSearchReplyWaits _kde_IceSearchReplyWaits |
Definition at line 533 of file ICElibint.h.
#define _IceSetReplyReady _kde_IceSetReplyReady |
Definition at line 542 of file ICElibint.h.
#define _SIZEOF | ( | x | ) | sz_##x |
Definition at line 35 of file ICElibint.h.
#define EXTRACT_CARD16 | ( | _pBuf, | |||
_swap, | |||||
_val | ) |
Value:
Definition at line 228 of file ICElibint.h.
#define EXTRACT_CARD32 | ( | _pBuf, | |||
_swap, | |||||
_val | ) |
Value:
Definition at line 236 of file ICElibint.h.
#define EXTRACT_CARD8 | ( | _pBuf, | |||
_val | ) |
#define EXTRACT_LISTOF_STRING | ( | _pBuf, | |||
_swap, | |||||
_count, | |||||
_strings | ) |
Value:
{ \ int _i; \ for (_i = 0; _i < _count; _i++) \ EXTRACT_STRING (_pBuf, _swap, _strings[_i]); \ }
Definition at line 284 of file ICElibint.h.
#define EXTRACT_STRING | ( | _pBuf, | |||
_swap, | |||||
_string | ) |
Value:
{ \ CARD16 _len; \ EXTRACT_CARD16 (_pBuf, _swap, _len); \ _string = (char *) malloc (_len + 1); \ memcpy (_string, _pBuf, _len); \ _pBuf += _len; \ _string[_len] = '\0'; \ if (PAD32 (2 + _len)) \ _pBuf += PAD32 (2 + _len); \ }
Definition at line 272 of file ICElibint.h.
#define ICE_CONNECTION_ERROR 2 |
Definition at line 331 of file ICElibint.h.
#define ICE_CONNECTION_REPLY 1 |
Definition at line 330 of file ICElibint.h.
#define ICE_INBUFSIZE 1024 |
Definition at line 105 of file ICElibint.h.
#define ICE_MAX_AUTH_DATA_ENTRIES 100 |
Definition at line 119 of file ICElibint.h.
#define ICE_OUTBUFSIZE 1024 |
Definition at line 107 of file ICElibint.h.
#define ICE_PROTOCOL_ERROR 4 |
Definition at line 333 of file ICElibint.h.
#define ICE_PROTOCOL_REPLY 3 |
Definition at line 332 of file ICElibint.h.
#define IceLockConn | ( | _iceConn | ) |
Definition at line 392 of file ICElibint.h.
#define IceReleaseString "1.0" |
Definition at line 58 of file ICElibint.h.
#define IceUnlockConn | ( | _iceConn | ) |
Definition at line 393 of file ICElibint.h.
#define IceVendorString "MIT" |
Definition at line 57 of file ICElibint.h.
#define lswapl | ( | _val | ) |
Value:
((((_val) & 0xff) << 24) |\ (((_val) & 0xff00) << 8) |\ (((_val) & 0xff0000) >> 8) |\ (((_val) >> 24) & 0xff))
Definition at line 316 of file ICElibint.h.
#define lswaps | ( | _val | ) | ((((_val) & 0xff) << 8) | (((_val) >> 8) & 0xff)) |
Definition at line 322 of file ICElibint.h.
#define MAX_ICE_AUTH_NAMES 32 |
Definition at line 118 of file ICElibint.h.
#define NULL 0 |
Definition at line 49 of file ICElibint.h.
#define PAD32 | ( | _bytes | ) | ((4 - ((unsigned int) (_bytes) % 4)) % 4) |
Definition at line 74 of file ICElibint.h.
#define PAD64 | ( | _bytes | ) | ((8 - ((unsigned int) (_bytes) % 8)) % 8) |
Definition at line 65 of file ICElibint.h.
#define PADDED_BYTES32 | ( | _bytes | ) | (_bytes + PAD32 (_bytes)) |
Definition at line 76 of file ICElibint.h.
#define PADDED_BYTES64 | ( | _bytes | ) | (_bytes + PAD64 (_bytes)) |
Definition at line 67 of file ICElibint.h.
#define SIZEOF | ( | x | ) | _SIZEOF(x) |
Definition at line 36 of file ICElibint.h.
#define SKIP_LISTOF_STRING | ( | _pBuf, | |||
_swap, | |||||
_count, | |||||
_end, | |||||
_bail | ) |
Value:
{ \ int _i; \ for (_i = 0; _i < _count; _i++) \ SKIP_STRING (_pBuf, _swap, _end, _bail); \ }
Definition at line 302 of file ICElibint.h.
#define SKIP_STRING | ( | _pBuf, | |||
_swap, | |||||
_end, | |||||
_bail | ) |
Value:
{ \ CARD16 _len; \ EXTRACT_CARD16 (_pBuf, _swap, _len); \ _pBuf += _len + PAD32(2+_len); \ if (_pBuf > _end) { \ _bail; \ } \ }
Definition at line 292 of file ICElibint.h.
#define STORE_CARD16 | ( | _pBuf, | |||
_val | ) |
#define STORE_CARD32 | ( | _pBuf, | |||
_val | ) |
#define STORE_CARD8 | ( | _pBuf, | |||
_val | ) |
#define STORE_STRING | ( | _pBuf, | |||
_string | ) |
Value:
{ \ CARD16 _len = strlen (_string); \ STORE_CARD16 (_pBuf, _len); \ memcpy (_pBuf, _string, _len); \ _pBuf += _len; \ if (PAD32 (2 + _len)) \ _pBuf += PAD32 (2 + _len); \ }
Definition at line 205 of file ICElibint.h.
#define STRING_BYTES | ( | _string | ) | (2 + strlen (_string) + PAD32 (2 + strlen (_string))) |
Definition at line 97 of file ICElibint.h.
#define WORD32COUNT | ( | _bytes | ) | (((unsigned int) ((_bytes) + 3)) >> 2) |
Definition at line 90 of file ICElibint.h.
#define WORD64COUNT | ( | _bytes | ) | (((unsigned int) ((_bytes) + 7)) >> 3) |
Definition at line 83 of file ICElibint.h.
Typedef Documentation
typedef void(* _IceProcessCoreMsgProc)() |
Definition at line 137 of file ICElibint.h.
Function Documentation
void _IceAddOpcodeMapping | ( | ) |
void _IceAddReplyWait | ( | ) |
Bool _IceCheckReplyReady | ( | ) |
void _IceConnectionClosed | ( | ) |
void _IceConnectionOpened | ( | ) |
void _IceErrorAuthenticationFailed | ( | ) |
void _IceErrorAuthenticationRejected | ( | ) |
void _IceErrorBadMajor | ( | ) |
void _IceErrorMajorOpcodeDuplicate | ( | ) |
void _IceErrorNoAuthentication | ( | ) |
void _IceErrorNoVersion | ( | ) |
void _IceErrorProtocolDuplicate | ( | ) |
void _IceErrorSetupFailed | ( | ) |
void _IceErrorUnknownProtocol | ( | ) |
void _IceFreeConnection | ( | ) |
void _IceGetPaAuthData | ( | ) |
void _IceGetPaValidAuthIndices | ( | ) |
char* _IceGetPeerName | ( | ) |
void _IceGetPoAuthData | ( | ) |
void _IceGetPoValidAuthIndices | ( | ) |
IceReplyWaitInfo* _IceSearchReplyWaits | ( | ) |
void _IceSetReplyReady | ( | ) |
Variable Documentation
int _IceAuthCount |
const char* _IceAuthNames[] |
char* _IceConnectionStrings[] |
int _IceVersionCount |