dcop
DCOPObject Class Reference
Provides an interface for receiving DCOP messages. More...
#include <dcopobject.h>
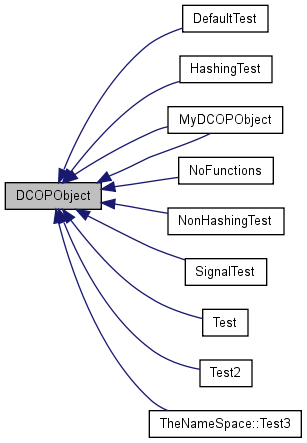
Public Member Functions | |
DCOPClient * | callingDcopClient () |
bool | connectDCOPSignal (const QCString &sender, const QCString &senderObj, const QCString &signal, const QCString &slot, bool Volatile) |
DCOPObject (const QCString &objId) | |
DCOPObject (QObject *obj) | |
DCOPObject () | |
bool | disconnectDCOPSignal (const QCString &sender, const QCString &senderObj, const QCString &signal, const QCString &slot) |
void | emitDCOPSignal (const QCString &signal, const QByteArray &data) |
virtual QCStringList | functions () |
virtual QCStringList | functionsDynamic () |
virtual QCStringList | interfaces () |
virtual QCStringList | interfacesDynamic () |
QCString | objId () const |
virtual bool | process (const QCString &fun, const QByteArray &data, QCString &replyType, QByteArray &replyData) |
virtual bool | processDynamic (const QCString &fun, const QByteArray &data, QCString &replyType, QByteArray &replyData) |
void | setCallingDcopClient (DCOPClient *) |
bool | setObjId (const QCString &objId) |
virtual | ~DCOPObject () |
Static Public Member Functions | |
static DCOPObject * | find (const QCString &objId) |
static bool | hasObject (const QCString &objId) |
static QPtrList< DCOPObject > | match (const QCString &partialId) |
static QCString | objectName (QObject *obj) |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
Provides an interface for receiving DCOP messages.This class provides an interface for receiving DCOP messages. To use it, simply multiply-inherit from DCOPObject and from some other class, and then implement the DCOPObject::process() method. Because this method is pure virtual, you must implement the method.
Note that it is usually more convenient to mark a section in the class with "k_dcop:", add your DCOP methods there and let the IDL compiler do the rest. Read the tutorials for more information.
- See also:
- DCOPClient
Definition at line 67 of file dcopobject.h.
Constructor & Destructor Documentation
DCOPObject::DCOPObject | ( | ) |
Creates a DCOPObject and calculates the object id using its physical memory address.
Definition at line 47 of file dcopobject.cpp.
DCOPObject::DCOPObject | ( | QObject * | obj | ) |
Creates a DCOPObject and calculates the object id using QObject::name().
- Parameters:
-
obj the object to extract the name from
Definition at line 54 of file dcopobject.cpp.
DCOPObject::DCOPObject | ( | const QCString & | objId | ) |
Creates a DCOPObject with object Id objId
.
- Parameters:
-
objId the object id of the DCOP object
Definition at line 69 of file dcopobject.cpp.
DCOPObject::~DCOPObject | ( | ) | [virtual] |
Destroys the DCOPObject and removes it from the map of known objects.
Definition at line 78 of file dcopobject.cpp.
Member Function Documentation
DCOPClient * DCOPObject::callingDcopClient | ( | ) |
Returns the DCOPClient responsible for making the call.
Only call this function while you are handling a DCOP call.
- Returns:
- the DCOPClient responsible for making the call. This information is only guaranteed to be correct when entering your DCOP function.
- Since:
- 3.1
Definition at line 88 of file dcopobject.cpp.
bool DCOPObject::connectDCOPSignal | ( | const QCString & | sender, | |
const QCString & | senderObj, | |||
const QCString & | signal, | |||
const QCString & | slot, | |||
bool | Volatile | |||
) |
Connects to a DCOP signal.
- Parameters:
-
sender the name of the client that emits the signal. When empty the signal will be passed from any client. senderObj the name of the sending object that emits the signal. signal the name of the signal. The arguments should match with slot. slot The name of the slot to call. Its arguments should match with signal. Volatile If true, the connection will not be reestablished when sender
unregisters and reregisters with DCOP. In this case thesender
must be registered when the connection is made. If false, the connection will be reestablished whensender
reregisters. In this case the connection can be made even ifsender
is not registered at that time.
- Returns:
- false if a connection could not be established. This will be the case when
Volatile
is true andsender
does not exist.signal
andslot
do not have matching arguments.
Definition at line 220 of file dcopobject.cpp.
bool DCOPObject::disconnectDCOPSignal | ( | const QCString & | sender, | |
const QCString & | senderObj, | |||
const QCString & | signal, | |||
const QCString & | slot | |||
) |
Disconnects a DCOP signal.
A special case is when both sender
& signal
are empty. In this case all connections related to this object in the current client are disconnected. (Both connections from as well as to this object!)
- Parameters:
-
sender the name of the client that emits the signal. senderObj the name of the object that emits the signal. If empty all objects will be disconnected. signal the name of the signal. The arguments should match with slot. If empty all objects will be disconnected. slot The name of the slot the signal is connected to. If empty all slots will be disconnected.
- Returns:
- false if no connection(s) where removed.
Definition at line 234 of file dcopobject.cpp.
void DCOPObject::emitDCOPSignal | ( | const QCString & | signal, | |
const QByteArray & | data | |||
) |
Emit signal
as DCOP signal from this object with data
as arguments.
- Parameters:
-
signal the signal to emit data the data to send
Definition at line 213 of file dcopobject.cpp.
DCOPObject * DCOPObject::find | ( | const QCString & | objId | ) | [static] |
Try to find a dcop object with the given id.
This function does not query the DCOPObjectProxy.
- Parameters:
-
objId the object id to search
- Returns:
- the DCOPObject for the id
objId
.
Definition at line 125 of file dcopobject.cpp.
QCStringList DCOPObject::functions | ( | ) | [virtual] |
Returns the list of functions understood by the object.
It gets reimplemented by the IDL compiler. If you don't use the IDL compiler, consider implementing this function manually if you want your object to be easily scriptable.
Rationale: functions() allows an interpreter to do client-side type-casting properly.
Note to implementators: remember to call the baseclasses implementation.
- Returns:
- a list of functions
Reimplemented in MyDCOPObject.
Definition at line 205 of file dcopobject.cpp.
QCStringList DCOPObject::functionsDynamic | ( | ) | [virtual] |
This function is of interest when you used an IDL compiler to generate the implementation for functions() but you still want to list some functions dynamically.
Dynamically means that the methods may appear and vanish during runtime.
- Returns:
- A list of the additional functions, default is an empty list.
- See also:
- functions(),
Definition at line 193 of file dcopobject.cpp.
bool DCOPObject::hasObject | ( | const QCString & | objId | ) | [static] |
Checks whether an object with the given id is known in this process.
- Returns:
- true if an object with the questionable
objId
is known in this process. This query does not ask proxies.
Definition at line 117 of file dcopobject.cpp.
QCStringList DCOPObject::interfaces | ( | ) | [virtual] |
Returns the names of the interfaces, specific ones last.
The functions gets reimplemented by the IDL compiler. If you don't use the IDL compiler, consider implementing this function manually if you want your object to be easily explorable.
- Returns:
- a list of interfaces
- See also:
- functions()
Definition at line 198 of file dcopobject.cpp.
QCStringList DCOPObject::interfacesDynamic | ( | ) | [virtual] |
This function is of interest when you used an IDL compiler to generate the implementation for interfaces() but you still want to list some interfaces dynamically.
Dynamically means that they may appear and vanish during runtime.
- Returns:
- A list of the additional interfaces, default is an empty list.
- See also:
- interfaces(),
Definition at line 187 of file dcopobject.cpp.
QPtrList< DCOPObject > DCOPObject::match | ( | const QCString & | partialId | ) | [static] |
Tries to find an object using a partial object id.
This function is used for multicasting a DCOP message to several objects inside a single process.
- Parameters:
-
partialId the partial object id to search for
- Returns:
- a list of DCOPObjects beginning with the string contained in
partialId
.
Definition at line 135 of file dcopobject.cpp.
Creates an object id for the QObject obj
.
This is done using the QObject::name() function.
- Parameters:
-
obj the object whose name will be used
- Returns:
- the created object id
Definition at line 146 of file dcopobject.cpp.
QCString DCOPObject::objId | ( | ) | const |
Returns the object id of the DCOPObject.
- Returns:
- the object's id
Definition at line 112 of file dcopobject.cpp.
bool DCOPObject::process | ( | const QCString & | fun, | |
const QByteArray & | data, | |||
QCString & | replyType, | |||
QByteArray & | replyData | |||
) | [virtual] |
Dispatches a message.
Usually you want to use an IDL compiler to automatically generate an implementation for this function.
If this function returns false, then processDynamic() is called.
Note to implementators: remember to call the baseclasses implementation. It handles the functions "functions()" and "interfaces()" which return the lists of supported functions and interfaces, respectively.
- Parameters:
-
fun is the normalized function signature. Such a signature usually looks like foobar(QString,int). The return type, qualifiers like "const" etc. are not part of the signature. data the received data replyType write the reply type in this string replyData write the reply data in this array
- Returns:
- true if successful, false otherwise. The default implementation returns false for all
fun
except "functions()" and "interfaces()".
Reimplemented in MyDCOPObject, and MyDCOPObject.
Definition at line 166 of file dcopobject.cpp.
bool DCOPObject::processDynamic | ( | const QCString & | fun, | |
const QByteArray & | data, | |||
QCString & | replyType, | |||
QByteArray & | replyData | |||
) | [virtual] |
This function is of interest when you used an IDL compiler to generate the implementation for process() but you still want to dispatch some functions dynamically.
Dynamically means that methods may appear and vanish during runtime.
- Parameters:
-
fun is the normalized function signature. Such a signature usually looks like foobar(QString,int). The return type, qualifiers like "const" etc. are not part of the signature. data the received data replyType write the reply type in this string replyData write the reply data in this array
- Returns:
- true if successful, false otherwise. The default implementation returns always false.
Definition at line 183 of file dcopobject.cpp.
void DCOPObject::setCallingDcopClient | ( | DCOPClient * | client | ) |
For internal use only.
Sets DCOPClient returned by callingDcopClient()
- Since:
- 3.1
Definition at line 93 of file dcopobject.cpp.
bool DCOPObject::setObjId | ( | const QCString & | objId | ) |
Renames a dcop object, if no other with the same name exists Use with care, all dcop signals are disconnected.
- Parameters:
-
objId the new object id
Definition at line 98 of file dcopobject.cpp.
void DCOPObject::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 279 of file dcopobject.cpp.
The documentation for this class was generated from the following files: