dcop
scanner.cc File Reference
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include "yacc.cc.h"
#include <qstring.h>
#include <qregexp.h>
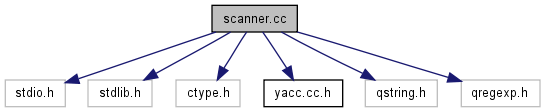
Go to the source code of this file.
Defines | |
#define | BEGIN yy_start = 1 + 2 * |
#define | ECHO (void) fwrite( yytext, yyleng, 1, yyout ) |
#define | EOB_ACT_CONTINUE_SCAN 0 |
#define | EOB_ACT_END_OF_FILE 1 |
#define | EOB_ACT_LAST_MATCH 2 |
#define | FLEX_SCANNER |
#define | INITIAL 0 |
#define | REJECT |
#define | unput(c) yyunput( c, yytext_ptr ) |
#define | YY_AT_BOL() (yy_current_buffer->yy_at_bol) |
#define | YY_BREAK break; |
#define | YY_BUF_SIZE 16384 |
#define | YY_BUFFER_EOF_PENDING 2 |
#define | YY_BUFFER_NEW 0 |
#define | YY_BUFFER_NORMAL 1 |
#define | YY_CURRENT_BUFFER yy_current_buffer |
#define | YY_DECL int yylex YY_PROTO(( void )) |
#define | YY_DO_BEFORE_ACTION |
#define | YY_END_OF_BUFFER 84 |
#define | YY_END_OF_BUFFER_CHAR 0 |
#define | YY_EXIT_FAILURE 2 |
#define | YY_FATAL_ERROR(msg) yy_fatal_error( msg ) |
#define | YY_FLEX_MAJOR_VERSION 2 |
#define | YY_FLEX_MINOR_VERSION 5 |
#define | YY_FLUSH_BUFFER yy_flush_buffer( yy_current_buffer ) |
#define | YY_INPUT(buf, result, max_size) |
#define | YY_MORE_ADJ 0 |
#define | YY_NEVER_INTERACTIVE 1 |
#define | yy_new_buffer yy_create_buffer |
#define | YY_NEW_FILE yyrestart( yyin ) |
#define | YY_NO_POP_STATE 1 |
#define | YY_NO_PUSH_STATE 1 |
#define | YY_NO_TOP_STATE 1 |
#define | YY_NO_UNPUT |
#define | YY_NULL 0 |
#define | YY_NUM_RULES 83 |
#define | YY_PROTO(proto) () |
#define | YY_READ_BUF_SIZE 8192 |
#define | YY_RESTORE_YY_MORE_OFFSET |
#define | YY_RULE_SETUP YY_USER_ACTION |
#define | YY_SC_TO_UI(c) ((unsigned int) (unsigned char) c) |
#define | yy_set_bol(at_bol) |
#define | yy_set_interactive(is_interactive) |
#define | YY_SKIP_YYWRAP |
#define | YY_START ((yy_start - 1) / 2) |
#define | YY_START_STACK_INCR 25 |
#define | YY_STATE_EOF(state) (YY_END_OF_BUFFER + state + 1) |
#define | YY_USES_REJECT |
#define | yyconst |
#define | yyless(n) |
#define | yyless(n) |
#define | yymore() yymore_used_but_not_detected |
#define | YYSTATE YY_START |
#define | yyterminate() return YY_NULL |
#define | yytext_ptr yytext |
#define | yywrap() 1 |
Typedefs | |
typedef struct yy_buffer_state * | YY_BUFFER_STATE |
typedef unsigned char | YY_CHAR |
typedef unsigned int | yy_size_t |
typedef int | yy_state_type |
Functions | |
static double | ascii_to_longdouble (const char *s) |
static long | ascii_to_longlong (long base, const char *s) |
if (yy_init) | |
static char | translate_char (const char *s) |
while (1) | |
static int | yy_get_next_buffer () |
static yy_state_type | yy_get_previous_state () |
static void yy_fatal_error | YY_PROTO ((yyconst char msg[])) |
static yy_state_type yy_try_NUL_trans | YY_PROTO ((yy_state_type current_state)) |
static void yy_flex_free | YY_PROTO ((void *)) |
static void *yy_flex_realloc | YY_PROTO ((void *, yy_size_t)) |
static void *yy_flex_alloc | YY_PROTO ((yy_size_t)) |
YY_BUFFER_STATE yy_scan_bytes | YY_PROTO ((yyconst char *bytes, int len)) |
YY_BUFFER_STATE yy_scan_string | YY_PROTO ((yyconst char *yy_str)) |
YY_BUFFER_STATE yy_scan_buffer | YY_PROTO ((char *base, yy_size_t size)) |
void yy_init_buffer | YY_PROTO ((YY_BUFFER_STATE b, FILE *file)) |
void yy_delete_buffer | YY_PROTO ((YY_BUFFER_STATE b)) |
YY_BUFFER_STATE yy_create_buffer | YY_PROTO ((FILE *file, int size)) |
void yy_load_buffer_state | YY_PROTO ((void)) |
void yy_switch_to_buffer | YY_PROTO ((YY_BUFFER_STATE new_buffer)) |
void yyrestart | YY_PROTO ((FILE *input_file)) |
static yy_state_type | yy_try_NUL_trans (yy_current_state) yy_state_type yy_current_state |
Variables | |
int | comment_mode |
FILE * | file |
int | function_mode = 0 |
int | idl_line_no |
int | len |
int | size |
static yyconst short int | yy_accept [312] |
static yyconst short int | yy_acclist [655] |
register int | yy_act |
static yyconst short int | yy_base [329] |
register char * | yy_bp |
static char * | yy_c_buf_p = (char *) 0 |
static yyconst short int | yy_chk [1180] |
register char * | yy_cp |
static YY_BUFFER_STATE | yy_current_buffer = 0 |
YY_DECL register yy_state_type | yy_current_state |
static yyconst short int | yy_def [329] |
static int | yy_did_buffer_switch_on_eof |
static yyconst int | yy_ec [256] |
static char * | yy_full_match |
static char | yy_hold_char |
static int | yy_init = 1 |
static int | yy_lp |
static yyconst int | yy_meta [74] |
static int | yy_n_chars |
static yyconst short int | yy_nxt [1180] |
static int | yy_start = 0 |
static yy_state_type | yy_state_buf [YY_BUF_SIZE+2] |
static yy_state_type * | yy_state_ptr |
FILE * | yyin = (FILE *) 0 |
int | yyleng |
FILE * | yyout = (FILE *) 0 |
char * | yytext |
Define Documentation
#define BEGIN yy_start = 1 + 2 * |
Definition at line 80 of file scanner.cc.
Definition at line 1012 of file scanner.cc.
#define EOB_ACT_CONTINUE_SCAN 0 |
Definition at line 105 of file scanner.cc.
#define EOB_ACT_END_OF_FILE 1 |
Definition at line 106 of file scanner.cc.
#define EOB_ACT_LAST_MATCH 2 |
Definition at line 107 of file scanner.cc.
#define FLEX_SCANNER |
Definition at line 8 of file scanner.cc.
#define INITIAL 0 |
Definition at line 813 of file scanner.cc.
#define REJECT |
Value:
{ \ *yy_cp = yy_hold_char; /* undo effects of setting up yytext */ \ yy_cp = yy_full_match; /* restore poss. backed-over text */ \ ++yy_lp; \ goto find_rule; \ }
Definition at line 801 of file scanner.cc.
#define unput | ( | c | ) | yyunput( c, yytext_ptr ) |
Definition at line 136 of file scanner.cc.
#define YY_AT_BOL | ( | ) | (yy_current_buffer->yy_at_bol) |
Definition at line 263 of file scanner.cc.
#define YY_BREAK break; |
Definition at line 1071 of file scanner.cc.
#define YY_BUF_SIZE 16384 |
Definition at line 98 of file scanner.cc.
#define YY_BUFFER_EOF_PENDING 2 |
Definition at line 199 of file scanner.cc.
#define YY_BUFFER_NEW 0 |
Definition at line 187 of file scanner.cc.
#define YY_BUFFER_NORMAL 1 |
Definition at line 188 of file scanner.cc.
#define YY_CURRENT_BUFFER yy_current_buffer |
Definition at line 208 of file scanner.cc.
#define YY_DECL int yylex YY_PROTO(( void )) |
Definition at line 1059 of file scanner.cc.
#define YY_DO_BEFORE_ACTION |
Value:
yytext_ptr = yy_bp; \ yyleng = (int) (yy_cp - yy_bp); \ yy_hold_char = *yy_cp; \ *yy_cp = '\0'; \ yy_c_buf_p = yy_cp;
Definition at line 284 of file scanner.cc.
#define YY_END_OF_BUFFER 84 |
Definition at line 292 of file scanner.cc.
#define YY_END_OF_BUFFER_CHAR 0 |
Definition at line 95 of file scanner.cc.
#define YY_EXIT_FAILURE 2 |
#define YY_FATAL_ERROR | ( | msg | ) | yy_fatal_error( msg ) |
Definition at line 1052 of file scanner.cc.
#define YY_FLEX_MAJOR_VERSION 2 |
Definition at line 9 of file scanner.cc.
#define YY_FLEX_MINOR_VERSION 5 |
Definition at line 10 of file scanner.cc.
#define YY_FLUSH_BUFFER yy_flush_buffer( yy_current_buffer ) |
Definition at line 237 of file scanner.cc.
#define YY_INPUT | ( | buf, | |||
result, | |||||
max_size | ) |
Value:
if ( yy_current_buffer->yy_is_interactive ) \ { \ int c = '*', n; \ for ( n = 0; n < max_size && \ (c = getc( yyin )) != EOF && c != '\n'; ++n ) \ buf[n] = (char) c; \ if ( c == '\n' ) \ buf[n++] = (char) c; \ if ( c == EOF && ferror( yyin ) ) \ YY_FATAL_ERROR( "input in flex scanner failed" ); \ result = n; \ } \ else if ( ((result = fread( buf, 1, max_size, yyin )) == 0) \ && ferror( yyin ) ) \ YY_FATAL_ERROR( "input in flex scanner failed" );
Definition at line 1019 of file scanner.cc.
#define YY_MORE_ADJ 0 |
Definition at line 809 of file scanner.cc.
#define YY_NEVER_INTERACTIVE 1 |
Definition at line 928 of file scanner.cc.
#define yy_new_buffer yy_create_buffer |
Definition at line 247 of file scanner.cc.
#define YY_NEW_FILE yyrestart( yyin ) |
Definition at line 93 of file scanner.cc.
#define YY_NO_POP_STATE 1 |
Definition at line 982 of file scanner.cc.
#define YY_NO_PUSH_STATE 1 |
Definition at line 981 of file scanner.cc.
#define YY_NO_TOP_STATE 1 |
Definition at line 983 of file scanner.cc.
#define YY_NO_UNPUT |
Definition at line 838 of file scanner.cc.
#define YY_NULL 0 |
Definition at line 67 of file scanner.cc.
#define YY_NUM_RULES 83 |
Definition at line 291 of file scanner.cc.
#define YY_PROTO | ( | proto | ) | () |
Definition at line 63 of file scanner.cc.
#define YY_READ_BUF_SIZE 8192 |
Definition at line 1003 of file scanner.cc.
#define YY_RESTORE_YY_MORE_OFFSET |
Definition at line 810 of file scanner.cc.
#define YY_RULE_SETUP YY_USER_ACTION |
Definition at line 1074 of file scanner.cc.
#define YY_SC_TO_UI | ( | c | ) | ((unsigned int) (unsigned char) c) |
Definition at line 74 of file scanner.cc.
#define yy_set_bol | ( | at_bol | ) |
Value:
{ \ if ( ! yy_current_buffer ) \ yy_current_buffer = yy_create_buffer( yyin, YY_BUF_SIZE ); \ yy_current_buffer->yy_at_bol = at_bol; \ }
Definition at line 256 of file scanner.cc.
#define yy_set_interactive | ( | is_interactive | ) |
Value:
{ \ if ( ! yy_current_buffer ) \ yy_current_buffer = yy_create_buffer( yyin, YY_BUF_SIZE ); \ yy_current_buffer->yy_is_interactive = is_interactive; \ }
Definition at line 249 of file scanner.cc.
#define YY_SKIP_YYWRAP |
Definition at line 269 of file scanner.cc.
#define YY_START ((yy_start - 1) / 2) |
Definition at line 86 of file scanner.cc.
#define YY_START_STACK_INCR 25 |
Definition at line 1047 of file scanner.cc.
#define YY_STATE_EOF | ( | state | ) | (YY_END_OF_BUFFER + state + 1) |
Definition at line 90 of file scanner.cc.
#define YY_USES_REJECT |
Definition at line 266 of file scanner.cc.
#define yyconst |
Definition at line 56 of file scanner.cc.
#define yyless | ( | n | ) |
Value:
do \ { \ /* Undo effects of setting up yytext. */ \ yytext[yyleng] = yy_hold_char; \ yy_c_buf_p = yytext + n; \ yy_hold_char = *yy_c_buf_p; \ *yy_c_buf_p = '\0'; \ yyleng = n; \ } \ while ( 0 )
Definition at line 125 of file scanner.cc.
#define yyless | ( | n | ) |
Value:
do \ { \ /* Undo effects of setting up yytext. */ \ *yy_cp = yy_hold_char; \ YY_RESTORE_YY_MORE_OFFSET \ yy_c_buf_p = yy_cp = yy_bp + n - YY_MORE_ADJ; \ YY_DO_BEFORE_ACTION; /* set up yytext again */ \ } \ while ( 0 )
Definition at line 125 of file scanner.cc.
#define yymore | ( | ) | yymore_used_but_not_detected |
Definition at line 808 of file scanner.cc.
#define YYSTATE YY_START |
Definition at line 87 of file scanner.cc.
#define yyterminate | ( | ) | return YY_NULL |
Definition at line 1042 of file scanner.cc.
#define yytext_ptr yytext |
Definition at line 274 of file scanner.cc.
#define yywrap | ( | ) | 1 |
Definition at line 268 of file scanner.cc.
Typedef Documentation
typedef struct yy_buffer_state* YY_BUFFER_STATE |
Definition at line 100 of file scanner.cc.
typedef unsigned char YY_CHAR |
Definition at line 270 of file scanner.cc.
typedef unsigned int yy_size_t |
Definition at line 142 of file scanner.cc.
typedef int yy_state_type |
Definition at line 272 of file scanner.cc.
Function Documentation
static double ascii_to_longdouble | ( | const char * | s | ) | [static] |
Definition at line 870 of file scanner.cc.
static long ascii_to_longlong | ( | long | base, | |
const char * | s | |||
) | [static] |
Definition at line 855 of file scanner.cc.
if | ( | yy_init | ) |
Definition at line 1088 of file scanner.cc.
static char translate_char | ( | const char * | s | ) | [static] |
Definition at line 888 of file scanner.cc.
while | ( | 1 | ) |
Definition at line 1112 of file scanner.cc.
static int yy_get_next_buffer | ( | ) | [static] |
Definition at line 1763 of file scanner.cc.
static yy_state_type yy_get_previous_state | ( | ) | [static] |
Definition at line 1895 of file scanner.cc.
static void yy_fatal_error YY_PROTO | ( | (yyconst char msg[]) | ) | [static] |
static yy_state_type yy_try_NUL_trans YY_PROTO | ( | (yy_state_type current_state) | ) | [static] |
static void yy_flex_free YY_PROTO | ( | (void *) | ) | [static] |
static void* yy_flex_realloc YY_PROTO | ( | (void *, yy_size_t) | ) | [static] |
static void* yy_flex_alloc YY_PROTO | ( | (yy_size_t) | ) | [static] |
YY_BUFFER_STATE yy_scan_bytes YY_PROTO | ( | (yyconst char *bytes, int len) | ) |
YY_BUFFER_STATE yy_scan_string YY_PROTO | ( | (yyconst char *yy_str) | ) |
YY_BUFFER_STATE yy_scan_buffer YY_PROTO | ( | (char *base, yy_size_t size) | ) |
void yy_init_buffer YY_PROTO | ( | (YY_BUFFER_STATE b, FILE *file) | ) |
void yy_flush_buffer YY_PROTO | ( | (YY_BUFFER_STATE b) | ) |
YY_BUFFER_STATE yy_create_buffer YY_PROTO | ( | (FILE *file, int size) | ) |
static int input YY_PROTO | ( | (void) | ) |
void yy_switch_to_buffer YY_PROTO | ( | (YY_BUFFER_STATE new_buffer) | ) |
void yyrestart YY_PROTO | ( | (FILE *input_file) | ) |
static yy_state_type yy_try_NUL_trans | ( | yy_current_state | ) | [static] |
Variable Documentation
int comment_mode |
Definition at line 849 of file scanner.cc.
FILE* file |
Definition at line 2163 of file scanner.cc.
int function_mode = 0 |
Definition at line 850 of file scanner.cc.
int idl_line_no |
int len |
Definition at line 2285 of file scanner.cc.
Definition at line 2074 of file scanner.cc.
yyconst short int yy_accept[312] [static] |
Definition at line 369 of file scanner.cc.
yyconst short int yy_acclist[655] [static] |
Definition at line 293 of file scanner.cc.
register int yy_act |
Definition at line 1081 of file scanner.cc.
yyconst short int yy_base[329] [static] |
Definition at line 452 of file scanner.cc.
register char * yy_bp |
Definition at line 1080 of file scanner.cc.
char* yy_c_buf_p = (char *) 0 [static] |
Definition at line 220 of file scanner.cc.
yyconst short int yy_chk[1180] [static] |
Definition at line 665 of file scanner.cc.
register char* yy_cp |
Definition at line 1080 of file scanner.cc.
YY_BUFFER_STATE yy_current_buffer = 0 [static] |
Definition at line 202 of file scanner.cc.
YY_DECL register yy_state_type yy_current_state |
Definition at line 1079 of file scanner.cc.
yyconst short int yy_def[329] [static] |
Definition at line 492 of file scanner.cc.
int yy_did_buffer_switch_on_eof [static] |
Definition at line 227 of file scanner.cc.
yyconst int yy_ec[256] [static] |
Definition at line 408 of file scanner.cc.
char* yy_full_match [static] |
Definition at line 799 of file scanner.cc.
char yy_hold_char [static] |
Definition at line 212 of file scanner.cc.
int yy_init = 1 [static] |
Definition at line 221 of file scanner.cc.
int yy_lp [static] |
Definition at line 800 of file scanner.cc.
yyconst int yy_meta[74] [static] |
Initial value:
{ 0, 1, 2, 3, 4, 1, 5, 1, 1, 5, 1, 1, 6, 1, 1, 1, 1, 1, 7, 7, 8, 1, 1, 1, 1, 1, 5, 8, 8, 8, 8, 8, 8, 4, 4, 4, 4, 4, 4, 4, 4, 4, 4, 4, 9, 1, 5, 1, 4, 7, 7, 8, 8, 8, 7, 4, 4, 4, 4, 4, 4, 9, 4, 4, 9, 4, 9, 4, 9, 9, 4, 1, 10, 1 }
Definition at line 440 of file scanner.cc.
int yy_n_chars [static] |
Definition at line 214 of file scanner.cc.
yyconst short int yy_nxt[1180] [static] |
Definition at line 532 of file scanner.cc.
int yy_start = 0 [static] |
Definition at line 222 of file scanner.cc.
yy_state_type yy_state_buf[YY_BUF_SIZE+2] [static] |
Definition at line 798 of file scanner.cc.
yy_state_type * yy_state_ptr [static] |
Definition at line 798 of file scanner.cc.
FILE * yyin = (FILE *) 0 |
Definition at line 271 of file scanner.cc.
int yyleng |
Definition at line 217 of file scanner.cc.
FILE * yyout = (FILE *) 0 |
Definition at line 271 of file scanner.cc.
char * yytext |
Definition at line 811 of file scanner.cc.