DNSSD
DNSSD::PublicService Class Reference
This class is most important for application that wants to announce its service on network. More...
#include <publicservice.h>
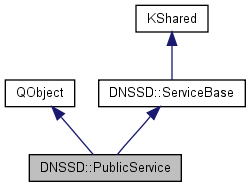
Signals | |
void | published (bool) |
Public Member Functions | |
bool | isPublished () const |
PublicService (const QString &name=QString::null, const QString &type=QString::null, unsigned int port=0, const QString &domain=QString::null) | |
bool | publish () |
void | publishAsync () |
void | setDomain (const QString &domain) |
void | setPort (unsigned short port) |
void | setServiceName (const QString &serviceName) |
void | setTextData (const QMap< QString, QString > &textData) |
void | setType (const QString &type) |
void | stop () |
const KURL | toInvitation (const QString &host=QString::null) |
~PublicService () | |
Protected Member Functions | |
virtual void | customEvent (QCustomEvent *event) |
virtual void | virtual_hook (int, void *) |
Detailed Description
This class is most important for application that wants to announce its service on network.Suppose that you want to make your web server public - this is simplest way:
DNSSD::PublicService *service = new DNSSD::PublicService("My files","_http._tcp",80); bool isOK = service->publish();
In this example publish() is synchronous - it will not return until publishing is complete. This is usually not too long but it can freeze application's GUI for a moment. Asynchronous publishing is better for responsiveness. Example:
DNSSD::PublicService *service = new DNSSD::PublicService("My files","_http._tcp",80); connect(service,SIGNAL(published(bool)),this,SLOT(wasPublished(bool))); service->publishAsync();
This class represents local service being published
Definition at line 56 of file publicservice.h.
Constructor & Destructor Documentation
DNSSD::PublicService::PublicService | ( | const QString & | name = QString::null , |
|
const QString & | type = QString::null , |
|||
unsigned int | port = 0 , |
|||
const QString & | domain = QString::null | |||
) |
- Parameters:
-
name Service name. If set to QString::null, computer name will be used and will be available via serviceName() after successful registration type Service type. Has to be in form _sometype._udp or _sometype._tcp port Port number. Set to 0 to "reserve" service name. domain Domain name. If left as QString:null, user configuration will be used. "local." means local LAN
Definition at line 53 of file publicservice.cpp.
DNSSD::PublicService::~PublicService | ( | ) |
Definition at line 64 of file publicservice.cpp.
Member Function Documentation
void DNSSD::PublicService::customEvent | ( | QCustomEvent * | event | ) | [protected, virtual] |
bool DNSSD::PublicService::isPublished | ( | ) | const |
bool DNSSD::PublicService::publish | ( | ) |
Synchrounous publish.
Application will be freezed until publishing is complete.
- Returns:
- true if successfull.
Definition at line 121 of file publicservice.cpp.
void DNSSD::PublicService::publishAsync | ( | ) |
Asynchronous version of publish().
It return immediately and emits signal published(bool) when completed. Note that in case of early detected error (like bad service type) signal may be emitted before return of this function.
Definition at line 134 of file publicservice.cpp.
void DNSSD::PublicService::published | ( | bool | ) | [signal] |
Emitted when publishing is complete - parameter is set to true if it was successfull.
It will also emitted when name, port or type of already published service is changed.
void DNSSD::PublicService::setDomain | ( | const QString & | domain | ) |
Sets domain where service is published.
"local." means local LAN. If service is currently published, it will be re-announced with new data.
Definition at line 79 of file publicservice.cpp.
void DNSSD::PublicService::setPort | ( | unsigned short | port | ) |
Sets port.
If service is currently published, it will be re-announced with new data.
Definition at line 98 of file publicservice.cpp.
void DNSSD::PublicService::setServiceName | ( | const QString & | serviceName | ) |
Sets name of the service.
If service is currently published, it will be re-announced with new data.
Definition at line 70 of file publicservice.cpp.
Sets new text properties.
If services is already published, it will be re-announced with new data.
Definition at line 112 of file publicservice.cpp.
void DNSSD::PublicService::setType | ( | const QString & | type | ) |
Sets type of service.
It has to in form of _type._udp or _type._tcp. If service is currently published, it will be re-announced with new data.
Definition at line 89 of file publicservice.cpp.
void DNSSD::PublicService::stop | ( | ) |
Stops publishing or abort incomplete publish request.
Useful when you want to disable service for some time.
Definition at line 128 of file publicservice.cpp.
Translates service into URL that can be sent to another user.
- Parameters:
-
host Use specified hostname. If left empty, public IP address (the one used for default route) will be used.
- Since:
- 3.5
Definition at line 175 of file publicservice.cpp.
void DNSSD::PublicService::virtual_hook | ( | int | , | |
void * | ||||
) | [protected, virtual] |
The documentation for this class was generated from the following files: