DNSSD
DNSSD::ServiceBrowser Class Reference
Most important class for applications that want to discover specific services on network. More...
#include <servicebrowser.h>
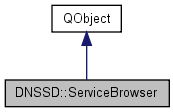
Public Types | |
enum | Flags { AutoDelete = 1, AutoResolve = 2 } |
enum | State { Working, Stopped, Unsupported } |
Public Slots | |
void | addDomain (const QString &domain) |
void | removeDomain (const QString &domain) |
Signals | |
void | finished () |
void | serviceAdded (DNSSD::RemoteService::Ptr) |
void | serviceRemoved (DNSSD::RemoteService::Ptr) |
Public Member Functions | |
const DomainBrowser * | browsedDomains () const |
ServiceBrowser (const QString &type, const QString &domain, bool autoResolve=false) | |
ServiceBrowser (const QString &type, const QString &domain, int flags) | |
ServiceBrowser (const QString &type, DomainBrowser *domains=0, bool autoResolve=false) | |
ServiceBrowser (const QStringList &types, DomainBrowser *domains, int flags) | |
const QValueList < RemoteService::Ptr > & | services () const |
virtual void | startBrowse () |
~ServiceBrowser () | |
Static Public Member Functions | |
static const State | isAvailable () |
Static Public Attributes | |
static const QString | AllServices = "_services._dns-sd._udp" |
Protected Member Functions | |
virtual void | virtual_hook (int, void *) |
Detailed Description
Most important class for applications that want to discover specific services on network.Suppose that you need list of web servers running. Example:
DNSSD::ServiceBrowser* browser = new DNSSD::ServiceBrowser("_http._tcp"); connect(browser,SIGNAL(serviceAdded(RemoteService::Ptr)),this,SLOT(addService(RemoteService::Ptr))); connect(browser,SIGNAL(serviceRemoved(RemoteService::Ptr)),this,SLOT(delService(RemoteService::Ptr))); browser->startBrowse();
In above example addService will be called for every web server already running or just appearing on network and delService will be called when server is stopped. Because no DomainBrowser was passed to constructor, domains configured by user will be searched.
Definition at line 54 of file servicebrowser.h.
Member Enumeration Documentation
- AutoDelete - DomainBrowser object passes in constructor should be deleted when ServiceBrowser is deleted
- AutoResolve - after disovering new service it will be resolved and then reported with serviceAdded() signal. It raises network usage by resolving all services, so use it only when necessary.
Definition at line 64 of file servicebrowser.h.
Availability of DNS-SD services.
- Working - available
- Stopped - not available because mdnsd daemon is not running.
- Unsupported - not available because KDE was compiled without DNS-SD support
Definition at line 75 of file servicebrowser.h.
Constructor & Destructor Documentation
DNSSD::ServiceBrowser::ServiceBrowser | ( | const QStringList & | types, | |
DomainBrowser * | domains, | |||
int | flags | |||
) |
ServiceBrowser constructor.
- Parameters:
-
types List of service types to browse for (example: "_http._tcp"). Can also be DNSSD::ServicesBrowser::AllServices to specify "metaquery" for all service types present on network domains DomainBrowser object used to specify domains to browse. You do not have to connect its domainAdded() signal - it will be done automatically. You can left this parameter as NULL for default domains. flags One or more values from Flags
- Since:
- 3.5
- Todo:
- KDE4: set default values for domains and flags
Definition at line 60 of file servicebrowser.cpp.
DNSSD::ServiceBrowser::ServiceBrowser | ( | const QString & | type, | |
DomainBrowser * | domains = 0 , |
|||
bool | autoResolve = false | |||
) |
The same as above, but allows only one service type.
- Parameters:
-
type Type of services to browse for domains DomainBrowser object used to specify domains to browse. You do not have to connect its domainAdded() signal - it will be done automatically. You can left this parameter as NULL for default domains. autoResolve auto resolve, if set
- Deprecated:
- use previous constructor instead
Definition at line 55 of file servicebrowser.cpp.
Overloaded constructor used to create browser for one domain.
- Parameters:
-
type Type of services to browse for domain Domain name. You can add more domains later using addDomain and remove them with removeDomain flags One or more values from Flags. AutoDelete flag has no effect
- Since:
- 3.5
Definition at line 81 of file servicebrowser.cpp.
DNSSD::ServiceBrowser::~ServiceBrowser | ( | ) |
Definition at line 103 of file servicebrowser.cpp.
Member Function Documentation
void DNSSD::ServiceBrowser::addDomain | ( | const QString & | domain | ) | [slot] |
const DomainBrowser * DNSSD::ServiceBrowser::browsedDomains | ( | ) | const |
Return DomainBrowser containing domains being browsed.
Valid object will returned even if 'domains' parameters in constructor was set to NULL or single domain constructor was used.
Definition at line 109 of file servicebrowser.cpp.
void DNSSD::ServiceBrowser::finished | ( | ) | [signal] |
Emitted when all services has been reported.
This signal can be used by application that just want to get list of currently available services (similar to directory listing) and do not care about dynamic adding/removing services later. This signal can be emitted many time: for example if new host has been connected to network and is announcing some services interesting to this ServiceBrowser, they will be reported by several serviceAdded() signals and whole batch will be concluded by finished().
const ServiceBrowser::State DNSSD::ServiceBrowser::isAvailable | ( | ) | [static] |
Checks availability of DNS-SD services (this also covers publishing).
If you use this function to report an error to the user, below is a suggestion on how to word the errors:
switch(DNSSD::ServiceBrowser::isAvailable()) { case DNSSD::ServiceBrowser::Working: return ""; case DNSSD::ServiceBrowser::Stopped: return i18n("<p>The Zeroconf daemon is not running. See the Service Discovery Handbook" " for more information.<br/>" "Other users will not see this system when browsing" " the network via zeroconf, but normal access will still work.</p>"); case DNSSD::ServiceBrowser::Unsupported: return i18n("<p>Zeroconf support is not available in this version of KDE." " See the Service Discovery Handbook for more information.<br/>" "Other users will not see this system when browsing" " the network via zeroconf, but normal access will still work.</p>"); default: return i18n("<p>Unknown error with Zeroconf.<br/>" "Other users will not see this system when browsing" " the network via zeroconf, but normal access will still work.</p>"); }
Definition at line 86 of file servicebrowser.cpp.
void DNSSD::ServiceBrowser::removeDomain | ( | const QString & | domain | ) | [slot] |
Remove one domain from list of domains to browse.
Definition at line 168 of file servicebrowser.cpp.
void DNSSD::ServiceBrowser::serviceAdded | ( | DNSSD::RemoteService::Ptr | ) | [signal] |
Emitted when new service is discovered (or resolved if autoresolve is set.
void DNSSD::ServiceBrowser::serviceRemoved | ( | DNSSD::RemoteService::Ptr | ) | [signal] |
Emitted when service is no longer announced.
RemoteService object is deleted from services list and destroyed immediately after this signal returns.
const QValueList< RemoteService::Ptr > & DNSSD::ServiceBrowser::services | ( | ) | const |
void DNSSD::ServiceBrowser::startBrowse | ( | ) | [virtual] |
Starts browsing for services.
To stop it just destroy the object.
Definition at line 132 of file servicebrowser.cpp.
void DNSSD::ServiceBrowser::virtual_hook | ( | int | , | |
void * | ||||
) | [protected, virtual] |
Definition at line 217 of file servicebrowser.cpp.
Member Data Documentation
const QString DNSSD::ServiceBrowser::AllServices = "_services._dns-sd._udp" [static] |
Special service type to search for all available service types.
Pass it as "type" parameter to ServiceBrowser constructor.
Definition at line 142 of file servicebrowser.h.
The documentation for this class was generated from the following files: