interfaces
KMediaPlayer::Player Class Reference
Player is the center of the KMediaPlayer interface. More...
#include <player.h>
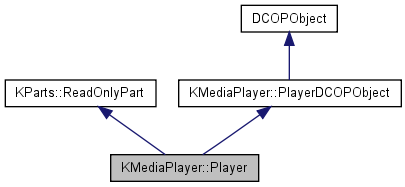
Public Types | |
enum | State { Empty, Stop, Pause, Play } |
Public Slots | |
virtual void | pause (void)=0 |
virtual void | play (void)=0 |
virtual void | seek (unsigned long msec)=0 |
void | setLooping (bool) |
virtual void | stop (void)=0 |
Signals | |
void | loopingChanged (bool) |
void | stateChanged (int) |
Public Member Functions | |
virtual bool | hasLength (void) const =0 |
bool | isLooping (void) const |
virtual bool | isSeekable (void) const =0 |
virtual unsigned long | length (void) const =0 |
Player (QWidget *parentWidget, const char *widgetName, QObject *parent, const char *name) | |
Player (QObject *parent, const char *name) | |
virtual unsigned long | position (void) const =0 |
int | state (void) const |
virtual View * | view (void)=0 |
virtual | ~Player (void) |
Protected Slots | |
void | setState (int) |
Detailed Description
Player is the center of the KMediaPlayer interface.It provides all of the necessary media player operations, and optionally provides the GUI to control them.
There are two servicetypes for Player: KMediaPlayer/Player and KMediaPlayer/Engine. KMediaPlayer/Player provides a widget (accessable through view as well as XML GUI KActions. KMediaPlayer/Engine omits the user interface facets, for those who wish to provide their own interface.
Definition at line 46 of file player.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
KMediaPlayer::Player::Player | ( | QObject * | parent, | |
const char * | name | |||
) |
This constructor is what to use when no GUI is required, as in the case of a KMediaPlayer/Engine.
Definition at line 39 of file player.cpp.
KMediaPlayer::Player::Player | ( | QWidget * | parentWidget, | |
const char * | widgetName, | |||
QObject * | parent, | |||
const char * | name | |||
) |
This constructor is what to use when a GUI is required, as in the case of a KMediaPlayer/Player.
Definition at line 31 of file player.cpp.
KMediaPlayer::Player::~Player | ( | void | ) | [virtual] |
Definition at line 47 of file player.cpp.
Member Function Documentation
virtual bool KMediaPlayer::Player::hasLength | ( | void | ) | const [pure virtual] |
Returns whether the current track has a length.
Some streams are endless, and do not have one.
Implements KMediaPlayer::PlayerDCOPObject.
bool KMediaPlayer::Player::isLooping | ( | void | ) | const [virtual] |
Return the current looping state.
Implements KMediaPlayer::PlayerDCOPObject.
Definition at line 60 of file player.cpp.
virtual bool KMediaPlayer::Player::isSeekable | ( | void | ) | const [pure virtual] |
virtual unsigned long KMediaPlayer::Player::length | ( | void | ) | const [pure virtual] |
void KMediaPlayer::Player::loopingChanged | ( | bool | ) | [signal] |
Emitted when the looping state is changed.
virtual void KMediaPlayer::Player::pause | ( | void | ) | [pure virtual, slot] |
virtual void KMediaPlayer::Player::play | ( | void | ) | [pure virtual, slot] |
virtual unsigned long KMediaPlayer::Player::position | ( | void | ) | const [pure virtual] |
virtual void KMediaPlayer::Player::seek | ( | unsigned long | msec | ) | [pure virtual, slot] |
Move the current playback position to the specified time in milliseconds, if the track is seekable.
Some streams may not be seeked.
Implements KMediaPlayer::PlayerDCOPObject.
void KMediaPlayer::Player::setLooping | ( | bool | b | ) | [virtual, slot] |
Set whether the Player should continue playing at the beginning of the track when the end of the track is reached.
Implements KMediaPlayer::PlayerDCOPObject.
Definition at line 51 of file player.cpp.
void KMediaPlayer::Player::setState | ( | int | s | ) | [protected, virtual, slot] |
Implementers use this to control what users see as the current state.
Implements KMediaPlayer::PlayerDCOPObject.
Definition at line 65 of file player.cpp.
int KMediaPlayer::Player::state | ( | void | ) | const [virtual] |
Return the current state of the player.
Implements KMediaPlayer::PlayerDCOPObject.
Definition at line 74 of file player.cpp.
void KMediaPlayer::Player::stateChanged | ( | int | ) | [signal] |
Emitted when the state changes.
virtual void KMediaPlayer::Player::stop | ( | void | ) | [pure virtual, slot] |
Stop playback of the media track and return to the beginning.
Implements KMediaPlayer::PlayerDCOPObject.
virtual View* KMediaPlayer::Player::view | ( | void | ) | [pure virtual] |
The documentation for this class was generated from the following files: