interfaces
KScriptClientInterface Class Reference
This class is used for allowing feedback to the main system. More...
#include <scriptclientinterface.h>
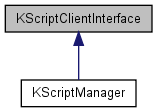
Public Types | |
enum | Result { ResultSuccess, ResultFailure, ResultContinue, ResultBreak } |
Public Member Functions | |
virtual void | done (KScriptClientInterface::Result result, const QVariant &returned)=0 |
virtual void | error (const QString &msg)=0 |
virtual void | output (const QString &msg)=0 |
virtual void | progress (int percent)=0 |
virtual void | warning (const QString &msg)=0 |
Detailed Description
This class is used for allowing feedback to the main system.To implement KScript in your application you would use this class to interface with the scripting engine. There are currently a few implementations of script managers around but developers can implement their own custom interfaces with this class.
class MyScript : public QObject, public KScriptClientInterface { Q_OBJECT public: MyScript(QObject *parent) { // Create your @ref KScriptInterface here. m_interface = KParts::ComponentFactory::createInstanceFromQuery<KScriptInterface>( "KScriptRunner/KScriptRunner", "([X-KDE-Script-Runner] == 'bash/shell')", this ); } virtual ~KScriptAction() { delete m_interface; } signals: void error ( const QString &msg ); void warning ( const QString &msg ); void output ( const QString &msg ); void progress ( int percent ); void done ( KScriptClientInterface::Result result, const QVariant &returned ); public slots: void activate(const QVariant &args) { m_interface->run(parent(), args); } private: KScriptInterface *m_interface; };
Definition at line 73 of file scriptclientinterface.h.
Member Enumeration Documentation
Definition at line 76 of file scriptclientinterface.h.
Member Function Documentation
virtual void KScriptClientInterface::done | ( | KScriptClientInterface::Result | result, | |
const QVariant & | returned | |||
) | [pure virtual] |
This function will allow feedback on completion of the script.
It turns the result as a KScriptInteface::Result, and a return value as a QVariant For script clients its best to implement this as a signal so feedback can be sent to the main application.
Implemented in KScriptManager.
virtual void KScriptClientInterface::error | ( | const QString & | msg | ) | [pure virtual] |
This function will allow the main application of any errors that have occurred during processing of the script.
For script clients its best to implement this as a signal so feedback can be sent to the main application.
Implemented in KScriptManager.
virtual void KScriptClientInterface::output | ( | const QString & | msg | ) | [pure virtual] |
This function will allow the main application of any normal output that has occurred during the processing of the script.
For script clients its best to implement this as a signal so feedback can be sent to the main application.
Implemented in KScriptManager.
virtual void KScriptClientInterface::progress | ( | int | percent | ) | [pure virtual] |
This function will allow feedback to any progress bars in the main application as to how far along the script is.
This is very useful when a script is processing files or doing some long operation that is of a known duration.] For script clients its best to implement this as a signal so feedback can be sent to the main application.
Implemented in KScriptManager.
virtual void KScriptClientInterface::warning | ( | const QString & | msg | ) | [pure virtual] |
This function will allow the main application of any warnings that have occurred during the processing of the script.
For script clients its best to implement this as a signal so feedback can be sent to the main application.
Implemented in KScriptManager.
The documentation for this class was generated from the following file: