Kate
KateSuperCursor Class Reference
Possible additional features:- Notification when a cursor enters or exits a view
- suggest something :).
#include <katesupercursor.h>
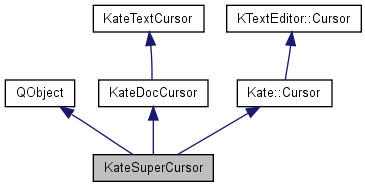
Signals | |
void | charDeletedAfter () |
void | charDeletedBefore () |
void | charInsertedAt () |
void | positionChanged () |
void | positionDeleted () |
void | positionDirectlyChanged () |
void | positionUnChanged () |
Public Member Functions | |
bool | atEndOfLine () const |
bool | atStartOfLine () const |
QChar | currentChar () const |
void | editLineInserted (uint line) |
void | editLineRemoved (uint line) |
void | editLineUnWrapped (uint line, uint col, bool removeLine=true, uint length=0) |
void | editLineWrapped (uint line, uint col, bool newLine=true) |
void | editTextInserted (uint line, uint col, uint len) |
void | editTextRemoved (uint line, uint col, uint len) |
bool | insertText (const QString &text) |
KateSuperCursor (KateDocument *doc, bool privateC, int lineNum=0, int col=0, QObject *parent=0L, const char *name=0L) | |
KateSuperCursor (KateDocument *doc, bool privateC, const KateTextCursor &cursor, QObject *parent=0L, const char *name=0L) | |
bool | moveOnInsert () const |
operator QString () | |
void | position (uint *line, uint *col) const |
bool | removeText (uint numberOfCharacters) |
virtual void | setCol (int colNum) |
virtual void | setLine (int lineNum) |
void | setMoveOnInsert (bool moveOnInsert) |
virtual void | setPos (int lineNum, int colNum) |
virtual void | setPos (const KateTextCursor &pos) |
bool | setPosition (uint line, uint col) |
~KateSuperCursor () |
Detailed Description
Possible additional features:- Notification when a cursor enters or exits a view
- suggest something :).
Unresolved issues:
- testing, testing, testing
- ie. everything which hasn't already been tested, you can see that which has inside the ifdefs
- api niceness A cursor which updates and gives off various interesting signals.
This aims to be a working version of what KateCursor was originally intended to be.
Definition at line 45 of file katesupercursor.h.
Constructor & Destructor Documentation
KateSuperCursor::KateSuperCursor | ( | KateDocument * | doc, | |
bool | privateC, | |||
const KateTextCursor & | cursor, | |||
QObject * | parent = 0L , |
|||
const char * | name = 0L | |||
) |
bool privateC says: if private, than don't show to apps using the cursorinterface in the list, all internally only used SuperCursors should be private or people could modify them from the outside breaking kate's internals
Definition at line 28 of file katesupercursor.cpp.
KateSuperCursor::KateSuperCursor | ( | KateDocument * | doc, | |
bool | privateC, | |||
int | lineNum = 0 , |
|||
int | col = 0 , |
|||
QObject * | parent = 0L , |
|||
const char * | name = 0L | |||
) |
Definition at line 41 of file katesupercursor.cpp.
KateSuperCursor::~KateSuperCursor | ( | ) |
Definition at line 54 of file katesupercursor.cpp.
Member Function Documentation
bool KateSuperCursor::atEndOfLine | ( | ) | const |
- Returns:
- true if the cursor is situated at the end of the line, false if it isn't.
Definition at line 90 of file katesupercursor.cpp.
bool KateSuperCursor::atStartOfLine | ( | ) | const |
- Returns:
- true if the cursor is situated at the start of the line, false if it isn't.
Definition at line 85 of file katesupercursor.cpp.
void KateSuperCursor::charDeletedAfter | ( | ) | [signal] |
The character immediately after the cursor was deleted.
void KateSuperCursor::charDeletedBefore | ( | ) | [signal] |
The character immediately before the cursor was deleted.
void KateSuperCursor::charInsertedAt | ( | ) | [signal] |
A character was inserted immediately before the cursor.
Whether the char was inserted before or after this cursor depends on moveOnInsert():
- true -> the char was inserted before
- false -> the char was inserted after
QChar KateSuperCursor::currentChar | ( | ) | const |
void KateSuperCursor::editLineInserted | ( | uint | line | ) |
Definition at line 239 of file katesupercursor.cpp.
void KateSuperCursor::editLineRemoved | ( | uint | line | ) |
Definition at line 252 of file katesupercursor.cpp.
void KateSuperCursor::editLineUnWrapped | ( | uint | line, | |
uint | col, | |||
bool | removeLine = true , |
|||
uint | length = 0 | |||
) |
Definition at line 211 of file katesupercursor.cpp.
Definition at line 185 of file katesupercursor.cpp.
Definition at line 129 of file katesupercursor.cpp.
Definition at line 150 of file katesupercursor.cpp.
bool KateSuperCursor::moveOnInsert | ( | ) | const |
Returns how this cursor behaves when text is inserted at the cursor.
Defaults to not moving on insert.
Definition at line 95 of file katesupercursor.cpp.
KateSuperCursor::operator QString | ( | ) |
void KateSuperCursor::positionChanged | ( | ) | [signal] |
The cursor's position was changed.
void KateSuperCursor::positionDeleted | ( | ) | [signal] |
The cursor's surrounding characters were both deleted simultaneously.
The cursor is automatically placed at the start of the deleted region.
void KateSuperCursor::positionDirectlyChanged | ( | ) | [signal] |
The cursor's position was directly changed by the program.
void KateSuperCursor::positionUnChanged | ( | ) | [signal] |
Athough an edit took place, the cursor's position was unchanged.
void KateSuperCursor::setCol | ( | int | colNum | ) | [virtual] |
void KateSuperCursor::setLine | ( | int | lineNum | ) | [virtual] |
void KateSuperCursor::setMoveOnInsert | ( | bool | moveOnInsert | ) |
Change the behavior of the cursor when text is inserted at the cursor.
If moveOnInsert
is true, the cursor will end up at the end of the insert.
Definition at line 100 of file katesupercursor.cpp.
void KateSuperCursor::setPos | ( | const KateTextCursor & | pos | ) | [virtual] |
The documentation for this class was generated from the following files: