Kate
KateTextLine Class Reference
The KateTextLine represents a line of text. More...
#include <katetextline.h>
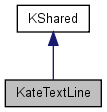
Public Types | |
enum | Flags { flagNoOtherData = 1, flagHlContinue = 2, flagAutoWrapped = 4, flagFoldingColumnsOutdated = 8, flagNoIndentationBasedFolding = 16, flagNoIndentationBasedFoldingAtStart = 32 } |
typedef KSharedPtr< KateTextLine > | Ptr |
Public Member Functions | |
uchar | attribute (uint pos) const |
uchar * | attributes () const |
const QMemArray< short > & | ctxArray () const |
int | cursorX (uint pos, uint tabChars) const |
char * | dump (char *buf, bool withHighlighting) const |
uint | dumpSize (bool withHighlighting) const |
bool | endingWith (const QString &match) const |
int | firstChar () const |
const QChar * | firstNonSpace () const |
bool | foldingColumnsOutdated () |
const QMemArray< uint > & | foldingListArray () const |
QChar | getChar (uint pos) const |
bool | hlLineContinue () const |
const QMemArray< unsigned short > & | indentationDepthArray () const |
uint | indentDepth (uint tabwidth) const |
void | insertText (uint pos, uint insLen, const QChar *insText, uchar *insAttribs=0) |
bool | isAutoWrapped () const |
KateTextLine () | |
int | lastChar () const |
uint | length () const |
uint | lengthWithTabs (uint tabChars) const |
int | nextNonSpaceChar (uint pos) const |
const bool | noIndentBasedFolding () const |
const bool | noIndentBasedFoldingAtStart () const |
int | previousNonSpaceChar (uint pos) const |
void | removeText (uint pos, uint delLen) |
char * | restore (char *buf) |
bool | searchText (uint startCol, const QRegExp ®exp, uint *foundAtCol, uint *matchLen, bool backwards=false) |
bool | searchText (uint startCol, const QString &text, uint *foundAtCol, uint *matchLen, bool casesensitive=true, bool backwards=false) |
void | setAutoWrapped (bool wrapped) |
void | setContext (QMemArray< short > &val) |
void | setFoldingColumnsOutdated (bool set) |
void | setFoldingList (QMemArray< uint > &val) |
void | setHlLineContinue (bool cont) |
void | setIndentationDepth (QMemArray< unsigned short > &val) |
void | setNoIndentBasedFolding (bool val) |
void | setNoIndentBasedFoldingAtStart (bool val) |
bool | startingWith (const QString &match) const |
QString | string (uint startCol, uint length) const |
const QString & | string () const |
bool | stringAtPos (uint pos, const QString &match) const |
const QChar * | text () const |
void | truncate (uint newLen) |
~KateTextLine () |
Detailed Description
The KateTextLine represents a line of text.A text line that contains the text, an attribute for each character, an attribute for the free space behind the last character and a context number for the syntax highlight. The attribute stores the index to a table that contains fonts and colors and also if a character is selected.
Definition at line 41 of file katetextline.h.
Member Typedef Documentation
typedef KSharedPtr<KateTextLine> KateTextLine::Ptr |
Member Enumeration Documentation
enum KateTextLine::Flags |
Used Flags.
- Enumerator:
-
flagNoOtherData flagHlContinue flagAutoWrapped flagFoldingColumnsOutdated flagNoIndentationBasedFolding flagNoIndentationBasedFoldingAtStart
Definition at line 53 of file katetextline.h.
Constructor & Destructor Documentation
KateTextLine::KateTextLine | ( | ) |
Constructor Creates an empty text line with given attribute and syntax highlight context.
Definition at line 30 of file katetextline.cpp.
KateTextLine::~KateTextLine | ( | ) |
Member Function Documentation
Gets the attribute at the given position use KRenderer::attributes to get the KTextAttribute for this.
- Parameters:
-
pos position of attribute requested
- Returns:
- value of attribute
- See also:
- attributes
Definition at line 271 of file katetextline.h.
uchar* KateTextLine::attributes | ( | ) | const [inline] |
Highlighting array The size of this is string().length().
This contains the index for the attributes (so you can only have a maximum of 2^8 different highlighting styles in a document)
To turn this into actual attributes (bold, green, etc), you need to feed these values into KRenderer::attributes
e.g: m_renderer->attributes(attributes[3]);
- Returns:
- hl-attributes array
Definition at line 167 of file katetextline.h.
const QMemArray<short>& KateTextLine::ctxArray | ( | ) | const [inline] |
Returns the x position of the cursor at the given position, which depends on the number of tab characters.
- Parameters:
-
pos position in chars tabChars tabulator width in chars
- Returns:
- position with tabulators calculated
Definition at line 237 of file katetextline.cpp.
char * KateTextLine::dump | ( | char * | buf, | |
bool | withHighlighting | |||
) | const |
Dumps the line to *buf and counts buff dumpSize bytes up as return value.
- Parameters:
-
buf buffer to dump to withHighlighting dump hl data, too?
- Returns:
- buffer index after dumping
Definition at line 335 of file katetextline.cpp.
Methodes for dump/restore of the line in the buffer.
Dumpsize in bytes
- Parameters:
-
withHighlighting should we dump the hl, too?
- Returns:
- size of line for dumping
Definition at line 384 of file katetextline.h.
Is the line ending with the given string.
- Parameters:
-
match string to test
- Returns:
- does the line end with given string?
Definition at line 219 of file katetextline.cpp.
int KateTextLine::firstChar | ( | ) | const |
Returns the position of the first non-whitespace character.
- Returns:
- position of first non-whitespace char or -1 if there is none
Definition at line 142 of file katetextline.cpp.
const QChar * KateTextLine::firstNonSpace | ( | ) | const |
Gets a null terminated pointer to first non space char.
- Returns:
- array of QChars starting at first non-whitespace char
Definition at line 152 of file katetextline.cpp.
bool KateTextLine::foldingColumnsOutdated | ( | ) | [inline] |
folding columns outdated ?
- Returns:
- folding columns outdated?
Definition at line 90 of file katetextline.h.
Gets the char at the given position.
- Parameters:
-
pos position
- Returns:
- character at the given position or QChar::null if position is beyond the length of the string
Definition at line 145 of file katetextline.h.
bool KateTextLine::hlLineContinue | ( | ) | const [inline] |
has the line the hl continue flag set
- Returns:
- hl continue set?
Definition at line 103 of file katetextline.h.
const QMemArray<unsigned short>& KateTextLine::indentationDepthArray | ( | ) | const [inline] |
indentation depth of this line
- Parameters:
-
tabwidth width of the tabulators
- Returns:
- indentation width
Definition at line 158 of file katetextline.cpp.
void KateTextLine::insertText | ( | uint | pos, | |
uint | insLen, | |||
const QChar * | insText, | |||
uchar * | insAttribs = 0 | |||
) |
insert text into line
- Parameters:
-
pos insert position insLen insert length insText text to insert insAttribs attributes for the insert text
Definition at line 39 of file katetextline.cpp.
bool KateTextLine::isAutoWrapped | ( | ) | const [inline] |
was this line automagically wrapped
- Returns:
- line auto-wrapped
Definition at line 109 of file katetextline.h.
int KateTextLine::lastChar | ( | ) | const |
Returns the position of the last non-whitespace character.
- Returns:
- position of last non-whitespace char or -1 if there is none
Definition at line 147 of file katetextline.cpp.
uint KateTextLine::length | ( | ) | const [inline] |
Returns the text length with tabs calced in.
- Parameters:
-
tabChars tabulator width in chars
- Returns:
- text length
Definition at line 256 of file katetextline.cpp.
Find the position of the next char that is not a space.
- Parameters:
-
pos Column of the character which is examined first.
- Returns:
- True if the specified or a following character is not a space Otherwise false.
Definition at line 110 of file katetextline.cpp.
const bool KateTextLine::noIndentBasedFolding | ( | ) | const [inline] |
- Returns:
- true if any context at the line end has the noIndentBasedFolding flag set
Definition at line 286 of file katetextline.h.
const bool KateTextLine::noIndentBasedFoldingAtStart | ( | ) | const [inline] |
Definition at line 287 of file katetextline.h.
Find the position of the previous char that is not a space.
- Parameters:
-
pos Column of the character which is examined first.
- Returns:
- The position of the first none-whitespace character preceeding pos, or -1 if none is found.
Definition at line 124 of file katetextline.cpp.
remove text at given position
- Parameters:
-
pos start position of remove delLen length to remove
Definition at line 76 of file katetextline.cpp.
char * KateTextLine::restore | ( | char * | buf | ) |
Restores the line from *buf and counts buff dumpSize bytes up as return value.
- Parameters:
-
buf buffer to restore from
- Returns:
- buffer index after restoring
Definition at line 383 of file katetextline.cpp.
bool KateTextLine::searchText | ( | uint | startCol, | |
const QRegExp & | regexp, | |||
uint * | foundAtCol, | |||
uint * | matchLen, | |||
bool | backwards = false | |||
) |
search given regexp
- Parameters:
-
startCol column to start search regexp regex to search for foundAtCol column where text was found matchLen length of matching backwards search backwards?
- Returns:
- regexp found?
Definition at line 304 of file katetextline.cpp.
bool KateTextLine::searchText | ( | uint | startCol, | |
const QString & | text, | |||
uint * | foundAtCol, | |||
uint * | matchLen, | |||
bool | casesensitive = true , |
|||
bool | backwards = false | |||
) |
search given string
- Parameters:
-
startCol column to start search text string to search for foundAtCol column where text was found matchLen length of matching casesensitive should search be case-sensitive backwards search backwards?
- Returns:
- string found?
Definition at line 273 of file katetextline.cpp.
void KateTextLine::setAutoWrapped | ( | bool | wrapped | ) | [inline] |
void KateTextLine::setContext | ( | QMemArray< short > & | val | ) | [inline] |
Sets the syntax highlight context number.
- Parameters:
-
val new context array
Definition at line 346 of file katetextline.h.
void KateTextLine::setFoldingColumnsOutdated | ( | bool | set | ) | [inline] |
Methods to get data.
Set the flag that only positions have changed, not folding region begins/ends themselve
Definition at line 83 of file katetextline.h.
void KateTextLine::setHlLineContinue | ( | bool | cont | ) | [inline] |
void KateTextLine::setIndentationDepth | ( | QMemArray< unsigned short > & | val | ) | [inline] |
update indentation stack
- Parameters:
-
val new indentation stack
Definition at line 373 of file katetextline.h.
void KateTextLine::setNoIndentBasedFolding | ( | bool | val | ) | [inline] |
sets if for the next line indent based folding should be disabled
Definition at line 351 of file katetextline.h.
void KateTextLine::setNoIndentBasedFoldingAtStart | ( | bool | val | ) | [inline] |
Definition at line 357 of file katetextline.h.
Is the line starting with the given string.
- Parameters:
-
match string to test
- Returns:
- does line start with given string?
Definition at line 202 of file katetextline.cpp.
Gets a substring.
- Parameters:
-
startCol start column of substring length length of substring
- Returns:
- wanted substring
Definition at line 181 of file katetextline.h.
const QString& KateTextLine::string | ( | ) | const [inline] |
Gets a QString.
- Returns:
- text of line as QString reference
Definition at line 173 of file katetextline.h.
Can we find the given string at the given position.
- Parameters:
-
pos startpostion of given string match string to match at given pos
- Returns:
- did the string match?
Definition at line 180 of file katetextline.cpp.
const QChar* KateTextLine::text | ( | ) | const [inline] |
Gets the text as a unicode representation.
- Returns:
- text of this line as QChar array
Definition at line 151 of file katetextline.h.
void KateTextLine::truncate | ( | uint | newLen | ) |
Truncates the textline to the new length.
- Parameters:
-
newLen new length of line
Definition at line 101 of file katetextline.cpp.
The documentation for this class was generated from the following files: