KDECore
KProcIO Class Reference
KProcIO. More...
#include <kprocio.h>
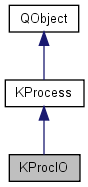
Signals | |
void | readReady (KProcIO *pio) |
Public Member Functions | |
void | ackRead () |
void | closeWhenDone () |
void | enableReadSignals (bool enable) |
KDE_DEPRECATED int | fgets (QString &line, bool autoAck=false) |
KDE_DEPRECATED bool | fputs (const QString &line, bool AppendNewLine=true) |
KProcIO (QTextCodec *codec=0) | |
int | readln (QString &line, bool autoAck=true, bool *partial=0) |
void | resetAll () |
void | setComm (Communication comm) |
bool | start (RunMode runmode=NotifyOnExit, bool includeStderr=false) |
bool | writeStdin (const QByteArray &data) |
bool | writeStdin (const QCString &line, bool appendnewline) |
bool | writeStdin (const QString &line, bool appendnewline=true) |
~KProcIO () | |
Protected Slots | |
void | received (KProcess *proc, char *buffer, int buflen) |
void | sent (KProcess *) |
Protected Member Functions | |
void | controlledEmission () |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
QTextCodec * | codec |
bool | needreadsignal |
QPtrList< QByteArray > | outbuffer |
int | rbi |
bool | readsignalon |
QCString | recvbuffer |
bool | writeready |
Detailed Description
KProcIO.This class provides a slightly simpler interface to the communication functions provided by KProcess. The simplifications are:
- The buffer for a write is copied to an internal KProcIO buffer and maintained/freed appropriately. There is no need to be concerned with wroteStdin() signals _at_all_.
- readln() reads a line of data and buffers any leftovers.
- Conversion from/to unicode.
Aside from these, and the fact that start() takes different parameters, use this class just like KProcess.
Definition at line 50 of file kprocio.h.
Constructor & Destructor Documentation
KProcIO::KProcIO | ( | QTextCodec * | codec = 0 |
) |
KProcIO::~KProcIO | ( | ) |
Member Function Documentation
void KProcIO::ackRead | ( | ) |
Call this after you have finished processing a readReady() signal.
This call need not be made in the slot that was signalled by readReady(). You won't receive any more readReady() signals until you acknowledge with ackRead(). This prevents your slot from being reentered while you are still processing the current data. If this doesn't matter, then call ackRead() right away in your readReady()-processing slot.
Definition at line 196 of file kprocio.cpp.
void KProcIO::closeWhenDone | ( | ) |
void KProcIO::controlledEmission | ( | ) | [protected] |
Definition at line 203 of file kprocio.cpp.
void KProcIO::enableReadSignals | ( | bool | enable | ) |
Turns readReady() signals on and off.
You can turn this off at will and not worry about losing any data. (as long as you turn it back on at some point...)
- Parameters:
-
enable true to turn the signals on, false to turn them off
Definition at line 217 of file kprocio.cpp.
This function calls readln().
- Parameters:
-
line is used to store the line that was read. autoAck when true, ackRead() is called for you.
- Returns:
- the number of characters read, or -1 if no data is available.
- Deprecated:
- use readln. Note that it has an inverted autoAck default, though.
This function just calls writeStdin().
- Parameters:
-
line Text to write. AppendNewLine if true, a newline '\n' is appended.
- Returns:
- true if successful, false otherwise
Reads a line of text (up to and including '\n').
Use readln() in response to a readReady() signal. You may use it multiple times if more than one line of data is available. Be sure to use ackRead() when you have finished processing the readReady() signal. This informs KProcIO that you are ready for another readReady() signal.
readln() never blocks.
autoAck==true makes these functions call ackRead() for you.
- Parameters:
-
line is used to store the line that was read. autoAck when true, ackRead() is called for you. partial when provided the line is returned even if it does not contain a '\n'. *partial will be set to false if the line contains a '\n' and false otherwise.
- Returns:
- the number of characters read, or -1 if no data is available.
Definition at line 225 of file kprocio.cpp.
void KProcIO::readReady | ( | KProcIO * | pio | ) | [signal] |
Emitted when the process is ready for reading.
- Parameters:
-
pio the process that emitted the signal
- See also:
- enableReadSignals()
void KProcIO::received | ( | KProcess * | proc, | |
char * | buffer, | |||
int | buflen | |||
) | [protected, slot] |
Definition at line 189 of file kprocio.cpp.
void KProcIO::resetAll | ( | ) |
void KProcIO::sent | ( | KProcess * | ) | [protected, slot] |
Definition at line 166 of file kprocio.cpp.
void KProcIO::setComm | ( | Communication | comm | ) |
Sets the communication mode to be passed to KProcess::start() by start().
The default communication mode is KProcess::All. You probably want to use this function in conjunction with KProcess::setUsePty().
- Parameters:
-
comm the communication mode
Definition at line 83 of file kprocio.cpp.
Starts the process.
It will fail in the following cases:
- The process is already running.
- The command line argument list is empty.
- The starting of the process failed (could not fork).
- The executable was not found.
- Parameters:
-
runmode For a detailed description of the various run modes, have a look at the general description of the KProcess class. includeStderr If true, data from both stdout and stderr is listened to. If false, only stdout is listened to.
- Returns:
- true on success, false on error.
Definition at line 88 of file kprocio.cpp.
void KProcIO::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
bool KProcIO::writeStdin | ( | const QByteArray & | data | ) |
Writes data to stdin of the process.
- Parameters:
-
data Data to write.
- Returns:
- true if successful, false otherwise
Definition at line 139 of file kprocio.cpp.
Writes text to stdin of the process.
- Parameters:
-
line Text to write. appendnewline if true, a newline '\n' is appended.
- Returns:
- true if successful, false otherwise
Definition at line 110 of file kprocio.cpp.
Writes text to stdin of the process.
- Parameters:
-
line Text to write. appendnewline if true, a newline '\n' is appended.
- Returns:
- true if successful, false otherwise
Definition at line 105 of file kprocio.cpp.
Member Data Documentation
QTextCodec* KProcIO::codec [protected] |
bool KProcIO::needreadsignal [protected] |
QPtrList<QByteArray> KProcIO::outbuffer [protected] |
int KProcIO::rbi [protected] |
bool KProcIO::readsignalon [protected] |
QCString KProcIO::recvbuffer [protected] |
bool KProcIO::writeready [protected] |
The documentation for this class was generated from the following files: