kdefx
KStyle Class Reference
Simplifies and extends the QStyle API to make style coding easier. More...
#include <kstyle.h>
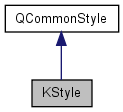
Public Types | |
typedef uint | KStyleFlags |
enum | KStyleOption { Default = 0x00000000, AllowMenuTransparency = 0x00000001, FilledFrameWorkaround = 0x00000002 } |
enum | KStylePixelMetric { KPM_MenuItemSeparatorHeight = 0x00000001, KPM_MenuItemHMargin = 0x00000002, KPM_MenuItemVMargin = 0x00000004, KPM_MenuItemHFrame = 0x00000008, KPM_MenuItemVFrame = 0x00000010, KPM_MenuItemCheckMarkHMargin = 0x00000020, KPM_MenuItemArrowHMargin = 0x00000040, KPM_MenuItemTabSpacing = 0x00000080, KPM_ListViewBranchThickness = 0x00000100 } |
enum | KStylePrimitive { KPE_DockWindowHandle, KPE_ToolBarHandle, KPE_GeneralHandle, KPE_SliderGroove, KPE_SliderHandle, KPE_ListViewExpander, KPE_ListViewBranch } |
enum | KStyleScrollBarType { WindowsStyleScrollBar = 0x00000000, PlatinumStyleScrollBar = 0x00000001, ThreeButtonScrollBar = 0x00000002, NextStyleScrollBar = 0x00000004 } |
Public Member Functions | |
void | drawComplexControl (ComplexControl control, QPainter *p, const QWidget *widget, const QRect &r, const QColorGroup &cg, SFlags flags=Style_Default, SCFlags controls=SC_All, SCFlags active=SC_None, const QStyleOption &=QStyleOption::Default) const |
void | drawControl (ControlElement element, QPainter *p, const QWidget *widget, const QRect &r, const QColorGroup &cg, SFlags flags=Style_Default, const QStyleOption &=QStyleOption::Default) const |
virtual void | drawKStylePrimitive (KStylePrimitive kpe, QPainter *p, const QWidget *widget, const QRect &r, const QColorGroup &cg, SFlags flags=Style_Default, const QStyleOption &=QStyleOption::Default) const |
void | drawPrimitive (PrimitiveElement pe, QPainter *p, const QRect &r, const QColorGroup &cg, SFlags flags=Style_Default, const QStyleOption &=QStyleOption::Default) const |
int | kPixelMetric (KStylePixelMetric kpm, const QWidget *widget=0) const |
KStyle (KStyleFlags flags=KStyle::Default, KStyleScrollBarType sbtype=KStyle::WindowsStyleScrollBar) | |
int | pixelMetric (PixelMetric m, const QWidget *widget=0) const |
void | polish (QWidget *widget) |
void | polishPopupMenu (QPopupMenu *) |
SubControl | querySubControl (ComplexControl control, const QWidget *widget, const QPoint &pos, const QStyleOption &=QStyleOption::Default) const |
QRect | querySubControlMetrics (ComplexControl control, const QWidget *widget, SubControl sc, const QStyleOption &=QStyleOption::Default) const |
virtual void | renderMenuBlendPixmap (KPixmap &pix, const QColorGroup &cg, const QPopupMenu *popup) const |
void | setScrollBarType (KStyleScrollBarType sbtype) |
KStyleFlags | styleFlags () const |
int | styleHint (StyleHint sh, const QWidget *w=0, const QStyleOption &opt=QStyleOption::Default, QStyleHintReturn *shr=0) const |
QPixmap | stylePixmap (StylePixmap stylepixmap, const QWidget *widget=0, const QStyleOption &=QStyleOption::Default) const |
QRect | subRect (SubRect r, const QWidget *widget) const |
void | unPolish (QWidget *widget) |
~KStyle () | |
Static Public Member Functions | |
static QString | defaultStyle () |
Protected Member Functions | |
bool | eventFilter (QObject *object, QEvent *event) |
virtual void | virtual_hook (int id, void *data) |
Related Functions | |
(Note that these are not member functions.) | |
KDEFX_EXPORT void | kColorBitmaps (QPainter *p, const QColorGroup &g, int x, int y, int w, int h, bool isXBitmaps=true, const uchar *lightColor=0, const uchar *midColor=0, const uchar *midlightColor=0, const uchar *darkColor=0, const uchar *blackColor=0, const uchar *whiteColor=0) |
KDEFX_EXPORT void | kColorBitmaps (QPainter *p, const QColorGroup &g, int x, int y, QBitmap *lightColor=0, QBitmap *midColor=0, QBitmap *midlightColor=0, QBitmap *darkColor=0, QBitmap *blackColor=0, QBitmap *whiteColor=0) |
KDEFX_EXPORT void | kDrawBeButton (QPainter *p, int x, int y, int w, int h, const QColorGroup &g, bool sunken=false, const QBrush *fill=0) |
KDEFX_EXPORT void | kDrawBeButton (QPainter *p, QRect &r, const QColorGroup &g, bool sunken=false, const QBrush *fill=0) |
KDEFX_EXPORT void | kDrawNextButton (QPainter *p, int x, int y, int w, int h, const QColorGroup &g, bool sunken=false, const QBrush *fill=0) |
KDEFX_EXPORT void | kDrawNextButton (QPainter *p, const QRect &r, const QColorGroup &g, bool sunken=false, const QBrush *fill=0) |
KDEFX_EXPORT void | kDrawRoundButton (QPainter *p, int x, int y, int w, int h, const QColorGroup &g, bool sunken=false) |
KDEFX_EXPORT void | kDrawRoundButton (QPainter *p, const QRect &r, const QColorGroup &g, bool sunken=false) |
KDEFX_EXPORT void | kDrawRoundMask (QPainter *p, int x, int y, int w, int h, bool clear=false) |
KDEFX_EXPORT void | kRoundMaskRegion (QRegion &r, int x, int y, int w, int h) |
Detailed Description
Simplifies and extends the QStyle API to make style coding easier.The KStyle class provides a simple internal menu transparency engine which attempts to use XRender for accelerated blending where requested, or falls back to fast internal software tinting/blending routines. It also simplifies more complex portions of the QStyle API, such as the PopupMenuItems, ScrollBars and Sliders by providing extra "primitive elements" which are simple to implement by the style writer.
- See also:
- QStyle::QStyle
- Version:
- Id
- kstyle.h 465272 2005-09-29 09:47:40Z mueller
Definition at line 57 of file kstyle.h.
Member Typedef Documentation
typedef uint KStyle::KStyleFlags |
KStyle Flags:.
- Default - Default style setting, where menu transparency and the FilledFrameWorkaround are disabled.
- AllowMenuTransparency - Enable this flag to use KStyle's internal menu transparency engine.
- FilledFrameWorkaround - Enable this flag to facilitate proper repaints of QMenuBars and QToolBars when the style chooses to paint the interior of a QFrame. The style primitives in question are PE_PanelMenuBar and PE_PanelDockWindow. The HighColor style uses this workaround to enable painting of gradients in menubars and toolbars.
Member Enumeration Documentation
enum KStyle::KStyleOption |
KStyle Primitive Elements:.
The KStyle class extends the Qt's Style API by providing certain simplifications for parts of QStyle. To do this, the KStylePrimitive elements were defined, which are very similar to Qt's PrimitiveElement.
The first three Handle primitives simplify and extend PE_DockWindowHandle, so do not reimplement PE_DockWindowHandle if you want the KStyle handle simplifications to be operable. Similarly do not reimplement CC_Slider, SC_SliderGroove and SC_SliderHandle when using the KStyle slider primitives. KStyle automatically double-buffers slider painting when they are drawn via these KStyle primitives to avoid flicker.
- KPE_DockWindowHandle - This primitive is already implemented in KStyle, and paints a bevelled rect with the DockWindow caption text. Re-implement this primitive to perform other more fancy effects when drawing the dock window handle.
- KPE_ToolBarHandle - This primitive must be reimplemented. It currently only paints a filled rectangle as default behavior. This primitive is used to render QToolBar handles.
- KPE_GeneralHandle - This primitive must be reimplemented. It is used to render general handles that are not part of a QToolBar or QDockWindow, such as the applet handles used in Kicker. The default implementation paints a filled rect of arbitrary color.
- KPE_SliderGroove - This primitive must be reimplemented. It is used to paint the slider groove. The default implementation paints a filled rect of arbitrary color.
- KPE_SliderHandle - This primitive must be reimplemented. It is used to paint the slider handle. The default implementation paints a filled rect of arbitrary color.
- KPE_ListViewExpander - This primitive is already implemented in KStyle. It is used to draw the Expand/Collapse element in QListViews. To indicate the expanded state, the style flags are set to Style_Off, while Style_On implies collapsed.
- KPE_ListViewBranch - This primitive is already implemented in KStyle. It is used to draw the ListView branches where necessary.
KStyle ScrollBarType:.
Allows the style writer to easily select what type of scrollbar should be used without having to duplicate large amounts of source code by implementing the complex control CC_ScrollBar.
- WindowsStyleScrollBar - Two button scrollbar with the previous button at the top/left, and the next button at the bottom/right.
- PlatinumStyleScrollBar - Two button scrollbar with both the previous and next buttons at the bottom/right.
- ThreeButtonScrollBar - KDE style three button scrollbar with two previous buttons, and one next button. The next button is always at the bottom/right, whilst the two previous buttons are on either end of the scrollbar.
- NextStyleScrollBar - Similar to the PlatinumStyle scroll bar, but with the buttons grouped on the opposite end of the scrollbar.
- See also:
- KStyle::KStyle()
Constructor & Destructor Documentation
KStyle::KStyle | ( | KStyleFlags | flags = KStyle::Default , |
|
KStyleScrollBarType | sbtype = KStyle::WindowsStyleScrollBar | |||
) |
Constructs a KStyle object.
Select the appropriate KStyle flags and scrollbar type for your style. The user's style preferences selected in KControl are read by using QSettings and are automatically applied to the style. As a fallback, KStyle paints progressbars and tabbars. It inherits from QCommonStyle for speed, so don't expect much to be implemented.
It is advisable to use a currently implemented style such as the HighColor style as a foundation for any new KStyle, so the limited number of drawing fallbacks should not prove problematic.
- Parameters:
-
flags the style to be applied sbtype the scroll bar type
Definition at line 173 of file kstyle.cpp.
KStyle::~KStyle | ( | ) |
Member Function Documentation
QString KStyle::defaultStyle | ( | ) | [static] |
Returns the default widget style depending on color depth.
Definition at line 238 of file kstyle.cpp.
void KStyle::drawComplexControl | ( | ComplexControl | control, | |
QPainter * | p, | |||
const QWidget * | widget, | |||
const QRect & | r, | |||
const QColorGroup & | cg, | |||
SFlags | flags = Style_Default , |
|||
SCFlags | controls = SC_All , |
|||
SCFlags | active = SC_None , |
|||
const QStyleOption & | opt = QStyleOption::Default | |||
) | const |
Definition at line 1013 of file kstyle.cpp.
void KStyle::drawControl | ( | ControlElement | element, | |
QPainter * | p, | |||
const QWidget * | widget, | |||
const QRect & | r, | |||
const QColorGroup & | cg, | |||
SFlags | flags = Style_Default , |
|||
const QStyleOption & | opt = QStyleOption::Default | |||
) | const |
Definition at line 563 of file kstyle.cpp.
void KStyle::drawKStylePrimitive | ( | KStylePrimitive | kpe, | |
QPainter * | p, | |||
const QWidget * | widget, | |||
const QRect & | r, | |||
const QColorGroup & | cg, | |||
SFlags | flags = Style_Default , |
|||
const QStyleOption & | = QStyleOption::Default | |||
) | const [virtual] |
This function is identical to Qt's QStyle::drawPrimitive(), except that it adds one further parameter, 'widget', that can be used to determine the widget state of the KStylePrimitive in question.
Definition at line 307 of file kstyle.cpp.
void KStyle::drawPrimitive | ( | PrimitiveElement | pe, | |
QPainter * | p, | |||
const QRect & | r, | |||
const QColorGroup & | cg, | |||
SFlags | flags = Style_Default , |
|||
const QStyleOption & | opt = QStyleOption::Default | |||
) | const |
Definition at line 520 of file kstyle.cpp.
Definition at line 1798 of file kstyle.cpp.
int KStyle::kPixelMetric | ( | KStylePixelMetric | kpm, | |
const QWidget * | widget = 0 | |||
) | const |
Definition at line 493 of file kstyle.cpp.
int KStyle::pixelMetric | ( | PixelMetric | m, | |
const QWidget * | widget = 0 | |||
) | const |
Definition at line 888 of file kstyle.cpp.
void KStyle::polish | ( | QWidget * | widget | ) |
Definition at line 247 of file kstyle.cpp.
void KStyle::polishPopupMenu | ( | QPopupMenu * | p | ) |
Definition at line 274 of file kstyle.cpp.
QStyle::SubControl KStyle::querySubControl | ( | ComplexControl | control, | |
const QWidget * | widget, | |||
const QPoint & | pos, | |||
const QStyleOption & | opt = QStyleOption::Default | |||
) | const |
Definition at line 1309 of file kstyle.cpp.
QRect KStyle::querySubControlMetrics | ( | ComplexControl | control, | |
const QWidget * | widget, | |||
SubControl | sc, | |||
const QStyleOption & | opt = QStyleOption::Default | |||
) | const |
Definition at line 1325 of file kstyle.cpp.
void KStyle::renderMenuBlendPixmap | ( | KPixmap & | pix, | |
const QColorGroup & | cg, | |||
const QPopupMenu * | popup | |||
) | const [virtual] |
This virtual function defines the pixmap used to blend between the popup menu and the background to create different menu transparency effects.
For example, you can fill the pixmap "pix" with a gradient based on the popup's colorGroup, a texture, or some other fancy painting routine. KStyle will then internally blend this pixmap with a snapshot of the background behind the popupMenu to create the illusion of transparency.
This virtual is never called if XRender/Software blending is disabled by the user in KDE's style control module.
Definition at line 300 of file kstyle.cpp.
void KStyle::setScrollBarType | ( | KStyleScrollBarType | sbtype | ) |
Modifies the scrollbar type used by the style.
This function is only provided for convenience. It allows you to make a late decision about what scrollbar type to use for the style after performing some processing in your style's constructor. In most situations however, setting the scrollbar type via the KStyle constructor should suffice.
- Parameters:
-
sbtype the scroll bar type
- See also:
- KStyle::KStyleScrollBarType
Definition at line 290 of file kstyle.cpp.
KStyle::KStyleFlags KStyle::styleFlags | ( | ) | const |
Returns the KStyle flags used to initialize the style.
This is used solely for the kcmstyle module, and hence is internal.
Definition at line 295 of file kstyle.cpp.
int KStyle::styleHint | ( | StyleHint | sh, | |
const QWidget * | w = 0 , |
|||
const QStyleOption & | opt = QStyleOption::Default , |
|||
QStyleHintReturn * | shr = 0 | |||
) | const |
Definition at line 1742 of file kstyle.cpp.
QPixmap KStyle::stylePixmap | ( | StylePixmap | stylepixmap, | |
const QWidget * | widget = 0 , |
|||
const QStyleOption & | opt = QStyleOption::Default | |||
) | const |
Definition at line 1710 of file kstyle.cpp.
Definition at line 866 of file kstyle.cpp.
void KStyle::unPolish | ( | QWidget * | widget | ) |
Definition at line 260 of file kstyle.cpp.
void KStyle::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 2158 of file kstyle.cpp.
Friends And Related Function Documentation
KDEFX_EXPORT void kColorBitmaps | ( | QPainter * | p, | |
const QColorGroup & | g, | |||
int | x, | |||
int | y, | |||
int | w, | |||
int | h, | |||
bool | isXBitmaps = true , |
|||
const uchar * | lightColor = 0 , |
|||
const uchar * | midColor = 0 , |
|||
const uchar * | midlightColor = 0 , |
|||
const uchar * | darkColor = 0 , |
|||
const uchar * | blackColor = 0 , |
|||
const uchar * | whiteColor = 0 | |||
) | [related] |
#include
<kdrawutil.h>
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 239 of file kdrawutil.cpp.
KDEFX_EXPORT void kColorBitmaps | ( | QPainter * | p, | |
const QColorGroup & | g, | |||
int | x, | |||
int | y, | |||
QBitmap * | lightColor = 0 , |
|||
QBitmap * | midColor = 0 , |
|||
QBitmap * | midlightColor = 0 , |
|||
QBitmap * | darkColor = 0 , |
|||
QBitmap * | blackColor = 0 , |
|||
QBitmap * | whiteColor = 0 | |||
) | [related] |
#include
<kdrawutil.h>
Paints the provided bitmaps in the painter, using the supplied colorgroup for the foreground colors. There's one bitmap for each color. If you want to skip a color, pass null for the corresponding bitmap.
- Note:
- The bitmaps will be self-masked automatically if not masked prior to calling this routine.
- Parameters:
-
p The painter to use for drawing the bitmaps. g Specifies the shading colors. x The X coordinate at which to draw the bitmaps. y The Y coordinate at which to draw the bitmaps. lightColor The bitmap to use for the light part. midColor The bitmap to use for the mid part. midlightColor The bitmap to use for the midlight part. darkColor The bitmap to use for the dark part. blackColor The bitmap to use for the black part. whiteColor The bitmap to use for the white part.
- See also:
- QColorGroup
Definition at line 217 of file kdrawutil.cpp.
KDEFX_EXPORT void kDrawBeButton | ( | QPainter * | p, | |
int | x, | |||
int | y, | |||
int | w, | |||
int | h, | |||
const QColorGroup & | g, | |||
bool | sunken = false , |
|||
const QBrush * | fill = 0 | |||
) | [related] |
#include
<kdrawutil.h>
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 49 of file kdrawutil.cpp.
KDEFX_EXPORT void kDrawBeButton | ( | QPainter * | p, | |
QRect & | r, | |||
const QColorGroup & | g, | |||
bool | sunken = false , |
|||
const QBrush * | fill = 0 | |||
) | [related] |
#include
<kdrawutil.h>
Draws a Be-style button.
- Parameters:
-
p The painter to use for drawing the button. r Specifies the rect in which to draw the button. g Specifies the shading colors. sunken Whether to draw the button as sunken (pressed) or not. fill The brush to use for filling the interior of the button. Pass null to prevent the button from being filled.
Definition at line 93 of file kdrawutil.cpp.
KDEFX_EXPORT void kDrawNextButton | ( | QPainter * | p, | |
int | x, | |||
int | y, | |||
int | w, | |||
int | h, | |||
const QColorGroup & | g, | |||
bool | sunken = false , |
|||
const QBrush * | fill = 0 | |||
) | [related] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 21 of file kdrawutil.cpp.
KDEFX_EXPORT void kDrawNextButton | ( | QPainter * | p, | |
const QRect & | r, | |||
const QColorGroup & | g, | |||
bool | sunken = false , |
|||
const QBrush * | fill = 0 | |||
) | [related] |
#include
<kdrawutil.h>
Draws a Next-style button (solid black shadow with light and midlight highlight).
- Parameters:
-
p The painter to use for drawing the button. r Specifies the rect in which to draw the button. g Specifies the shading colors. sunken Whether to draw the button as sunken (pressed) or not. fill The brush to use for filling the interior of the button. Pass null to prevent the button from being filled.
Definition at line 43 of file kdrawutil.cpp.
KDEFX_EXPORT void kDrawRoundButton | ( | QPainter * | p, | |
int | x, | |||
int | y, | |||
int | w, | |||
int | h, | |||
const QColorGroup & | g, | |||
bool | sunken = false | |||
) | [related] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 139 of file kdrawutil.cpp.
KDEFX_EXPORT void kDrawRoundButton | ( | QPainter * | p, | |
const QRect & | r, | |||
const QColorGroup & | g, | |||
bool | sunken = false | |||
) | [related] |
#include
<kdrawutil.h>
Draws a rounded oval button. This function doesn't fill the button. See kRoundMaskRegion() for setting masks for fills.
- Parameters:
-
p The painter to use for drawing the button. r Specifies the rect in which to draw the button. g Specifies the shading colors. sunken Whether to draw the button as sunken (pressed) or not.
Definition at line 99 of file kdrawutil.cpp.
KDEFX_EXPORT void kDrawRoundMask | ( | QPainter * | p, | |
int | x, | |||
int | y, | |||
int | w, | |||
int | h, | |||
bool | clear = false | |||
) | [related] |
#include
<kdrawutil.h>
Paints the pixels covered by a round button of the given size with Qt::color1. This function is useful in QStyle::drawControlMask().
- Parameters:
-
p The painter to use for drawing the button. x The X coordinate of the button. y The Y coordinate of the button. w The width of the button. h The height of the button. clear Whether to clear the rectangle specified by (x, y, w, h) to Qt::color0 before drawing the mask.
Definition at line 148 of file kdrawutil.cpp.
KDEFX_EXPORT void kRoundMaskRegion | ( | QRegion & | r, | |
int | x, | |||
int | y, | |||
int | w, | |||
int | h | |||
) | [related] |
#include
<kdrawutil.h>
Sets a region to the pixels covered by a round button of the given size. You can use this to set clipping regions.
- Parameters:
-
r Reference to the region to set. x The X coordinate of the button. y The Y coordinate of the button. w The width of the button. h The height of the button.
- See also:
- kDrawRoundButton() and kDrawRoundMask()
Definition at line 197 of file kdrawutil.cpp.
The documentation for this class was generated from the following files: