kdefx
kimageeffect.cpp File Reference
#include <math.h>
#include <assert.h>
#include <qimage.h>
#include <stdlib.h>
#include <iostream>
#include "kimageeffect.h"
#include "kcpuinfo.h"
#include <config.h>
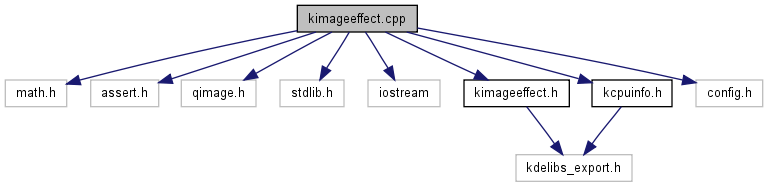
Go to the source code of this file.
Defines | |
#define | DegreesToRadians(x) ((x)*M_PI/180.0) |
#define | FXCLAMP(x, low, high) fxClamp(x,low,high) |
#define | KernelRank 3 |
#define | MagickEpsilon 1.0e-12 |
#define | MagickPI 3.14159265358979323846264338327950288419716939937510 |
#define | MagickSQ2PI 2.50662827463100024161235523934010416269302368164062 |
#define | MaxRGB 255L |
#define | MOD(x, y) ((x) < 0 ? ((y) - 1 - ((y) - 1 - (x)) % (y)) : (x) % (y)) |
#define | NoiseEpsilon 1.0e-5 |
#define | NoiseMask 0x7fff |
#define | SigmaGaussian 4.0 |
#define | SigmaImpulse 0.10 |
#define | SigmaLaplacian 10.0 |
#define | SigmaMultiplicativeGaussian 0.5 |
#define | SigmaPoisson 0.05 |
#define | SigmaUniform 4.0 |
#define | TauGaussian 20.0 |
Functions | |
static void | bumpmap_convert_row (uint *row, int width, int bpp, int has_alpha, uchar *lut, int waterlevel) |
static void | bumpmap_row (uint *src, uint *dest, int width, int bpp, int has_alpha, uint *bm_row1, uint *bm_row2, uint *bm_row3, int bm_width, int bm_xofs, bool tiled, bool row_in_bumpmap, int ambient, bool compensate, BumpmapParams *params) |
template<class T > | |
const T & | fxClamp (const T &x, const T &low, const T &high) |
static unsigned int | intensityValue (unsigned int color) |
template<typename T > | |
static void | liberateMemory (T **memory) |
Define Documentation
#define DegreesToRadians | ( | x | ) | ((x)*M_PI/180.0) |
Definition at line 65 of file kimageeffect.cpp.
#define FXCLAMP | ( | x, | |||
low, | |||||
high | ) | fxClamp(x,low,high) |
A typesafe function that returns x if it's between low and high values. low if x is smaller than then low and high if x is bigger than high.
Definition at line 76 of file kimageeffect.cpp.
#define KernelRank 3 |
#define MagickEpsilon 1.0e-12 |
Definition at line 67 of file kimageeffect.cpp.
#define MagickPI 3.14159265358979323846264338327950288419716939937510 |
Definition at line 68 of file kimageeffect.cpp.
#define MagickSQ2PI 2.50662827463100024161235523934010416269302368164062 |
Definition at line 66 of file kimageeffect.cpp.
#define MaxRGB 255L |
Definition at line 64 of file kimageeffect.cpp.
#define MOD | ( | x, | |||
y | ) | ((x) < 0 ? ((y) - 1 - ((y) - 1 - (x)) % (y)) : (x) % (y)) |
Definition at line 69 of file kimageeffect.cpp.
#define NoiseEpsilon 1.0e-5 |
#define NoiseMask 0x7fff |
#define SigmaGaussian 4.0 |
#define SigmaImpulse 0.10 |
#define SigmaLaplacian 10.0 |
#define SigmaMultiplicativeGaussian 0.5 |
#define SigmaPoisson 0.05 |
#define SigmaUniform 4.0 |
#define TauGaussian 20.0 |
Function Documentation
static void bumpmap_convert_row | ( | uint * | row, | |
int | width, | |||
int | bpp, | |||
int | has_alpha, | |||
uchar * | lut, | |||
int | waterlevel | |||
) | [static] |
Definition at line 4709 of file kimageeffect.cpp.
static void bumpmap_row | ( | uint * | src, | |
uint * | dest, | |||
int | width, | |||
int | bpp, | |||
int | has_alpha, | |||
uint * | bm_row1, | |||
uint * | bm_row2, | |||
uint * | bm_row3, | |||
int | bm_width, | |||
int | bm_xofs, | |||
bool | tiled, | |||
bool | row_in_bumpmap, | |||
int | ambient, | |||
bool | compensate, | |||
BumpmapParams * | params | |||
) | [static] |
NOTE: if we want to work with non-32bit images the alpha handling would also change
Definition at line 4739 of file kimageeffect.cpp.
const T& fxClamp | ( | const T & | x, | |
const T & | low, | |||
const T & | high | |||
) | [inline] |
Definition at line 78 of file kimageeffect.cpp.
static unsigned int intensityValue | ( | unsigned int | color | ) | [inline, static] |
Definition at line 85 of file kimageeffect.cpp.
static void liberateMemory | ( | T ** | memory | ) | [inline, static] |
Definition at line 93 of file kimageeffect.cpp.