KDEsu
PtyProcess Class Reference
Synchronous communication with tty programs. More...
#include <process.h>
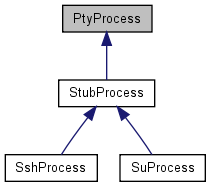
Public Types | |
enum | checkPidStatus { Error = -1, NotExited = -2, Killed = -3 } |
Public Member Functions | |
int | enableLocalEcho (bool enable=true) |
int | exec (const QCString &command, const QCStringList &args) |
int | fd () |
int | pid () |
PtyProcess () | |
QCString | readAll (bool block=true) |
QCString | readLine (bool block=true) |
void | setEnvironment (const QCStringList &env) |
void | setErase (bool erase) |
void | setExitString (const QCString &exit) |
void | setTerminal (bool terminal) |
void | unreadLine (const QCString &line, bool addNewline=true) |
int | waitForChild () |
int | WaitSlave () |
void | writeLine (const QCString &line, bool addNewline=true) |
virtual | ~PtyProcess () |
Static Public Member Functions | |
static bool | checkPid (pid_t pid) |
static int | checkPidExited (pid_t pid) |
static int | waitMS (int fd, int ms) |
Protected Member Functions | |
const QCStringList & | environment () const |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
bool | m_bErase |
bool | m_bTerminal |
QCString | m_Command |
QCString | m_Exit |
int | m_Fd |
int | m_Pid |
Detailed Description
Synchronous communication with tty programs.PtyProcess provides synchronous communication with tty based programs. The communications channel used is a pseudo tty (as opposed to a pipe) This means that programs which require a terminal will work.
Definition at line 36 of file process.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
PtyProcess::PtyProcess | ( | ) |
Definition at line 124 of file process.cpp.
PtyProcess::~PtyProcess | ( | ) | [virtual] |
Definition at line 152 of file process.cpp.
Member Function Documentation
bool PtyProcess::checkPid | ( | pid_t | pid | ) | [static] |
Basic check for the existence of pid
.
Returns true iff pid
is an extant process, (one you could kill - see man kill(2) for signal 0).
Definition at line 75 of file process.cpp.
int PtyProcess::checkPidExited | ( | pid_t | pid | ) | [static] |
Definition at line 96 of file process.cpp.
const QCStringList & PtyProcess::environment | ( | ) | const [protected] |
Definition at line 164 of file process.cpp.
int PtyProcess::exec | ( | const QCString & | command, | |
const QCStringList & | args | |||
) |
Forks off and execute a command.
The command's standard in and output are connected to the pseudo tty. They are accessible with readLine and writeLine.
- Parameters:
-
command The command to execute. args The arguments to the command.
Definition at line 324 of file process.cpp.
int PtyProcess::fd | ( | ) | [inline] |
int PtyProcess::pid | ( | ) | [inline] |
Read all available output from the program's standard out.
- Parameters:
-
block If no output is in the buffer, should the function block
- Returns:
- The output.
Definition at line 247 of file process.cpp.
Reads a line from the program's standard out.
Depending on the block parameter, this call blocks until a single, full line is read.
- Parameters:
-
block Block until a full line is read?
- Returns:
- The output string.
Definition at line 175 of file process.cpp.
void PtyProcess::setEnvironment | ( | const QCStringList & | env | ) |
void PtyProcess::setErase | ( | bool | erase | ) | [inline] |
void PtyProcess::setExitString | ( | const QCString & | exit | ) | [inline] |
Sets the exit string.
If a line of program output matches this, waitForChild() will terminate the program and return.
void PtyProcess::setTerminal | ( | bool | terminal | ) | [inline] |
Puts back a line of input.
- Parameters:
-
line The line to put back. addNewline Adds a '
' to the line.
Definition at line 311 of file process.cpp.
void PtyProcess::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Reimplemented in SshProcess, StubProcess, and SuProcess.
Definition at line 625 of file process.cpp.
int PtyProcess::waitForChild | ( | ) |
Wait ms
miliseconds (ie.
1/10th of a second is 100ms), using fd
as a filedescriptor to wait on. Returns select(2)'s result, which is -1 on error, 0 on timeout, or positive if there is data on one of the selected fd's.
ms
must be in the range 0..999 (ie. the maximum wait duration is 999ms, almost one second).
Definition at line 59 of file process.cpp.
int PtyProcess::WaitSlave | ( | ) |
Waits until the pty has cleared the ECHO flag.
This is useful when programs write a password prompt before they disable ECHO. Disabling it might flush any input that was written.
Definition at line 412 of file process.cpp.
Writes a line of text to the program's standard in.
- Parameters:
-
line The text to write. addNewline Adds a '
' to the line.
Definition at line 302 of file process.cpp.
Member Data Documentation
bool PtyProcess::m_bErase [protected] |
bool PtyProcess::m_bTerminal [protected] |
QCString PtyProcess::m_Command [protected] |
QCString PtyProcess::m_Exit [protected] |
int PtyProcess::m_Fd [protected] |
int PtyProcess::m_Pid [protected] |
The documentation for this class was generated from the following files: