kdeui
KActionCollection Class Reference
A managed set of KAction objects. More...
#include <kactioncollection.h>
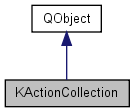
Public Slots | |
void | clear () |
Signals | |
void | actionHighlighted (KAction *action, bool highlight) |
void | actionHighlighted (KAction *action) |
void | actionStatusText (const QString &text) |
void | clearStatusText () |
void | inserted (KAction *) |
void | removed (KAction *) |
Public Member Functions | |
virtual const KAccel * | accel () const KDE_DEPRECATED |
virtual KAccel * | accel () KDE_DEPRECATED |
virtual KAction * | action (const char *name, const char *classname=0) const |
virtual KAction * | action (int index) const |
virtual KActionPtrList | actions () const |
virtual KActionPtrList | actions (const QString &group) const |
bool | addDocCollection (KActionCollection *pDoc) |
KAccel * | builderKAccel () const |
void | connectHighlight (QWidget *container, KAction *action) |
virtual uint | count () const |
void | disconnectHighlight (QWidget *container, KAction *action) |
virtual QStringList | groups () const |
bool | highlightingEnabled () const |
void | insert (KAction *action) |
KInstance * | instance () const |
bool | isAutoConnectShortcuts () |
bool | isEmpty () const |
const KAccel * | kaccel () const |
KAccel * | kaccel () |
KActionCollection (QObject *parent, const char *name=0, KInstance *instance=0) | |
KActionCollection (const KActionCollection ©) | |
KActionCollection (QWidget *watch, QObject *parent, const char *name=0, KInstance *instance=0) | |
KActionCollection (QWidget *parent, const char *name=0, KInstance *instance=0) | |
KActionCollection | operator+ (const KActionCollection &) const |
KActionCollection & | operator+= (const KActionCollection &) |
KActionCollection & | operator= (const KActionCollection &) |
const KXMLGUIClient * | parentGUIClient () const |
bool | readShortcutSettings (const QString &sConfigGroup=QString::null, KConfigBase *pConfig=0) |
void | remove (KAction *action) |
void | setAutoConnectShortcuts (bool) |
void | setHighlightingEnabled (bool enable) |
void | setInstance (KInstance *instance) |
virtual void | setWidget (QWidget *widget) |
void | setXMLFile (const QString &) |
KAction * | take (KAction *action) |
bool | writeShortcutSettings (const QString &sConfigGroup=QString::null, KConfigBase *pConfig=0) const |
const QString & | xmlFile () const |
virtual | ~KActionCollection () |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
A managed set of KAction objects.If you set the tooltips on KActions and want the tooltip to show in statusbar (recommended) then you will need to connect a couple of the actionclass signals to the toolbar. The easiest way of doing this is in your KMainWindow subclass, where you create a statusbar, do:
actionCollection()->setHighlightingEnabled(true); connect(actionCollection(), SIGNAL( actionStatusText( const QString & ) ), statusBar(), SLOT( message( const QString & ) ) ); connect(actionCollection(), SIGNAL( clearStatusText() ), statusBar(), SLOT( clear() ) );
Definition at line 78 of file kactioncollection.h.
Constructor & Destructor Documentation
KActionCollection::KActionCollection | ( | QWidget * | parent, | |
const char * | name = 0 , |
|||
KInstance * | instance = 0 | |||
) |
Definition at line 79 of file kactioncollection.cpp.
KActionCollection::KActionCollection | ( | QWidget * | watch, | |
QObject * | parent, | |||
const char * | name = 0 , |
|||
KInstance * | instance = 0 | |||
) |
Use this constructor if you want the collection's actions to restrict their accelerator keys to watch
rather than the parent
.
If you don't require shortcuts, you can pass a null to the watch
parameter.
Definition at line 92 of file kactioncollection.cpp.
KActionCollection::KActionCollection | ( | const KActionCollection & | copy | ) |
Definition at line 120 of file kactioncollection.cpp.
KActionCollection::~KActionCollection | ( | ) | [virtual] |
Definition at line 138 of file kactioncollection.cpp.
KActionCollection::KActionCollection | ( | QObject * | parent, | |
const char * | name = 0 , |
|||
KInstance * | instance = 0 | |||
) |
Definition at line 106 of file kactioncollection.cpp.
Member Function Documentation
const KAccel * KActionCollection::accel | ( | ) | const [virtual] |
- Deprecated:
- Deprecated because of ambiguous name. Use kaccel()
Definition at line 370 of file kactioncollection.cpp.
KAccel * KActionCollection::accel | ( | ) | [virtual] |
Returns the number of widgets which this collection is associated with.
Returns true if the collection has its own KAccel object. This will be the case if it was constructed with a valid widget ptr or if setWidget() was called.
- Deprecated:
- Deprecated because of ambiguous name. Use kaccel()
Definition at line 369 of file kactioncollection.cpp.
KAction * KActionCollection::action | ( | const char * | name, | |
const char * | classname = 0 | |||
) | const [virtual] |
Find an action (optionally, of a given subclass of KAction) in the action collection.
- Returns:
- A pointer to the first KAction in the collection which matches the parameters or null if nothing matches.
Definition at line 373 of file kactioncollection.cpp.
KAction * KActionCollection::action | ( | int | index | ) | const [virtual] |
Return the KAction* at position "index" in the action collection.
- See also:
- count()
Definition at line 400 of file kactioncollection.cpp.
void KActionCollection::actionHighlighted | ( | KAction * | action, | |
bool | highlight | |||
) | [signal] |
Emitted when action
is highlighed or loses highlighting.
This is only emitted if you have setHighlightingEnabled()
void KActionCollection::actionHighlighted | ( | KAction * | action | ) | [signal] |
Emitted when action
is highlighted.
This is only emitted if you have setHighlightingEnabled()
KActionPtrList KActionCollection::actions | ( | ) | const [virtual] |
Returns the list of actions managed by this action collection.
Definition at line 449 of file kactioncollection.cpp.
KActionPtrList KActionCollection::actions | ( | const QString & | group | ) | const [virtual] |
Returns the list of actions in a particular group managed by this action collection.
- Parameters:
-
group The name of the group.
Definition at line 435 of file kactioncollection.cpp.
void KActionCollection::actionStatusText | ( | const QString & | text | ) | [signal] |
Emitted when an action is highlighted, with text being the tooltip for the action.
This is only emitted if you have setHighlightingEnabled()
This is useful to connect to KStatusBar::message(). See this class overview for more information.
- See also:
- setHighlightingEnabled()
bool KActionCollection::addDocCollection | ( | KActionCollection * | pDoc | ) |
This sets the default shortcut scope for new actions created in this collection.
The default is ScopeUnspecified. Ideally the default would have been ScopeWidget, but that would cause some backwards compatibility problems. Doc/View model. This lets you add the action collection of a document to a view's action collection.
Definition at line 177 of file kactioncollection.cpp.
KAccel * KActionCollection::builderKAccel | ( | ) | const |
For internal use only.
, for KAction::kaccelCurrent()
Definition at line 371 of file kactioncollection.cpp.
void KActionCollection::clear | ( | ) | [slot] |
Clears the entire actionCollection, deleting all actions.
- See also:
- remove
Definition at line 368 of file kactioncollection.cpp.
void KActionCollection::clearStatusText | ( | ) | [signal] |
Emitted when an action loses highlighting.
This is only emitted if you have setHighlightingEnabled()
- See also:
- setHighlightingEnabled()
Call this function if you want to receive a signal whenever a KAction is highlighted in a menu or a toolbar.
This is only needed if you do not add this action to this container. You will generally not need to call this function.
- Parameters:
-
container A container in which the KAction is plugged (must inherit QPopupMenu or KToolBar) action The action you are interested in
- See also:
- disconnectHighlight()
Definition at line 493 of file kactioncollection.cpp.
uint KActionCollection::count | ( | ) | const [virtual] |
Returns the KAccel object associated with widget #.
Returns the number of actions in the collection
Definition at line 418 of file kactioncollection.cpp.
Disconnect highlight notifications for a particular pair of contianer and action.
This is only needed if you do not add this action to this container. You will generally not need to call this function.
- Parameters:
-
container A container in which the KAction is plugged (must inherit QPopupMenu or KToolBar) action The action you are interested in
- See also:
- connectHighlight()
Definition at line 526 of file kactioncollection.cpp.
QStringList KActionCollection::groups | ( | ) | const [virtual] |
Returns a list of all the groups of all the KActions in this action collection.
- See also:
- KAction::group()
Definition at line 423 of file kactioncollection.cpp.
bool KActionCollection::highlightingEnabled | ( | ) | const |
Return whether highlighting notifications are enabled.
- See also:
- connectHighlight()
Definition at line 488 of file kactioncollection.cpp.
void KActionCollection::insert | ( | KAction * | action | ) |
Add an action to the collection.
Generally you don't have to call this. The action inserts itself automatically into its parent collection. This can be useful however for a short-lived collection (e.g. for a popupmenu, where the signals from the collection are needed too). (don't forget that in the simple case, a list of actions should be a simple KActionPtrList). If you manually insert actions into a 2nd collection, don't forget to take them out again before destroying the collection.
- Parameters:
-
action The KAction to add.
Definition at line 365 of file kactioncollection.cpp.
void KActionCollection::inserted | ( | KAction * | ) | [signal] |
KInstance * KActionCollection::instance | ( | ) | const |
The instance with which this class is associated.
Definition at line 468 of file kactioncollection.cpp.
bool KActionCollection::isAutoConnectShortcuts | ( | ) |
This indicates whether new actions which are created in this collection have their keyboard shortcuts automatically connected on construction.
- See also:
- setAutoConnectShortcuts()
Definition at line 172 of file kactioncollection.cpp.
bool KActionCollection::isEmpty | ( | ) | const [inline] |
Definition at line 163 of file kactioncollection.h.
const KAccel * KActionCollection::kaccel | ( | ) | const |
Returns the KAccel object of the most recently set widget.
Const version for convenience.
Definition at line 291 of file kactioncollection.cpp.
KAccel * KActionCollection::kaccel | ( | ) |
Returns the KAccel object of the most recently set widget.
Definition at line 282 of file kactioncollection.cpp.
KActionCollection KActionCollection::operator+ | ( | const KActionCollection & | c | ) | const |
Definition at line 634 of file kactioncollection.cpp.
KActionCollection & KActionCollection::operator+= | ( | const KActionCollection & | c | ) |
Definition at line 662 of file kactioncollection.cpp.
KActionCollection & KActionCollection::operator= | ( | const KActionCollection & | copy | ) |
Definition at line 648 of file kactioncollection.cpp.
const KXMLGUIClient * KActionCollection::parentGUIClient | ( | ) | const |
The parent KXMLGUIClient, return 0L if not available.
Definition at line 627 of file kactioncollection.cpp.
bool KActionCollection::readShortcutSettings | ( | const QString & | sConfigGroup = QString::null , |
|
KConfigBase * | pConfig = 0 | |||
) |
Used for reading shortcut configuration from a non-XML rc file.
Definition at line 408 of file kactioncollection.cpp.
void KActionCollection::remove | ( | KAction * | action | ) |
Removes an action from the collection and deletes it.
Since the KAction destructor removes the action from the collection, you generally don't have to call this.
- Parameters:
-
action The KAction to remove.
Definition at line 366 of file kactioncollection.cpp.
void KActionCollection::removed | ( | KAction * | ) | [signal] |
void KActionCollection::setAutoConnectShortcuts | ( | bool | b | ) |
This indicates whether new actions which are created in this collection should have their keyboard shortcuts automatically connected on construction.
Set to 'false' if you will be loading XML-based settings. This is automatically done by KParts. The default is 'true'.
- See also:
- isAutoConnectShortcuts()
Definition at line 167 of file kactioncollection.cpp.
void KActionCollection::setHighlightingEnabled | ( | bool | enable | ) |
Enable highlighting notification for specific KActions.
This is false by default, so, by default, the highlighting signals will not be emitted.
- See also:
- connectHighlight()
Definition at line 483 of file kactioncollection.cpp.
void KActionCollection::setInstance | ( | KInstance * | instance | ) |
Definition at line 460 of file kactioncollection.cpp.
void KActionCollection::setWidget | ( | QWidget * | widget | ) | [virtual] |
This sets the widget to which the keyboard shortcuts should be attached.
You only need to call this if a null pointer was passed in the constructor.
Definition at line 152 of file kactioncollection.cpp.
void KActionCollection::setXMLFile | ( | const QString & | sXMLFile | ) |
Removes an action from the collection.
Since the KAction destructor removes the action from the collection, you generally don't have to call this.
- Returns:
- NULL if not found else returns action.
- Parameters:
-
action the KAction to remove.
Definition at line 367 of file kactioncollection.cpp.
void KActionCollection::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 796 of file kactioncollection.cpp.
bool KActionCollection::writeShortcutSettings | ( | const QString & | sConfigGroup = QString::null , |
|
KConfigBase * | pConfig = 0 | |||
) | const |
Used for writing shortcut configuration to a non-XML rc file.
Definition at line 413 of file kactioncollection.cpp.
const QString & KActionCollection::xmlFile | ( | ) | const |
The documentation for this class was generated from the following files: