kdeui
KCharSelect Class Reference
Character selection widget. More...
#include <kcharselect.h>
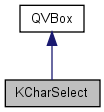
Signals | |
void | activated () |
void | activated (const QChar &c) |
void | doubleClicked () |
void | focusItemChanged (const QChar &c) |
void | focusItemChanged () |
void | fontChanged (const QString &_font) |
void | highlighted () |
void | highlighted (const QChar &c) |
Public Member Functions | |
virtual QChar | chr () const |
virtual void | enableFontCombo (bool e) |
virtual void | enableTableSpinBox (bool e) |
virtual QString | font () const |
virtual bool | isFontComboEnabled () const |
virtual bool | isTableSpinBoxEnabled () const |
KCharSelect (QWidget *parent, const char *name, const QString &font=QString::null, const QChar &chr= ' ', int tableNum=0) | |
virtual void | setChar (const QChar &chr) |
virtual void | setFont (const QString &font) |
virtual void | setTableNum (int tableNum) |
virtual QSize | sizeHint () const |
virtual int | tableNum () const |
~KCharSelect () | |
Protected Slots | |
void | charActivated () |
void | charActivated (const QChar &c) |
void | charFocusItemChanged (const QChar &c) |
void | charFocusItemChanged () |
void | charHighlighted () |
void | charHighlighted (const QChar &c) |
void | charTableDown () |
void | charTableUp () |
void | fontSelected (const QString &_font) |
void | slotDoubleClicked () |
void | slotUnicodeEntered () |
void | slotUpdateUnicode (const QChar &c) |
void | tableChanged (int _value) |
Protected Member Functions | |
virtual void | fillFontCombo () |
virtual void | virtual_hook (int id, void *data) |
Static Protected Member Functions | |
static void | cleanupFontDatabase () |
Protected Attributes | |
KCharSelectTable * | charTable |
QComboBox * | fontCombo |
QStringList | fontList |
QSpinBox * | tableSpinBox |
Static Protected Attributes | |
static QFontDatabase * | fontDataBase = 0 |
Properties | |
bool | fontComboEnabled |
QString | fontFamily |
int | tableNum |
bool | tableSpinBoxEnabled |
Detailed Description
Character selection widget.This widget allows the user to select a character of a specified font in a table
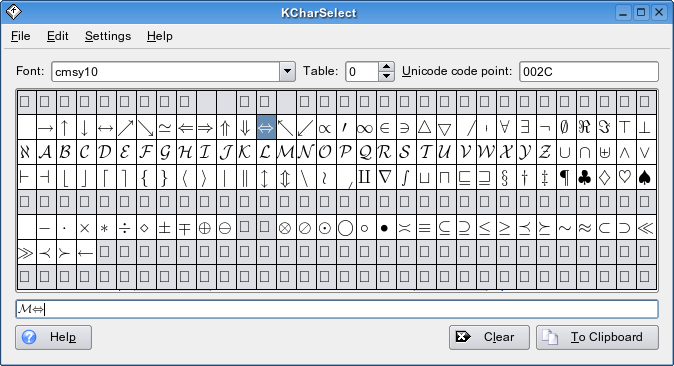
Character Selection Widget
KCharSelect supports keyboard and mouse navigation. Click+Move always selects the character below the mouse cursor. Using the arrow keys moves the focus mark around and pressing RETURN or SPACE selects the cell which contains the focus mark.
To get the current selected character, use the chr() method. You can set the character which should be displayed with setChar() and the table number which should be displayed with setTableNum().
Definition at line 140 of file kcharselect.h.
Constructor & Destructor Documentation
KCharSelect::KCharSelect | ( | QWidget * | parent, | |
const char * | name, | |||
const QString & | font = QString::null , |
|||
const QChar & | chr = ' ' , |
|||
int | tableNum = 0 | |||
) |
Constructor.
font
specifies which font should be displayed, chr
which character should be selected and tableNum
specifies the number of the table which should be displayed.
Definition at line 376 of file kcharselect.cpp.
KCharSelect::~KCharSelect | ( | ) |
Definition at line 449 of file kcharselect.cpp.
Member Function Documentation
void KCharSelect::activated | ( | ) | [signal] |
void KCharSelect::activated | ( | const QChar & | c | ) | [signal] |
void KCharSelect::charActivated | ( | ) | [inline, protected, slot] |
Definition at line 239 of file kcharselect.h.
void KCharSelect::charActivated | ( | const QChar & | c | ) | [inline, protected, slot] |
Definition at line 238 of file kcharselect.h.
void KCharSelect::charFocusItemChanged | ( | const QChar & | c | ) | [inline, protected, slot] |
Definition at line 241 of file kcharselect.h.
void KCharSelect::charFocusItemChanged | ( | ) | [inline, protected, slot] |
Definition at line 240 of file kcharselect.h.
void KCharSelect::charHighlighted | ( | ) | [inline, protected, slot] |
Definition at line 237 of file kcharselect.h.
void KCharSelect::charHighlighted | ( | const QChar & | c | ) | [inline, protected, slot] |
Definition at line 236 of file kcharselect.h.
void KCharSelect::charTableDown | ( | ) | [inline, protected, slot] |
Definition at line 243 of file kcharselect.h.
void KCharSelect::charTableUp | ( | ) | [inline, protected, slot] |
Definition at line 242 of file kcharselect.h.
virtual QChar KCharSelect::chr | ( | ) | const [inline, virtual] |
void KCharSelect::cleanupFontDatabase | ( | ) | [static, protected] |
Definition at line 53 of file kcharselect.cpp.
void KCharSelect::doubleClicked | ( | ) | [signal] |
virtual void KCharSelect::enableFontCombo | ( | bool | e | ) | [inline, virtual] |
If e
is set to true, the combobox which allows the user to select the font which should be displayed is enabled, else disabled.
Definition at line 197 of file kcharselect.h.
virtual void KCharSelect::enableTableSpinBox | ( | bool | e | ) | [inline, virtual] |
If e
is set to true, the spinbox which allows the user to specify which characters of the font should be displayed, is enabled, else disabled.
Definition at line 205 of file kcharselect.h.
void KCharSelect::fillFontCombo | ( | ) | [protected, virtual] |
Definition at line 490 of file kcharselect.cpp.
void KCharSelect::focusItemChanged | ( | const QChar & | c | ) | [signal] |
void KCharSelect::focusItemChanged | ( | ) | [signal] |
virtual QString KCharSelect::font | ( | ) | const [inline, virtual] |
void KCharSelect::fontChanged | ( | const QString & | _font | ) | [signal] |
void KCharSelect::fontSelected | ( | const QString & | _font | ) | [protected, slot] |
Definition at line 501 of file kcharselect.cpp.
void KCharSelect::highlighted | ( | ) | [signal] |
void KCharSelect::highlighted | ( | const QChar & | c | ) | [signal] |
virtual bool KCharSelect::isFontComboEnabled | ( | ) | const [inline, virtual] |
Returns wether the font combobox on the top is enabled or disabled.
- See also:
- enableFontCombo()
Definition at line 213 of file kcharselect.h.
virtual bool KCharSelect::isTableSpinBoxEnabled | ( | ) | const [inline, virtual] |
Returns wether the table spinbox on the top is enabled or disabled.
- See also:
- enableTableSpinBox()
Definition at line 221 of file kcharselect.h.
void KCharSelect::setChar | ( | const QChar & | chr | ) | [virtual] |
void KCharSelect::setFont | ( | const QString & | font | ) | [virtual] |
void KCharSelect::setTableNum | ( | int | tableNum | ) | [virtual] |
QSize KCharSelect::sizeHint | ( | void | ) | const [virtual] |
void KCharSelect::slotDoubleClicked | ( | ) | [inline, protected, slot] |
Definition at line 244 of file kcharselect.h.
void KCharSelect::slotUnicodeEntered | ( | ) | [protected, slot] |
Definition at line 514 of file kcharselect.cpp.
void KCharSelect::slotUpdateUnicode | ( | const QChar & | c | ) | [protected, slot] |
Definition at line 533 of file kcharselect.cpp.
void KCharSelect::tableChanged | ( | int | _value | ) | [protected, slot] |
Definition at line 508 of file kcharselect.cpp.
virtual int KCharSelect::tableNum | ( | ) | const [inline, virtual] |
void KCharSelect::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 544 of file kcharselect.cpp.
Member Data Documentation
KCharSelectTable* KCharSelect::charTable [protected] |
Definition at line 229 of file kcharselect.h.
QComboBox* KCharSelect::fontCombo [protected] |
Definition at line 227 of file kcharselect.h.
QFontDatabase * KCharSelect::fontDataBase = 0 [static, protected] |
Definition at line 231 of file kcharselect.h.
QStringList KCharSelect::fontList [protected] |
Definition at line 230 of file kcharselect.h.
QSpinBox* KCharSelect::tableSpinBox [protected] |
Definition at line 228 of file kcharselect.h.
Property Documentation
bool KCharSelect::fontComboEnabled [read, write] |
Definition at line 145 of file kcharselect.h.
QString KCharSelect::fontFamily [read, write] |
Definition at line 143 of file kcharselect.h.
int KCharSelect::tableNum [read, write] |
Definition at line 144 of file kcharselect.h.
bool KCharSelect::tableSpinBoxEnabled [read, write] |
Definition at line 146 of file kcharselect.h.
The documentation for this class was generated from the following files: