kdeui
KEditListBox Class Reference
An editable listbox. More...
#include <keditlistbox.h>
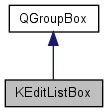
Classes | |
class | CustomEditor |
Custom editor class. More... | |
Public Types | |
enum | { All = Add|Remove|UpDown } |
enum | Button { Add = 1, Remove = 2, UpDown = 4 } |
Signals | |
void | added (const QString &text) |
void | changed () |
void | removed (const QString &text) |
Public Member Functions | |
QPushButton * | addButton () const |
int | buttons () const |
void | clear () |
int | count () const |
int | currentItem () const |
QString | currentText () const |
QPushButton * | downButton () const |
void | insertItem (const QString &text, int index=-1) |
void | insertStringList (const QStringList &list, int index=-1) |
void | insertStrList (const char **list, int numStrings=-1, int index=-1) |
void | insertStrList (const QStrList &list, int index=-1) |
void | insertStrList (const QStrList *list, int index=-1) |
QStringList | items () const |
KEditListBox (const QString &title, const CustomEditor &customEditor, QWidget *parent=0, const char *name=0, bool checkAtEntering=false, int buttons=All) | |
KEditListBox (const QString &title, QWidget *parent=0, const char *name=0, bool checkAtEntering=false, int buttons=All) | |
KEditListBox (QWidget *parent=0, const char *name=0, bool checkAtEntering=false, int buttons=All) | |
KLineEdit * | lineEdit () const |
QListBox * | listBox () const |
QPushButton * | removeButton () const |
void | setButtons (uint buttons) |
void | setItems (const QStringList &items) |
QString | text (int index) const |
QPushButton * | upButton () const |
virtual | ~KEditListBox () |
Protected Slots | |
void | addItem () |
void | enableMoveButtons (int index) |
void | moveItemDown () |
void | moveItemUp () |
void | removeItem () |
void | typedSomething (const QString &text) |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Properties | |
Button | buttons |
QStringList | items |
Detailed Description
An editable listbox.This class provides a editable listbox ;-), this means a listbox which is accompanied by a line edit to enter new items into the listbox and pushbuttons to add and remove items from the listbox and two buttons to move items up and down.
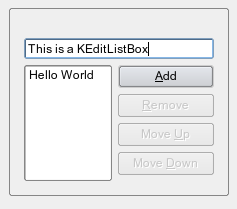
KDE Edit List Box Widget
Definition at line 44 of file keditlistbox.h.
Member Enumeration Documentation
anonymous enum |
Enumeration of the buttons, the listbox offers.
Specify them in the constructor in the buttons parameter, or in setButtons.
Definition at line 95 of file keditlistbox.h.
Constructor & Destructor Documentation
KEditListBox::KEditListBox | ( | QWidget * | parent = 0 , |
|
const char * | name = 0 , |
|||
bool | checkAtEntering = false , |
|||
int | buttons = All | |||
) |
Create an editable listbox.
If checkAtEntering
is true, after every character you type in the line edit KEditListBox will enable or disable the Add-button, depending whether the current content of the line edit is already in the listbox. Maybe this can become a performance hit with large lists on slow machines. If checkAtEntering
is false, it will be checked if you press the Add-button. It is not possible to enter items twice into the listbox.
Definition at line 48 of file keditlistbox.cpp.
KEditListBox::KEditListBox | ( | const QString & | title, | |
QWidget * | parent = 0 , |
|||
const char * | name = 0 , |
|||
bool | checkAtEntering = false , |
|||
int | buttons = All | |||
) |
Create an editable listbox.
The same as the other constructor, additionally it takes title
, which will be the title of the frame around the listbox.
Definition at line 55 of file keditlistbox.cpp.
KEditListBox::KEditListBox | ( | const QString & | title, | |
const CustomEditor & | customEditor, | |||
QWidget * | parent = 0 , |
|||
const char * | name = 0 , |
|||
bool | checkAtEntering = false , |
|||
int | buttons = All | |||
) |
Another constructor, which allows to use a custom editing widget instead of the standard KLineEdit widget.
E.g. you can use a KURLRequester or a KComboBox as input widget. The custom editor must consist of a lineedit and optionally another widget that is used as representation. A KComboBox or a KURLRequester have a KLineEdit as child-widget for example, so the KComboBox is used as the representation widget.
- See also:
- KURLRequester::customEditor()
- Since:
- 3.1
Definition at line 62 of file keditlistbox.cpp.
KEditListBox::~KEditListBox | ( | ) | [virtual] |
Definition at line 71 of file keditlistbox.cpp.
Member Function Documentation
QPushButton* KEditListBox::addButton | ( | ) | const [inline] |
void KEditListBox::added | ( | const QString & | text | ) | [signal] |
This signal is emitted when the user adds a new string to the list, the parameter is the added string.
- Since:
- 3.2
void KEditListBox::addItem | ( | ) | [protected, slot] |
Definition at line 250 of file keditlistbox.cpp.
int KEditListBox::buttons | ( | ) | const |
Returns which buttons are visible.
void KEditListBox::changed | ( | ) | [signal] |
void KEditListBox::clear | ( | ) |
int KEditListBox::count | ( | ) | const [inline] |
int KEditListBox::currentItem | ( | ) | const |
QString KEditListBox::currentText | ( | ) | const [inline] |
QPushButton* KEditListBox::downButton | ( | ) | const [inline] |
void KEditListBox::enableMoveButtons | ( | int | index | ) | [protected, slot] |
Definition at line 321 of file keditlistbox.cpp.
void KEditListBox::insertItem | ( | const QString & | text, | |
int | index = -1 | |||
) | [inline] |
void KEditListBox::insertStringList | ( | const QStringList & | list, | |
int | index = -1 | |||
) |
void KEditListBox::insertStrList | ( | const char ** | list, | |
int | numStrings = -1 , |
|||
int | index = -1 | |||
) |
void KEditListBox::insertStrList | ( | const QStrList & | list, | |
int | index = -1 | |||
) |
void KEditListBox::insertStrList | ( | const QStrList * | list, | |
int | index = -1 | |||
) |
QStringList KEditListBox::items | ( | ) | const |
- Returns:
- a stringlist of all items in the listbox
KLineEdit* KEditListBox::lineEdit | ( | ) | const [inline] |
QListBox* KEditListBox::listBox | ( | ) | const [inline] |
void KEditListBox::moveItemDown | ( | ) | [protected, slot] |
Definition at line 227 of file keditlistbox.cpp.
void KEditListBox::moveItemUp | ( | ) | [protected, slot] |
Definition at line 204 of file keditlistbox.cpp.
QPushButton* KEditListBox::removeButton | ( | ) | const [inline] |
void KEditListBox::removed | ( | const QString & | text | ) | [signal] |
This signal is emitted when the user removes a string from the list, the parameter is the removed string.
- Since:
- 3.2
void KEditListBox::removeItem | ( | ) | [protected, slot] |
Definition at line 301 of file keditlistbox.cpp.
void KEditListBox::setButtons | ( | uint | buttons | ) |
void KEditListBox::setItems | ( | const QStringList & | items | ) |
Clears the listbox and sets the contents to items
.
- Since:
- 3.4
Definition at line 393 of file keditlistbox.cpp.
QString KEditListBox::text | ( | int | index | ) | const [inline] |
void KEditListBox::typedSomething | ( | const QString & | text | ) | [protected, slot] |
Definition at line 168 of file keditlistbox.cpp.
QPushButton* KEditListBox::upButton | ( | ) | const [inline] |
void KEditListBox::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 404 of file keditlistbox.cpp.
Property Documentation
int KEditListBox::buttons [read, write] |
Definition at line 49 of file keditlistbox.h.
QStringList KEditListBox::items [read, write] |
Definition at line 50 of file keditlistbox.h.
The documentation for this class was generated from the following files: