kdeui
KPanelExtension Class Reference
KDE Panel Extension class More...
#include <kpanelextension.h>
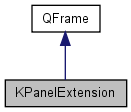
Public Types | |
enum | Action { About = 1, Help = 2, Preferences = 4, ReportBug = 8 } |
enum | Alignment { LeftTop = 0, Center, RightBottom } |
enum | Position { Left = 0, Right, Top, Bottom, Floating } |
enum | Size { SizeTiny = 0, SizeSmall, SizeNormal, SizeLarge, SizeCustom } |
enum | Type { Normal = 0, Stretch } |
Signals | |
void | maintainFocus (bool) |
void | updateLayout () |
Public Member Functions | |
virtual void | action (Action a) |
int | actions () const |
KConfig * | config () const |
QPopupMenu * | customMenu () const |
int | customSize () const |
KPanelExtension (const QString &configFile, Type t=Normal, int actions=0, QWidget *parent=0, const char *name=0) | |
virtual Position | preferedPosition () const |
bool | reserveStrut () const |
void | setAlignment (Alignment a) |
void | setPosition (Position p) |
void | setSize (Size size, int customSize) |
virtual QSize | sizeHint (Position, QSize maxsize) const |
Size | sizeSetting () const |
Type | type () const |
~KPanelExtension () | |
Protected Member Functions | |
virtual void | about () |
Alignment | alignment () const |
virtual void | alignmentChange (Alignment) |
virtual void | help () |
Orientation | orientation () |
Position | position () const |
virtual void | positionChange (Position) |
virtual void | preferences () |
virtual void | reportBug () |
void | setCustomMenu (QPopupMenu *) |
void | setReserveStrut (bool shouldUseStrut) |
int | sizeInPixels () const |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
KDE Panel Extension classPanel extensions
- Are small applications living in the Window Manager dock managed by the panel.
- Are implemented as DSOs (Dynamic Shared Objects).
The panel locates available extensions by searching for extension desktop files in (ALL_KDEDIRS)/share/apps/kicker/extensions. Every panel extension should install a desktop file there to be recognized by the panel.
Besides standard keys like "Name", "Comment" and "Icon" there are two panel extension specific keys:
X-KDE-Library
Used by the panel to locate the extension DSO (Dynamic Shared Object) Example: X-KDE-Library=libexampleextension
X-KDE-UniqueExtension
Similar to KApplication and KUniqueApplication there are two types of panel extensions. Use unique extensions when it makes no sence to run more than one instance of an extension in the panel. A good example for unique extensions is the taskbar extension. Use normal extensions when you need instance specific configuration. An example is a subpanel extension where you might want to run more than one instances. X-KDE-UniqueExtension is a boolean key which defaults to "false". Example: X-KDE-UniqueExtension=true
Back to panel extension DSOs, the following conventions are used for KDE: Name: lib<extensionname>extension.la LDFLAGS: -module -no-undefined
To implement a panel extension it is not enough to write a class inheriting from KPanelExtension but you also have to provide a factory function in your DSO. A sample factory function could look like this:
extern "C" { KPanelExtension* init(QWidget *parent, const QString& configFile) { KGlobal::locale()->insertCatalogue("exampleextension"); return new ExampleExtension(configFile, KPanelExtension::Normal, KPanelExtension::About | KPanelExtension::Help | KPanelExtension::Preferences, parent, "exampleextension"); } }
Note: Don't change the factory function signature or the panel will fail to load your extension.
Definition at line 98 of file kpanelextension.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
KPanelExtension::KPanelExtension | ( | const QString & | configFile, | |
Type | t = Normal , |
|||
int | actions = 0 , |
|||
QWidget * | parent = 0 , |
|||
const char * | name = 0 | |||
) |
Constructs a KPanelExtension just like any other widget.
- Parameters:
-
configFile The configFile handed over in the factory function. t The extension type(). actions Standard RMB menu actions supported by the extension (see action() ). parent The pointer to the parent widget handed over in the factory function. name A Qt object name for your extension.
Definition at line 47 of file kpanelextension.cpp.
KPanelExtension::~KPanelExtension | ( | ) |
Member Function Documentation
virtual void KPanelExtension::about | ( | ) | [inline, protected, virtual] |
Is called when the user selects "About" from the extensions RMB menu.
Reimplement this function to launch a about dialog.
Note that this is called only when your extension supports the About action. See Action.
Definition at line 250 of file kpanelextension.h.
void KPanelExtension::action | ( | Action | a | ) | [virtual] |
Generic action dispatcher.
Called when the user selects an item from the extensions RMB menu.
Reimplement this function to handle actions.
For About, Help, Preferences and ReportBug use the convenience handlers ref about(), help(), preferences(), reportBug()
Definition at line 89 of file kpanelextension.cpp.
int KPanelExtension::actions | ( | ) | const [inline] |
- Returns:
- int indicating the supported RMB menu actions. Action
Definition at line 163 of file kpanelextension.h.
Alignment KPanelExtension::alignment | ( | ) | const [inline, protected] |
- Returns:
- the extension's alignment. (left/top, center, or right/bottom)
Definition at line 288 of file kpanelextension.h.
virtual void KPanelExtension::alignmentChange | ( | Alignment | ) | [inline, protected, virtual] |
This extension has changed its alignment.
Reimplement this change handler in order to adjust the look of your applet.
Definition at line 313 of file kpanelextension.h.
KConfig* KPanelExtension::config | ( | ) | const [inline] |
Always use this KConfig object to save/load your extensions configuration.
For unique extensions this config object will write to a config file called <extensionname>rc in the users local KDE directory.
For normal extensions this config object will write to a instance specific config file called <extensionname><instanceid>rc in the users local KDE directory.
Definition at line 151 of file kpanelextension.h.
QPopupMenu * KPanelExtension::customMenu | ( | ) | const |
- Returns:
- the extension's custom menu, usually the same as the context menu, or 0 if none
- See also:
- setCustomMenu(QPopupMenu*)
- Since:
- 3.4
Definition at line 141 of file kpanelextension.cpp.
int KPanelExtension::customSize | ( | ) | const |
- Returns:
- the custom sizel setting in pixels
- Since:
- 3.1
Definition at line 114 of file kpanelextension.cpp.
virtual void KPanelExtension::help | ( | ) | [inline, protected, virtual] |
Is called when the user selects "Help" from the extensions RMB menu.
Reimplement this function to launch a manual or help page.
Note that this is called only when your extension supports the Help action. See Action.
Definition at line 259 of file kpanelextension.h.
void KPanelExtension::maintainFocus | ( | bool | ) | [signal] |
Emit this signal to make the panel maintain focus, e.g.
don't autohide
- Since:
- 3.4
Qt::Orientation KPanelExtension::orientation | ( | ) | [protected] |
- Returns:
- the extensions orientation. (horizontal or vertical)
Definition at line 101 of file kpanelextension.cpp.
Position KPanelExtension::position | ( | ) | const [inline, protected] |
- Returns:
- the extension's position. (left, right, top, bottom)
Definition at line 283 of file kpanelextension.h.
virtual void KPanelExtension::positionChange | ( | Position | ) | [inline, protected, virtual] |
This extension has changed its position.
Reimplement this change handler in order to adjust the look of your applet.
Definition at line 306 of file kpanelextension.h.
virtual Position KPanelExtension::preferedPosition | ( | ) | const [inline, virtual] |
Reimplement this function to set a preferred dock position for your extension.
The extension manager will try to place new instances of this extension according to this setting.
- Returns:
- Position
Definition at line 184 of file kpanelextension.h.
virtual void KPanelExtension::preferences | ( | ) | [inline, protected, virtual] |
Is called when the user selects "Preferences" from the extensions RMB menu.
Reimplement this function to launch a preferences dialog or kcontrol module.
Note that this is called only when your extension supports the preferences action. See Action.
Definition at line 268 of file kpanelextension.h.
virtual void KPanelExtension::reportBug | ( | ) | [inline, protected, virtual] |
Is called when the user selects "Report bug" from the applet's RMB menu.
Reimplement this function to launch a bug reporting dialog.
Note that this is called only when your applet supports the ReportBug action. See Action.
Definition at line 278 of file kpanelextension.h.
bool KPanelExtension::reserveStrut | ( | ) | const |
- Returns:
- whether or not to set a desktop geometry claiming strut for this panel defaults to true
- See also:
- setReservetrut(bool)
- Since:
- 3.4
Definition at line 151 of file kpanelextension.cpp.
void KPanelExtension::setAlignment | ( | Alignment | a | ) |
void KPanelExtension::setCustomMenu | ( | QPopupMenu * | menu | ) | [protected] |
Use this method to set the custom menu for this extensions so that it can be shown at the appropriate places/times that the extension many not itself be aware of.
The extension itself is still responsible for deleting and managing the the menu.
If the menu is deleted during the life of the extension, be sure to call this method again with the new menu (or 0) to avoid crashes
- Since:
- 3.4
Definition at line 146 of file kpanelextension.cpp.
void KPanelExtension::setPosition | ( | Position | p | ) |
void KPanelExtension::setReserveStrut | ( | bool | shouldUseStrut | ) | [protected] |
Use this method to set the return value for reserveStrut.
- See also:
- reserveStrut
- Since:
- 3.4
Definition at line 156 of file kpanelextension.cpp.
void KPanelExtension::setSize | ( | Size | size, | |
int | customSize | |||
) |
Returns the preferred size for a given Position.
Every extension should reimplement this function.
Depending on the panel position the extensions can choose a preferred size for that location in the Window Manager Dock. Please note that the size can not be larger than the maxsize given by the handler.
Definition at line 140 of file kpanelextension.h.
int KPanelExtension::sizeInPixels | ( | ) | const [protected] |
- Returns:
- the appropriate size in pixels for the panel
- Since:
- 3.1
Definition at line 119 of file kpanelextension.cpp.
KPanelExtension::Size KPanelExtension::sizeSetting | ( | ) | const |
Type KPanelExtension::type | ( | ) | const [inline] |
void KPanelExtension::updateLayout | ( | ) | [signal] |
Emit this signal to make the panel relayout all extensions in the dock, when you want to change the extensions size.
The panel is going to relayout all extensions based on their preferred size.
void KPanelExtension::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 161 of file kpanelextension.cpp.
The documentation for this class was generated from the following files: