kdeui
KSpell Class Reference
KDE Spellchecker More...
#include <kspell.h>
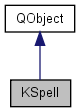
Public Types | |
enum | SpellerType { Text = 0, HTML, TeX, Nroff } |
enum | spellStatus { Starting = 0, Running, Cleaning, Finished, Error, Crashed, FinishedNoMisspellingsEncountered } |
Signals | |
void | addword (const QString &originalword) |
void | corrected (const QString &originalword, const QString &newword, unsigned int pos) |
void | death () |
void | dialog3 () |
void | done (bool) |
void | done (const QString &buffer) |
void | ignoreall (const QString &originalword) |
void | ignoreword (const QString &originalword) |
void | misspelling (const QString &originalword, const QStringList &suggestions, unsigned int pos) |
void | progress (unsigned int i) |
void | ready (KSpell *) |
void | replaceall (const QString &origword, const QString &replacement) |
Public Member Functions | |
virtual bool | addPersonal (const QString &word) |
virtual bool | check (const QString &_buffer, bool usedialog=true) |
virtual bool | checkList (QStringList *_wordlist, bool usedialog=true) |
bool | checkWord (const QString &buffer, bool _usedialog, bool suggest) |
virtual bool | checkWord (const QString &_buffer, bool usedialog=false) |
virtual void | cleanUp () |
int | dlgResult () const |
int | heightDlg () const |
void | hide () |
virtual bool | ignore (const QString &word) |
QString | intermediateBuffer () const |
KSpellConfig | ksConfig () const |
KSpell (QWidget *parent, const QString &caption, QObject *receiver, const char *slot, KSpellConfig *kcs, bool progressbar, bool modal, SpellerType type) | |
KSpell (QWidget *parent, const QString &caption, QObject *receiver, const char *slot, KSpellConfig *kcs=0, bool progressbar=true, bool modal=false) | |
int | lastPosition () const |
void | moveDlg (int x, int y) |
void | setAutoDelete (bool _autoDelete) |
void | setIgnoreTitleCase (bool b) |
void | setIgnoreUpperWords (bool b) |
void | setProgressResolution (unsigned int res) |
spellStatus | status () const |
QStringList | suggestions () const |
int | widthDlg () const |
virtual | ~KSpell () |
Static Public Member Functions | |
static int | modalCheck (QString &text, KSpellConfig *kcs) |
static int | modalCheck (QString &text) KDE_DEPRECATED |
Protected Slots | |
void | check2 (KProcIO *) |
void | check3 () |
void | checkList2 () |
void | checkList3a (KProcIO *) |
void | checkList4 () |
void | checkListReplaceCurrent () |
void | checkNext () |
void | checkWord2 (KProcIO *) |
void | checkWord3 () |
void | dialog2 (int dlgresult) |
void | emitDeath () |
void | ispellErrors (KProcess *, char *, int) |
void | ispellExit (KProcess *) |
void | KSpell2 (KProcIO *) |
void | slotStopCancel (int) |
void | suggestWord (KProcIO *) |
Protected Member Functions | |
bool | cleanFputs (const QString &s, bool appendCR=true) |
bool | cleanFputsWord (const QString &s, bool appendCR=true) |
void | dialog (const QString &word, QStringList &sugg, const char *_slot) |
void | emitProgress () |
QString | funnyWord (const QString &word) |
void | initialize (QWidget *_parent, const QString &_caption, QObject *obj, const char *slot, KSpellConfig *_ksc, bool _progressbar, bool _modal, SpellerType type) |
int | parseOneResponse (const QString &_buffer, QString &word, QStringList &sugg) |
QString | replacement () const |
void | setUpDialog (bool reallyusedialogbox=true) |
void | startIspell () |
bool | writePersonalDictionary () |
Protected Attributes | |
bool | autoDelete |
QString | caption |
QTextCodec * | codec |
unsigned int | curprog |
QString | cwword |
QString | dialog3slot |
bool | dialogsetup |
bool | dialogwillprocess |
bool | dlgon |
QString | dlgorigword |
QString | dlgreplacement |
int | dlgresult |
QStringList | ignorelist |
KSpellConfig * | ksconfig |
KSpellDlg * | ksdlg |
unsigned int | lastlastline |
unsigned int | lastline |
int | lastpos |
spellStatus | m_status |
int | maxtrystart |
bool | modaldlg |
QString | newbuffer |
unsigned int | offset |
QString | orig |
QString | origbuffer |
QWidget * | parent |
bool | personaldict |
unsigned int | posinline |
KProcIO * | proc |
unsigned int | progres |
bool | progressbar |
QStringList | replacelist |
QStringList | sugg |
bool | texmode |
unsigned int | totalpos |
int | trystart |
bool | usedialog |
QStringList::Iterator | wlIt |
QStringList * | wordlist |
Static Protected Attributes | |
static int | modalreturn = 0 |
static QString | modaltext |
static QWidget * | modalWidgetHack = 0 |
Detailed Description
KDE SpellcheckerA KDE programmer's interface to International ISpell 3.1, ASpell, HSpell and ZPSpell.. A static method, modalCheck() is provided for convenient access to the spellchecker.
- See also:
- KSpellConfig, KSyntaxHighlighter
Definition at line 46 of file kspell.h.
Member Enumeration Documentation
enum KSpell::SpellerType |
These are possible types of documents which the spell checker can check.
Text
- The default type, checks every wordHTML
- For HTML/SGML/XML documents, will skip the tags,TeX
- For TeX/LaTeX documents, will skip commands,Nroff
- For nroff/troff documents.
enum KSpell::spellStatus |
Possible states of the spell checker.
Starting
- After creation of KSpell.Running
- After the ready signal has been emitted.Cleaning
- After cleanUp() has been called.Finished
- After cleanUp() has been completed.
Error
- An error occurred in theStarting
state.Crashed
- An error occurred in theRunning
state.
Constructor & Destructor Documentation
KSpell::KSpell | ( | QWidget * | parent, | |
const QString & | caption, | |||
QObject * | receiver, | |||
const char * | slot, | |||
KSpellConfig * | kcs = 0 , |
|||
bool | progressbar = true , |
|||
bool | modal = false | |||
) |
Starts the spellchecker.
KSpell emits ready() when it has verified that ISpell/ASpell is working properly. Pass the name of a slot -- do not pass zero! Be sure to call cleanUp() when you are done with KSpell.
If KSpell could not be started correctly, death() is emitted.
- Parameters:
-
parent Parent of KSpellConfig dialog.. caption Caption of KSpellConfig dialog. receiver Receiver object for the ready(KSpell *) signal. slot Receiver's slot, will be connected to the ready(KSpell *) signal. kcs Configuration for KSpell. progressbar Indicates if progress bar should be shown. modal Indicates modal or non-modal dialog.
Definition at line 107 of file kspell.cpp.
KSpell::KSpell | ( | QWidget * | parent, | |
const QString & | caption, | |||
QObject * | receiver, | |||
const char * | slot, | |||
KSpellConfig * | kcs, | |||
bool | progressbar, | |||
bool | modal, | |||
SpellerType | type | |||
) |
Starts the spellchecker.
KSpell emits ready() when it has verified that ISpell/ASpell is working properly. Pass the name of a slot -- do not pass zero! Be sure to call cleanUp() when you are done with KSpell.
If KSpell could not be started correctly, death() is emitted.
- Parameters:
-
parent Parent of KSpellConfig dialog.. caption Caption of KSpellConfig dialog. receiver Receiver object for the ready(KSpell *) signal. slot Receiver's slot, will be connected to the ready(KSpell *) signal. kcs Configuration for KSpell. progressbar Indicates if progress bar should be shown. modal Indicates modal or non-modal dialog. type Type of the document to check
Definition at line 115 of file kspell.cpp.
KSpell::~KSpell | ( | ) | [virtual] |
The destructor instructs ISpell/ASpell to write out the personal dictionary and then terminates ISpell/ASpell.
Definition at line 1285 of file kspell.cpp.
Member Function Documentation
bool KSpell::addPersonal | ( | const QString & | word | ) | [virtual] |
Adds a word to the user's personal dictionary.
- Returns:
- false if
word
is not a word or there was an error communicating with ISpell/ASpell.
Definition at line 391 of file kspell.cpp.
void KSpell::addword | ( | const QString & | originalword | ) | [signal] |
Emitted when the user pressed "Add" in the dialog.
This could be used to make an external user dictionary independent of the ISpell personal dictionary.
bool KSpell::check | ( | const QString & | _buffer, | |
bool | usedialog = true | |||
) | [virtual] |
Spellchecks a buffer of many words in plain text format.
The _buffer
is not modified. The signal done() will be sent when check() is finished and the argument will be a spell-corrected version of _buffer
.
The spell check may be stopped by the user before the entire buffer has been checked. You can check lastPosition() to see how far in _buffer
check() reached before stopping.
Definition at line 976 of file kspell.cpp.
void KSpell::check2 | ( | KProcIO * | ) | [protected, slot] |
Definition at line 1036 of file kspell.cpp.
void KSpell::check3 | ( | ) | [protected, slot] |
Definition at line 1152 of file kspell.cpp.
bool KSpell::checkList | ( | QStringList * | _wordlist, | |
bool | usedialog = true | |||
) | [virtual] |
Spellchecks a list of words.
checkList() is more flexible than check(). You could parse any type of document (HTML, TeX, etc.) into a list of spell-checkable words and send the list to checkList(). Sending a marked-up document to check() would result in the mark-up tags being spell checked.
Definition at line 782 of file kspell.cpp.
void KSpell::checkList2 | ( | ) | [protected, slot] |
Definition at line 809 of file kspell.cpp.
void KSpell::checkList3a | ( | KProcIO * | ) | [protected, slot] |
Definition at line 840 of file kspell.cpp.
void KSpell::checkList4 | ( | ) | [protected, slot] |
Definition at line 927 of file kspell.cpp.
void KSpell::checkListReplaceCurrent | ( | ) | [protected, slot] |
Definition at line 912 of file kspell.cpp.
void KSpell::checkNext | ( | ) | [protected, slot] |
Definition at line 596 of file kspell.cpp.
bool KSpell::checkWord | ( | const QString & | buffer, | |
bool | _usedialog, | |||
bool | suggest | |||
) |
bool KSpell::checkWord | ( | const QString & | _buffer, | |
bool | usedialog = false | |||
) | [virtual] |
Spellchecks a single word.
checkWord() is the most flexible function. Some applications might need this flexibility but will sacrifice speed when checking large numbers of words. Consider checkList() for checking many words.
Use this method for implementing "online" spellchecking (i.e., spellcheck as-you-type).
checkWord() returns false
if buffer
is not a single word (e.g. if it contains white space), otherwise it returns true
;
If usedialog
is set to true
, KSpell will open the standard dialog if the word is not found. The dialog results can be queried by using dlgResult() and replacement().
The signal corrected() is emitted when the check is complete. You can look at suggestions() to see what the suggested replacements were.
set the dialog signal handler
Definition at line 473 of file kspell.cpp.
void KSpell::checkWord2 | ( | KProcIO * | ) | [protected, slot] |
Definition at line 558 of file kspell.cpp.
void KSpell::checkWord3 | ( | ) | [protected, slot] |
Definition at line 634 of file kspell.cpp.
bool KSpell::cleanFputs | ( | const QString & | s, | |
bool | appendCR = true | |||
) | [protected] |
Definition at line 451 of file kspell.cpp.
bool KSpell::cleanFputsWord | ( | const QString & | s, | |
bool | appendCR = true | |||
) | [protected] |
Definition at line 424 of file kspell.cpp.
void KSpell::cleanUp | ( | ) | [virtual] |
Cleans up ISpell.
Write out the personal dictionary and close ISpell's stdin. A death() signal will be emitted when the cleanup is complete, but this method will return immediately.
Definition at line 1302 of file kspell.cpp.
void KSpell::corrected | ( | const QString & | originalword, | |
const QString & | newword, | |||
unsigned int | pos | |||
) | [signal] |
Emitted after the "Replace" or "Replace All" buttons of the dialog was pressed, or if the word was corrected without calling the dialog (i.e., the user previously chose "Replace All" for this word).
Results from the dialog may be checked with dlgResult() and replacement().
Note, that when using checkList() this signal can occur more then once with same list position, when checking a word with hyphens. In this case originalword
is the last replacement.
- See also:
- check()
void KSpell::death | ( | ) | [signal] |
void KSpell::dialog | ( | const QString & | word, | |
QStringList & | sugg, | |||
const char * | _slot | |||
) | [protected] |
Definition at line 1208 of file kspell.cpp.
void KSpell::dialog2 | ( | int | dlgresult | ) | [protected, slot] |
Definition at line 1237 of file kspell.cpp.
void KSpell::dialog3 | ( | ) | [signal] |
int KSpell::dlgResult | ( | ) | const [inline] |
Gets the result code of the dialog box.
After calling checkWord, you can use this to get the dialog box's result code. The possible values are (from kspelldlg.h):
- KS_CANCEL
- KS_REPLACE
- KS_REPLACEALL
- KS_IGNORE
- KS_IGNOREALL
- KS_ADD
- KS_STOP
void KSpell::done | ( | bool | ) | [signal] |
Emitted when checkList() is done.
If the argument is true
, then you should update your text from the wordlist, otherwise not.
void KSpell::done | ( | const QString & | buffer | ) | [signal] |
void KSpell::emitDeath | ( | ) | [protected, slot] |
Definition at line 1343 of file kspell.cpp.
void KSpell::emitProgress | ( | ) | [protected] |
Definition at line 1356 of file kspell.cpp.
Definition at line 641 of file kspell.cpp.
int KSpell::heightDlg | ( | ) | const |
void KSpell::hide | ( | ) |
Hides the dialog box.
You'll need to do this when you are done with checkWord();
Definition at line 123 of file kspell.cpp.
bool KSpell::ignore | ( | const QString & | word | ) | [virtual] |
Tells ISpell/ASpell to ignore this word for the life of this KSpell instance.
- Returns:
- false if
word
is not a word or there was an error communicating with ISpell/ASpell.
Definition at line 410 of file kspell.cpp.
void KSpell::ignoreall | ( | const QString & | originalword | ) | [signal] |
Emitted when the user pressed "Ignore All" in the dialog.
This could be used to make an application or file specific user dictionary.
void KSpell::ignoreword | ( | const QString & | originalword | ) | [signal] |
Emitted when the user pressed "Ignore" in the dialog.
Don't know if this could be useful.
void KSpell::initialize | ( | QWidget * | _parent, | |
const QString & | _caption, | |||
QObject * | obj, | |||
const char * | slot, | |||
KSpellConfig * | _ksc, | |||
bool | _progressbar, | |||
bool | _modal, | |||
SpellerType | type | |||
) | [protected] |
Definition at line 1453 of file kspell.cpp.
QString KSpell::intermediateBuffer | ( | ) | const [inline] |
void KSpell::ispellErrors | ( | KProcess * | , | |
char * | buffer, | |||
int | buflen | |||
) | [protected, slot] |
Definition at line 316 of file kspell.cpp.
void KSpell::ispellExit | ( | KProcess * | ) | [protected, slot] |
Definition at line 1316 of file kspell.cpp.
KSpellConfig KSpell::ksConfig | ( | ) | const |
- Returns:
- the KSpellConfig object being used by this KSpell instance.
Definition at line 1295 of file kspell.cpp.
void KSpell::KSpell2 | ( | KProcIO * | ) | [protected, slot] |
Definition at line 322 of file kspell.cpp.
int KSpell::lastPosition | ( | ) | const [inline] |
Returns the position (when using check()) or word number (when using checkList()) of the last word checked.
void KSpell::misspelling | ( | const QString & | originalword, | |
const QStringList & | suggestions, | |||
unsigned int | pos | |||
) | [signal] |
Emitted whenever a misspelled word is found by check() or by checkWord().
If it is emitted by checkWord(), pos=0
. If it is emitted by check(), then pos
indicates the position of the misspelled word in the (original) _buffer
. (The first position is zero.) If it is emitted by checkList(), pos
is the index to the misspelled word in the QStringList passed to checkList(). Note, that originalword
can be only a word part, if it's word with hyphens.
These are called _before_ the dialog is opened, so that the calling program's GUI may be updated. (e.g. the misspelled word may be highlighted).
int KSpell::modalCheck | ( | QString & | text, | |
KSpellConfig * | kcs | |||
) | [static] |
Performs a synchronous spellcheck.
This method does not return until spellchecking is done or canceled. Your application's GUI will still be updated, however.
This overloaded method uses the spell-check configuration passed as parameter.
Definition at line 1397 of file kspell.cpp.
int KSpell::modalCheck | ( | QString & | text | ) | [static] |
- Deprecated:
- Performs a synchronous spellcheck.
Definition at line 1391 of file kspell.cpp.
void KSpell::moveDlg | ( | int | x, | |
int | y | |||
) |
Moves the dialog.
If the dialog is not currently visible, it will be placed at this position when it becomes visible. Use this to get the dialog out of the way of a highlighted misspelled word in a document.
Definition at line 1367 of file kspell.cpp.
int KSpell::parseOneResponse | ( | const QString & | _buffer, | |
QString & | word, | |||
QStringList & | sugg | |||
) | [protected] |
Definition at line 679 of file kspell.cpp.
void KSpell::progress | ( | unsigned int | i | ) | [signal] |
Emitted during a check().
i
is between 1 and 100.
void KSpell::ready | ( | KSpell * | ) | [signal] |
Emitted after KSpell has verified that ISpell/ASpell is running and working properly.
Emitted when the user pressed "ReplaceAll" in the dialog.
void KSpell::setAutoDelete | ( | bool | _autoDelete | ) | [inline] |
void KSpell::setIgnoreTitleCase | ( | bool | b | ) |
Call setIgnoreTitleCase(true) to tell the spell-checker to ignore words with a 'title' case, i.e.
starting with an uppercase letter. They are spell-checked by default.
Definition at line 1379 of file kspell.cpp.
void KSpell::setIgnoreUpperWords | ( | bool | b | ) |
Call setIgnoreUpperWords(true) to tell the spell-checker to ignore words that are completely uppercase.
They are spell-checked by default.
Definition at line 1374 of file kspell.cpp.
void KSpell::setProgressResolution | ( | unsigned int | res | ) |
Sets the resolution (in percent) of the progress() signals.
E.g. setProgressResolution (10) instructs KSpell to send progress signals (at most) every 10% (10%, 20%, 30%...). The default is 10%.
Definition at line 1351 of file kspell.cpp.
void KSpell::setUpDialog | ( | bool | reallyusedialogbox = true |
) | [protected] |
Definition at line 368 of file kspell.cpp.
void KSpell::slotStopCancel | ( | int | result | ) | [protected, slot] |
Definition at line 1191 of file kspell.cpp.
void KSpell::startIspell | ( | ) | [protected] |
Definition at line 154 of file kspell.cpp.
spellStatus KSpell::status | ( | ) | const [inline] |
QStringList KSpell::suggestions | ( | ) | const [inline] |
Returns list of suggested word replacements.
After calling checkWord() (an in response to a misspelled() signal you can use this to get the list of suggestions (if any were available).
void KSpell::suggestWord | ( | KProcIO * | ) | [protected, slot] |
Definition at line 611 of file kspell.cpp.
int KSpell::widthDlg | ( | ) | const |
bool KSpell::writePersonalDictionary | ( | ) | [protected] |
Definition at line 405 of file kspell.cpp.
Member Data Documentation
bool KSpell::autoDelete [protected] |
QString KSpell::caption [protected] |
QTextCodec* KSpell::codec [protected] |
unsigned int KSpell::curprog [protected] |
QString KSpell::cwword [protected] |
QString KSpell::dialog3slot [protected] |
bool KSpell::dialogsetup [protected] |
bool KSpell::dialogwillprocess [protected] |
bool KSpell::dlgon [protected] |
QString KSpell::dlgorigword [protected] |
QString KSpell::dlgreplacement [protected] |
int KSpell::dlgresult [protected] |
QStringList KSpell::ignorelist [protected] |
KSpellConfig* KSpell::ksconfig [protected] |
KSpellDlg* KSpell::ksdlg [protected] |
unsigned int KSpell::lastlastline [protected] |
unsigned int KSpell::lastline [protected] |
int KSpell::lastpos [protected] |
spellStatus KSpell::m_status [protected] |
int KSpell::maxtrystart [protected] |
bool KSpell::modaldlg [protected] |
int KSpell::modalreturn = 0 [static, protected] |
QString KSpell::modaltext [static, protected] |
QWidget * KSpell::modalWidgetHack = 0 [static, protected] |
QString KSpell::newbuffer [protected] |
unsigned int KSpell::offset [protected] |
QString KSpell::orig [protected] |
QString KSpell::origbuffer [protected] |
QWidget* KSpell::parent [protected] |
bool KSpell::personaldict [protected] |
unsigned int KSpell::posinline [protected] |
KProcIO* KSpell::proc [protected] |
unsigned int KSpell::progres [protected] |
bool KSpell::progressbar [protected] |
QStringList KSpell::replacelist [protected] |
QStringList KSpell::sugg [protected] |
bool KSpell::texmode [protected] |
unsigned int KSpell::totalpos [protected] |
int KSpell::trystart [protected] |
bool KSpell::usedialog [protected] |
QStringList::Iterator KSpell::wlIt [protected] |
QStringList* KSpell::wordlist [protected] |
The documentation for this class was generated from the following files: