kdeui
KToolBarButton Class Reference
A toolbar button. More...
#include <ktoolbarbutton.h>
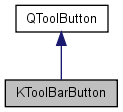
Public Slots | |
void | modeChange () |
virtual void | setTextLabel (const QString &, bool tipToo) |
Signals | |
void | buttonClicked (int, Qt::ButtonState state) |
void | clicked (int) |
void | doubleClicked (int) |
void | highlighted (int, bool) |
void | pressed (int) |
void | released (int) |
void | toggled (int) |
Public Member Functions | |
int | id () const |
KToolBarButton (QWidget *parent=0L, const char *name=0L) | |
KToolBarButton (const QPixmap &pixmap, int id, QWidget *parent, const char *name=0L, const QString &txt=QString::null) | |
KToolBarButton (const QString &icon, int id, QWidget *parent, const char *name=0L, const QString &txt=QString::null, KInstance *_instance=KGlobal::instance()) | |
void | on (bool flag=true) |
QPopupMenu * | popup () |
void | setDefaultIcon (const QString &icon) KDE_DEPRECATED |
void | setDefaultPixmap (const QPixmap &pixmap) KDE_DEPRECATED |
void | setDelayedPopup (QPopupMenu *p, bool unused=false) |
void | setDisabledIcon (const QString &icon) KDE_DEPRECATED |
void | setDisabledPixmap (const QPixmap &pixmap) KDE_DEPRECATED |
KDE_DEPRECATED void | setIcon (const QString &icon, bool generate) |
virtual void | setIcon (const QPixmap &pixmap) |
virtual void | setIcon (const QString &icon) |
virtual void | setIconSet (const QIconSet &iconset) |
void | setNoStyle (bool no_style=true) |
virtual void | setPixmap (const QPixmap &pixmap) KDE_DEPRECATED |
void | setPopup (QPopupMenu *p, bool unused=false) |
void | setRadio (bool f=true) |
virtual void | setText (const QString &text) |
void | setToggle (bool toggle=true) |
void | toggle () |
~KToolBarButton () | |
Protected Slots | |
void | slotClicked () |
void | slotDelayTimeout () |
void | slotPressed () |
void | slotReleased () |
void | slotToggled () |
Protected Member Functions | |
void | drawButton (QPainter *p) |
void | enterEvent (QEvent *e) |
bool | event (QEvent *e) |
bool | eventFilter (QObject *o, QEvent *e) |
int | iconTextMode () const |
bool | isActive () const |
bool | isRaised () const |
void | leaveEvent (QEvent *e) |
QSize | minimumSize () const |
QSize | minimumSizeHint () const |
void | mousePressEvent (QMouseEvent *) |
void | mouseReleaseEvent (QMouseEvent *) |
void | paletteChange (const QPalette &) |
void | showMenu () |
QSize | sizeHint () const |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
A toolbar button.This is used internally by KToolBar, use the KToolBar methods instead.
For internal use only.
Definition at line 44 of file ktoolbarbutton.h.
Constructor & Destructor Documentation
KToolBarButton::KToolBarButton | ( | const QString & | icon, | |
int | id, | |||
QWidget * | parent, | |||
const char * | name = 0L , |
|||
const QString & | txt = QString::null , |
|||
KInstance * | _instance = KGlobal::instance() | |||
) |
Construct a button with an icon loaded by the button itself.
This will trust the button to load the correct icon with the correct size.
- Parameters:
-
icon Name of icon to load (may be absolute or relative) id Id of this button parent This button's parent name This button's internal name txt This button's text (in a tooltip or otherwise) _instance the instance to use for this button
Definition at line 110 of file ktoolbarbutton.cpp.
KToolBarButton::KToolBarButton | ( | const QPixmap & | pixmap, | |
int | id, | |||
QWidget * | parent, | |||
const char * | name = 0L , |
|||
const QString & | txt = QString::null | |||
) |
Construct a button with an existing pixmap.
It is not recommended that you use this as the internal icon loading code will almost always get it "right".
- Parameters:
-
pixmap Name of icon to load (may be absolute or relative) id Id of this button parent This button's parent name This button's internal name txt This button's text (in a tooltip or otherwise)
Definition at line 144 of file ktoolbarbutton.cpp.
KToolBarButton::KToolBarButton | ( | QWidget * | parent = 0L , |
|
const char * | name = 0L | |||
) |
Construct a separator button.
- Parameters:
-
parent This button's parent name This button's internal name
Definition at line 100 of file ktoolbarbutton.cpp.
KToolBarButton::~KToolBarButton | ( | ) |
Member Function Documentation
void KToolBarButton::buttonClicked | ( | int | , | |
Qt::ButtonState | state | |||
) | [signal] |
Emitted when the toolbar button is clicked (with any mouse button).
- Parameters:
-
state makes it possible to find out which button was pressed, and whether any keyboard modifiers were held.
- Since:
- 3.4
void KToolBarButton::clicked | ( | int | ) | [signal] |
Emitted when the toolbar button is clicked (with LMB or MMB).
void KToolBarButton::doubleClicked | ( | int | ) | [signal] |
void KToolBarButton::drawButton | ( | QPainter * | p | ) | [protected] |
Definition at line 482 of file ktoolbarbutton.cpp.
void KToolBarButton::enterEvent | ( | QEvent * | e | ) | [protected] |
Definition at line 385 of file ktoolbarbutton.cpp.
bool KToolBarButton::event | ( | QEvent * | e | ) | [protected] |
Definition at line 641 of file ktoolbarbutton.cpp.
Definition at line 406 of file ktoolbarbutton.cpp.
void KToolBarButton::highlighted | ( | int | , | |
bool | ||||
) | [signal] |
int KToolBarButton::iconTextMode | ( | ) | const [protected] |
int KToolBarButton::id | ( | ) | const |
bool KToolBarButton::isActive | ( | ) | const [protected] |
bool KToolBarButton::isRaised | ( | ) | const [protected] |
void KToolBarButton::leaveEvent | ( | QEvent * | e | ) | [protected] |
Definition at line 373 of file ktoolbarbutton.cpp.
QSize KToolBarButton::minimumSize | ( | ) | const [protected] |
Definition at line 747 of file ktoolbarbutton.cpp.
QSize KToolBarButton::minimumSizeHint | ( | void | ) | const [protected] |
Definition at line 742 of file ktoolbarbutton.cpp.
void KToolBarButton::modeChange | ( | ) | [slot] |
This slot should be called whenever the toolbar mode has potentially changed.
This includes such events as text changing, orientation changing, etc.
Definition at line 181 of file ktoolbarbutton.cpp.
void KToolBarButton::mousePressEvent | ( | QMouseEvent * | e | ) | [protected] |
void KToolBarButton::mouseReleaseEvent | ( | QMouseEvent * | e | ) | [protected] |
void KToolBarButton::on | ( | bool | flag = true |
) |
Turn this button on or off.
- Parameters:
-
flag true or false
Definition at line 710 of file ktoolbarbutton.cpp.
void KToolBarButton::paletteChange | ( | const QPalette & | ) | [protected] |
Definition at line 632 of file ktoolbarbutton.cpp.
QPopupMenu * KToolBarButton::popup | ( | ) |
Return a pointer to this button's popup menu (if it exists).
Definition at line 353 of file ktoolbarbutton.cpp.
void KToolBarButton::pressed | ( | int | ) | [signal] |
void KToolBarButton::released | ( | int | ) | [signal] |
void KToolBarButton::setDefaultIcon | ( | const QString & | icon | ) |
- Deprecated:
- Force the button to use this icon as the default one rather then generating it using effects.
- Parameters:
-
icon The icon to use as the default (normal) one
Definition at line 325 of file ktoolbarbutton.cpp.
void KToolBarButton::setDefaultPixmap | ( | const QPixmap & | pixmap | ) |
- Deprecated:
- Force the button to use this pixmap as the default one rather then generating it using effects.
- Parameters:
-
pixmap The pixmap to use as the default (normal) one
Definition at line 311 of file ktoolbarbutton.cpp.
void KToolBarButton::setDelayedPopup | ( | QPopupMenu * | p, | |
bool | unused = false | |||
) |
Gives this button a delayed popup menu.
This function allows you to add a delayed popup menu to the button. The popup menu is then only displayed when the button is pressed and held down for about half a second.
- Parameters:
-
p the new popup menu unused Has no effect - ignore it.
Definition at line 367 of file ktoolbarbutton.cpp.
void KToolBarButton::setDisabledIcon | ( | const QString & | icon | ) |
- Deprecated:
- Force the button to use this icon when disabled one rather then generating it using effects.
- Parameters:
-
icon The icon to use when disabled
Definition at line 339 of file ktoolbarbutton.cpp.
void KToolBarButton::setDisabledPixmap | ( | const QPixmap & | pixmap | ) |
- Deprecated:
- Force the button to use this pixmap when disabled one rather then generating it using effects.
- Parameters:
-
pixmap The pixmap to use when disabled
Definition at line 318 of file ktoolbarbutton.cpp.
KDE_DEPRECATED void KToolBarButton::setIcon | ( | const QString & | icon, | |
bool | generate | |||
) | [inline] |
- Deprecated:
- Set the active icon for this button. The pixmap itself is loaded internally based on the icon size... .. the disabled and default pixmaps, however will only be constructed if generate is true. This function is preferred over setPixmap
- Parameters:
-
icon The name of the active icon generate If true, then the other icons are automagically generated from this one
Definition at line 164 of file ktoolbarbutton.h.
virtual void KToolBarButton::setIcon | ( | const QPixmap & | pixmap | ) | [inline, virtual] |
void KToolBarButton::setIcon | ( | const QString & | icon | ) | [virtual] |
Set the icon for this button.
The icon will be loaded internally with the correct size. This function is preferred over setIconSet
- Parameters:
-
icon The name of the icon
Definition at line 279 of file ktoolbarbutton.cpp.
void KToolBarButton::setIconSet | ( | const QIconSet & | iconset | ) | [virtual] |
Set the pixmaps for this toolbar button from a QIconSet.
If you call this you don't need to call any of the other methods that set icons or pixmaps.
- Parameters:
-
iconset The iconset to use
Reimplemented from QToolButton.
Definition at line 293 of file ktoolbarbutton.cpp.
void KToolBarButton::setNoStyle | ( | bool | no_style = true |
) |
Toolbar buttons naturally will assume the global styles concerning icons, icons sizes, etc.
You can use this function to explicitly turn this off, if you like.
- Parameters:
-
no_style Will disable styles if true
Definition at line 695 of file ktoolbarbutton.cpp.
void KToolBarButton::setPixmap | ( | const QPixmap & | pixmap | ) | [virtual] |
- Deprecated:
- Set the pixmap directly for this button. This pixmap should be the active one... the dimmed and disabled pixmaps are constructed based on this one. However, don't use this function unless you are positive that you don't want to use setIcon.
- Parameters:
-
pixmap The active pixmap
Definition at line 299 of file ktoolbarbutton.cpp.
void KToolBarButton::setPopup | ( | QPopupMenu * | p, | |
bool | unused = false | |||
) |
Give this button a popup menu.
There will not be a delay when you press the button. Use setDelayedPopup if you want that behavior.
- Parameters:
-
p The new popup menu unused Has no effect - ignore it.
Definition at line 360 of file ktoolbarbutton.cpp.
void KToolBarButton::setRadio | ( | bool | f = true |
) |
Turn this button into a radio button.
- Parameters:
-
f true or false
Definition at line 704 of file ktoolbarbutton.cpp.
void KToolBarButton::setText | ( | const QString & | text | ) | [virtual] |
Set the text for this button.
The text will be either used as a tooltip (IconOnly) or will be along side the icon
- Parameters:
-
text The button (or tooltip) text
Definition at line 273 of file ktoolbarbutton.cpp.
void KToolBarButton::setTextLabel | ( | const QString & | text, | |
bool | tipToo | |||
) | [virtual, slot] |
void KToolBarButton::setToggle | ( | bool | toggle = true |
) |
Turn this button into a toggle button or disable the toggle aspects of it.
This does not toggle the button itself. Use toggle() for that.
- Parameters:
-
toggle true or false
Definition at line 728 of file ktoolbarbutton.cpp.
void KToolBarButton::showMenu | ( | ) | [protected] |
Definition at line 655 of file ktoolbarbutton.cpp.
QSize KToolBarButton::sizeHint | ( | void | ) | const [protected] |
Definition at line 737 of file ktoolbarbutton.cpp.
void KToolBarButton::slotClicked | ( | ) | [protected, slot] |
Definition at line 667 of file ktoolbarbutton.cpp.
void KToolBarButton::slotDelayTimeout | ( | ) | [protected, slot] |
Definition at line 661 of file ktoolbarbutton.cpp.
void KToolBarButton::slotPressed | ( | ) | [protected, slot] |
Definition at line 680 of file ktoolbarbutton.cpp.
void KToolBarButton::slotReleased | ( | ) | [protected, slot] |
Definition at line 685 of file ktoolbarbutton.cpp.
void KToolBarButton::slotToggled | ( | ) | [protected, slot] |
Definition at line 690 of file ktoolbarbutton.cpp.
void KToolBarButton::toggle | ( | ) |
Toggle this button.
Reimplemented from QToolButton.
Definition at line 722 of file ktoolbarbutton.cpp.
void KToolBarButton::toggled | ( | int | ) | [signal] |
void KToolBarButton::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 778 of file ktoolbarbutton.cpp.
The documentation for this class was generated from the following files: