KHTML
KJavaApplet Class Reference
#include <kjavaapplet.h>
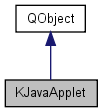
Detailed Description
Definition at line 50 of file kjavaapplet.h.
Member Enumeration Documentation
Definition at line 57 of file kjavaapplet.h.
Constructor & Destructor Documentation
KJavaApplet::KJavaApplet | ( | KJavaAppletWidget * | _parent, | |
KJavaAppletContext * | _context = 0 | |||
) |
Definition at line 49 of file kjavaapplet.cpp.
KJavaApplet::~KJavaApplet | ( | ) |
Definition at line 65 of file kjavaapplet.cpp.
Member Function Documentation
QString & KJavaApplet::appletClass | ( | ) |
Get the name of the Class file the applet should run.
Definition at line 89 of file kjavaapplet.cpp.
int KJavaApplet::appletId | ( | ) |
QString & KJavaApplet::appletName | ( | ) |
Get the name the applet should be called in its context.
Definition at line 176 of file kjavaapplet.cpp.
QString & KJavaApplet::archives | ( | ) |
Get the list of Archives that should be searched for class files and other resources.
Definition at line 144 of file kjavaapplet.cpp.
const QString& KJavaApplet::authName | ( | ) | const [inline] |
Definition at line 222 of file kjavaapplet.h.
QString & KJavaApplet::baseURL | ( | ) |
get the Base URL of the document embedding the applet
Definition at line 114 of file kjavaapplet.cpp.
QString & KJavaApplet::codeBase | ( | ) |
void KJavaApplet::create | ( | ) |
Send message to AppletServer to create this applet's frame to be swallowed and download the applet classes.
Definition at line 181 of file kjavaapplet.cpp.
bool KJavaApplet::failed | ( | ) | const |
Definition at line 284 of file kjavaapplet.cpp.
KJavaAppletContext* KJavaApplet::getContext | ( | ) | const [inline] |
Definition at line 204 of file kjavaapplet.h.
QString & KJavaApplet::getWindowName | ( | ) |
void KJavaApplet::init | ( | ) |
Send message to AppletServer to Initialize and show this applet.
Definition at line 188 of file kjavaapplet.cpp.
bool KJavaApplet::isAlive | ( | ) | const |
Definition at line 272 of file kjavaapplet.cpp.
bool KJavaApplet::isCreated | ( | ) |
Returns status of applet- whether it's been created or not.
Definition at line 73 of file kjavaapplet.cpp.
void KJavaApplet::jsData | ( | const QStringList & | args | ) | [inline] |
void KJavaApplet::jsEvent | ( | const QStringList & | args | ) | [signal] |
Look up the parameter value for the given Parameter.
Returns QString::null if the name has not been set.
Definition at line 94 of file kjavaapplet.cpp.
const QString& KJavaApplet::password | ( | ) | const [inline] |
Definition at line 216 of file kjavaapplet.h.
void KJavaApplet::setAppletClass | ( | const QString & | clazzName | ) |
Specify the name of the class file to run.
For example 'Lake.class'.
Definition at line 84 of file kjavaapplet.cpp.
void KJavaApplet::setAppletContext | ( | KJavaAppletContext * | _context | ) |
void KJavaApplet::setAppletId | ( | int | id | ) |
void KJavaApplet::setAppletName | ( | const QString & | name | ) |
Set the name the applet should be called in its context.
Definition at line 161 of file kjavaapplet.cpp.
void KJavaApplet::setArchives | ( | const QString & | _archives | ) |
Set the list of archives at the Applet's codebase to search in for class files and other resources.
Definition at line 139 of file kjavaapplet.cpp.
void KJavaApplet::setAuthName | ( | const QString & | _auth | ) | [inline] |
void KJavaApplet::setBaseURL | ( | const QString & | base | ) |
void KJavaApplet::setCodeBase | ( | const QString & | codeBase | ) |
void KJavaApplet::setFailed | ( | ) |
Definition at line 268 of file kjavaapplet.cpp.
void KJavaApplet::setPassword | ( | const QString & | _password | ) | [inline] |
void KJavaApplet::setSize | ( | QSize | size | ) |
void KJavaApplet::setUser | ( | const QString & | _user | ) | [inline] |
void KJavaApplet::setWindowName | ( | const QString & | title | ) |
QSize KJavaApplet::size | ( | ) |
void KJavaApplet::start | ( | ) |
KJavaApplet::AppletState KJavaApplet::state | ( | ) | const |
Definition at line 280 of file kjavaapplet.cpp.
void KJavaApplet::stateChange | ( | const int | newState | ) |
called from the protocol engine changes the status according to the one on the java side.
Do not call this yourself!
Definition at line 213 of file kjavaapplet.cpp.
void KJavaApplet::stop | ( | ) |
const QString& KJavaApplet::user | ( | ) | const [inline] |
Definition at line 210 of file kjavaapplet.h.
The documentation for this class was generated from the following files: