KHTML
KJavaProcess Class Reference
#include <kjavaprocess.h>
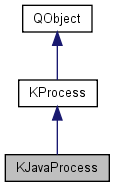
Signals | |
void | exited (int status) |
void | received (const QByteArray &) |
Public Member Functions | |
void | flushBuffers () |
bool | isRunning () |
KJavaProcess () | |
void | send (char cmd_code, const QStringList &args, const QByteArray &data) |
void | send (char cmd_code, const QStringList &args) |
void | setClassArgs (const QString &classArgs) |
void | setClasspath (const QString &classpath) |
void | setExtraArgs (const QString &args) |
void | setJVMPath (const QString &path) |
void | setMainClass (const QString &clazzName) |
void | setSystemProperty (const QString &name, const QString &value) |
bool | startJava () |
void | stopJava () |
virtual | ~KJavaProcess () |
Protected Slots | |
void | slotExited (KProcess *process) |
void | slotReceivedData (int, int &) |
void | slotWroteData () |
Protected Member Functions | |
QByteArray * | addArgs (char cmd_code, const QStringList &args) |
virtual bool | invokeJVM () |
virtual void | killJVM () |
void | popBuffer () |
void | sendBuffer (QByteArray *buff) |
void | storeSize (QByteArray *buff) |
Protected Attributes | |
KProcess * | javaProcess |
Detailed Description
Definition at line 42 of file kjavaprocess.h.
Constructor & Destructor Documentation
KJavaProcess::KJavaProcess | ( | ) |
Creates a process object, the process is NOT invoked at this point.
You should first set the process's parameters, and then call startJava.
Definition at line 49 of file kjavaprocess.cpp.
KJavaProcess::~KJavaProcess | ( | ) | [virtual] |
Definition at line 68 of file kjavaprocess.cpp.
Member Function Documentation
QByteArray * KJavaProcess::addArgs | ( | char | cmd_code, | |
const QStringList & | args | |||
) | [protected] |
Definition at line 127 of file kjavaprocess.cpp.
void KJavaProcess::exited | ( | int | status | ) | [signal] |
void KJavaProcess::flushBuffers | ( | ) |
bool KJavaProcess::invokeJVM | ( | ) | [protected, virtual] |
Definition at line 252 of file kjavaprocess.cpp.
bool KJavaProcess::isRunning | ( | ) |
Returns the status of the java Process- true if it's ok, false if it has died.
It calls KProcess::isRunning()
Definition at line 80 of file kjavaprocess.cpp.
void KJavaProcess::killJVM | ( | ) | [protected, virtual] |
Definition at line 319 of file kjavaprocess.cpp.
void KJavaProcess::popBuffer | ( | ) | [protected] |
Definition at line 212 of file kjavaprocess.cpp.
void KJavaProcess::received | ( | const QByteArray & | ) | [signal] |
void KJavaProcess::send | ( | char | cmd_code, | |
const QStringList & | args, | |||
const QByteArray & | data | |||
) |
Sends a command to the KJAS Applet Server by building a QByteArray out of the data, and then writes it standard out.
It adds each QString in the arg list, and then adds the data array.
Definition at line 194 of file kjavaprocess.cpp.
void KJavaProcess::send | ( | char | cmd_code, | |
const QStringList & | args | |||
) |
Sends a command to the KJAS Applet Server by building a QByteArray out of the data, and then writes it standard out.
Definition at line 183 of file kjavaprocess.cpp.
void KJavaProcess::sendBuffer | ( | QByteArray * | buff | ) | [protected] |
Definition at line 174 of file kjavaprocess.cpp.
void KJavaProcess::setClassArgs | ( | const QString & | classArgs | ) |
Arguments passed to the main class.
They will be very last in the java command line, after the main class.
Definition at line 121 of file kjavaprocess.cpp.
void KJavaProcess::setClasspath | ( | const QString & | classpath | ) |
This will set the classpath the Java process will use.
It's used as a the -cp command line option. It adds every jar file stored in $KDEDIRS/share/apps/kjava/ to the classpath, and then adds the $CLASSPATH environmental variable. This allows users to simply drop the JSSE (Java Secure Sockets Extension classes into that directory without having to modify the jvm configuration files.
Definition at line 100 of file kjavaprocess.cpp.
void KJavaProcess::setExtraArgs | ( | const QString & | args | ) |
void KJavaProcess::setJVMPath | ( | const QString & | path | ) |
Used to specify the path to the Java executable to be run.
Definition at line 95 of file kjavaprocess.cpp.
void KJavaProcess::setMainClass | ( | const QString & | clazzName | ) |
Set a property on the java command line as -Dname=value, or -Dname if value is QString::null.
For example, you could call setSystemProperty( "kjas.debug", "" ) to set the kjas.debug property.
Definition at line 105 of file kjavaprocess.cpp.
void KJavaProcess::slotExited | ( | KProcess * | process | ) | [protected, slot] |
This slot is called when the Java Process writes to standard out.
We then process the data from the file descriptor that is passed to us and send the command to the AppletServer
Definition at line 340 of file kjavaprocess.cpp.
void KJavaProcess::slotWroteData | ( | ) | [protected, slot] |
This slot is called whenever something is written to stdin of the process.
It's called again to make sure we keep emptying out the buffer that contains the messages we need send.
Definition at line 239 of file kjavaprocess.cpp.
bool KJavaProcess::startJava | ( | ) |
Invoke the JVM with the parameters that have been set.
The Java process will start after this call.
Definition at line 85 of file kjavaprocess.cpp.
void KJavaProcess::stopJava | ( | ) |
void KJavaProcess::storeSize | ( | QByteArray * | buff | ) | [protected] |
Definition at line 163 of file kjavaprocess.cpp.
Member Data Documentation
KProcess* KJavaProcess::javaProcess [protected] |
Definition at line 153 of file kjavaprocess.h.
The documentation for this class was generated from the following files: