kioslave
HTTPProtocol Class Reference
#include <http.h>
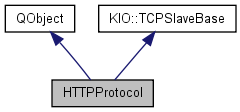
Classes | |
struct | DAVRequest |
DAV-specific request elements for the current connection. More... | |
struct | DigestAuthInfo |
struct | HTTPRequest |
The request for the current connection. More... | |
struct | HTTPState |
HTTP / DAV method. More... | |
Public Types | |
enum | HTTP_AUTH { AUTH_None, AUTH_Basic, AUTH_NTLM, AUTH_Digest, AUTH_Negotiate } |
enum | HTTP_REV { HTTP_None, HTTP_Unknown, HTTP_10, HTTP_11, SHOUTCAST } |
Public Member Functions | |
void | cacheUpdate (const KURL &url, bool nocache, time_t expireDate) |
bool | checkRequestURL (const KURL &) |
virtual void | closeConnection () |
virtual void | copy (const KURL &src, const KURL &dest, int permissions, bool overwrite) |
QString | davError (int code=-1, QString url=QString::null) |
void | davFinished () |
void | davGeneric (const KURL &url, KIO::HTTP_METHOD method) |
bool | davHostOk () |
void | davLock (const KURL &url, const QString &scope, const QString &type, const QString &owner) |
void | davUnlock (const KURL &url) |
virtual void | del (const KURL &url, bool isfile) |
virtual void | get (const KURL &url) |
void | httpError () |
HTTPProtocol (const QCString &protocol, const QCString &pool, const QCString &app) | |
bool | isOffline (const KURL &url) |
virtual void | listDir (const KURL &url) |
virtual void | mimetype (const KURL &url) |
virtual void | mkdir (const KURL &url, int permissions) |
void | multiGet (const QByteArray &data) |
void | post (const KURL &url) |
virtual void | put (const KURL &url, int permissions, bool overwrite, bool resume) |
virtual void | rename (const KURL &src, const KURL &dest, bool overwrite) |
virtual void | reparseConfiguration () |
virtual void | setHost (const QString &host, int port, const QString &user, const QString &pass) |
virtual void | slave_status () |
virtual void | special (const QByteArray &data) |
virtual void | stat (const KURL &url) |
virtual | ~HTTPProtocol () |
Protected Slots | |
void | error (int _errid, const QString &_text) |
void | slotData (const QByteArray &) |
Protected Member Functions | |
void | addCookies (const QString &url, const QCString &cookieHeader) |
void | addEncoding (QString, QStringList &) |
void | calculateResponse (DigestAuthInfo &info, QCString &Response) |
FILE * | checkCacheEntry (bool readWrite=false) |
void | cleanCache () |
void | closeCacheEntry () |
int | codeFromResponse (const QString &response) |
void | configAuth (char *, bool) |
QString | createBasicAuth (bool isForProxy=false) |
void | createCacheEntry (const QString &mimetype, time_t expireDate) |
QString | createDigestAuth (bool isForProxy=false) |
QString | createNegotiateAuth () |
QString | createNTLMAuth (bool isForProxy=false) |
void | davParseActiveLocks (const QDomNodeList &activeLocks, uint &lockCount) |
void | davParsePropstats (const QDomNodeList &propstats, KIO::UDSEntry &entry) |
QString | davProcessLocks () |
void | davSetRequest (const QCString &requestXML) |
void | davStatList (const KURL &url, bool stat=true) |
QString | findCookies (const QString &url) |
void | forwardHttpResponseHeader () |
bool | getAuthorization () |
char * | gets (char *str, int size) |
QCString | gssError (int major_status, int minor_status) |
void | httpCheckConnection () |
void | httpClose (bool keepAlive) |
void | httpCloseConnection () |
bool | httpOpen () |
bool | httpOpenConnection () |
long | parseDateTime (const QString &input, const QString &type) |
void | promptInfo (KIO::AuthInfo &info) |
QString | proxyAuthenticationHeader () |
ssize_t | read (void *b, size_t nbytes) |
bool | readBody (bool dataInternal=false) |
int | readChunked () |
bool | readHeader () |
int | readLimited () |
int | readUnlimited () |
void | resetConnectionSettings () |
void | resetResponseSettings () |
void | resetSessionSettings () |
void | retrieveContent (bool dataInternal=false) |
bool | retrieveHeader (bool close_connection=true) |
bool | retryPrompt () |
void | rewind () |
void | saveAuthorization () |
bool | sendBody () |
void | setRewindMarker () |
void | updateExpireDate (time_t expireDate, bool updateCreationDate=false) |
ssize_t | write (const void *buf, size_t nbytes) |
void | writeCacheEntry (const char *buffer, int nbytes) |
Protected Attributes | |
HTTP_AUTH | Authentication |
bool | m_bBusy |
bool | m_bChunked |
bool | m_bEOD |
bool | m_bEOF |
bool | m_bError |
bool | m_bFirstRequest |
bool | m_bIsTunneled |
bool | m_bKeepAlive |
bool | m_bNeedTunnel |
bool | m_bPersistentProxyConnection |
bool | m_bProxyAuthValid |
bool | m_bRedirect |
QByteArray | m_bufPOST |
QByteArray | m_bufReceive |
QByteArray | m_bufWebDavData |
bool | m_bUnauthorized |
bool | m_bUseProxy |
bool | m_cpMimeBuffer |
bool | m_dataInternal |
QStringList | m_davCapabilities |
bool | m_davHostOk |
bool | m_davHostUnsupported |
KIO::filesize_t | m_iBytesLeft |
KIO::filesize_t | m_iContentLeft |
short unsigned int | m_iProxyAuthCount |
int | m_iProxyPort |
KIO::filesize_t | m_iSize |
short unsigned int | m_iWWWAuthCount |
int | m_keepAliveTimeout |
char | m_lineBuf [1024] |
char * | m_lineBufUnget |
size_t | m_lineCount |
size_t | m_lineCountUnget |
char * | m_linePtr |
char * | m_linePtrUnget |
int | m_maxCacheAge |
long | m_maxCacheSize |
QByteArray | m_mimeTypeBuffer |
int | m_pid |
unsigned int | m_prevResponseCode |
QCString | m_protocol |
int | m_proxyConnTimeout |
KURL | m_proxyURL |
QStringList | m_qContentEncodings |
QStringList | m_qTransferEncodings |
KURL | m_redirectLocation |
int | m_remoteConnTimeout |
int | m_remoteRespTimeout |
HTTPRequest | m_request |
QPtrList< HTTPRequest > | m_requestQueue |
unsigned int | m_responseCode |
QStringList | m_responseHeader |
char | m_rewindBuf [8192] |
size_t | m_rewindCount |
QString | m_sContentMD5 |
HTTPState | m_state |
QString | m_strAuthorization |
QString | m_strCacheDir |
QString | m_strMimeType |
QString | m_strProxyAuthorization |
QString | m_strProxyRealm |
QString | m_strRealm |
HTTP_AUTH | ProxyAuthentication |
Detailed Description
Definition at line 49 of file http.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
Member Function Documentation
void HTTPProtocol::addEncoding | ( | QString | encoding, | |
QStringList & | encs | |||
) | [protected] |
void HTTPProtocol::cacheUpdate | ( | const KURL & | url, | |
bool | nocache, | |||
time_t | expireDate | |||
) |
void HTTPProtocol::calculateResponse | ( | DigestAuthInfo & | info, | |
QCString & | Response | |||
) | [protected] |
FILE * HTTPProtocol::checkCacheEntry | ( | bool | readWrite = false |
) | [protected] |
Do a cache lookup for the current url.
(m_state.url)
- Parameters:
-
readWrite If true, file is opened read/write. If false, file is opened read-only.
- Returns:
- a file stream open for reading and at the start of the header section when the Cache entry exists and is valid. 0 if no cache entry could be found, or if the entry is not valid (any more).
void HTTPProtocol::cleanCache | ( | ) | [protected] |
void HTTPProtocol::closeCacheEntry | ( | ) | [protected] |
int HTTPProtocol::codeFromResponse | ( | const QString & | response | ) | [protected] |
void HTTPProtocol::configAuth | ( | char * | p, | |
bool | isForProxy | |||
) | [protected] |
void HTTPProtocol::copy | ( | const KURL & | src, | |
const KURL & | dest, | |||
int | permissions, | |||
bool | overwrite | |||
) | [virtual] |
QString HTTPProtocol::createBasicAuth | ( | bool | isForProxy = false |
) | [protected] |
void HTTPProtocol::createCacheEntry | ( | const QString & | mimetype, | |
time_t | expireDate | |||
) | [protected] |
QString HTTPProtocol::createDigestAuth | ( | bool | isForProxy = false |
) | [protected] |
QString HTTPProtocol::createNegotiateAuth | ( | ) | [protected] |
QString HTTPProtocol::createNTLMAuth | ( | bool | isForProxy = false |
) | [protected] |
void HTTPProtocol::davGeneric | ( | const KURL & | url, | |
KIO::HTTP_METHOD | method | |||
) |
void HTTPProtocol::davParseActiveLocks | ( | const QDomNodeList & | activeLocks, | |
uint & | lockCount | |||
) | [protected] |
void HTTPProtocol::davParsePropstats | ( | const QDomNodeList & | propstats, | |
KIO::UDSEntry & | entry | |||
) | [protected] |
QString HTTPProtocol::davProcessLocks | ( | ) | [protected] |
void HTTPProtocol::davSetRequest | ( | const QCString & | requestXML | ) | [protected] |
void HTTPProtocol::davStatList | ( | const KURL & | url, | |
bool | stat = true | |||
) | [protected] |
void HTTPProtocol::del | ( | const KURL & | url, | |
bool | isfile | |||
) | [virtual] |
void HTTPProtocol::error | ( | int | _errid, | |
const QString & | _text | |||
) | [protected, slot] |
void HTTPProtocol::forwardHttpResponseHeader | ( | ) | [protected] |
bool HTTPProtocol::getAuthorization | ( | ) | [protected] |
char * HTTPProtocol::gets | ( | char * | str, | |
int | size | |||
) | [protected] |
QCString HTTPProtocol::gssError | ( | int | major_status, | |
int | minor_status | |||
) | [protected] |
void HTTPProtocol::httpClose | ( | bool | keepAlive | ) | [protected] |
bool HTTPProtocol::httpOpen | ( | ) | [protected] |
This function is responsible for opening up the connection to the remote HTTP server and sending the header.
If this requires special authentication or other such fun stuff, then it will handle it. This function will NOT receive anything from the server, however. This is in contrast to previous incarnations of 'httpOpen'.
The reason for the change is due to one small fact: some requests require data to be sent in addition to the header (POST requests) and there is no way for this function to get that data. This function is called in the slotPut() or slotGet() functions which, in turn, are called (indirectly) as a result of a KIOJob::put() or KIOJob::get(). It is those latter functions which are responsible for starting up this ioslave in the first place. This means that 'httpOpen' is called (essentially) as soon as the ioslave is created -- BEFORE any data gets to this slave.
The basic process now is this:
1) Open up the socket and port 2) Format our request/header 3) Send the header to the remote server
void HTTPProtocol::mkdir | ( | const KURL & | url, | |
int | permissions | |||
) | [virtual] |
void HTTPProtocol::multiGet | ( | const QByteArray & | data | ) |
void HTTPProtocol::promptInfo | ( | KIO::AuthInfo & | info | ) | [protected] |
Creates authorization prompt info.
QString HTTPProtocol::proxyAuthenticationHeader | ( | ) | [protected] |
void HTTPProtocol::put | ( | const KURL & | url, | |
int | permissions, | |||
bool | overwrite, | |||
bool | resume | |||
) | [virtual] |
ssize_t HTTPProtocol::read | ( | void * | b, | |
size_t | nbytes | |||
) | [protected] |
bool HTTPProtocol::readBody | ( | bool | dataInternal = false |
) | [protected] |
This function is our "receive" function.
It is responsible for downloading the message (not the header) from the HTTP server. It is called either as a response to a client's KIOJob::dataEnd() (meaning that the client is done sending data) or by 'httpOpen()' (if we are in the process of a PUT/POST request). It can also be called by a webDAV function, to receive stat/list/property/etc. data; in this case the data is stored in m_bufWebDavData.
int HTTPProtocol::readChunked | ( | ) | [protected] |
bool HTTPProtocol::readHeader | ( | ) | [protected] |
void HTTPProtocol::rename | ( | const KURL & | src, | |
const KURL & | dest, | |||
bool | overwrite | |||
) | [virtual] |
void HTTPProtocol::resetConnectionSettings | ( | ) | [protected] |
void HTTPProtocol::resetResponseSettings | ( | ) | [protected] |
void HTTPProtocol::resetSessionSettings | ( | ) | [protected] |
void HTTPProtocol::retrieveContent | ( | bool | dataInternal = false |
) | [protected] |
bool HTTPProtocol::retrieveHeader | ( | bool | close_connection = true |
) | [protected] |
bool HTTPProtocol::retryPrompt | ( | ) | [protected] |
void HTTPProtocol::saveAuthorization | ( | ) | [protected] |
void HTTPProtocol::slotData | ( | const QByteArray & | _d | ) | [protected, slot] |
void HTTPProtocol::special | ( | const QByteArray & | data | ) | [virtual] |
void HTTPProtocol::updateExpireDate | ( | time_t | expireDate, | |
bool | updateCreationDate = false | |||
) | [protected] |
ssize_t HTTPProtocol::write | ( | const void * | buf, | |
size_t | nbytes | |||
) | [protected] |
A "smart" wrapper around write that will use SSL_write or write(2) depending on whether you've got an SSL connection or not.
The only shortcomming is that it uses the "global" file handles and soforth. So you can't really use this on individual files/sockets.
void HTTPProtocol::writeCacheEntry | ( | const char * | buffer, | |
int | nbytes | |||
) | [protected] |
Member Data Documentation
HTTP_AUTH HTTPProtocol::Authentication [protected] |
bool HTTPProtocol::m_bBusy [protected] |
bool HTTPProtocol::m_bChunked [protected] |
bool HTTPProtocol::m_bEOD [protected] |
bool HTTPProtocol::m_bEOF [protected] |
bool HTTPProtocol::m_bError [protected] |
bool HTTPProtocol::m_bFirstRequest [protected] |
bool HTTPProtocol::m_bIsTunneled [protected] |
bool HTTPProtocol::m_bKeepAlive [protected] |
bool HTTPProtocol::m_bNeedTunnel [protected] |
bool HTTPProtocol::m_bPersistentProxyConnection [protected] |
bool HTTPProtocol::m_bProxyAuthValid [protected] |
bool HTTPProtocol::m_bRedirect [protected] |
QByteArray HTTPProtocol::m_bufPOST [protected] |
QByteArray HTTPProtocol::m_bufReceive [protected] |
QByteArray HTTPProtocol::m_bufWebDavData [protected] |
bool HTTPProtocol::m_bUnauthorized [protected] |
bool HTTPProtocol::m_bUseProxy [protected] |
bool HTTPProtocol::m_cpMimeBuffer [protected] |
bool HTTPProtocol::m_dataInternal [protected] |
QStringList HTTPProtocol::m_davCapabilities [protected] |
bool HTTPProtocol::m_davHostOk [protected] |
bool HTTPProtocol::m_davHostUnsupported [protected] |
KIO::filesize_t HTTPProtocol::m_iBytesLeft [protected] |
KIO::filesize_t HTTPProtocol::m_iContentLeft [protected] |
short unsigned int HTTPProtocol::m_iProxyAuthCount [protected] |
int HTTPProtocol::m_iProxyPort [protected] |
KIO::filesize_t HTTPProtocol::m_iSize [protected] |
short unsigned int HTTPProtocol::m_iWWWAuthCount [protected] |
int HTTPProtocol::m_keepAliveTimeout [protected] |
char HTTPProtocol::m_lineBuf[1024] [protected] |
char* HTTPProtocol::m_lineBufUnget [protected] |
size_t HTTPProtocol::m_lineCount [protected] |
size_t HTTPProtocol::m_lineCountUnget [protected] |
char* HTTPProtocol::m_linePtr [protected] |
char* HTTPProtocol::m_linePtrUnget [protected] |
int HTTPProtocol::m_maxCacheAge [protected] |
long HTTPProtocol::m_maxCacheSize [protected] |
QByteArray HTTPProtocol::m_mimeTypeBuffer [protected] |
int HTTPProtocol::m_pid [protected] |
unsigned int HTTPProtocol::m_prevResponseCode [protected] |
QCString HTTPProtocol::m_protocol [protected] |
int HTTPProtocol::m_proxyConnTimeout [protected] |
KURL HTTPProtocol::m_proxyURL [protected] |
QStringList HTTPProtocol::m_qContentEncodings [protected] |
QStringList HTTPProtocol::m_qTransferEncodings [protected] |
KURL HTTPProtocol::m_redirectLocation [protected] |
int HTTPProtocol::m_remoteConnTimeout [protected] |
int HTTPProtocol::m_remoteRespTimeout [protected] |
HTTPRequest HTTPProtocol::m_request [protected] |
QPtrList<HTTPRequest> HTTPProtocol::m_requestQueue [protected] |
unsigned int HTTPProtocol::m_responseCode [protected] |
QStringList HTTPProtocol::m_responseHeader [protected] |
char HTTPProtocol::m_rewindBuf[8192] [protected] |
size_t HTTPProtocol::m_rewindCount [protected] |
QString HTTPProtocol::m_sContentMD5 [protected] |
HTTPState HTTPProtocol::m_state [protected] |
QString HTTPProtocol::m_strAuthorization [protected] |
QString HTTPProtocol::m_strCacheDir [protected] |
QString HTTPProtocol::m_strMimeType [protected] |
QString HTTPProtocol::m_strProxyAuthorization [protected] |
QString HTTPProtocol::m_strProxyRealm [protected] |
QString HTTPProtocol::m_strRealm [protected] |
HTTP_AUTH HTTPProtocol::ProxyAuthentication [protected] |
The documentation for this class was generated from the following files: