kioslaves
imapParser Class Reference
#include <imapparser.h>
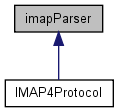
Detailed Description
Definition at line 187 of file imapparser.h.
Member Enumeration Documentation
the different states the client can be in
- Enumerator:
-
ISTATE_NO Not connected. ISTATE_CONNECT Connected but not logged in. ISTATE_LOGIN Logged in. ISTATE_SELECT A folder is currently selected.
Definition at line 193 of file imapparser.h.
Constructor & Destructor Documentation
imapParser::imapParser | ( | ) |
Definition at line 75 of file imapparser.cc.
imapParser::~imapParser | ( | ) | [virtual] |
Definition at line 84 of file imapparser.cc.
Member Function Documentation
static QCString imapParser::b2c | ( | const QByteArray & | ba | ) | [inline, static] |
Definition at line 376 of file imapparser.h.
bool imapParser::clientAuthenticate | ( | KIO::SlaveBase * | slave, | |
KIO::AuthInfo & | ai, | |||
const QString & | aFQDN, | |||
const QString & | aAuth, | |||
bool | isSSL, | |||
QString & | resultInfo | |||
) |
non-plaintext login
- Parameters:
-
aUser Username aPass Password aAuth authentication method isSSL are we using SSL resultInfo The resultinfo from the command
- Returns:
- success or failure
Definition at line 218 of file imapparser.cc.
plaintext login
- Parameters:
-
aUser Username aPass Password resultInfo The resultinfo from the command
- Returns:
- success or failure
Definition at line 149 of file imapparser.cc.
imapCommand * imapParser::doCommand | ( | imapCommand * | aCmd | ) |
perform a command and wait to parse the result
- Parameters:
-
aCmd The command to perform
- Returns:
- The completed command
Definition at line 91 of file imapparser.cc.
const QByteArray& imapParser::getContinuation | ( | ) | [inline] |
Definition at line 417 of file imapparser.h.
const QString imapParser::getCurrentBox | ( | ) | [inline] |
Definition at line 211 of file imapparser.h.
imapCache* imapParser::getLastHandled | ( | ) | [inline] |
return the last handled foo
- Todo:
- work out what a foo is
Definition at line 395 of file imapparser.h.
const QStringList& imapParser::getResults | ( | ) | [inline] |
const imapInfo& imapParser::getSelected | ( | ) | [inline] |
enum IMAP_STATE imapParser::getState | ( | ) | [inline] |
const imapInfo& imapParser::getStatus | ( | ) | [inline] |
bool imapParser::hasCapability | ( | const QString & | cap | ) |
void imapParser::parseAcl | ( | parseString & | result | ) |
const mailAddress & imapParser::parseAddress | ( | parseString & | inWords, | |
mailAddress & | buffer | |||
) |
void imapParser::parseAddressList | ( | parseString & | inWords, | |
QPtrList< mailAddress > & | list | |||
) |
void imapParser::parseAnnotation | ( | parseString & | result | ) |
void imapParser::parseBody | ( | parseString & | inWords | ) |
mimeHeader * imapParser::parseBodyStructure | ( | parseString & | inWords, | |
QString & | section, | |||
mimeHeader * | inHeader = 0 | |||
) |
void imapParser::parseCapability | ( | parseString & | result | ) |
void imapParser::parseCustom | ( | parseString & | result | ) |
void imapParser::parseDelegate | ( | parseString & | result | ) |
QAsciiDict< QString > imapParser::parseDisposition | ( | parseString & | inWords | ) |
parse the disposition list (disposition (name value pairs)) the disposition has the key 'content-disposition'
Definition at line 1018 of file imapparser.cc.
mailHeader * imapParser::parseEnvelope | ( | parseString & | inWords | ) |
void imapParser::parseExists | ( | ulong | value, | |
parseString & | result | |||
) |
void imapParser::parseExpunge | ( | ulong | value, | |
parseString & | result | |||
) |
void imapParser::parseFetch | ( | ulong | value, | |
parseString & | inWords | |||
) |
parses the results of a fetch command processes it with the following sub parsers
Definition at line 1464 of file imapparser.cc.
void imapParser::parseFlags | ( | parseString & | result | ) |
void imapParser::parseList | ( | parseString & | result | ) |
void imapParser::parseListRights | ( | parseString & | result | ) |
QByteArray imapParser::parseLiteral | ( | parseString & | inWords, | |
bool | relay = false , |
|||
bool | stopAtBracket = false | |||
) | [inline] |
Definition at line 365 of file imapparser.h.
QCString imapParser::parseLiteralC | ( | parseString & | inWords, | |
bool | relay = false , |
|||
bool | stopAtBracket = false , |
|||
int * | outlen = 0 | |||
) |
int imapParser::parseLoop | ( | ) |
main loop for the parser reads one line and dispatches it to the appropriate sub parser
Definition at line 1747 of file imapparser.cc.
void imapParser::parseLsub | ( | parseString & | result | ) |
void imapParser::parseMyRights | ( | parseString & | result | ) |
void imapParser::parseNamespace | ( | parseString & | result | ) |
bool imapParser::parseOneNumber | ( | parseString & | inWords, | |
ulong & | num | |||
) | [static] |
QCString imapParser::parseOneWordC | ( | parseString & | inWords, | |
bool | stopAtBracket = FALSE , |
|||
int * | len = 0 | |||
) | [static] |
parse one word (maybe quoted) upto next space " ) ] }
Definition at line 1959 of file imapparser.cc.
void imapParser::parseOtherUser | ( | parseString & | result | ) |
void imapParser::parseOutOfOffice | ( | parseString & | result | ) |
QAsciiDict< QString > imapParser::parseParameters | ( | parseString & | inWords | ) |
void imapParser::parseQuota | ( | parseString & | result | ) |
void imapParser::parseQuotaRoot | ( | parseString & | result | ) |
bool imapParser::parseRead | ( | QByteArray & | buffer, | |
ulong | len, | |||
ulong | relay = 0 | |||
) | [virtual] |
read at least len bytes
Reimplemented in IMAP4Protocol.
Definition at line 1823 of file imapparser.cc.
bool imapParser::parseReadLine | ( | QByteArray & | buffer, | |
ulong | relay = 0 | |||
) | [virtual] |
read at least a line (up to CRLF)
Reimplemented in IMAP4Protocol.
Definition at line 1833 of file imapparser.cc.
void imapParser::parseRecent | ( | ulong | value, | |
parseString & | result | |||
) |
void imapParser::parseRelay | ( | ulong | len | ) | [virtual] |
relay hook to announce the fetched data directly to an upper level
Reimplemented in IMAP4Protocol.
Definition at line 1816 of file imapparser.cc.
void imapParser::parseRelay | ( | const QByteArray & | buffer | ) | [virtual] |
relay hook to send the fetched data directly to an upper level
Reimplemented in IMAP4Protocol.
Definition at line 1808 of file imapparser.cc.
void imapParser::parseResult | ( | QByteArray & | result, | |
parseString & | rest, | |||
const QString & | command = QString::null | |||
) |
void imapParser::parseSearch | ( | parseString & | result | ) |
void imapParser::parseSentence | ( | parseString & | inWords | ) |
mimeHeader * imapParser::parseSimplePart | ( | parseString & | inWords, | |
QString & | section, | |||
mimeHeader * | localPart = 0 | |||
) |
void imapParser::parseStatus | ( | parseString & | result | ) |
void imapParser::parseUntagged | ( | parseString & | result | ) |
parses all untagged responses and passes them on to the following parsers
Definition at line 341 of file imapparser.cc.
void imapParser::parseURL | ( | const KURL & | _url, | |
QString & | _box, | |||
QString & | _section, | |||
QString & | _type, | |||
QString & | _uid, | |||
QString & | _validity, | |||
QString & | _info | |||
) | [static] |
extract the box,section,list type, uid, uidvalidity,info from an url
Definition at line 1851 of file imapparser.cc.
void imapParser::parseWriteLine | ( | const QString & | str | ) | [virtual] |
write argument to server
Reimplemented in IMAP4Protocol.
Definition at line 1843 of file imapparser.cc.
void imapParser::removeCapability | ( | const QString & | cap | ) |
Definition at line 2064 of file imapparser.cc.
imapCommand * imapParser::sendCommand | ( | imapCommand * | aCmd | ) |
do setup and send the command to parseWriteLine
- Parameters:
-
aCmd The command to perform
- Returns:
- The completed command
Definition at line 104 of file imapparser.cc.
void imapParser::setState | ( | enum IMAP_STATE | state | ) | [inline] |
static void imapParser::skipWS | ( | parseString & | inWords | ) | [inline, static] |
Definition at line 427 of file imapparser.h.
Member Data Documentation
ulong imapParser::commandCounter [protected] |
Definition at line 477 of file imapparser.h.
QPtrList< imapCommand > imapParser::completeQueue [protected] |
Definition at line 463 of file imapparser.h.
QByteArray imapParser::continuation [protected] |
the last continuation request (there MUST not be more than one pending)
Definition at line 471 of file imapparser.h.
QString imapParser::currentBox [protected] |
enum IMAP_STATE imapParser::currentState [protected] |
QStringList imapParser::imapCapabilities [protected] |
QStringList imapParser::imapNamespaces [protected] |
list of namespaces in the form: section=namespace=delimiter section is 0 (personal), 1 (other users) or 2 (shared)
Definition at line 492 of file imapparser.h.
imapCache* imapParser::lastHandled [protected] |
Definition at line 475 of file imapparser.h.
QStringList imapParser::lastResults [protected] |
imapInfo imapParser::lastStatus [protected] |
QValueList< imapList > imapParser::listResponses [protected] |
QMap<QString, QString> imapParser::namespaceToDelimiter [protected] |
namespace prefix - delimiter association The namespace is cleaned before so that it does not contain the delimiter
Definition at line 486 of file imapparser.h.
QString imapParser::seenUid [protected] |
imapInfo imapParser::selectInfo [protected] |
here we store the result from select/examine and unsolicited updates
Definition at line 450 of file imapparser.h.
QPtrList< imapCommand > imapParser::sentQueue [protected] |
QStringList imapParser::unhandled [protected] |
everything we didn't handle, everything but the greeting is bogus
Definition at line 468 of file imapparser.h.
The documentation for this class was generated from the following files: