kpilot
ListCategorizer Class Reference
This Widget extends KListView for a particular purpose: sorting some items into some bins. More...
#include <listCat.h>
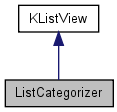
Public Member Functions | |
void | addCategories (const QStringList &) |
QListViewItem * | addCategory (const QString &name, const QString &description=QString::null) |
QListViewItem * | addItem (const QString &category, const QString &name, const QString &description=QString::null) |
QStringList | categories () const |
QListViewItem * | findCategory (const QString &categoryName) const |
QStringList | items (const QString &category, int column=0) const |
ListCategorizer (const QStringList &categories, bool startOpen, QWidget *parent, const char *name=0) | |
ListCategorizer (QWidget *parent, const char *name=0) | |
QStringList | listSiblings (const QListViewItem *p, int column=0) const |
void | setStartOpen (bool b) |
bool | startOpen () const |
Protected Member Functions | |
virtual bool | acceptDrag (QDropEvent *event) const |
virtual void | contentsDropEvent (QDropEvent *) |
virtual void | startDrag () |
Detailed Description
This Widget extends KListView for a particular purpose: sorting some items into some bins.This can be useful for putting items in an enabled / disabled state, or into categories, or configuring toolbars (putting icons onto toolbars).
You can use all of the standard KListView signals and slots. You may in particular want to change the names of the columns, for example:
ListCategorizer *lc = new ListCategorizer(this,colors); lc->setColumnText(0,i18n("Color")); lc->setColumnText(1,i18n("HTML")); QListViewItem *stdKDE = lc->addCategory(i18n("Standard KDE")); (void) new QListViewItem(stdKDE,i18n("red"),"#FF0000");
- Version:
- Id
- listCat.h 437980 2005-07-23 19:53:57Z kainhofe
Definition at line 64 of file listCat.h.
Constructor & Destructor Documentation
ListCategorizer::ListCategorizer | ( | QWidget * | parent, | |
const char * | name = 0 | |||
) |
Constructor.
This creates a new empty ListCategorizer with startOpen set to false. The parameters parent
and name
are the usual Qt ones.
Definition at line 39 of file listCat.cc.
ListCategorizer::ListCategorizer | ( | const QStringList & | categories, | |
bool | startOpen, | |||
QWidget * | parent, | |||
const char * | name = 0 | |||
) |
Constructor.
This creates a ListCategorizer with the given categories
already inserted. In addition, this constructor lets you specify whether or not startOpen is set.
Definition at line 48 of file listCat.cc.
Member Function Documentation
bool ListCategorizer::acceptDrag | ( | QDropEvent * | event | ) | const [protected, virtual] |
void ListCategorizer::addCategories | ( | const QStringList & | l | ) |
Add a list of categories to the ListCategorizer.
All the categories are added without descriptions; use addCategory on a per-category basis for that.
Definition at line 59 of file listCat.cc.
QListViewItem * ListCategorizer::addCategory | ( | const QString & | name, | |
const QString & | description = QString::null | |||
) |
Add a category with name name
and optional description
.
This can be useful if you want either a description for the category or want to refer to this category in the future without using findCategory().
- Returns:
- the QListViewItem created for the category
Definition at line 70 of file listCat.cc.
QListViewItem * ListCategorizer::addItem | ( | const QString & | category, | |
const QString & | name, | |||
const QString & | description = QString::null | |||
) |
Add a single item to the category named category
, with name name
and description set to description
.
This might be a convenience function, but it's probably more convenient to just use QListViewItem's constructor. That way you can also hide more data in the remaining columns.
Definition at line 174 of file listCat.cc.
QStringList ListCategorizer::categories | ( | ) | const [inline] |
Returns the list of names of the categories in the ListCategorizer.
void ListCategorizer::contentsDropEvent | ( | QDropEvent * | e | ) | [protected, virtual] |
QListViewItem * ListCategorizer::findCategory | ( | const QString & | categoryName | ) | const |
Given a category categoryName return the QListViewItem that represents that category.
Probably a useless function, since just remembering the pointer addCategory gives you is faster and uses hardly any memory.
Definition at line 159 of file listCat.cc.
QStringList ListCategorizer::items | ( | const QString & | category, | |
int | column = 0 | |||
) | const [inline] |
Returns the list of strings in column column
under category category
.
You can do this to get, for example the names of all the items categorized under a given category, or, more usefully, set column
to something other that 0 (name) or 1 (description) to return the QStringList hidden in the non-visible columns.
QStringList ListCategorizer::listSiblings | ( | const QListViewItem * | p, | |
int | column = 0 | |||
) | const |
Return the list of strings in column column
of all siblings of the given item p
.
If you remembered a pointer to a category, you can use
QStringList l = lc->listSiblings(stdKDE->firstChild(),2);
Definition at line 145 of file listCat.cc.
void ListCategorizer::setStartOpen | ( | bool | b | ) | [inline] |
void ListCategorizer::startDrag | ( | ) | [protected, virtual] |
bool ListCategorizer::startOpen | ( | ) | const [inline] |
The documentation for this class was generated from the following files: