kpilot
PilotAddress Class Reference
A wrapper class around the Address struct provided by pi-address.h. More...
#include <pilotAddress.h>
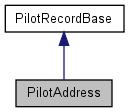
Detailed Description
A wrapper class around the Address struct provided by pi-address.h.This class allows the user to set and get address field values. For everything but phone fields, the user can simply pass the the pi-address enum for the index for setField() and getField() such as entryLastname.
Phone fields are a bit trickier. The structure allows for 8 possible phone fields with 5 possible slots. That means there could be three fields that don't have available storage. The setPhoneField() method will attempt to store the extra fields in a custom field if there is an overflow.
There are eight possible fields for 5 view slots:
- fields: Work, Home, Fax, Other, Pager, Mobile, E-mail, Main
- slots: entryPhone1, entryPhone2, entryPhone3, entryPhone4, entryPhone5
Internally in the pilot-link library, the AddressAppInfo phone array stores the strings for the eight possible phone values. Their English string values are :
- phone[0] = Work
- phone[1] = Home
- phone[2] = Fax
- phone[3] = Other
- phone[4] = E-mail
- phone[5] = Main
- phone[6] = Pager
- phone[7] = Mobile
Apparently, this order is kept for all languages, just with localized strings. The implementation of the internal methods will assume this order is kept. In other languages, main can replaced with Corporation.
Definition at line 190 of file pilotAddress.h.
Member Enumeration Documentation
Definition at line 245 of file pilotAddress.h.
Constructor & Destructor Documentation
PilotAddress::PilotAddress | ( | PilotRecord * | rec = 0L |
) |
Definition at line 179 of file pilotAddress.cc.
PilotAddress::PilotAddress | ( | const PilotAddress & | copyFrom | ) |
Definition at line 203 of file pilotAddress.cc.
PilotAddress::~PilotAddress | ( | ) | [virtual] |
Definition at line 270 of file pilotAddress.cc.
Member Function Documentation
struct Address* PilotAddress::address | ( | ) | const [inline, read] |
Definition at line 312 of file pilotAddress.h.
QStringList PilotAddress::getEmails | ( | ) | const |
Return list of all email addresses.
This will search through our "phone" fields and will return only those which are e-mail addresses.
Definition at line 416 of file pilotAddress.cc.
QString PilotAddress::getField | ( | int | field | ) | const |
Returns the text value of a given field field
(or QString::null if there is no such field).
Definition at line 462 of file pilotAddress.cc.
const char* PilotAddress::getFieldP | ( | int | field | ) | const [inline, protected] |
Definition at line 319 of file pilotAddress.h.
QString PilotAddress::getPhoneField | ( | PilotAddressInfo::EPhoneType | type | ) | const |
- Parameters:
-
type is the type of phone checkCustom4 flag if true, checks the entryCustom4 field for extra phone fields
- Returns:
- the field associated with the type
Definition at line 534 of file pilotAddress.cc.
PilotAddressInfo::EPhoneType PilotAddress::getPhoneType | ( | const PhoneSlot & | field | ) | const |
Get the phone type (label) for a given field field
in the record.
The field
must be within the phone range (entryPhone1 .. entryPhone5).
- Returns:
- Phone type for phone field
field
.eNone
(fake phone type) iffield
is invalid.
Definition at line 590 of file pilotAddress.cc.
PhoneSlot PilotAddress::getShownPhone | ( | ) | const |
Returns the slot of the phone number selected by the user to be shown in the overview of addresses.
- Returns:
- Slot of phone entry (between entryPhone1 and entryPhone5)
Definition at line 547 of file pilotAddress.cc.
QString PilotAddress::getTextRepresentation | ( | const PilotAddressInfo * | info, | |
Qt::TextFormat | richText | |||
) | const |
Returns a text representation of the address.
If richText
is true, the text will be formatted with Qt-HTML tags. The AppInfo structure info
is used to figure out the phone labels; if it is NULL then bogus labels are used to identify phone types.
Definition at line 276 of file pilotAddress.cc.
PilotAddress & PilotAddress::operator= | ( | const PilotAddress & | r | ) |
Definition at line 211 of file pilotAddress.cc.
bool PilotAddress::operator== | ( | const PilotAddress & | r | ) |
Definition at line 219 of file pilotAddress.cc.
PilotRecord * PilotAddress::pack | ( | ) | const |
Definition at line 623 of file pilotAddress.cc.
void PilotAddress::setEmails | ( | const QStringList & | emails | ) |
Definition at line 436 of file pilotAddress.cc.
Set a field i
to a given text value.
Uses the phone slots only.
Definition at line 220 of file pilotAddress.h.
void PilotAddress::setField | ( | int | field, | |
const QString & | text | |||
) |
- Parameters:
-
text set the field value field int values associated with the enum defined in pi-address.h. The copied possible enum's are: (copied from pi-address.h on 1/12/01) enum { entryLastname, entryFirstname, entryCompany, entryPhone1, entryPhone2, entryPhone3, entryPhone4, entryPhone5, entryAddress, entryCity, entryState, entryZip, entryCountry, entryTitle, entryCustom1, entryCustom2, entryCustom3, entryCustom4, entryNote };
Definition at line 602 of file pilotAddress.cc.
PhoneSlot PilotAddress::setPhoneField | ( | PilotAddressInfo::EPhoneType | type, | |
const QString & | value, | |||
PhoneHandlingFlags | flags | |||
) |
- Parameters:
-
type is the type of phone field is value to store overflowCustom is true, and entryPhone1 to entryPhone5 is full it will use entryCustom4 field to store the field overwriteExisting is true, it will overwrite an existing record-type with the field, else it will always search for the first available slot
- Returns:
- index of the field that this information was set to
Definition at line 489 of file pilotAddress.cc.
Set the shown phone (the one preferred by the user for display on the handheld's overview page) to the given slot
.
- Returns:
v
Definition at line 553 of file pilotAddress.cc.
PhoneSlot PilotAddress::setShownPhone | ( | PilotAddressInfo::EPhoneType | phoneType | ) |
Set the shown phone (the one preferred by the user for display on the handheld's overview page) to the type (not index) indicated.
Looks through the phone entries of this record to find the first one one of this type.
- Returns:
- Slot of phone entry.
- Note:
- Sets the shown phone to the first entry if no field of type
phoneType
can be found and no Home phone field (the fallback) can be found either.
Definition at line 563 of file pilotAddress.cc.
The documentation for this class was generated from the following files: