kpilot
PilotDateEntry Class Reference
This class is a wrapper for pilot-link's datebook entries (struct Appointment). More...
#include <pilotDateEntry.h>
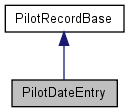
Public Member Functions | |
unsigned int | alarmLeadTime () const |
bool | doesFloat () const |
QDateTime | dtAlarm () const |
QDateTime | dtEnd () const |
QDateTime | dtRepeatEnd () const |
QDateTime | dtStart () const |
int | getAdvance () const |
int | getAdvanceUnits () const |
QString | getDescription () const |
struct tm | getEventEnd () const |
struct tm * | getEventEnd_p () const |
struct tm | getEventStart () const |
struct tm * | getEventStart_p () const |
int | getExceptionCount () const |
struct tm * | getExceptions () const |
QString | getLocation () const |
QString | getNote () const |
DayOfMonthType | getRepeatDay () const |
const int * | getRepeatDays () const |
struct tm | getRepeatEnd () const |
int | getRepeatForever () const |
int | getRepeatFrequency () const |
repeatTypes | getRepeatType () const |
QString | getTextRepresentation (Qt::TextFormat richText) |
bool | isAlarmEnabled () const |
bool | isEvent () const |
bool | isMultiDay () const |
PilotDateEntry & | operator= (const PilotDateEntry &e) |
PilotRecord * | pack () const |
PilotDateEntry (const PilotDateEntry &e) | |
PilotDateEntry (PilotRecord *rec) | |
PilotDateEntry () | |
void | setAdvance (int advance) |
void | setAdvanceUnits (int units) |
void | setAlarmEnabled (bool b) |
void | setDescription (const QString &) |
void | setEventEnd (struct tm &end) |
void | setEventStart (struct tm &start) |
void | setExceptionCount (int e) |
void | setExceptions (struct tm *e) |
void | setFloats (bool f) |
void | setLocation (const QString &) |
void | setNote (const QString &) |
void | setRepeatDay (DayOfMonthType rd) |
void | setRepeatDays (QBitArray rba) |
void | setRepeatDays (int *rd) |
void | setRepeatEnd (struct tm tm) |
void | setRepeatForever (int f=1) |
void | setRepeatFrequency (int f) |
void | setRepeatType (repeatTypes r) |
~PilotDateEntry () | |
Protected Member Functions | |
const char * | getDescriptionP () const |
const char * | getNoteP () const |
void | setDescriptionP (const char *desc, int l=-1) |
void | setNoteP (const char *note, int l=-1) |
Detailed Description
This class is a wrapper for pilot-link's datebook entries (struct Appointment).
Definition at line 72 of file pilotDateEntry.h.
Constructor & Destructor Documentation
PilotDateEntry::PilotDateEntry | ( | ) |
PilotDateEntry::PilotDateEntry | ( | PilotRecord * | rec | ) |
Constructor.
Interprets the given record as an appointment.
Definition at line 72 of file pilotDateEntry.cc.
PilotDateEntry::PilotDateEntry | ( | const PilotDateEntry & | e | ) |
PilotDateEntry::~PilotDateEntry | ( | ) | [inline] |
Member Function Documentation
unsigned int PilotDateEntry::alarmLeadTime | ( | ) | const |
Returns the number of seconds "lead time" the alarm should sound before the actual appointment.
This interprets the advance number and units. The value is always positive, 0 if no alarms are enabled.
Definition at line 303 of file pilotDateEntry.cc.
bool PilotDateEntry::doesFloat | ( | ) | const [inline] |
Is this appointment a "floating" appointment?
Floating appointments are those that have a day assigned, but no time in that day (birthday appointments are like that). You can think of these as "events", which don't have a time associated with them for a given day, as opposed to a regular "appointment", which does normally have a time associated with it.
Definition at line 107 of file pilotDateEntry.h.
QDateTime PilotDateEntry::dtAlarm | ( | ) | const [inline] |
Returns the absolute date and time that the alarm should sound for this appointment.
Definition at line 238 of file pilotDateEntry.h.
QDateTime PilotDateEntry::dtEnd | ( | ) | const |
Get the end time of this appointment.
For floating appointments, the time is undefined (perhaps 1 minute past midnight).
Floating appointments are those that have a day assigned, but no time in that day (birthday appointments are like that).
Definition at line 291 of file pilotDateEntry.cc.
QDateTime PilotDateEntry::dtRepeatEnd | ( | ) | const |
Returns the date and time that the repeat ends.
If there is no repeat, returns an invalid date and time.
Definition at line 297 of file pilotDateEntry.cc.
QDateTime PilotDateEntry::dtStart | ( | ) | const |
Get the start time of this appointment.
For floating appointments, the time is undefined (perhaps 1 minute past midnight).
Floating appointments are those that have a day assigned, but no time in that day (birthday appointments are like that).
Definition at line 285 of file pilotDateEntry.cc.
int PilotDateEntry::getAdvance | ( | ) | const [inline] |
Get the numeric part of "alarm: __ (v) minutes" on the pilot -- you set the alarm time in two parts, a number and a unit type to use; unit types are minutes, hours, days and the number is whatever you like.
If alarms are not enabled for this appointment, returns garbage.
- See also:
- alarmLeadTime()
Definition at line 206 of file pilotDateEntry.h.
int PilotDateEntry::getAdvanceUnits | ( | ) | const [inline] |
Returns the units part of the alarm time.
See getAdvance .
Definition at line 218 of file pilotDateEntry.h.
QString PilotDateEntry::getDescription | ( | ) | const |
Gets the description of the appointment.
See setDescription for meaning.
Definition at line 474 of file pilotDateEntry.cc.
const char* PilotDateEntry::getDescriptionP | ( | ) | const [inline, protected] |
Definition at line 358 of file pilotDateEntry.h.
struct tm PilotDateEntry::getEventEnd | ( | ) | const [inline, read] |
Get the end time of this appointment.
See dtEnd() for caveats.
Definition at line 157 of file pilotDateEntry.h.
struct tm* PilotDateEntry::getEventEnd_p | ( | ) | const [inline, read] |
Get a pointer to the end time of this appointment.
See dtEnd() for caveats.
Definition at line 163 of file pilotDateEntry.h.
struct tm PilotDateEntry::getEventStart | ( | ) | const [inline, read] |
Get the start time of this appointment.
See dtStart() for caveats.
Definition at line 134 of file pilotDateEntry.h.
struct tm* PilotDateEntry::getEventStart_p | ( | ) | const [inline, read] |
Get a pointer to the start time of this appointment.
See dtStart() for caveats.
Definition at line 137 of file pilotDateEntry.h.
int PilotDateEntry::getExceptionCount | ( | ) | const [inline] |
Definition at line 313 of file pilotDateEntry.h.
struct tm* PilotDateEntry::getExceptions | ( | ) | const [inline, read] |
Definition at line 322 of file pilotDateEntry.h.
QString PilotDateEntry::getLocation | ( | ) | const |
Gets the location for this appointment.
See setNote for meaning.
Definition at line 438 of file pilotDateEntry.cc.
QString PilotDateEntry::getNote | ( | ) | const |
Gets the note for this appointment.
See setNote for meaning.
Definition at line 466 of file pilotDateEntry.cc.
const char* PilotDateEntry::getNoteP | ( | ) | const [inline, protected] |
Definition at line 364 of file pilotDateEntry.h.
DayOfMonthType PilotDateEntry::getRepeatDay | ( | ) | const [inline] |
Definition at line 285 of file pilotDateEntry.h.
const int* PilotDateEntry::getRepeatDays | ( | ) | const [inline] |
Definition at line 294 of file pilotDateEntry.h.
struct tm PilotDateEntry::getRepeatEnd | ( | ) | const [inline, read] |
Definition at line 262 of file pilotDateEntry.h.
int PilotDateEntry::getRepeatForever | ( | ) | const [inline] |
Definition at line 253 of file pilotDateEntry.h.
int PilotDateEntry::getRepeatFrequency | ( | ) | const [inline] |
Definition at line 276 of file pilotDateEntry.h.
repeatTypes PilotDateEntry::getRepeatType | ( | ) | const [inline] |
Definition at line 244 of file pilotDateEntry.h.
QString PilotDateEntry::getTextRepresentation | ( | Qt::TextFormat | richText | ) |
Create a textual representation (human-readable) of this appointment.
If richText
is true, then the text representation uses qt style tags as well.
Definition at line 163 of file pilotDateEntry.cc.
bool PilotDateEntry::isAlarmEnabled | ( | ) | const [inline] |
Does this appointment have an alarm set? On the Pilot, an event may have an alarm (or not).
If it has one, it is also enabled and causes the Pilot to beep (or whatever is set in the system preferences).
Definition at line 186 of file pilotDateEntry.h.
bool PilotDateEntry::isEvent | ( | ) | const [inline] |
Is this a non-time-related event as opposed to an appointment that has a time associated with it?.
Definition at line 115 of file pilotDateEntry.h.
bool PilotDateEntry::isMultiDay | ( | ) | const [inline] |
Definition at line 370 of file pilotDateEntry.h.
PilotDateEntry & PilotDateEntry::operator= | ( | const PilotDateEntry & | e | ) |
PilotRecord * PilotDateEntry::pack | ( | ) | const |
Definition at line 328 of file pilotDateEntry.cc.
void PilotDateEntry::setAdvance | ( | int | advance | ) | [inline] |
Set the numeric part of the alarm setting.
See getAdvance for details.
Definition at line 212 of file pilotDateEntry.h.
void PilotDateEntry::setAdvanceUnits | ( | int | units | ) | [inline] |
Sets the unites part of the alarm time.
See getAdvance .
Definition at line 224 of file pilotDateEntry.h.
void PilotDateEntry::setAlarmEnabled | ( | bool | b | ) | [inline] |
void PilotDateEntry::setDescription | ( | const QString & | s | ) |
Sets the description of the appointment.
This is the short string entered in the day view on the handheld, and it is called the summary in libkcal.
Definition at line 460 of file pilotDateEntry.cc.
void PilotDateEntry::setDescriptionP | ( | const char * | desc, | |
int | l = -1 | |||
) | [protected] |
Definition at line 356 of file pilotDateEntry.cc.
void PilotDateEntry::setEventEnd | ( | struct tm & | end | ) | [inline] |
void PilotDateEntry::setEventStart | ( | struct tm & | start | ) | [inline] |
void PilotDateEntry::setExceptionCount | ( | int | e | ) | [inline] |
Definition at line 317 of file pilotDateEntry.h.
void PilotDateEntry::setExceptions | ( | struct tm * | e | ) |
Definition at line 347 of file pilotDateEntry.cc.
void PilotDateEntry::setFloats | ( | bool | f | ) | [inline] |
Sets this appointment's floating status.
Floating appointments are those that have a day assigned, but no time in that day (birthday appointments are like that). You can think of these as "events", which don't have a time associated with them for a given day, as opposed to a regular "appointment", which does normally have a time associated with it.
Definition at line 128 of file pilotDateEntry.h.
void PilotDateEntry::setLocation | ( | const QString & | s | ) |
Sets the location for the appointment.
For now it will be placed within the notes on the handheld. It will be placed on one line and starts with: Location: {location}. Everything on that line will be counted as location. TODO: Make distinguish between handhelds that support the location field and the ones that don't. (Shouldn't this be done in the pilot-link lib?)
Definition at line 411 of file pilotDateEntry.cc.
void PilotDateEntry::setNote | ( | const QString & | s | ) |
Sets the note for the appointment.
The note is the long text entry that is possible - but clumsy - on the handheld. It is called the description in libkcal.
Definition at line 405 of file pilotDateEntry.cc.
void PilotDateEntry::setNoteP | ( | const char * | note, | |
int | l = -1 | |||
) | [protected] |
Definition at line 381 of file pilotDateEntry.cc.
void PilotDateEntry::setRepeatDay | ( | DayOfMonthType | rd | ) | [inline] |
Definition at line 289 of file pilotDateEntry.h.
void PilotDateEntry::setRepeatDays | ( | QBitArray | rba | ) | [inline] |
Definition at line 305 of file pilotDateEntry.h.
void PilotDateEntry::setRepeatDays | ( | int * | rd | ) | [inline] |
Definition at line 298 of file pilotDateEntry.h.
void PilotDateEntry::setRepeatEnd | ( | struct tm | tm | ) | [inline] |
Definition at line 266 of file pilotDateEntry.h.
void PilotDateEntry::setRepeatForever | ( | int | f = 1 |
) | [inline] |
Definition at line 257 of file pilotDateEntry.h.
void PilotDateEntry::setRepeatFrequency | ( | int | f | ) | [inline] |
Definition at line 280 of file pilotDateEntry.h.
void PilotDateEntry::setRepeatType | ( | repeatTypes | r | ) | [inline] |
Definition at line 248 of file pilotDateEntry.h.
The documentation for this class was generated from the following files: