kpilot
PilotTodoEntry Class Reference
A decoded ToDo item. More...
#include <pilotTodoEntry.h>
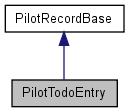
Public Member Functions | |
QString | getCategoryLabel () const |
int | getComplete () const |
QString | getDescription () const |
struct tm | getDueDate () const |
int | getIndefinite () const |
QString | getNote () const |
int | getPriority () const |
QString | getTextRepresentation (Qt::TextFormat richText) |
PilotTodoEntry & | operator= (const PilotTodoEntry &e) |
PilotRecord * | pack () const |
PilotTodoEntry (const PilotTodoEntry &e) | |
PilotTodoEntry (PilotRecord *rec) | |
PilotTodoEntry () | |
void | setComplete (int c) |
void | setDescription (const QString &) |
void | setDueDate (struct tm &d) |
void | setIndefinite (int i) |
void | setNote (const QString ¬e) |
void | setPriority (int p) |
~PilotTodoEntry () | |
Protected Member Functions | |
const char * | getDescriptionP () const |
const char * | getNoteP () const |
void | setDescriptionP (const char *, int len=-1) |
void | setNoteP (const char *, int len=-1) |
Detailed Description
A decoded ToDo item.
Definition at line 49 of file pilotTodoEntry.h.
Constructor & Destructor Documentation
PilotTodoEntry::PilotTodoEntry | ( | ) |
PilotTodoEntry::PilotTodoEntry | ( | PilotRecord * | rec | ) |
Constructor.
Create a ToDo item and fill it with data from the uninterpreted record rec
. The record may be NULL, in which case the todo is empty and its category and ID are zero, as in the constructor above.
Definition at line 52 of file pilotTodoEntry.cc.
PilotTodoEntry::PilotTodoEntry | ( | const PilotTodoEntry & | e | ) |
PilotTodoEntry::~PilotTodoEntry | ( | ) | [inline] |
Member Function Documentation
QString PilotTodoEntry::getCategoryLabel | ( | ) | const |
Returns the label for the category this ToDo item is in.
int PilotTodoEntry::getComplete | ( | ) | const [inline] |
Return whether the ToDo is complete (done, finished) or not.
Definition at line 120 of file pilotTodoEntry.h.
QString PilotTodoEntry::getDescription | ( | ) | const |
Get the ToDo item's description (which is the title shown on the handheld, and the item's Title in KDE).
This uses the default codec.
Definition at line 221 of file pilotTodoEntry.cc.
const char* PilotTodoEntry::getDescriptionP | ( | ) | const [inline, protected] |
Definition at line 152 of file pilotTodoEntry.h.
struct tm PilotTodoEntry::getDueDate | ( | ) | const [inline, read] |
int PilotTodoEntry::getIndefinite | ( | ) | const [inline] |
Return the indefinite status of the ToDo (? that is, whether it had a Due Date that is relevant or not).
Return values are 0 (not indefinite) or non-0.
Definition at line 93 of file pilotTodoEntry.h.
QString PilotTodoEntry::getNote | ( | ) | const |
Get the ToDo item's note (the longer text, not immediately accessible on the handheld).
This uses the default codec.
Definition at line 266 of file pilotTodoEntry.cc.
const char* PilotTodoEntry::getNoteP | ( | ) | const [inline, protected] |
Definition at line 154 of file pilotTodoEntry.h.
int PilotTodoEntry::getPriority | ( | ) | const [inline] |
Return the priority of the ToDo item.
The priority ranges from 1-5 on the handheld, so this needs to be mapped (perhaps) onto KOrganizer's priority levels.
Definition at line 108 of file pilotTodoEntry.h.
QString PilotTodoEntry::getTextRepresentation | ( | Qt::TextFormat | richText | ) |
Return a string for the ToDo item.
If
- Parameters:
-
richText is true, then use qt style markup to make the string clearer when displayed.
Definition at line 118 of file pilotTodoEntry.cc.
PilotTodoEntry & PilotTodoEntry::operator= | ( | const PilotTodoEntry & | e | ) |
PilotRecord * PilotTodoEntry::pack | ( | ) | const |
Definition at line 166 of file pilotTodoEntry.cc.
void PilotTodoEntry::setComplete | ( | int | c | ) | [inline] |
void PilotTodoEntry::setDescription | ( | const QString & | desc | ) |
void PilotTodoEntry::setDescriptionP | ( | const char * | desc, | |
int | len = -1 | |||
) | [protected] |
Definition at line 192 of file pilotTodoEntry.cc.
void PilotTodoEntry::setDueDate | ( | struct tm & | d | ) | [inline] |
void PilotTodoEntry::setIndefinite | ( | int | i | ) | [inline] |
void PilotTodoEntry::setNote | ( | const QString & | note | ) |
void PilotTodoEntry::setNoteP | ( | const char * | note, | |
int | len = -1 | |||
) | [protected] |
Definition at line 238 of file pilotTodoEntry.cc.
void PilotTodoEntry::setPriority | ( | int | p | ) | [inline] |
The documentation for this class was generated from the following files: