libkonq
#include <konq_operations.h>
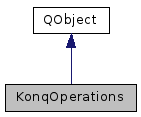
Public Types | |
enum | ConfirmationType { DEFAULT_CONFIRMATION, SKIP_CONFIRMATION, FORCE_CONFIRMATION } |
enum | NewDirFlag { ViewShowsHiddenFile = 1 } |
enum | Operation { TRASH, DEL, COPY, MOVE, LINK, EMPTYTRASH, STAT, MKDIR, RESTORE, UNKNOWN, PUT, RENAME } |
Signals | |
void | aboutToCreate (const QPoint &pos, const QList< KIO::CopyInfo > &files) |
void | aboutToCreate (const KUrl::List &urls) |
void | renamingFailed (const KUrl &oldUrl, const KUrl &newUrl) |
void | statFinished (const KFileItem &item) |
Public Member Functions | |
KUrl::List | droppedUrls () const |
QPoint | dropPosition () const |
Static Public Member Functions | |
static bool | askDeleteConfirmation (const KUrl::List &selectedUrls, int method, ConfirmationType confirmation, QWidget *widget) |
static void | copy (QWidget *parent, Operation method, const KUrl::List &selectedUrls, const KUrl &destUrl) |
static void | del (QWidget *parent, Operation method, const KUrl::List &selectedUrls) |
static void | doDrop (const KFileItem &destItem, const KUrl &destUrl, QDropEvent *ev, QWidget *parent) |
static KonqOperations * | doDrop (const KFileItem &destItem, const KUrl &destUrl, QDropEvent *ev, QWidget *parent, const QList< QAction * > &userActions) |
static void | doPaste (QWidget *parent, const KUrl &destUrl, const QPoint &pos=QPoint()) |
static KonqOperations * | doPasteV2 (QWidget *parent, const KUrl &destUrl, const QPoint &pos=QPoint()) |
static void | editMimeType (const QString &mimeType, QWidget *parent) |
static void | emptyTrash (QWidget *parent) |
static KIO::SimpleJob * | mkdir (QWidget *parent, const KUrl &url) |
static KIO::SimpleJob * | newDir (QWidget *parent, const KUrl &baseUrl) |
static KIO::SimpleJob * | newDir (QWidget *parent, const KUrl &baseUrl, NewDirFlags flags) |
static QPair< bool, QString > | pasteInfo (const KUrl &targetUrl) |
static void | rename (QWidget *parent, const KUrl &oldurl, const QString &name) |
static void | rename (QWidget *parent, const KUrl &oldurl, const KUrl &newurl) |
static KonqOperations * | renameV2 (QWidget *parent, const KUrl &oldurl, const QString &name) |
static KonqOperations * | renameV2 (QWidget *parent, const KUrl &oldurl, const KUrl &newurl) |
static void | restoreTrashedItems (const KUrl::List &urls, QWidget *parent) |
static void | statUrl (const KUrl &url, const QObject *receiver, const char *member, QWidget *parent) |
Protected Slots | |
void | asyncDrop (const KFileItem &item) |
void | doDropFileCopy () |
void | slotAboutToCreate (KIO::Job *job, const QList< KIO::CopyInfo > &files) |
void | slotCopyingDone (KIO::Job *job, const KUrl &from, const KUrl &to) |
void | slotCopyingLinkDone (KIO::Job *job, const KUrl &from, const QString &target, const KUrl &to) |
void | slotResult (KJob *job) |
void | slotStatResult (KJob *job) |
Protected Member Functions | |
KonqOperations (QWidget *parent) | |
virtual | ~KonqOperations () |
Detailed Description
Implements file operations (move,del,trash,paste,copy,move,link...) for file managers.
Definition at line 42 of file konq_operations.h.
Member Enumeration Documentation
Enumerator | |
---|---|
DEFAULT_CONFIRMATION | |
SKIP_CONFIRMATION | |
FORCE_CONFIRMATION |
Definition at line 229 of file konq_operations.h.
Enumerator | |
---|---|
ViewShowsHiddenFile |
Definition at line 162 of file konq_operations.h.
Enumerator | |
---|---|
TRASH | |
DEL | |
COPY | |
MOVE | |
LINK | |
EMPTYTRASH | |
STAT | |
MKDIR | |
RESTORE | |
UNKNOWN | |
PUT | |
RENAME |
Definition at line 56 of file konq_operations.h.
Constructor & Destructor Documentation
|
protected |
Definition at line 79 of file konq_operations.cpp.
|
protectedvirtual |
Definition at line 86 of file konq_operations.cpp.
Member Function Documentation
|
signal |
|
signal |
|
static |
Ask for confirmation before deleting/trashing selectedUrls
.
- Parameters
-
selectedUrls the urls about to be deleted method the type of deletion (DEL for real deletion, anything else for trash) confirmation default (based on config file), skip (no confirmation) or force (always confirm) widget parent widget for message boxes
- Returns
- true if confirmed
Definition at line 264 of file konq_operations.cpp.
|
protectedslot |
Definition at line 368 of file konq_operations.cpp.
|
static |
Copy the selectedUrls
to the destination destUrl
.
- Parameters
-
parent parent widget (for error dialog box if any) method should be COPY, MOVE or LINK selectedUrls the URLs to copy destUrl destination of the copy
- Todo:
- document restrictions on the kind of destination
Definition at line 171 of file konq_operations.cpp.
|
static |
Delete the selectedUrls
if possible.
- Parameters
-
parent parent widget (for error dialog box if any) method should be TRASH or DEL selectedUrls the URLs to be deleted
Definition at line 101 of file konq_operations.cpp.
|
static |
Drop.
- Parameters
-
destItem destination KFileItem for the drop (background or item) destUrl destination URL for the drop. ev the drop event parent parent widget (for error dialog box if any)
If destItem is 0L, doDrop will stat the URL to determine it.
Definition at line 285 of file konq_operations.cpp.
|
static |
Drop.
- Parameters
-
destItem destination KFileItem for the drop (background or item) destUrl destination URL for the drop. ev the drop event parent parent widget (for error dialog box if any) userActions additional actions to include in the drop menu
This is an overloaded member function that lets you add your own actions to the drop menu shown by KonqOperations.
The drop menu will be shown when the application re-enters the event loop.
If destItem is 0L, doDrop will stat the URL to determine it.
Note that the returned KonqOperations object will be deleted automatically when the drop is completed.
It is still valid when a slot connected to a triggered() signal in one of the user actions is invoked, but should not be assumed to be valid after the slot returns.
- Returns
- The KonqOperations object
- Since
- 4.3
Definition at line 290 of file konq_operations.cpp.
|
protectedslot |
Definition at line 461 of file konq_operations.cpp.
|
static |
Paste the clipboard contents.
Definition at line 136 of file konq_operations.cpp.
|
static |
Paste the clipboard contents.
- Returns
- The KonqOperations object
- Since
- 4.10
- Todo:
- TODO KDE 5,0 - Merge doPaste and doPasteV2
Definition at line 141 of file konq_operations.cpp.
KUrl::List KonqOperations::droppedUrls | ( | ) | const |
Returns the list of dropped URL's.
You can call this method on the object returned by KonqOperations::doDrop(), to obtain the list of URL's this object handles.
- Since
- 4.3
Definition at line 451 of file konq_operations.cpp.
QPoint KonqOperations::dropPosition | ( | ) | const |
Returns the position where the drop occurred.
- Since
- 4.3
Definition at line 456 of file konq_operations.cpp.
|
static |
Pop up properties dialog for mimetype mimeType
.
- Parameters
-
parent parent widget (for dialogs)
Definition at line 92 of file konq_operations.cpp.
|
static |
Empty the trash.
Definition at line 115 of file konq_operations.cpp.
|
static |
Create a directory.
Same as KIO::mkdir but records job into KonqFileUndoManager for undo/redo purposes.
Definition at line 127 of file konq_operations.cpp.
|
static |
Ask for the name of a new directory and create it.
Calls KonqOperations::mkdir.
- Parameters
-
parent the parent widget baseUrl the directory to create the new directory in
- Returns
- the job used to create the directory or 0 if the creation was cancelled by the user
Definition at line 922 of file konq_operations.cpp.
|
static |
Ask for the name of a new directory and create it.
Calls KonqOperations::mkdir.
- Parameters
-
parent the parent widget baseUrl the directory to create the new directory in flags see NewDirFlags
- Returns
- the job used to create the directory or 0 if the creation was cancelled by the user
Definition at line 927 of file konq_operations.cpp.
|
static |
Returns the state of the paste action: first is whether the action should be enabled second is the text for the action.
- Since
- 4.3
Definition at line 1028 of file konq_operations.cpp.
|
static |
Do a renaming.
- Parameters
-
parent the parent widget, passed to KonqOperations ctor oldurl the current url of the file to be renamed name the new name for the file. Shouldn't include '/'.
Definition at line 893 of file konq_operations.cpp.
|
static |
Do a renaming.
- Parameters
-
parent the parent widget, passed to KonqOperations ctor oldurl the current url of the file to be renamed newurl the new url for the file Use this version if the other one wouldn't work :) (e.g. because name could be a relative path, including a '/').
Definition at line 754 of file konq_operations.cpp.
|
static |
Do a renaming.
- Parameters
-
parent the parent widget, passed to KonqOperations ctor oldurl the current url of the file to be renamed name the new name for the file. Shouldn't include '/'.
- Returns
- The KonqOperations object
- Since
- 4.11
- Todo:
- TODO KDE 5.0 - Merge rename and renameV2
Definition at line 898 of file konq_operations.cpp.
|
static |
Do a renaming.
- Parameters
-
parent the parent widget, passed to KonqOperations ctor oldurl the current url of the file to be renamed newurl the new url for the file Use this version if the other one wouldn't work :) (e.g. because name could be a relative path, including a '/').
- Returns
- The KonqOperations object
- Since
- 4.11
- Todo:
- TODO KDE 5.0 - Merge rename and renameV2
Definition at line 759 of file konq_operations.cpp.
|
signal |
|
static |
Restore trashed items.
Definition at line 121 of file konq_operations.cpp.
|
protectedslot |
Definition at line 812 of file konq_operations.cpp.
|
protectedslot |
Definition at line 684 of file konq_operations.cpp.
|
protectedslot |
Definition at line 689 of file konq_operations.cpp.
|
protectedslot |
Definition at line 850 of file konq_operations.cpp.
|
protectedslot |
Definition at line 833 of file konq_operations.cpp.
|
signal |
|
static |
Get info about a given URL, and when that's done (it's asynchronous!), call a given slot with a const KFileItem& as argument.
The KFileItem will be deleted by statUrl after calling the slot. Make a copy if you need one !
Definition at line 817 of file konq_operations.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.