Konsole
#include <ProcessInfo.h>
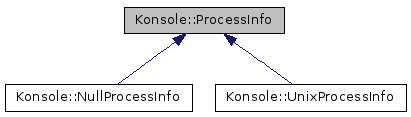
Public Types | |
enum | Error { NoError, UnknownError, PermissionsError } |
Public Member Functions | |
virtual | ~ProcessInfo () |
QVector< QString > | arguments (bool *ok) const |
QString | currentDir (bool *ok) const |
QMap< QString, QString > | environment (bool *ok) const |
Error | error () const |
int | foregroundPid (bool *ok) const |
QString | format (const QString &text) const |
bool | isValid () const |
QString | name (bool *ok) const |
int | parentPid (bool *ok) const |
int | pid (bool *ok) const |
void | setUserHomeDir () |
void | update () |
QString | userHomeDir () const |
int | userId (bool *ok) const |
QString | userName () const |
QString | validCurrentDir () const |
Static Public Member Functions | |
static QString | localHost () |
static ProcessInfo * | newInstance (int pid, bool readEnvironment=false) |
Protected Member Functions | |
ProcessInfo (int pid, bool readEnvironment=false) | |
void | addArgument (const QString &argument) |
void | addEnvironmentBinding (const QString &name, const QString &value) |
void | clearArguments () |
virtual bool | readProcessInfo (int pid, bool readEnvironment)=0 |
virtual void | readUserName (void)=0 |
void | setCurrentDir (const QString &dir) |
void | setError (Error error) |
void | setFileError (QFile::FileError error) |
void | setForegroundPid (int pid) |
void | setName (const QString &name) |
void | setParentPid (int pid) |
void | setPid (int pid) |
void | setUserId (int uid) |
void | setUserName (const QString &name) |
Detailed Description
Takes a snapshot of the state of a process and provides access to information such as the process name, parent process, the foreground process in the controlling terminal, the arguments with which the process was started and the environment.
To create a new snapshot, construct a new ProcessInfo instance, using ProcessInfo::newInstance(), passing the process identifier of the process you are interested in.
After creating a new instance, call the update() method to take a snapshot of the current state of the process.
Before calling any additional methods, check that the process state was read successfully using the isValid() method.
Each accessor method which provides information about the process state ( such as pid(), currentDir(), name() ) takes a pointer to a boolean as an argument. If the information requested was read successfully then the boolean is set to true, otherwise it is set to false, in which case the return value from the function should be ignored. If this boolean is set to false, it may indicate an error reading the process information, or it may indicate that the information is not available on the current platform.
eg.
Definition at line 74 of file ProcessInfo.h.
Member Enumeration Documentation
This enum describes the errors which can occur when trying to read a process's information.
Enumerator | |
---|---|
NoError |
No error occurred. |
UnknownError |
The nature of the error is unknown. |
PermissionsError |
Konsole does not have permission to obtain the process information. |
Definition at line 197 of file ProcessInfo.h.
Constructor & Destructor Documentation
|
inlinevirtual |
Definition at line 90 of file ProcessInfo.h.
|
explicitprotected |
Constructs a new process instance.
You should not call the constructor of ProcessInfo or its subclasses directly. Instead use the static ProcessInfo::newInstance() method which will return a suitable ProcessInfo instance for the current platform.
Definition at line 68 of file ProcessInfo.cpp.
Member Function Documentation
|
protected |
Adds a commandline argument for the process, as returned by arguments()
Definition at line 318 of file ProcessInfo.cpp.
|
protected |
Adds an environment binding for the process, as returned by environment()
- Parameters
-
name The name of the environment variable, eg. "PATH" value The value of the environment variable, eg. "/bin"
Definition at line 328 of file ProcessInfo.cpp.
QVector< QString > ProcessInfo::arguments | ( | bool * | ok | ) | const |
Returns the command-line arguments which the process was started with.
The first argument is the name used to launch the process.
- Parameters
-
ok Set to true if the arguments were read successfully or false otherwise.
Definition at line 193 of file ProcessInfo.cpp.
|
protected |
clear the commandline arguments for the process, as returned by arguments()
Definition at line 323 of file ProcessInfo.cpp.
QString ProcessInfo::currentDir | ( | bool * | ok | ) | const |
Returns the current working directory of the process.
- Parameters
-
ok Set to true if the current working directory was read successfully or false otherwise
Definition at line 300 of file ProcessInfo.cpp.
QMap< QString, QString > ProcessInfo::environment | ( | bool * | ok | ) | const |
Returns the environment bindings which the process was started with.
In the returned map, the key is the name of the environment variable, and the value is the corresponding value.
- Parameters
-
ok Set to true if the environment bindings were read successfully or false otherwise
Definition at line 200 of file ProcessInfo.cpp.
ProcessInfo::Error ProcessInfo::error | ( | ) | const |
Returns the last error which occurred.
Definition at line 87 of file ProcessInfo.cpp.
int ProcessInfo::foregroundPid | ( | bool * | ok | ) | const |
Returns the id of the current foreground process.
NOTE: Using the foregroundProcessGroup() method of the Pty instance associated with the terminal of interest is preferred over using this method.
- Parameters
-
ok Set to true if the foreground process id was read successfully or false otherwise
Definition at line 226 of file ProcessInfo.cpp.
QString ProcessInfo::format | ( | const QString & | text | ) | const |
Parses an input string, looking for markers beginning with a '' character and returns a string with the markers replaced with information from this process description.
The markers recognized are:
- u - Name of the user which owns the process.
- n - Replaced with the name of the process.
-
d - Replaced with the last part of the path name of the process' current working directory.
(eg. if the current directory is '/home/bob' then 'bob' would be returned)
- D - Replaced with the current working directory of the process.
Definition at line 120 of file ProcessInfo.cpp.
bool ProcessInfo::isValid | ( | ) | const |
Returns true if the process state was read successfully.
Definition at line 207 of file ProcessInfo.cpp.
|
static |
Returns the local host.
Definition at line 257 of file ProcessInfo.cpp.
QString ProcessInfo::name | ( | bool * | ok | ) | const |
Returns the name of the current process.
Definition at line 233 of file ProcessInfo.cpp.
|
static |
Constructs a new instance of a suitable ProcessInfo sub-class for the current platform which provides information about a given process.
- Parameters
-
pid The pid of the process to examine readEnvironment Specifies whether environment bindings should be read. If this is false, then environment() calls will always fail. This is an optimization to avoid the overhead of reading the (potentially large) environment data when it is not required.
Definition at line 1165 of file ProcessInfo.cpp.
int ProcessInfo::parentPid | ( | bool * | ok | ) | const |
Returns the id of the parent process id was read successfully or false otherwise.
- Parameters
-
ok Set to true if the parent process id
Definition at line 219 of file ProcessInfo.cpp.
int ProcessInfo::pid | ( | bool * | ok | ) | const |
Returns the process id.
- Parameters
-
ok Set to true if the process id was read successfully or false otherwise
Definition at line 212 of file ProcessInfo.cpp.
|
protectedpure virtual |
This is called on construction to read the process state Subclasses should reimplement this function to provide platform-specific process state reading functionality.
When called, readProcessInfo() should attempt to read all of the necessary state information. If the attempt is successful, it should set the process id using setPid(), and update the other relevant information using setParentPid(), setName(), setArguments() etc.
Calls to isValid() will return true only if the process id has been set using setPid()
- Parameters
-
pid The process id of the process to read readEnvironment Specifies whether the environment bindings for the process should be read
Implemented in Konsole::UnixProcessInfo, and Konsole::NullProcessInfo.
|
protectedpure virtual |
Implemented in Konsole::UnixProcessInfo, and Konsole::NullProcessInfo.
|
protected |
Sets the current working directory for the process.
Definition at line 307 of file ProcessInfo.cpp.
|
protected |
Sets the error.
Definition at line 91 of file ProcessInfo.cpp.
|
protected |
Convenience method.
Sets the error based on a QFile error code.
Definition at line 333 of file ProcessInfo.cpp.
|
protected |
Sets the foreground process id as returned by foregroundPid()
Definition at line 294 of file ProcessInfo.cpp.
|
protected |
Sets the name of the process as returned by name()
Definition at line 313 of file ProcessInfo.cpp.
|
protected |
Sets the parent process id as returned by parentPid()
Definition at line 289 of file ProcessInfo.cpp.
|
protected |
Sets the process id associated with this ProcessInfo instance.
Definition at line 262 of file ProcessInfo.cpp.
void ProcessInfo::setUserHomeDir | ( | ) |
Forces the user home directory to be calculated.
Definition at line 280 of file ProcessInfo.cpp.
|
protected |
Sets the user id associated with this ProcessInfo instance.
Definition at line 268 of file ProcessInfo.cpp.
|
protected |
Sets the user name of the process as set by readUserName()
Definition at line 274 of file ProcessInfo.cpp.
void ProcessInfo::update | ( | ) |
Updates the information about the process.
This must be called before attempting to use any of the accessor methods.
Definition at line 96 of file ProcessInfo.cpp.
QString ProcessInfo::userHomeDir | ( | ) | const |
Returns the user's home directory of the process.
Definition at line 252 of file ProcessInfo.cpp.
int ProcessInfo::userId | ( | bool * | ok | ) | const |
Definition at line 240 of file ProcessInfo.cpp.
QString ProcessInfo::userName | ( | ) | const |
Returns the user's name of the process.
Definition at line 247 of file ProcessInfo.cpp.
QString ProcessInfo::validCurrentDir | ( | ) | const |
Returns the current working directory of the process (or its parent)
Definition at line 101 of file ProcessInfo.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:24 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.