Konsole
#include <Profile.h>
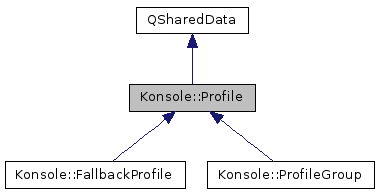
Public Member Functions | |
Profile (Ptr parent=Ptr()) | |
virtual | ~Profile () |
bool | antiAliasFonts () const |
QStringList | arguments () const |
const GroupPtr | asGroup () const |
GroupPtr | asGroup () |
bool | autoCopySelectedText () const |
bool | bidiRenderingEnabled () const |
bool | blinkingCursorEnabled () const |
bool | blinkingTextEnabled () const |
bool | boldIntense () const |
void | clone (Ptr profile, bool differentOnly=true) |
QString | colorScheme () const |
QString | command () const |
QColor | customCursorColor () const |
QString | defaultEncoding () const |
QString | defaultWorkingDirectory () const |
QStringList | environment () const |
bool | flowControlEnabled () const |
QFont | font () const |
int | historySize () const |
QString | icon () const |
bool | isEmpty () const |
bool | isHidden () const |
virtual bool | isPropertySet (Property property) const |
QString | keyBindings () const |
int | lineSpacing () const |
QString | localTabTitleFormat () const |
QString | menuIndex () const |
int | menuIndexAsInt () const |
bool | mouseWheelZoomEnabled () const |
QString | name () const |
const Ptr | parent () const |
QString | path () const |
const QStringList | propertiesInfoList () const |
template<class T > | |
T | property (Property property) const |
template<> | |
QVariant | property (Property aProperty) const |
QString | remoteTabTitleFormat () const |
bool | saveGeometryOnExit () const |
void | setHidden (bool hidden) |
void | setParent (Ptr parent) |
virtual QHash< Property, QVariant > | setProperties () const |
virtual void | setProperty (Property property, const QVariant &value) |
bool | showTerminalSizeHint () const |
int | silenceSeconds () const |
bool | startInCurrentSessionDir () const |
bool | underlineLinksEnabled () const |
QString | untranslatedName () const |
bool | useCustomCursorColor () const |
QString | wordCharacters () const |
Static Public Member Functions | |
static Property | lookupByName (const QString &name) |
Detailed Description
Represents a terminal set-up which can be used to set the initial state of new terminal sessions or applied to existing sessions.
Profiles consist of a number of named properties, which can be retrieved using property() and set using setProperty(). isPropertySet() can be used to check whether a particular property has been set in a profile.
Profiles support a simple form of inheritance. When a new Profile is constructed, a pointer to a parent profile can be passed to the constructor. When querying a particular property of a profile using property(), the profile will return its own value for that property if one has been set or otherwise it will return the parent's value for that property.
Profiles can be loaded from disk using ProfileReader instances and saved to disk using ProfileWriter instances.
Member Typedef Documentation
typedef KSharedPtr<ProfileGroup> Konsole::Profile::GroupPtr |
typedef KSharedPtr<Profile> Konsole::Profile::Ptr |
Member Enumeration Documentation
This enum describes the available properties which a Profile may consist of.
Properties can be set using setProperty() and read using property()
Enumerator | |
---|---|
Path |
(QString) Path to the profile's configuration file on-disk. |
Name |
(QString) The descriptive name of this profile. |
UntranslatedName |
(QString) The untranslated name of this profile. Warning: this is an internal property. Do not touch it. |
Icon |
(QString) The name of the icon associated with this profile. This is used in menus and tabs to represent the profile. |
Command |
(QString) The command to execute ( excluding arguments ) when creating a new terminal session using this profile. |
Arguments |
(QStringList) The arguments which are passed to the program specified by the Command property when creating a new terminal session using this profile. |
Environment |
(QStringList) Additional environment variables (in the form of NAME=VALUE pairs) which are passed to the program specified by the Command property when creating a new terminal session using this profile. |
Directory |
(QString) The initial working directory for sessions created using this profile. |
LocalTabTitleFormat |
(QString) The format used for tab titles when running normal commands. |
RemoteTabTitleFormat |
(QString) The format used for tab titles when the session is running a remote command (eg. SSH) |
ShowTerminalSizeHint |
(bool) Specifies whether show hint for terminal size after resizing the application window. |
SaveGeometryOnExit |
(bool) Specifies whether the geometry information is saved when window is closed. |
Font |
(QFont) The font to use in terminal displays using this profile. |
ColorScheme |
(QString) The name of the color scheme to use in terminal displays using this profile. Color schemes are managed by the ColorSchemeManager class. |
KeyBindings |
(QString) The name of the key bindings. Key bindings are managed by the KeyboardTranslatorManager class. |
HistoryMode |
(HistoryModeEnum) Specifies the storage type used for keeping the output produced by terminal sessions using this profile. |
HistorySize |
(int) Specifies the number of lines of output to remember in terminal sessions using this profile. Once the limit is reached, the oldest lines are lost if the HistoryMode property is FixedSizeHistory |
ScrollBarPosition |
(ScrollBarPositionEnum) Specifies the position of the scroll bar in terminal displays using this profile. |
ScrollFullPage |
(bool) Specifies whether the PageUp/Down will scroll the full height or half height. |
BidiRenderingEnabled |
(bool) Specifies whether the terminal will enable Bidirectional text display |
BlinkingTextEnabled |
(bool) Specifies whether text in terminal displays is allowed to blink. |
FlowControlEnabled |
(bool) Specifies whether the flow control keys (typically Ctrl+S, Ctrl+Q) have any effect. Also known as Xon/Xoff |
LineSpacing |
(int) Specifies the pixels between the terminal lines. |
BlinkingCursorEnabled |
(bool) Specifies whether the cursor blinks ( in a manner similar to text editing applications ) |
UseCustomCursorColor |
(bool) If true, terminal displays use a fixed color to draw the cursor, specified by the CustomCursorColor property. Otherwise the cursor changes color to match the character underneath it. |
CursorShape |
(CursorShapeEnum) The shape used by terminal displays to represent the cursor. |
CustomCursorColor |
(QColor) The color used by terminal displays to draw the cursor. Only applicable if the UseCustomCursorColor property is true. |
WordCharacters |
(QString) A string consisting of the characters used to delimit words when selecting text in the terminal display. |
TripleClickMode |
(TripleClickModeEnum) Specifies which part of current line should be selected with triple click action. |
UnderlineLinksEnabled |
(bool) If true, text that matches a link or an email address is underlined when hovered by the mouse pointer. |
OpenLinksByDirectClickEnabled |
(bool) If true, links can be opened by direct mouse click. |
CtrlRequiredForDrag |
(bool) If true, control key must be pressed to click and drag selected text. |
AutoCopySelectedText |
(bool) If true, automatically copy selected text into the clipboard |
TrimTrailingSpacesInSelectedText |
(bool) If true, trailing spaces are trimmed in selected text |
PasteFromSelectionEnabled |
(bool) If true, middle mouse button pastes from X Selection |
PasteFromClipboardEnabled |
(bool) If true, middle mouse button pastes from Clipboard |
MiddleClickPasteMode |
(MiddleClickPasteModeEnum) Specifies the source from which mouse middle click pastes data. |
DefaultEncoding |
(String) Default text codec |
AntiAliasFonts |
(bool) Whether fonts should be aliased or not |
BoldIntense |
(bool) Whether character with intense colors should be rendered in bold font or just in bright color. |
StartInCurrentSessionDir |
(bool) Whether new sessions should be started in the same directory as the currently active session. |
SilenceSeconds |
(int) Specifies the threshold of detected silence in seconds. |
BellMode |
(BellModeEnum) Specifies the behavior of bell. |
TerminalColumns |
(int) Specifies the preferred columns. |
TerminalRows |
(int) Specifies the preferred rows. |
MenuIndex |
Index of profile in the File Menu WARNING: this is currently an internal field, which is expected to be zero on disk. Do not modify it manually. In future, the format might be #.#.# to account for levels |
MouseWheelZoomEnabled |
(bool) If true, mouse wheel scroll with Ctrl key pressed increases/decreases the terminal font size. |
Constructor & Destructor Documentation
|
explicit |
Constructs a new profile.
- Parameters
-
parent The parent profile. When querying the value of a property using property(), if the property has not been set in this profile then the parent's value for the property will be returned.
Definition at line 205 of file Profile.cpp.
|
virtual |
Definition at line 229 of file Profile.cpp.
Member Function Documentation
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
void Profile::clone | ( | Profile::Ptr | profile, |
bool | differentOnly = true |
||
) |
Copies all properties except Name and Path from the specified profile
into this profile.
- Parameters
-
profile The profile to copy properties from differentOnly If true, only properties in profile
which have a different value from this profile's current value (either set via setProperty() or inherited from the parent profile) will be set.
Definition at line 210 of file Profile.cpp.
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
bool Profile::isEmpty | ( | ) | const |
Returns true if no properties have been set in this Profile instance.
Definition at line 250 of file Profile.cpp.
bool Profile::isHidden | ( | ) | const |
Returns true if this is a 'hidden' profile which should not be displayed in menus or saved to disk.
This is used for the fallback profile, in case there are no profiles on disk which can be loaded, or for overlay profiles created to handle command-line arguments which change profile properties.
Definition at line 232 of file Profile.cpp.
|
virtual |
Returns true if the specified property has been set in this Profile instance.
Definition at line 262 of file Profile.cpp.
|
inline |
|
inline |
|
inline |
|
static |
Returns the element from the Property enum associated with the specified name
.
- Parameters
-
name The name of the property to look for, this is case insensitive.
Definition at line 267 of file Profile.cpp.
|
inline |
int Profile::menuIndexAsInt | ( | ) | const |
Definition at line 285 of file Profile.cpp.
|
inline |
|
inline |
const Profile::Ptr Profile::parent | ( | ) | const |
Returns the parent profile.
Definition at line 245 of file Profile.cpp.
|
inline |
const QStringList Profile::propertiesInfoList | ( | ) | const |
Return a list of all properties names and their type (for use with -p option).
Definition at line 295 of file Profile.cpp.
|
inline |
Returns the current value of the specified property
, cast to type T.
Internally properties are stored using the QVariant type and cast to T using QVariant::value<T>();
If the specified property
has not been set in this profile, and a non-null parent was specified in the Profile's constructor, the parent's value for property
will be returned.
|
inline |
|
inline |
|
inline |
void Profile::setHidden | ( | bool | hidden | ) |
Specifies whether this is a hidden profile.
See isHidden()
Definition at line 236 of file Profile.cpp.
void Profile::setParent | ( | Profile::Ptr | parent | ) |
Changes the parent profile.
When calling the property() method, if the specified property has not been set for this profile, the parent's value for the property will be returned instead.
Definition at line 241 of file Profile.cpp.
|
virtual |
Returns a map of the properties set in this Profile instance.
Definition at line 254 of file Profile.cpp.
|
virtual |
Sets the value of the specified property
to value
.
Reimplemented in Konsole::ProfileGroup.
Definition at line 258 of file Profile.cpp.
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:25 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.