libs/libkipi/libkipi
#include <interface.h>
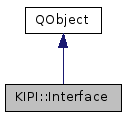
Signals | |
void | currentAlbumChanged (bool hasSelection) |
void | gotThumbnail (const KUrl &, const QPixmap &) |
void | progressCanceled (const QString &id) |
void | reservationCleared (const KUrl &url) |
void | reservedForAction (const KUrl &url, const QString &descriptionOfAction) |
void | selectionChanged (bool hasSelection) |
Public Member Functions | |
Interface (QObject *const parent, const char *name=0) | |
virtual | ~Interface () |
virtual void | aboutToEdit (const KUrl &url, EditHints hints) |
virtual bool | addImage (const KUrl &, QString &err) |
virtual QList< ImageCollection > | allAlbums ()=0 |
virtual void | clearReservation (const KUrl &url, QObject *const reservingObject) |
virtual FileReadWriteLock * | createReadWriteLock (const KUrl &url) const |
virtual ImageCollection | currentAlbum ()=0 |
virtual ImageCollection | currentSelection ()=0 |
virtual void | delImage (const KUrl &) |
virtual void | editingFinished (const KUrl &url, EditHints hints) |
virtual QAbstractItemModel * | getTagTree () const |
bool | hasFeature (Features feature) const |
virtual QVariant | hostSetting (const QString &settingName) |
virtual ImageCollectionSelector * | imageCollectionSelector (QWidget *parent)=0 |
virtual ImageInfo | info (const KUrl &)=0 |
virtual bool | itemIsReserved (const KUrl &url, QString *const descriptionOfAction=0) const |
virtual void | progressCompleted (const QString &id) |
virtual QString | progressScheduled (const QString &title, bool canBeCanceled, bool hasThumb) const |
virtual void | progressStatusChanged (const QString &id, const QString &status) |
virtual void | progressThumbnailChanged (const QString &id, const QPixmap &thumb) |
virtual void | progressValueChanged (const QString &id, float percent) |
virtual void | refreshImages (const KUrl::List &) |
virtual bool | reserveForAction (const KUrl &url, QObject *const reservingObject, const QString &descriptionOfAction) const |
virtual void | thumbnail (const KUrl &url, int size) |
virtual void | thumbnails (const KUrl::List &list, int size) |
virtual UploadWidget * | uploadWidget (QWidget *parent)=0 |
Static Public Member Functions | |
static QString | version () |
Protected Member Functions | |
virtual int | features () const =0 |
Detailed Description
Definition at line 158 of file interface.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 71 of file interface.cpp.
|
virtual |
Definition at line 77 of file interface.cpp.
Member Function Documentation
|
virtual |
Supported if HostSupportsEditHints.
Before an edit operation starts when it has finished, specify a hint for it. Change hints are optional and may allow optimizations.
When aboutToEdit has been called, editingFinished must be called afterwards. It is strongly recommended to use the EditHintScope instead of these methods.
Definition at line 316 of file interface.cpp.
|
virtual |
Tells to host application that a new image has been made available to it.
Returns true if the host application did accept the new image, otherwise err will be filled with an error description.
Definition at line 137 of file interface.cpp.
|
pure virtual |
Returns a list of albums.
Definition at line 160 of file interface.cpp.
|
virtual |
Supported if HostSupportsItemReservation.
Clears a reservation made previously with reserveForAction for the given reservingObject. You must clear any reservation you made, or, alternatively, delete the reserving object.
Definition at line 298 of file interface.cpp.
|
virtual |
Supported if HostSupportsReadWriteLock Creates a ReadWriteLock for the given URL.
You must unlock the ReadWriteLock as often as you locked. Deleting the object does not unlock it. The implementation KIPI host application must be thread-safe.
Definition at line 309 of file interface.cpp.
|
pure virtual |
Returns list of all images in current album.
If there are no current album, the returned KIPI::ImageCollection::isValid() will return false.
Definition at line 148 of file interface.cpp.
|
signal |
Emit when current album selection as changed from host application user interface.
Boolean argument is true if album are select or not in collection.
|
pure virtual |
Current selection in a thumbnail view for example.
If there are no current selection, the returned KIPI::ImageCollection::isValid() will return false.
Definition at line 154 of file interface.cpp.
|
virtual |
Tells to host application that a new image has been removed.
Definition at line 143 of file interface.cpp.
|
virtual |
Definition at line 320 of file interface.cpp.
|
protectedpure virtual |
Return a bitwise or of the KIPI::Features that thus application support.
Definition at line 166 of file interface.cpp.
|
virtual |
Definition at line 250 of file interface.cpp.
|
signal |
Emit when host application has redered item thumbnail.
See thumbnail() and thumbnails() methods for details.
bool KIPI::Interface::hasFeature | ( | Features | feature | ) | const |
Tells whether the host application under which the plugin currently executes a given feature.
See KIPI::Features for details on the individual features.
Definition at line 91 of file interface.cpp.
|
virtual |
Tells to host application to return a setting to share with plugins.
SETTING NAME | VALUE RETURNED | COMMENTS |
---|---|---|
"WriteMetadataUpdateFiletimeStamp" | bool value | Returns true if file timestamp are updated when metadata are saved. |
"WriteMetadataToRAW" | bool value | Returns true if RAW files metadata can be writted to image. |
"UseXMPSidecar4Reading" | bool value | Returns true if XMP sidecar is used to read metadata from item. |
"MetadataWritingMode" | integer | Returns mode to write metadata to item. See KExiv2::MetadataWritingMode for details. |
"FileExtensions" | QString | Returns all file extensions (image, sound, video) managed by host application, separated by blank spaces, (ex: "JPG PNG TIF NEF AVI MP3"). |
"ImagesExtensions" | QString | Returns images file extensions managed by host application, not incuding RAW formats, separated by blank spaces, (ex: "JPG PNG TIF"). |
"RawExtensions" | QString | Returns RAW file extensions managed by host application, separated by blank spaces, (ex: "NEF CR2 ARW PEF"). |
"VideoExtensions" | QString | Returns video file extensions managed by host application, separated by blank spaces, (ex: "AVI MOV MPG"). |
"AudioExtensions" | QString | Return audio file extensions managed by host application, separated by blank spaces, (ex: "MP3 WAV OGG"). |
This method return the default settings. Re-implement this method in your dedicated kipi interface to control kipi-plugins rules with your kipi host application settings.
NOTE: If you want to manage host settings from kipi-plugins, use wrapper class KIPIPlugins::KPHostSettings, not this method directly.
Definition at line 210 of file interface.cpp.
|
pure virtual |
|
pure virtual |
Returns the image info container for item pointed by url.
|
virtual |
Supported if HostSupportsItemReservation.
Returns if the item is reserved. You can pass a pointer to a QString; if the return value is true, the string will be set to the descriptionOfAction set with reserveForAction.
Definition at line 303 of file interface.cpp.
|
signal |
This signal is emit from kipi host when a progress item is canceled.
id is identification string of progress item.
|
virtual |
Definition at line 286 of file interface.cpp.
|
virtual |
Ask to Kipi host application to prepare progress manager for a new entry.
This method must return from host a string identification about progress item created. This id will be used later to change in host progress item value and text. Title is text used to name progress item in host application. Set canBeCanceled to true if you want that progress item provide a cancel button to close process from kipi host. Use progressCanceled() signal to manage feedback from kipi host when cancel button is pressed. Set hasThumb to true if you want that progress item support small thumbnail near progress bar. Use progresssThumbnailChanged() to change thumbnail in kipi host and progressValueChanged() to advance progress bar in percent. Use progressStatusChanged() to change description string of progress item. To close progress item in kipi host, for example when all is done in plugin, use progressCompleted() method. If you Host do not re-implement this method, value returned is a null string. You must re-implement this method if your host support HostSupportsProgressBar feature.
Definition at line 256 of file interface.cpp.
|
virtual |
Definition at line 272 of file interface.cpp.
|
virtual |
Definition at line 279 of file interface.cpp.
|
virtual |
To manage progress state from plugin to host application.
id is identification string of process item returned from host by progressScheduled() method.
Definition at line 265 of file interface.cpp.
|
virtual |
Tells to host application that the following images has changed on disk.
Definition at line 86 of file interface.cpp.
|
signal |
|
signal |
Supported if HostSupportsItemReservation.
Emitted from reservedForAction and clearReservation, respectively.
|
virtual |
Supported if HostSupportsItemReservation.
If an item is scheduled in a plugin for an action which will edit the object, call this method. If the user tries to subject the reserved item to another operation, possibly conflicting, a warning message or other action may be taken.
Give the URL of the item and a QObject which acts as the holder of the reservation. The object must not be null, and the reservation will be cancelled when the object is deleted. descriptionOfAction is a user-presentable string describing the action for which the reservation was made.
Returns true if a reservation was made, or false if a reservation could not be made.
Definition at line 292 of file interface.cpp.
|
signal |
Emit when item selection has changed from host application user interface.
Boolean argument is true if items are select or not in collection.
|
virtual |
Tells to host application to render a thumbnail for an image.
If this method is not re-implemented in host, standard KIO::filePreview is used to generated a thumbnail. Use gotThumbnail() signal to take thumb.
Definition at line 172 of file interface.cpp.
|
virtual |
Ask to Kipi host application to render thumbnails for a list of images.
If this method is not re-implemented in host, standard KIO::filePreview is used to generated a thumbnail. Use gotThumbnail() signal to take thumbs.
Definition at line 177 of file interface.cpp.
|
pure virtual |
|
static |
Returns a string version of libkipi release ID.
Definition at line 81 of file interface.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:45:21 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.