okular
#include <bookmarkmanager.h>
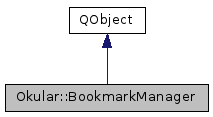
Signals | |
void | bookmarksChanged (const KUrl &url) |
void | openUrl (const KUrl &url) |
void | saved () |
Public Member Functions | |
virtual | ~BookmarkManager () |
QList< QAction * > | actionsForUrl (const KUrl &url) const |
void | addBookmark (int page) |
void | addBookmark (const DocumentViewport &vp) |
bool | addBookmark (const KUrl &referurl, const Okular::DocumentViewport &vp, const QString &title=QString()) |
KBookmark | bookmark (int page) const |
KBookmark | bookmark (const DocumentViewport &viewport) const |
KBookmark::List | bookmarks (const KUrl &url) const |
KBookmark::List | bookmarks () const |
KBookmark::List | bookmarks (int page) const |
KUrl::List | files () const |
bool | isBookmarked (int page) const |
bool | isBookmarked (const DocumentViewport &viewport) const |
KBookmark | nextBookmark (const DocumentViewport &viewport) const |
KBookmark | previousBookmark (const DocumentViewport &viewport) const |
void | removeBookmark (int page) |
void | removeBookmark (const DocumentViewport &vp) |
int | removeBookmark (const KUrl &referurl, const KBookmark &bm) |
void | removeBookmarks (const KUrl &referurl, const KBookmark::List &list) |
void | renameBookmark (KBookmark *bm, const QString &newName) |
void | renameBookmark (const KUrl &referurl, const QString &newName) |
void | save () const |
QString | titleForUrl (const KUrl &referurl) const |
Detailed Description
Bookmarks manager utility.
This class is responsible for loading and saving the bookmarks using the proper format, and for working with them (eg querying, adding, removing).
Definition at line 32 of file bookmarkmanager.h.
Constructor & Destructor Documentation
|
virtual |
Definition at line 148 of file bookmarkmanager.cpp.
Member Function Documentation
QList< QAction * > BookmarkManager::actionsForUrl | ( | const KUrl & | url | ) | const |
Returns a list of actions for the bookmarks of the specified url
.
- Note
- the actions will have no parents, so you have to delete them yourself
Definition at line 587 of file bookmarkmanager.cpp.
void BookmarkManager::addBookmark | ( | int | page | ) |
Adds a bookmark for the given page
.
Definition at line 385 of file bookmarkmanager.cpp.
void BookmarkManager::addBookmark | ( | const DocumentViewport & | vp | ) |
Adds a bookmark for the given viewport vp
.
- Since
- 0.15 (KDE 4.9)
Definition at line 394 of file bookmarkmanager.cpp.
bool BookmarkManager::addBookmark | ( | const KUrl & | referurl, |
const Okular::DocumentViewport & | vp, | ||
const QString & | title = QString() |
||
) |
Adds a new bookmark for the referurl
at the specified viewport vp
, with an optional title
.
If no title
is specified, then #n will be used.
Definition at line 399 of file bookmarkmanager.cpp.
KBookmark BookmarkManager::bookmark | ( | int | page | ) | const |
Returns the bookmark for the given page of the document.
- Since
- 0.14 (KDE 4.8)
Definition at line 292 of file bookmarkmanager.cpp.
KBookmark BookmarkManager::bookmark | ( | const DocumentViewport & | viewport | ) | const |
Returns the bookmark for the given viewport
of the document.
- Since
- 0.15 (KDE 4.9)
Definition at line 306 of file bookmarkmanager.cpp.
KBookmark::List BookmarkManager::bookmarks | ( | const KUrl & | url | ) | const |
Returns the list of bookmarks for the specified url
.
Definition at line 248 of file bookmarkmanager.cpp.
KBookmark::List BookmarkManager::bookmarks | ( | ) | const |
Returns the list of bookmarks for document.
- Since
- 0.14 (KDE 4.8)
Definition at line 271 of file bookmarkmanager.cpp.
KBookmark::List BookmarkManager::bookmarks | ( | int | page | ) | const |
Returns the list of bookmarks for the given page of the document.
- Since
- 0.15 (KDE 4.9)
Definition at line 276 of file bookmarkmanager.cpp.
|
signal |
The bookmarks for specified url
were changed.
- Since
- 0.7 (KDE 4.1)
KUrl::List BookmarkManager::files | ( | ) | const |
Returns the list of documents with bookmarks.
Definition at line 234 of file bookmarkmanager.cpp.
bool BookmarkManager::isBookmarked | ( | int | page | ) | const |
Returns whether the given page
is bookmarked.
Definition at line 689 of file bookmarkmanager.cpp.
bool BookmarkManager::isBookmarked | ( | const DocumentViewport & | viewport | ) | const |
Return whether the given viewport
is bookmarked.
- Since
- 0.15 (KDE 4.9)
Definition at line 694 of file bookmarkmanager.cpp.
KBookmark BookmarkManager::nextBookmark | ( | const DocumentViewport & | viewport | ) | const |
Given a viewport
, returns the next bookmark.
- Since
- 0.15 (KDE 4.9)
Definition at line 701 of file bookmarkmanager.cpp.
|
signal |
The bookmark manager is requesting to open the specified url
.
KBookmark BookmarkManager::previousBookmark | ( | const DocumentViewport & | viewport | ) | const |
Given a viewport
, returns the previous bookmark.
- Since
- 0.15 (KDE 4.9)
Definition at line 720 of file bookmarkmanager.cpp.
void BookmarkManager::removeBookmark | ( | int | page | ) |
Remove a bookmark for the given page
.
Definition at line 459 of file bookmarkmanager.cpp.
void BookmarkManager::removeBookmark | ( | const DocumentViewport & | vp | ) |
Remove a bookmark for the given viewport vp
.
- Since
- 0.15 (KDE 4.9)
Definition at line 468 of file bookmarkmanager.cpp.
int BookmarkManager::removeBookmark | ( | const KUrl & | referurl, |
const KBookmark & | bm | ||
) |
Removes the bookmark bm
for the referurl
specified.
Definition at line 513 of file bookmarkmanager.cpp.
void BookmarkManager::removeBookmarks | ( | const KUrl & | referurl, |
const KBookmark::List & | list | ||
) |
Removes the bookmarks in list
for the referurl
specified.
- Note
- it will remove only the bookmarks which belong to
referurl
- Since
- 0.11 (KDE 4.5)
Definition at line 539 of file bookmarkmanager.cpp.
void BookmarkManager::renameBookmark | ( | KBookmark * | bm, |
const QString & | newName | ||
) |
Returns the bookmark given bookmark of the document.
- Since
- 0.14 (KDE 4.8)
Definition at line 477 of file bookmarkmanager.cpp.
void BookmarkManager::renameBookmark | ( | const KUrl & | referurl, |
const QString & | newName | ||
) |
Renames the top-level bookmark for the referurl
specified with the newName
specified.
- Since
- 0.15 (KDE 4.9)
Definition at line 489 of file bookmarkmanager.cpp.
void BookmarkManager::save | ( | ) | const |
Forces to save the list of bookmarks.
Definition at line 331 of file bookmarkmanager.cpp.
|
signal |
This signal is emitted whenever bookmarks have been saved.
QString BookmarkManager::titleForUrl | ( | const KUrl & | referurl | ) | const |
Returns title for the referurl
.
- Since
- 0.15 (KDE 4.9)
Definition at line 504 of file bookmarkmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:45:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.