okular
#include <annotations.h>
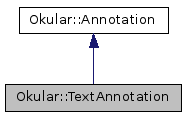
Public Types | |
enum | InplaceIntent { Unknown, Callout, TypeWriter } |
enum | TextType { Linked, InPlace } |
![]() | |
enum | AdditionalActionType { PageOpening, PageClosing } |
typedef void(* | DisposeDataFunction )(const Okular::Annotation *) |
enum | Flag { Hidden = 1, FixedSize = 2, FixedRotation = 4, DenyPrint = 8, DenyWrite = 16, DenyDelete = 32, ToggleHidingOnMouse = 64, External = 128, ExternallyDrawn = 256, BeingMoved = 512 } |
enum | LineEffect { NoEffect = 1, Cloudy = 2 } |
enum | LineStyle { Solid = 1, Dashed = 2, Beveled = 4, Inset = 8, Underline = 16 } |
enum | RevisionScope { Reply = 1, Group = 2, Delete = 4 } |
enum | RevisionType { None = 1, Marked = 2, Unmarked = 4, Accepted = 8, Rejected = 16, Cancelled = 32, Completed = 64 } |
enum | SubType { AText = 1, ALine = 2, AGeom = 3, AHighlight = 4, AStamp = 5, AInk = 6, ACaret = 8, AFileAttachment = 9, ASound = 10, AMovie = 11, AScreen = 12, AWidget = 13, A_BASE = 0 } |
Public Member Functions | |
TextAnnotation () | |
TextAnnotation (const QDomNode &description) | |
~TextAnnotation () | |
int | inplaceAlignment () const |
NormalizedPoint | inplaceCallout (int index) const |
InplaceIntent | inplaceIntent () const |
void | setInplaceAlignment (int alignment) |
void | setInplaceCallout (const NormalizedPoint &point, int index) |
void | setInplaceIntent (InplaceIntent intent) |
void | setTextFont (const QFont &font) |
void | setTextIcon (const QString &icon) |
void | setTextType (TextType type) |
void | store (QDomNode &node, QDomDocument &document) const |
SubType | subType () const |
QFont | textFont () const |
QString | textIcon () const |
TextType | textType () const |
NormalizedPoint | transformedInplaceCallout (int index) const |
![]() | |
virtual | ~Annotation () |
QString | author () const |
NormalizedRect | boundingRectangle () const |
bool | canBeMoved () const |
QString | contents () const |
QDateTime | creationDate () const |
int | flags () const |
QDomNode | getAnnotationPropertiesDomNode () const |
QDateTime | modificationDate () const |
QVariant | nativeId () const |
bool | openDialogAfterCreation () const |
QLinkedList< Revision > & | revisions () |
const QLinkedList< Revision > & | revisions () const |
void | setAnnotationProperties (const QDomNode &node) |
void | setAuthor (const QString &author) |
void | setBoundingRectangle (const NormalizedRect &rectangle) |
void | setContents (const QString &contents) |
void | setCreationDate (const QDateTime &date) |
void | setDisposeDataFunction (DisposeDataFunction func) |
void | setFlags (int flags) |
void | setModificationDate (const QDateTime &date) |
void | setNativeId (const QVariant &id) |
void | setUniqueName (const QString &name) |
Style & | style () |
const Style & | style () const |
NormalizedRect | transformedBoundingRectangle () const |
void | translate (const NormalizedPoint &coord) |
QString | uniqueName () const |
Window & | window () |
const Window & | window () const |
Detailed Description
Definition at line 731 of file annotations.h.
Member Enumeration Documentation
Describes the style of the text.
Enumerator | |
---|---|
Unknown |
Unknown style. |
Callout |
Callout style. |
TypeWriter |
Type writer style. |
Definition at line 746 of file annotations.h.
Describes the type of the text.
Enumerator | |
---|---|
Linked |
The annotation is linked to a text. |
InPlace |
The annotation is located next to the text. |
Definition at line 737 of file annotations.h.
Constructor & Destructor Documentation
TextAnnotation::TextAnnotation | ( | ) |
Creates a new text annotation.
Definition at line 1004 of file annotations.cpp.
TextAnnotation::TextAnnotation | ( | const QDomNode & | description | ) |
Creates a new text annotation from the xml description
.
Definition at line 1009 of file annotations.cpp.
TextAnnotation::~TextAnnotation | ( | ) |
Destroys the text annotation.
Definition at line 1014 of file annotations.cpp.
Member Function Documentation
int TextAnnotation::inplaceAlignment | ( | ) | const |
Returns the inplace alignment of the text annotation.
Definition at line 1060 of file annotations.cpp.
NormalizedPoint TextAnnotation::inplaceCallout | ( | int | index | ) | const |
Returns the inplace callout point for index
.
index
must be between 0 and 2.
Definition at line 1075 of file annotations.cpp.
TextAnnotation::InplaceIntent TextAnnotation::inplaceIntent | ( | ) | const |
Returns the inplace intent of the text annotation.
Definition at line 1099 of file annotations.cpp.
void TextAnnotation::setInplaceAlignment | ( | int | alignment | ) |
Sets the inplace alignment
of the text annotation.
Definition at line 1054 of file annotations.cpp.
void TextAnnotation::setInplaceCallout | ( | const NormalizedPoint & | point, |
int | index | ||
) |
Sets the inplace callout point
at index
.
index
must be between 0 and 2.
Definition at line 1066 of file annotations.cpp.
void TextAnnotation::setInplaceIntent | ( | InplaceIntent | intent | ) |
Returns the inplace intent
of the text annotation.
- See also
- InplaceIntent
Definition at line 1093 of file annotations.cpp.
void TextAnnotation::setTextFont | ( | const QFont & | font | ) |
Sets the font
of the text annotation.
Definition at line 1042 of file annotations.cpp.
void TextAnnotation::setTextIcon | ( | const QString & | icon | ) |
Sets the icon
of the text annotation.
Definition at line 1030 of file annotations.cpp.
void TextAnnotation::setTextType | ( | TextType | type | ) |
Sets the text type
of the text annotation.
- See also
- TextType
Definition at line 1018 of file annotations.cpp.
|
virtual |
Stores the text annotation as xml in document
under the given parent node
.
Reimplemented from Okular::Annotation.
Definition at line 1110 of file annotations.cpp.
|
virtual |
Returns the sub type of the text annotation.
Implements Okular::Annotation.
Definition at line 1105 of file annotations.cpp.
QFont TextAnnotation::textFont | ( | ) | const |
Returns the font of the text annotation.
Definition at line 1048 of file annotations.cpp.
QString TextAnnotation::textIcon | ( | ) | const |
Returns the icon of the text annotation.
Definition at line 1036 of file annotations.cpp.
TextAnnotation::TextType TextAnnotation::textType | ( | ) | const |
Returns the text type of the text annotation.
Definition at line 1024 of file annotations.cpp.
NormalizedPoint TextAnnotation::transformedInplaceCallout | ( | int | index | ) | const |
Returns the transformed (e.g.
rotated) inplace callout point for index
.
index
must be between 0 and 2.
Definition at line 1084 of file annotations.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:45:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.