Interfaces
#include <kimproxy.h>
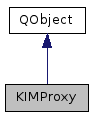
Public Slots | |
bool | addContact (const QString &contactId, const QString &protocol) |
QStringList | allContacts () |
bool | canReceiveFiles (const QString &uid) |
bool | canRespond (const QString &uid) |
void | chatWithContact (const QString &uid) |
void | contactPresenceChanged (const QString &uid, const QString &appId, int presence) |
QString | context (const QString &uid) |
QString | displayName (const QString &uid) |
QStringList | fileTransferContacts () |
bool | imAppsAvailable () |
bool | isPresent (const QString &uid) |
QString | locate (const QString &contactId, const QString &protocol) |
void | messageContact (const QString &uid, const QString &message) |
QStringList | onlineContacts () |
QPixmap | presenceIcon (const QString &uid) |
int | presenceNumeric (const QString &uid) |
QString | presenceString (const QString &uid) |
QStringList | reachableContacts () |
void | sendFile (const QString &uid, const QString &sourceURL, const QString &altFileName=QString(), uint fileSize=0) |
bool | startPreferredApp () |
Signals | |
void | sigContactPresenceChanged (const QString &uid) |
void | sigPresenceInfoExpired () |
Public Member Functions | |
~KIMProxy () | |
bool | initialize () |
Static Public Member Functions | |
static KIMProxy * | instance () |
Protected Member Functions | |
void | pollAll (const QString &uid) |
void | pollApp (const QString &appId) |
QString | preferredApp () |
OrgKdeKIMInterface * | stubForProtocol (const QString &protocol) |
OrgKdeKIMInterface * | stubForUid (const QString &uid) |
bool | updatePresence (const QString &uid, const QString &appId, int presence) |
Detailed Description
This class provides an easy-to-use interface to any instant messengers or chat programs that you have installed that implement KIMIface.
It works simultaneously with any running programs that implement the ServiceType DBUS/InstantMessenger If a UID is reachable with more than one program, KIMProxy aggregates the available information and presents the 'best' presence. For example, for a contact who can be seen to be Away in IRC on program A but Online using ICQ on program B, the information from program B will be used. KIMProxy is designed for simple information in a wide number of cases, not for detailed messaging.
Most operations work in terms of uids belonging to KABC::Addressee, but use of the address book is optional. You can get a list of known contacts with imAddresseeUids and then check their presence using the various accessor methods presenceString, presenceNumeric and display the IM programs' display names for them using displayName.
To use, just get an instance using instance.
Definition at line 68 of file kimproxy.h.
Constructor & Destructor Documentation
KIMProxy::~KIMProxy | ( | ) |
Definition at line 210 of file kimproxy.cpp.
Member Function Documentation
Add a contact to the contact list.
- Parameters
-
contactId the protocol specific identifier for the contact, eg UIN for ICQ, screenname for AIM, nick for IRC. protocol the protocol, eg one of "AIMProtocol", "MSNProtocol", "ICQProtocol",
- Returns
- whether the add succeeded. False may signal already present, protocol not supported, or add operation not supported.
Definition at line 520 of file kimproxy.cpp.
|
slot |
Obtain a list of IM-contactable entries in the KDE address book.
- Returns
- a list of KABC uids.
Definition at line 378 of file kimproxy.cpp.
Indicate if a given uid can receive files.
- Parameters
-
uid the KABC uid you are interested in.
- Returns
- Whether the specified addressee can receive files.
Definition at line 445 of file kimproxy.cpp.
Some media are unidirectional (eg, sending SMS via a web interface).
- Parameters
-
uid the KABC uid you are interested in.
- Returns
- Whether the specified addressee can respond.
Definition at line 455 of file kimproxy.cpp.
|
slot |
Start a chat session with the specified addressee.
- Parameters
-
uid the KABC uid you want to chat with.
Definition at line 475 of file kimproxy.cpp.
|
slot |
Just exists to let the idl compiler make the D-Bus signal for this.
Definition at line 312 of file kimproxy.cpp.
Get the supplied addressee's current context (home, work, or any).
- Parameters
-
uid the KABC uid you want the context for.
- Returns
- A QString describing the context, or null if not supported.
Definition at line 465 of file kimproxy.cpp.
Obtain the IM app's idea of the contact's display name Useful if KABC lookups may be too slow.
- Parameters
-
uid the KABC uid you are interested in.
- Returns
- The corresponding display name.
Definition at line 433 of file kimproxy.cpp.
|
slot |
Obtain a list of KDE address book entries who may receive file transfers.
- Returns
- a list of KABC uids capable of file transfer.
Definition at line 412 of file kimproxy.cpp.
|
slot |
Are there any compatible instant messaging apps installed?
- Returns
- true if there are any apps installed, to see if they are running, initialize instead.
Definition at line 540 of file kimproxy.cpp.
bool KIMProxy::initialize | ( | ) |
Get the proxy ready to connect Discover any running preferred IM clients and set up stubs for it.
- Returns
- whether the proxy is ready to use. False if there are no apps running.
Definition at line 215 of file kimproxy.cpp.
|
static |
Obtain an instance of KIMProxy.
- Returns
- The singleton instance of this class.
Definition at line 175 of file kimproxy.cpp.
Confirm if a given KABC uid is known to KIMProxy.
- Parameters
-
uid the KABC uid you are interested in.
- Returns
- whether one of the chat programs KIMProxy talks to knows of this KABC uid.
Definition at line 428 of file kimproxy.cpp.
Get the KABC uid corresponding to the supplied IM address Protocols should be.
- Parameters
-
contactId the protocol specific identifier for the contact, eg UIN for ICQ, screenname for AIM, nick for IRC. protocol the protocol, eg one of "AIMProtocol", "MSNProtocol", "ICQProtocol",
- Returns
- a KABC uid or null if none found/
Definition at line 530 of file kimproxy.cpp.
Send a single message to the specified addressee Any response will be handled by the IM client as a normal conversation.
- Parameters
-
uid the KABC uid you want to chat with. message the message to send them.
Definition at line 488 of file kimproxy.cpp.
|
slot |
Obtain a list of KDE address book entries who are currently online.
- Returns
- a list of KABC uids who are online with unspecified presence.
Definition at line 400 of file kimproxy.cpp.
|
protected |
Bootstrap our presence data by polling all known apps.
Definition at line 566 of file kimproxy.cpp.
|
protected |
Bootstrap our presence data for a newly registered app.
Definition at line 585 of file kimproxy.cpp.
|
protected |
Get the name of the user's IM weapon of choice.
Definition at line 645 of file kimproxy.cpp.
|
slot |
Obtain the icon representing IM presence for the specified addressee.
- Parameters
-
uid the KABC uid you want the presence for.
- Returns
- a pixmap representing the uid's presence.
Definition at line 357 of file kimproxy.cpp.
|
slot |
Obtain the IM presence as a number (see KIMIface) for the specified addressee.
- Parameters
-
uid the KABC uid you want the presence for.
- Returns
- a numeric representation of presence - currently one of 0 (Unknown), 1 (Offline), 2 (Connecting), 3 (Away), 4 (Online)
Definition at line 330 of file kimproxy.cpp.
Obtain the IM presence as a i18ned string for the specified addressee.
- Parameters
-
uid the KABC uid you want the presence for.
- Returns
- the i18ned string describing presence.
Definition at line 342 of file kimproxy.cpp.
|
slot |
Obtain a list of KDE address book entries who are currently reachable.
- Returns
- a list of KABC uids who can receive a message, even if online.
Definition at line 384 of file kimproxy.cpp.
|
slot |
Send the file to the contact.
- Parameters
-
uid the KABC uid you are sending to. sourceURL a KUrl to send. altFileName an alternate filename describing the file fileSize file size in bytes
Definition at line 501 of file kimproxy.cpp.
|
signal |
Indicates that the specified UID's presence changed.
- Parameters
-
uid the KABC uid whose presence changed.
|
signal |
Indicates that the sources of presence information have changed so any previously supplied presence info is invalid.
|
slot |
Start the user's preferred IM application.
- Returns
- whether a preferred app was found. No guarantee that it started correctly
Definition at line 545 of file kimproxy.cpp.
|
protected |
Get the app stub for this protocol.
Take the preferred app first, then any other.
Definition at line 618 of file kimproxy.cpp.
|
protected |
Get the app stub best able to reach this uid.
Definition at line 610 of file kimproxy.cpp.
|
protected |
Update our records with the given data.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:10 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.