KTextEditor
#include <editor.h>
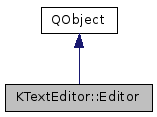
Signals | |
void | documentCreated (KTextEditor::Editor *editor, KTextEditor::Document *document) |
Public Member Functions | |
Editor (QObject *parent) | |
virtual | ~Editor () |
virtual const KAboutData * | aboutData () const =0 |
virtual void | configDialog (QWidget *parent)=0 |
virtual bool | configDialogSupported () const =0 |
virtual ConfigPage * | configPage (int number, QWidget *parent)=0 |
virtual QString | configPageFullName (int number) const =0 |
virtual KIcon | configPageIcon (int number) const =0 |
virtual QString | configPageName (int number) const =0 |
virtual int | configPages () const =0 |
virtual Document * | createDocument (QObject *parent)=0 |
const QString & | defaultEncoding () const |
virtual const QList< Document * > & | documents ()=0 |
virtual void | readConfig (KConfig *config=0)=0 |
void | setSimpleMode (bool on) |
bool | simpleMode () const |
virtual void | writeConfig (KConfig *config=0)=0 |
Protected Member Functions | |
void | setDefaultEncoding (const QString &defaultEncoding) |
Detailed Description
Accessor interface for Editor part.
Topics:
Introduction
The Editor part can be accessed via the KTextEditor::Factory or the KTextEditor::EditorChooser and provides general information and configuration methods for the Editor implementation, for example KAboutData by using aboutData().
The Editor implementation has a list of all opened documents. Get this list with documents(). To create a new Document call createDocument(). The signal documentCreated() is emitted whenever the Editor created a new document.
Editor Configuration
If the Editor implementation supports a config dialog configDialogSupported() returns true, then the config dialog can be shown with configDialog(). Instead of using the config dialog, the config pages can be embedded into the application's config dialog. To do this, configPages() returns the number of config pages the Editor implementation provides and configPage() returns the requested page. Further, a config page has a short descriptive name, get it with configPageName(). You can get more detailed name by using configPageFullName(). Also every config page has a pixmap, get it with configPagePixmap(). The configuration can be saved and loaded with readConfig() and writeConfig().
- Note
- We recommend to embedd the config pages into the main application's config dialog instead of using a separate config dialog, if the config dialog does not look cluttered then. This way, all settings are grouped together in one place.
Implementation Notes
Usually only one instance of the Editor exists. The Kate Part implementation internally uses a static accessor to make sure that only one Kate Part Editor object exists. So several factories still use the same Editor.
readConfig() and writeConfig() should be forwarded to all loaded plugins as well, see KTextEditor::Plugin::readConfig() and KTextEditor::Plugin::writeConfig().
Editor Extension Interfaces
There is only a single extension interface for the Editor: the CommandInterface. With the CommandInterface it is possible to add and remove new command line commands which are valid for all documents. Common use cases are for example commands like find or setting document variables. For further details read the detailed descriptions in the class KTextEditor::CommandInterface.
Constructor & Destructor Documentation
Editor::Editor | ( | QObject * | parent | ) |
Constructor.
Create the Editor object with parent
.
- Parameters
-
parent parent object
Definition at line 85 of file ktexteditor.cpp.
|
virtual |
Virtual destructor.
Definition at line 91 of file ktexteditor.cpp.
Member Function Documentation
|
pure virtual |
Get the about data of this Editor part.
- Returns
- about data
|
pure virtual |
Show the editor's config dialog, changes will be applied to the editor, but not saved anywhere automagically, call writeConfig()
to save them.
- Note
- Instead of using the config dialog, the config pages can be embedded into your own config dialog by using configPages() and configPage().
- Parameters
-
parent parent widget
- See also
- configDialogSupported()
|
pure virtual |
Check, whether this editor has a configuration dialog.
- Returns
- true, if the editor has a configuration dialog, otherwise false
- See also
- configDialog()
|
pure virtual |
Get the config page with the number
, config pages from 0 to configPages()-1 are available if configPages() > 0.
- Parameters
-
number index of config page parent parent widget for config page
- Returns
- created config page or NULL, if the number is out of bounds
- See also
- configPages()
|
pure virtual |
Get a readable full name for the config page number.
The name should be translated.
Example: If the name is "Filetypes", the full name could be "Filetype Specific Settings". For "Shortcuts" the full name would be something like "Shortcut Configuration".
- Parameters
-
number index of config page
- Returns
- full name of given page index
- See also
- configPageName(), configPagePixmap()
|
pure virtual |
Get a pixmap with size
for the config page number
.
- Parameters
-
number index of config page
- Returns
- pixmap for the given page index
- See also
- configPageName(), configPageFullName()
|
pure virtual |
Get a readable name for the config page number
.
The name should be translated.
- Parameters
-
number index of config page
- Returns
- name of given page index
- See also
- configPageFullName(), configPagePixmap()
|
pure virtual |
Get the number of available config pages.
If the editor returns a number < 1, it does not support config pages and the embedding application should use configDialog() instead.
- Returns
- number of config pages
- See also
- configPage(), configDialog()
Create a new document object with parent
.
- Parameters
-
parent parent object
- Returns
- new KTextEditor::Document object
- See also
- documents()
const QString & Editor::defaultEncoding | ( | ) | const |
Get the current default encoding for this Editor part.
- Returns
- default encoding
- Since
- 4.5
Definition at line 106 of file ktexteditor.cpp.
|
signal |
The editor
emits this signal whenever a document
was successfully created.
- Parameters
-
editor editor which created the new document document the newly created document instance
- See also
- createDocument()
Get a list of all documents of this editor.
- Returns
- list of all existing documents
- See also
- createDocument()
|
pure virtual |
Read editor configuration from KConfig config
.
- Note
- Implementation Notes: If
config
is NULL you should use kapp->config() as a fallback solution. Additionally the readConfig() call should be forwarded to every loaded plugin.
- Parameters
-
config config object
- See also
- writeConfig()
|
protected |
Set the current default encoding for this Editor part.
Editor part implementation should call this internally on creation and config changes.
- Parameters
-
defaultEncoding new default encoding
- Since
- 4.5
Definition at line 111 of file ktexteditor.cpp.
void Editor::setSimpleMode | ( | bool | on | ) |
Switch editor to simple mode for average users.
Switch the editor to a simple mode which will hide advanced stuff from average user or switch it back to normal mode. This mode will only affect documents/views created after the change.
- Parameters
-
on turn simple mode on or not
Definition at line 96 of file ktexteditor.cpp.
bool Editor::simpleMode | ( | ) | const |
Query the editor whether simple mode is on or not.
- Returns
- true if simple mode is on, otherwise false
- See also
- setSimpleMode()
Definition at line 101 of file ktexteditor.cpp.
|
pure virtual |
Write editor configuration to KConfig config
.
- Note
- Implementation Notes: If
config
is NULL you should use kapp->config() as a fallback solution. Additionally the writeConfig() call should be forwarded to every loaded plugin.
- Parameters
-
config config object
- See also
- readConfig()
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.