KDE3Support
#include <k3command.h>
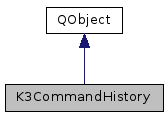
Public Slots | |
virtual void | documentSaved () |
virtual void | redo () |
virtual void | undo () |
Signals | |
void | commandExecuted (K3Command *command) |
void | commandHistoryChanged () |
void | documentRestored () |
Public Member Functions | |
K3CommandHistory () | |
K3CommandHistory (KActionCollection *actionCollection, bool withMenus=true) | |
virtual | ~K3CommandHistory () |
void | addCommand (K3Command *command, bool execute=true) |
void | clear () |
bool | isRedoAvailable () const |
bool | isUndoAvailable () const |
K3Command * | presentCommand () const |
QList< K3Command * > | redoCommands (int maxCommands=0) const |
int | redoLimit () const |
void | setRedoLimit (int limit) |
void | setUndoLimit (int limit) |
QList< K3Command * > | undoCommands (int maxCommands=0) const |
int | undoLimit () const |
void | updateActions () |
Detailed Description
The command history stores a (user) configurable amount of Commands.
It keeps track of its size and deletes commands if it gets too large. The user can set a maximum undo and a maximum redo limit (e.g. max. 50 undo / 30 redo commands). The K3CommandHistory keeps track of the "borders" and deletes commands, if appropriate. It also activates/deactivates the undo/redo actions in the menu and changes the text according to the name of the command.
Note: you might want to use the QUndo framework instead. It didn't exist when K3Command was written.
Definition at line 180 of file k3command.h.
Constructor & Destructor Documentation
K3CommandHistory::K3CommandHistory | ( | ) |
Creates a command history, to store commands.
This constructor doesn't create actions, so you need to call undo and redo yourself.
Definition at line 149 of file k3command.cpp.
K3CommandHistory::K3CommandHistory | ( | KActionCollection * | actionCollection, |
bool | withMenus = true |
||
) |
Creates a command history, to store commands.
This also creates an undo and a redo action, in the actionCollection
, using the standard names ("edit_undo" and "edit_redo").
- Parameters
-
withMenus if true, the actions will display a menu when plugged into a toolbar. actionCollection the parent collection
Definition at line 155 of file k3command.cpp.
|
virtual |
Destructs the command history object.
Definition at line 176 of file k3command.cpp.
Member Function Documentation
Adds a command to the history.
Call this for each command
you create. Unless you set execute
to false, this will also execute the command. This means, most of the application's code will look like MyCommand * cmd = new MyCommand( parameters ); m_historyCommand.addCommand( cmd );
Note that the command history takes ownership of the command, it will delete it when the undo limit is reached, or when deleting the command history itself.
Definition at line 188 of file k3command.cpp.
void K3CommandHistory::clear | ( | ) |
Erases all the undo/redo history.
Use this when reloading the data, for instance, since this invalidates all the commands.
Definition at line 180 of file k3command.cpp.
|
signal |
Emitted every time a command is executed (whether by addCommand, undo or redo).
You can use this to update the GUI, for instance.
- Parameters
-
command was executed
|
signal |
Emitted whenever the command history has changed, i.e.
after addCommand, undo or redo. This is used by the actions to update themselves.
|
signal |
Emitted every time we reach the index where you saved the document for the last time.
See documentSaved
|
virtualslot |
Remembers when you saved the document.
Call this right after saving the document. As soon as the history reaches the current index again (via some undo/redo operations) it will emit documentRestored If you implemented undo/redo properly the document is the same you saved before.
Definition at line 248 of file k3command.cpp.
bool K3CommandHistory::isRedoAvailable | ( | ) | const |
- Returns
- true if redo is available i.e. there is at least one command that can be redone right now
Definition at line 307 of file k3command.cpp.
bool K3CommandHistory::isUndoAvailable | ( | ) | const |
- Returns
- true if undo is available, i.e. there is at least one command that can be undone right now
Definition at line 302 of file k3command.cpp.
K3Command * K3CommandHistory::presentCommand | ( | ) | const |
- Returns
- the present command, i.e. the one that undo() would unexecute. This can be used to e.g. show selection.
Definition at line 212 of file k3command.cpp.
|
virtualslot |
Redoes the last undone action.
Call this if you don't use the builtin KActions.
Definition at line 235 of file k3command.cpp.
- Returns
- the list of next
maxCommands
actions that will be redone by redo() The returned list is empty if !isRedoAvailable(). Otherwise the list starts with the next command to redo.
- Parameters
-
maxCommands maximum number of commands requested. 0 means no maximum, all stored redo commands (within redoLimit()) are returned.
Definition at line 323 of file k3command.cpp.
int K3CommandHistory::redoLimit | ( | ) | const |
- Returns
- the maximum number of items in the redo history
Definition at line 340 of file k3command.cpp.
void K3CommandHistory::setRedoLimit | ( | int | limit | ) |
Sets the maximum number of items in the redo history.
Definition at line 259 of file k3command.cpp.
void K3CommandHistory::setUndoLimit | ( | int | limit | ) |
Sets the maximum number of items in the undo history.
Definition at line 252 of file k3command.cpp.
|
virtualslot |
Undoes the last action.
Call this if you don't use the builtin KActions.
Definition at line 219 of file k3command.cpp.
- Returns
- the list of next
maxCommands
actions that will be undone by undo() The returned list is empty if !isUndoAvailable(). Otherwise the list starts with the next command to undo, i.e. the order of the commands in the list is the reverse of the chronological order of the commands.
- Parameters
-
maxCommands maximum number of commands requested. 0 means no maximum, all stored undo commands (within undoLimit()) are returned.
Definition at line 312 of file k3command.cpp.
int K3CommandHistory::undoLimit | ( | ) | const |
- Returns
- the maximum number of items in the undo history
Definition at line 335 of file k3command.cpp.
void K3CommandHistory::updateActions | ( | ) |
Enable or disable the undo and redo actions.
This isn't usually necessary, but this method can be useful if you disable all actions (to go to a "readonly" state), and then want to come back to a readwrite mode.
Definition at line 296 of file k3command.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:51:59 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.