KDE3Support
#include <k3dockwidget.h>
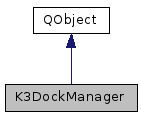
Public Types | |
enum | EnReadDockConfigMode { Unknown, WrapExistingWidgetsOnly, RestoreAllDockwidgets } |
Signals | |
void | change () |
void | replaceDock (K3DockWidget *oldDock, K3DockWidget *newDock) |
void | setDockDefaultPos (K3DockWidget *) |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
The manager that knows all dockwidgets and handles the dock process (and member of the dockwidget class set).
More or less a helper class for the K3DockWidget class set but of interest for some functionality that can be called within a K3DockMainWindow or a K3DockWidget .
An important feature is the ability to read or save the current state of all things concerning to dockwidgets to KConfig .
The dockmanager is also often used when a certain dockwidget or a child of such dockwidget must be found.
Definition at line 884 of file k3dockwidget.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Unknown | |
WrapExistingWidgetsOnly | |
RestoreAllDockwidgets |
Definition at line 891 of file k3dockwidget.h.
Constructor & Destructor Documentation
K3DockManager::K3DockManager | ( | QWidget * | mainWindow, |
const char * | name = 0L |
||
) |
Constructs a dockmanager.
Some initialization happen:
- It installs an event filter for the main window,
- a control list for dock objects
- a control list for menu items concerning to menus provided by the dockmanager
- Some state variables are set
- Parameters
-
mainWindow the main window controlled by this name the internal QOject name
Definition at line 1731 of file k3dockwidget.cpp.
|
virtual |
Destructs a dockmanager.
Definition at line 1785 of file k3dockwidget.cpp.
Member Function Documentation
void K3DockManager::activate | ( | ) |
Shows all encapsulated widgets of all controlled dockwidgets and shows all dockwidgets which are parent of a dockwidget tab group.
Definition at line 1802 of file k3dockwidget.cpp.
|
signal |
Signals changes of the docking state of a dockwidget.
Usually the dock-toolbar will be updated then.
Q3PopupMenu * K3DockManager::dockHideShowMenu | ( | ) | const |
- Returns
- the popupmenu for showing/hiding dockwidgets
Definition at line 3081 of file k3dockwidget.cpp.
void K3DockManager::dumpDockWidgets | ( | ) |
Definition at line 2975 of file k3dockwidget.cpp.
It's more or less a method that catches several events which are interesting for the dockmanager.
Mainly mouse events during the drag process of a dockwidgets are of interest here.
- Parameters
-
object the object that sends the event event the event
- Returns
- the return value of the method call of the base class method
Definition at line 1815 of file k3dockwidget.cpp.
K3DockWidget * K3DockManager::findWidgetParentDock | ( | QWidget * | w | ) | const |
This method finds out what a widgets' dockwidget is.
That means the dockmanager has a look at all dockwidgets it knows and tells you when one of those dockwidgets covers the given widget.
- Parameters
-
w any widget that is supposed to be encapsulated by one of the controlled dockwidgets
- Returns
- the dockwidget that encapsulates that widget, otherwise 0
Definition at line 3062 of file k3dockwidget.cpp.
void K3DockManager::finishReadDockConfig | ( | ) |
Definition at line 2627 of file k3dockwidget.cpp.
K3DockWidget * K3DockManager::getDockWidgetFromName | ( | const QString & | dockName | ) |
- Parameters
-
dockName an internal QObject name
- Returns
- the dockwidget that has got that internal QObject name
Definition at line 2986 of file k3dockwidget.cpp.
void K3DockManager::makeWidgetDockVisible | ( | QWidget * | w | ) |
Works like makeDockVisible() but can be called for widgets that covered by a dockwidget.
- Parameters
-
w the widget that is encapsulated by a dockwidget that turns to visible.
Definition at line 3076 of file k3dockwidget.cpp.
Like writeConfig but reads the whole stuff in.
In order to restore a window configuration from a config file, it looks up widgets by name (QObject::name) in the childDock variable of K3DockManager. This list in turn contains all K3DockWidgets (according to the K3DockWidget constructor). So in principle, in order to restore a window layout, one must first construct all widgets, put each of them in a K3DockWidget and then call readConfig(). And for all that to work, each widget must have a unique name.
Definition at line 2788 of file k3dockwidget.cpp.
void K3DockManager::readConfig | ( | QDomElement & | base | ) |
Reads the current dock window layout from a DOM tree below the given element.
Definition at line 2423 of file k3dockwidget.cpp.
void K3DockManager::removeFromAutoCreateList | ( | K3DockWidget * | pDockWidget | ) |
Definition at line 2621 of file k3dockwidget.cpp.
|
signal |
Signals a dockwidget is replaced with another one.
|
signal |
Signals a dockwidget without parent (toplevel) is shown.
void K3DockManager::setMainDockWidget2 | ( | K3DockWidget * | w | ) |
Definition at line 1780 of file k3dockwidget.cpp.
void K3DockManager::setReadDockConfigMode | ( | int | mode | ) |
Definition at line 2633 of file k3dockwidget.cpp.
void K3DockManager::setSpecialBottomDockContainer | ( | K3DockWidget * | container | ) |
Definition at line 3161 of file k3dockwidget.cpp.
void K3DockManager::setSpecialLeftDockContainer | ( | K3DockWidget * | container | ) |
Definition at line 3148 of file k3dockwidget.cpp.
void K3DockManager::setSpecialRightDockContainer | ( | K3DockWidget * | container | ) |
Definition at line 3156 of file k3dockwidget.cpp.
void K3DockManager::setSpecialTopDockContainer | ( | K3DockWidget * | container | ) |
Definition at line 3152 of file k3dockwidget.cpp.
void K3DockManager::setSplitterHighResolution | ( | bool | b = true | ) |
Operate the splitter with a higher resolution.
Off by default. Call this method before you create any dock widgets! If high resolution is used all splitter position parameters are percent*100 instead of percent.
- Note
- Since KDE 3.5 this is ignored. Internally the splitter always calcualtes in high resolution values. For KDE 4, this will be removed.
Definition at line 3024 of file k3dockwidget.cpp.
void K3DockManager::setSplitterKeepSize | ( | bool | b = true | ) |
Try to preserve the widget's size.
Works like KeepSize resize mode of QSplitter. Off by default. Call this method before you create any dock widgets!
Definition at line 3014 of file k3dockwidget.cpp.
void K3DockManager::setSplitterOpaqueResize | ( | bool | b = true | ) |
Enables opaque resizing.
Opaque resizing defaults to KGlobalSettings::opaqueResize(). Call this method before you create any dock widgets!
Definition at line 3004 of file k3dockwidget.cpp.
bool K3DockManager::splitterHighResolution | ( | ) | const |
Returns true if the splitter uses the high resolution, false otherwise.
Definition at line 3029 of file k3dockwidget.cpp.
bool K3DockManager::splitterKeepSize | ( | ) | const |
Returns true if the KeepSize is enabled, false otherwise.
Definition at line 3019 of file k3dockwidget.cpp.
bool K3DockManager::splitterOpaqueResize | ( | ) | const |
Returns true if opaque resizing is enabled, false otherwise.
Definition at line 3009 of file k3dockwidget.cpp.
|
protectedvirtual |
Definition at line 3460 of file k3dockwidget.cpp.
Saves the current state of the dockmanager and of all controlled widgets.
State means here to save the geometry, visibility, parents, internal object names, orientation, separator positions, dockwidget-group information, tab widget states (if it is a tab group) and last but not least some necessary things for recovering the dockmainwindow state.
Definition at line 2639 of file k3dockwidget.cpp.
void K3DockManager::writeConfig | ( | QDomElement & | base | ) |
Saves the current dock window layout into a DOM tree below the given element.
Definition at line 2307 of file k3dockwidget.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:51:59 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.