KDE3Support
#include <k3popupmenu.h>
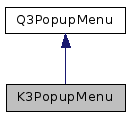
Signals | |
void | aboutToShowContextMenu (K3PopupMenu *menu, QAction *menuAction, QMenu *ctxMenu) |
void | aboutToShowContextMenu (K3PopupMenu *menu, int menuItem, Q3PopupMenu *ctxMenu) |
Public Member Functions | |
K3PopupMenu (QWidget *parent=0) | |
K3PopupMenu (const QString &title, QWidget *parent=0) | |
~K3PopupMenu () | |
virtual void | activateItemAt (int index) |
QAction * | addTitle (const QString &text, QAction *before=0L) |
QAction * | addTitle (const QIcon &icon, const QString &text, QAction *before=0L) |
void | changeTitle (int id, const QString &text) |
void | changeTitle (int id, const QPixmap &icon, const QString &text) |
Q3PopupMenu * | contextMenu () |
const Q3PopupMenu * | contextMenu () const |
void | hideContextMenu () |
int | insertTitle (const QString &text, int id=-1, int index=-1) |
int | insertTitle (const QPixmap &icon, const QString &text, int id=-1, int index=-1) |
Qt::KeyboardModifiers | keyboardModifiers () const |
Qt::MouseButtons | mouseButtons () const |
void | setKeyboardShortcutsEnabled (bool enable) |
void | setKeyboardShortcutsExecute (bool enable) |
void | setTitle (const QString &title) |
Qt::ButtonState | state () const |
QString | title (int id=-1) const |
QPixmap | titlePixmap (int id) const |
Static Public Member Functions | |
static int | actionId (QAction *action) |
static K3PopupMenu * | contextMenuFocus () |
static QAction * | contextMenuFocusAction () |
static int | contextMenuFocusItem () |
Protected Slots | |
void | actionHovered (QAction *action) |
void | ctxMenuHideShowingMenu () |
void | ctxMenuHiding () |
void | resetKeyboardVars (bool noMatches=false) |
void | showCtxMenu (const QPoint &pos) |
QString | underlineText (const QString &text, uint length) |
Protected Member Functions | |
virtual void | closeEvent (QCloseEvent *) |
virtual void | contextMenuEvent (QContextMenuEvent *e) |
virtual bool | focusNextPrevChild (bool next) |
virtual void | hideEvent (QHideEvent *) |
virtual void | keyPressEvent (QKeyEvent *e) |
virtual void | mousePressEvent (QMouseEvent *e) |
virtual void | mouseReleaseEvent (QMouseEvent *e) |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
A menu with keyboard searching and convenience methods for title items.
K3PopupMenu is a compatibility class for KPopupMenu from KDE 3. It provides menus with standard title items and keyboard accessibility for popups with many options and/or varying options. It acts identically to QMenu, with the addition of insertTitle(), changeTitle(), setKeyboardShortcutsEnabled() and setKeyboardShortcutsExecute() methods.
The titles support a text string and an icon.
The keyboard search algorithm is incremental with additional underlining for user feedback.
Definition at line 49 of file k3popupmenu.h.
Constructor & Destructor Documentation
K3PopupMenu::K3PopupMenu | ( | QWidget * | parent = 0 | ) |
Constructs a K3PopupMenu.
Definition at line 88 of file k3popupmenu.cpp.
K3PopupMenu::~K3PopupMenu | ( | ) |
Destructs the object.
Definition at line 96 of file k3popupmenu.cpp.
- Deprecated:
- Obsolete method provided for backwards compatibility only. Use the normal constructor and insertTitle instead.
Definition at line 547 of file k3popupmenu.cpp.
Member Function Documentation
|
signal |
connect to this signal to be notified when a context menu is about to be shown
- Parameters
-
menu The menu that the context menu is about to be shown for menuAction The action that the context menu is currently on ctxMenu The context menu itself
|
signal |
compat
|
protectedslot |
Definition at line 434 of file k3popupmenu.cpp.
|
inlinestatic |
Helper for porting things.
Returns ID of action, or -1 if passed null.
KDE4: should be able to remove on Monday
Definition at line 194 of file k3popupmenu.h.
|
virtual |
Reimplemented for internal purposes.
Definition at line 140 of file k3popupmenu.cpp.
Inserts a title item with no icon.
Definition at line 108 of file k3popupmenu.cpp.
Inserts a title item with the given icon and title.
Definition at line 119 of file k3popupmenu.cpp.
void K3PopupMenu::changeTitle | ( | int | id, |
const QString & | text | ||
) |
Changes the title of the item at the specified id.
If a icon was previously set it is cleared.
Definition at line 582 of file k3popupmenu.cpp.
void K3PopupMenu::changeTitle | ( | int | id, |
const QPixmap & | icon, | ||
const QString & | text | ||
) |
Changes the title and icon of the title item at the specified id.
Definition at line 593 of file k3popupmenu.cpp.
|
protectedvirtual |
This is re-implemented for keyboard navigation.
Definition at line 133 of file k3popupmenu.cpp.
Q3PopupMenu * K3PopupMenu::contextMenu | ( | ) |
Returns the context menu associated with this menu.
Definition at line 403 of file k3popupmenu.cpp.
const Q3PopupMenu * K3PopupMenu::contextMenu | ( | ) | const |
Returns the context menu associated with this menu.
Definition at line 414 of file k3popupmenu.cpp.
|
protectedvirtual |
Definition at line 501 of file k3popupmenu.cpp.
|
static |
Returns the K3PopupMenu associated with the current context menu.
Definition at line 429 of file k3popupmenu.cpp.
|
static |
returns the QAction associated with the current context menu
Definition at line 424 of file k3popupmenu.cpp.
|
static |
returns the ID of the menuitem associated with the current context menu
Definition at line 627 of file k3popupmenu.cpp.
|
protectedslot |
Definition at line 484 of file k3popupmenu.cpp.
|
protectedslot |
Definition at line 491 of file k3popupmenu.cpp.
Definition at line 330 of file k3popupmenu.cpp.
void K3PopupMenu::hideContextMenu | ( | ) |
Hides the context menu if shown.
Definition at line 419 of file k3popupmenu.cpp.
|
protectedvirtual |
Definition at line 521 of file k3popupmenu.cpp.
int K3PopupMenu::insertTitle | ( | const QString & | text, |
int | id = -1 , |
||
int | index = -1 |
||
) |
Inserts a title item with no icon.
Definition at line 557 of file k3popupmenu.cpp.
int K3PopupMenu::insertTitle | ( | const QPixmap & | icon, |
const QString & | text, | ||
int | id = -1 , |
||
int | index = -1 |
||
) |
Inserts a title item with the given icon and title.
Definition at line 569 of file k3popupmenu.cpp.
Qt::KeyboardModifiers K3PopupMenu::keyboardModifiers | ( | ) | const |
Return the state of the keyboard modifiers when the last menuitem was activated.
Definition at line 162 of file k3popupmenu.cpp.
|
protectedvirtual |
Definition at line 167 of file k3popupmenu.cpp.
Qt::MouseButtons K3PopupMenu::mouseButtons | ( | ) | const |
Return the state of the mouse buttons when the last menuitem was activated.
Definition at line 157 of file k3popupmenu.cpp.
|
protectedvirtual |
|
protectedvirtual |
Definition at line 389 of file k3popupmenu.cpp.
|
protectedslot |
Definition at line 346 of file k3popupmenu.cpp.
void K3PopupMenu::setKeyboardShortcutsEnabled | ( | bool | enable | ) |
Enables keyboard navigation by searching for the entered key sequence.
Also underlines the currently selected item, providing feedback on the search.
Defaults to off.
WARNING: calls to text() of currently keyboard-selected items will contain additional ampersand characters.
WARNING: though pre-existing keyboard shortcuts will not interfere with the operation of this feature, they may be confusing to the user as the existing shortcuts will not work.
Definition at line 361 of file k3popupmenu.cpp.
void K3PopupMenu::setKeyboardShortcutsExecute | ( | bool | enable | ) |
Enables execution of the menu item once it is uniquely specified.
Defaults to off.
Definition at line 366 of file k3popupmenu.cpp.
void K3PopupMenu::setTitle | ( | const QString & | title | ) |
- Deprecated:
- Obsolete method provided for backwards compatibility only. Use insertTitle and changeTitle instead.
Definition at line 622 of file k3popupmenu.cpp.
|
protectedslot |
Definition at line 445 of file k3popupmenu.cpp.
Qt::ButtonState K3PopupMenu::state | ( | ) | const |
Return the state of the mouse button and keyboard modifiers when the last menuitem was activated.
Definition at line 151 of file k3popupmenu.cpp.
QString K3PopupMenu::title | ( | int | id = -1 | ) | const |
Returns the title of the title item at the specified id.
The default id of -1 is for backwards compatibility only, you should always specify the id.
Definition at line 604 of file k3popupmenu.cpp.
QPixmap K3PopupMenu::titlePixmap | ( | int | id | ) | const |
Returns the icon of the title item at the specified id.
Definition at line 613 of file k3popupmenu.cpp.
Definition at line 336 of file k3popupmenu.cpp.
|
protectedvirtual |
end of RMB menus on menus support
Definition at line 543 of file k3popupmenu.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:51:59 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.