KUnitTest
#include <runner.h>
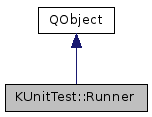
Public Slots | |
void | reset () |
void | runMatchingTests (const QString &prefix) |
void | runTest (const char *name) |
int | runTests () |
Signals | |
void | finished (const char *name, Tester *test) |
void | invoke () |
Public Member Functions | |
int | numberOfExpectedFailures () const |
int | numberOfFailedTests () const |
int | numberOfPassedTests () const |
int | numberOfSkippedTests () const |
int | numberOfTestCases () |
int | numberOfTests () const |
Registry & | registry () |
Static Public Member Functions | |
static void | loadModules (const QString &folder, const QString &query) |
static void | registerTester (const char *name, Tester *test) |
static Runner * | self () |
static void | setDebugCapturingEnabled (bool enabled) |
Protected Member Functions | |
Runner () | |
Detailed Description
The Runner class holds a list of registered Tester classes and is able to run those test cases. The Runner class follows the singleton design pattern, which means that you can only have one Runner instance. This instance can be retrieved using the Runner::self() method.
The registry is an object of type Registry, it is able to map the name of a test to a pointer to a Tester object. The registry is also a singleton and can be accessed via Runner::registry(). Since there is only one registry, which can be accessed at all times, test cases can be added without having to worry if a Runner instance is present or not. This allows for a design in which the KUnitTest library can be kept separate from the test case sources. Test cases (classes inheriting from Tester) can be added using the static registerTester(const char *name, Tester *test) method. Allthough most users will want to use the KUNITTEST_REGISTER_TESTER macro.
- See also
- KUNITTEST_REGISTER_TESTER
Constructor & Destructor Documentation
|
protected |
Definition at line 117 of file runner.cpp.
Member Function Documentation
|
signal |
Emitted after a test is finished.
- Parameters
-
name The name of the test. test A pointer to the Tester object.
|
signal |
Load all modules found in the folder.
- Parameters
-
folder The folder where to look for modules. query A regular expression. Only modules which match the query will be run.
Definition at line 56 of file runner.cpp.
int KUnitTest::Runner::numberOfExpectedFailures | ( | ) | const |
- Returns
- The number of failed tests which were expected.
Definition at line 137 of file runner.cpp.
int KUnitTest::Runner::numberOfFailedTests | ( | ) | const |
- Returns
- The number of failed tests, this includes the number of expected failures.
Definition at line 132 of file runner.cpp.
int KUnitTest::Runner::numberOfPassedTests | ( | ) | const |
- Returns
- The number of passed tests.
Definition at line 127 of file runner.cpp.
int KUnitTest::Runner::numberOfSkippedTests | ( | ) | const |
- Returns
- The number of skipped tests.
Definition at line 142 of file runner.cpp.
int KUnitTest::Runner::numberOfTestCases | ( | ) |
- Returns
- The number of registered test cases.
Definition at line 103 of file runner.cpp.
int KUnitTest::Runner::numberOfTests | ( | ) | const |
- Returns
- The number of finished tests.
Definition at line 122 of file runner.cpp.
|
static |
Registers a test case. A registry will be automatically created if necessary.
- Parameters
-
name The name of the test case. test A pointer to a Tester object.
Definition at line 51 of file runner.cpp.
Registry & KUnitTest::Runner::registry | ( | ) |
- Returns
- The registry holding all the Tester objects.
Definition at line 98 of file runner.cpp.
|
slot |
Reset the Runner in order to prepare it to run one or more tests again.
Definition at line 147 of file runner.cpp.
|
slot |
Call this slot to run tests with names starting with prefix.
- Parameters
-
prefix Only run tests starting with the string prefix.
Definition at line 202 of file runner.cpp.
|
slot |
Call this slot to run a single test.
- Parameters
-
name The name of the test case. This name has to correspond to the name that was used to register the test. If the KUNITTEST_REGISTER_TESTER macro was used to register the test case then this name is the class name.
Definition at line 210 of file runner.cpp.
|
slot |
Call this slot to run all the registered tests.
- Returns
- The number of finished tests.
Definition at line 157 of file runner.cpp.
|
static |
- Returns
- The global Runner instance. If necessary an instance will be created.
Definition at line 108 of file runner.cpp.
|
static |
The runner can spit out special debug messages needed by the Perl script: kunittest_debughelper. This script can attach the debug output of each suite to the results in the KUnitTest GUI. Not very useful for console minded developers, so this static method can be used to disable those debug messages.
- Parameters
-
enabled If true the debug messages are enabled (default), otherwise they are disabled.
Definition at line 92 of file runner.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:07 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.