KDEsu
#include <kdesu/process.h>
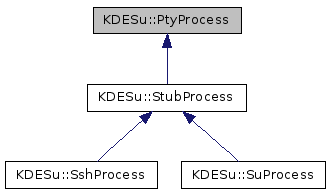
Public Types | |
enum | checkPidStatus { Error =-1, NotExited =-2, Killed =-3 } |
Public Member Functions | |
PtyProcess () | |
virtual | ~PtyProcess () |
int | enableLocalEcho (bool enable=true) |
int | exec (const QByteArray &command, const QList< QByteArray > &args) |
int | fd () const |
int | pid () const |
QByteArray | readAll (bool block=true) |
QByteArray | readLine (bool block=true) |
void | setEnvironment (const QList< QByteArray > &env) |
void | setErase (bool erase) |
void | setExitString (const QByteArray &exit) |
void | setTerminal (bool terminal) |
void | unreadLine (const QByteArray &line, bool addNewline=true) |
int | waitForChild () |
int | WaitSlave () |
void | writeLine (const QByteArray &line, bool addNewline=true) |
Static Public Member Functions | |
static bool | checkPid (pid_t pid) |
static int | checkPidExited (pid_t pid) |
Public Attributes | |
public | __pad0__: static int waitMS(int fd |
public int | ms |
Protected Member Functions | |
QList< QByteArray > | environment () const |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
bool | m_bErase |
bool | m_bTerminal |
QByteArray | m_Command |
QByteArray | m_Exit |
int | m_Pid |
Detailed Description
Synchronous communication with tty programs.
PtyProcess provides synchronous communication with tty based programs. The communications channel used is a pseudo tty (as opposed to a pipe) This means that programs which require a terminal will work.
Member Enumeration Documentation
Error return values for checkPidExited()
Enumerator | |
---|---|
Error |
No child. |
NotExited |
Child hasn't exited. |
Killed |
Child terminated by signal. |
Constructor & Destructor Documentation
KDESu::PtyProcess::PtyProcess | ( | ) |
Definition at line 137 of file process.cpp.
|
virtual |
Definition at line 159 of file process.cpp.
Member Function Documentation
|
static |
Basic check for the existence of pid
.
Returns true iff pid
is an extant process, (one you could kill - see man kill(2) for signal 0).
Definition at line 81 of file process.cpp.
|
static |
Check process exit status for process pid
.
If child pid
has exited, return its exit status, (which may be zero). On error (no child, no exit), return -1. If child has
not exited, return -2.
Definition at line 102 of file process.cpp.
int KDESu::PtyProcess::enableLocalEcho | ( | bool | enable = true | ) |
Enables/disables local echo on the pseudo tty.
Definition at line 406 of file process.cpp.
|
protected |
Returns the additional environment variables set by setEnvironment()
Definition at line 181 of file process.cpp.
int KDESu::PtyProcess::exec | ( | const QByteArray & | command, |
const QList< QByteArray > & | args | ||
) |
Forks off and execute a command.
The command's standard in and output are connected to the pseudo tty. They are accessible with readLine and writeLine.
- Parameters
-
command The command to execute. args The arguments to the command.
- Returns
- 0 on success, -1 on error. errno might give more information then.
Definition at line 291 of file process.cpp.
int KDESu::PtyProcess::fd | ( | ) | const |
Returns the filedescriptor of the process.
Definition at line 170 of file process.cpp.
int KDESu::PtyProcess::pid | ( | ) | const |
Returns the pid of the process.
Definition at line 175 of file process.cpp.
QByteArray KDESu::PtyProcess::readAll | ( | bool | block = true | ) |
Read all available output from the program's standard out.
- Parameters
-
block If no output is in the buffer, should the function block (else it will return an empty QByteArray)?
- Returns
- The output.
Definition at line 187 of file process.cpp.
QByteArray KDESu::PtyProcess::readLine | ( | bool | block = true | ) |
Reads a line from the program's standard out.
Depending on the block parameter, this call blocks until something was read. Note that in some situations this function will return less than a full line of output, but never more. Newline characters are stripped.
- Parameters
-
block Block until a full line is read?
- Returns
- The output string.
Definition at line 239 of file process.cpp.
void KDESu::PtyProcess::setEnvironment | ( | const QList< QByteArray > & | env | ) |
Set additinal environment variables.
Set additional environment variables.
Definition at line 165 of file process.cpp.
void KDESu::PtyProcess::setErase | ( | bool | erase | ) |
Overwrites the password as soon as it is used.
Relevant only to some subclasses.
Definition at line 417 of file process.cpp.
void KDESu::PtyProcess::setExitString | ( | const QByteArray & | exit | ) |
Sets the exit string.
If a line of program output matches this, waitForChild() will terminate the program and return.
Definition at line 282 of file process.cpp.
void KDESu::PtyProcess::setTerminal | ( | bool | terminal | ) |
Enables/disables terminal output.
Relevant only to some subclasses.
Definition at line 412 of file process.cpp.
void KDESu::PtyProcess::unreadLine | ( | const QByteArray & | line, |
bool | addNewline = true |
||
) |
Puts back a line of input.
- Parameters
-
line The line to put back. addNewline Adds a '
' to the line.
Definition at line 273 of file process.cpp.
|
protectedvirtual |
Standard hack to add virtual methods in a BC way.
Unused.
Reimplemented in KDESu::StubProcess, KDESu::SshProcess, and KDESu::SuProcess.
Definition at line 554 of file process.cpp.
int KDESu::PtyProcess::waitForChild | ( | ) |
int KDESu::PtyProcess::WaitSlave | ( | ) |
Waits until the pty has cleared the ECHO flag.
This is useful when programs write a password prompt before they disable ECHO. Disabling it might flush any input that was written.
Definition at line 377 of file process.cpp.
void KDESu::PtyProcess::writeLine | ( | const QByteArray & | line, |
bool | addNewline = true |
||
) |
Writes a line of text to the program's standard in.
- Parameters
-
line The text to write. addNewline Adds a '
' to the line.
Definition at line 264 of file process.cpp.
Member Data Documentation
|
protected |
- See also
- setErase()
|
protected |
Indicates running in a terminal, causes additional newlines to be printed after output.
Set to false
in constructor.
- See also
- setTerminal()
|
protected |
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:48 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.