KFile
#include <kfilefiltercombo.h>
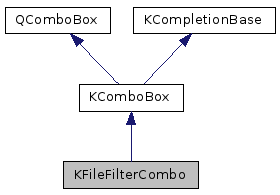
Signals | |
void | filterChanged () |
![]() | |
void | aboutToShowContextMenu (QMenu *p) |
void | completion (const QString &) |
void | completionModeChanged (KGlobalSettings::Completion) |
void | returnPressed (const QString &) |
void | returnPressed () |
void | substringCompletion (const QString &) |
void | textRotation (KCompletionBase::KeyBindingType) |
Public Member Functions | |
KFileFilterCombo (QWidget *parent=0) | |
~KFileFilterCombo () | |
QString | currentFilter () const |
QString | defaultFilter () const |
QStringList | filters () const |
bool | isMimeFilter () const |
void | setCurrentFilter (const QString &filter) |
void | setDefaultFilter (const QString &filter) |
void | setFilter (const QString &filter) |
void | setMimeFilter (const QStringList &types, const QString &defaultType) |
bool | showsAllTypes () const |
![]() | |
KComboBox (QWidget *parent=0) | |
KComboBox (bool rw, QWidget *parent=0) | |
virtual | ~KComboBox () |
virtual | ~KCompletionBase () |
void | addUrl (const KUrl &url) |
void | addUrl (const QIcon &icon, const KUrl &url) |
bool | autoCompletion () const |
void | changeURL (const QPixmap &pixmap, const KUrl &url, int index) |
void | changeUrl (int index, const KUrl &url) |
void | changeUrl (int index, const QIcon &icon, const KUrl &url) |
void | changeURL (const KUrl &url, int index) |
KCompletionBox * | completionBox (bool create=true) |
KGlobalSettings::Completion | completionMode () const |
KCompletion * | completionObject (bool hsig=true) |
KCompletion * | compObj () const |
bool | contains (const QString &text) const |
int | cursorPosition () const |
bool | emitSignals () const |
KShortcut | getKeyBinding (KeyBindingType item) const |
bool | handleSignals () const |
void | insertURL (const QPixmap &pixmap, const KUrl &url, int index=-1) |
void | insertUrl (int index, const QIcon &icon, const KUrl &url) |
void | insertUrl (int index, const KUrl &url) |
void | insertURL (const KUrl &url, int index=-1) |
bool | isCompletionObjectAutoDeleted () const |
KCompletionBase () | |
virtual void | setAutoCompletion (bool autocomplete) |
void | setAutoDeleteCompletionObject (bool autoDelete) |
virtual void | setCompletedItems (const QStringList &items, bool autoSuggest=true)=0 |
virtual void | setCompletedText (const QString &text)=0 |
virtual void | setCompletionMode (KGlobalSettings::Completion mode) |
virtual void | setCompletionObject (KCompletion *compObj, bool hsig=true) |
virtual void | setContextMenuEnabled (bool showMenu) |
void | setEditable (bool editable) |
void | setEditUrl (const KUrl &url) |
void | setEnableSignals (bool enable) |
virtual void | setHandleSignals (bool handle) |
bool | setKeyBinding (KeyBindingType item, const KShortcut &key) |
virtual void | setLineEdit (QLineEdit *) |
void | setTrapReturnKey (bool trap) |
void | setUrlDropsEnabled (bool enable) |
bool | trapReturnKey () const |
bool | urlDropsEnabled () const |
void | useGlobalKeyBindings () |
Protected Member Functions | |
virtual bool | eventFilter (QObject *, QEvent *) |
![]() | |
virtual void | create (WId=0, bool initializeWindow=true, bool destroyOldWindow=true) |
KCompletionBase * | delegate () const |
KeyBindingMap | getKeyBindings () const |
virtual QSize | minimumSizeHint () const |
virtual void | setCompletedText (const QString &, bool) |
void | setDelegate (KCompletionBase *delegate) |
virtual void | virtual_hook (int id, void *data) |
virtual void | wheelEvent (QWheelEvent *ev) |
Additional Inherited Members | |
![]() | |
typedef QMap< KeyBindingType, KShortcut > | KeyBindingMap |
enum | KeyBindingType |
![]() | |
void | rotateText (KCompletionBase::KeyBindingType type) |
void | setCompletedItems (const QStringList &items, bool autosubject=true) |
virtual void | setCompletedText (const QString &) |
void | setCurrentItem (const QString &item, bool insert=false, int index=-1) |
![]() | |
virtual void | makeCompletion (const QString &) |
![]() | |
bool | autoCompletion |
bool | trapReturnKey |
bool | urlDropsEnabled |
Detailed Description
Definition at line 29 of file kfilefiltercombo.h.
Constructor & Destructor Documentation
KFileFilterCombo::KFileFilterCombo | ( | QWidget * | parent = 0 | ) |
Creates a new filter combo box.
- Parameters
-
parent The parent widget.
Definition at line 57 of file kfilefiltercombo.cpp.
KFileFilterCombo::~KFileFilterCombo | ( | ) |
Destroys the filter combo box.
Definition at line 68 of file kfilefiltercombo.cpp.
Member Function Documentation
QString KFileFilterCombo::currentFilter | ( | ) | const |
- Returns
- the current filter, either something like "*.cpp *.h" or the current mimetype, like "text/html", or a list of those, like " "text/html text/plain image/png", all separated with one space.
Definition at line 104 of file kfilefiltercombo.cpp.
QString KFileFilterCombo::defaultFilter | ( | ) | const |
- Returns
- the default filter, used when an empty filter is set.
- See also
- setDefaultFilter
Definition at line 222 of file kfilefiltercombo.cpp.
Reimplemented from KComboBox.
Definition at line 207 of file kfilefiltercombo.cpp.
|
signal |
This signal is emitted whenever the filter has been changed.
QStringList KFileFilterCombo::filters | ( | ) | const |
- Returns
- all filters (this can be a list of patterns or a list of mimetypes)
Definition at line 126 of file kfilefiltercombo.cpp.
bool KFileFilterCombo::isMimeFilter | ( | ) | const |
Returns true if the filter has been set using setMimeFilter().
- Since
- 4.6.1
Definition at line 227 of file kfilefiltercombo.cpp.
void KFileFilterCombo::setCurrentFilter | ( | const QString & | filter | ) |
Sets the current filter.
Filter must match one of the filter items passed before to this widget.
Definition at line 131 of file kfilefiltercombo.cpp.
void KFileFilterCombo::setDefaultFilter | ( | const QString & | filter | ) |
This method allows you to set a default-filter, that is used when an empty filter is set.
Make sure you call this before calling setFilter().
By default, this is set to i18n("*|All Files")
- See also
- defaultFilter
Definition at line 217 of file kfilefiltercombo.cpp.
void KFileFilterCombo::setFilter | ( | const QString & | filter | ) |
Sets the filter
string.
Definition at line 73 of file kfilefiltercombo.cpp.
void KFileFilterCombo::setMimeFilter | ( | const QStringList & | types, |
const QString & | defaultType | ||
) |
Sets a list of mimetypes.
If defaultType
is set, it will be set as the current item. Otherwise, a first item showing all the mimetypes will be created.
Definition at line 137 of file kfilefiltercombo.cpp.
bool KFileFilterCombo::showsAllTypes | ( | ) | const |
- Returns
- true if the filter's first item is the list of all mimetypes
Definition at line 121 of file kfilefiltercombo.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.